IO stream
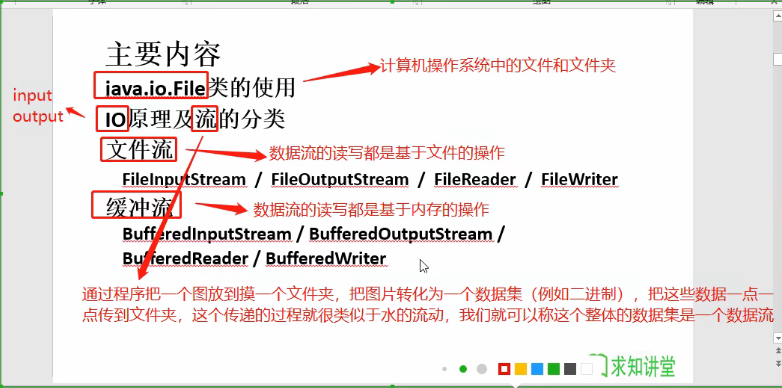
-
primary coverage
-
java. io. Use of File class (File: files and folders in the computer operating system)
-
File class
- java.io.Filelei: abstract representation of file and directory pathnames, which is platform independent
- File can create, delete and rename files and directories, but file cannot access the file content itself. If you need to access the file content itself, you need to use input / output streams.
- The File object can be passed as a parameter to the constructor of the stream
- Common construction methods of File class:
- public File(String pathname)
- Create a File object with pathname as the path, which can be an absolute path or a relative path. If pathname is a relative path, the default current path is in the system attribute user Stored in dir
- public File(String parent,String child)
- Create a File object with parent as the parent path and child as the child path
- The static property String separator of File stores the path separator of the current system
- This field is' / 'in UNIX and' \ \ 'in Windows
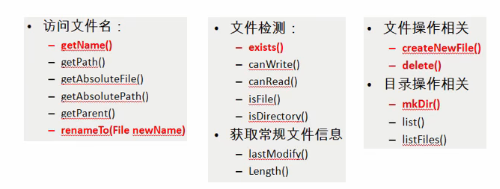
import java.io.File;
public class FileDemo {
public static void main(String[] args) {
//At this time, the object f is TT Txt file
File f = new File("D:\\desktop\\java\\test\\tt.txt");
File f2 = new File("D:\\desktop\\java\\test");
//File f2 = new File("D:"+File.separator + "desktop \ \ java\test\tt.txt");
// File f1 = new File("D: \ \ desktop \ \ java","test\tt.txt");
//Note that \ is the separator of the path in the file, but in java programming, a \ means the conversion character, and in java, \ \ or / is the separator of the file
//You can also file Separator as file separator
//Obtaining the file name is indeed the last level of obtaining the path
System.out.println(f.getName());//tt.txt
System.out.println(f2.getName());//test
//Get current path
System.out.println(f.getPath());//D: \ desktop \ Java \ test \ TT txt
//Output full path through relative path
File f5 = new File("java daima\\src\\OOP\\IO\\FileDemo.Java");//Use relative paths to create File objects
System.out.println(f5.getAbsolutePath());//D:\IDEA\java daima\java daima\src\OOP\IO\FileDemo.java
System.out.println(f5.getPath());//java daima\src\OOP\IO\FileDemo.java
//Returns a file object constructed by the absolute path of the current file
System.out.println(f5);//java daima\src\OOP\IO\FileDemo.java
System.out.println(f5.getAbsoluteFile());//D:\IDEA\java daima\java daima\src\OOP\IO\FileDemo.Java
//Returns the parent path of the current file or folder
System.out.println(f.getParent());
//Rename the file represented by this abstract pathname. It can only be done once
//f. Rename to (new file ("D: \ \ desktop \ \ java\test\hh.txt");
File file = new File("D:\\desktop\\java\\test\\hh.txt");
f.renameTo(file);
//Determine whether the folder or folder exists
System.out.println(file.exists());
//Determine whether the file is readable,
System.out.println(file.canRead());
System.out.println(file.canWrite());//Judge whether the file is writable
System.out.println(file.isFile());//Judge whether the File object is a File
System.out.println(file.isDirectory());//Judge whether the current File object is a folder
System.out.println(file.lastModified());//Gets the last modification time of the file, and returns a millisecond
System.out.println(file.length());//Returns the length of the file in bytes
File file1 = new File("D:\\desktop\\java\\test\\zz.txt");
// if (!file1.exists()) {
// try {
// file1.createNewFile();// Create a new file
// } catch (IOException e) {
// e.printStackTrace();
// }
// }
// file1.delete();// Delete file
File file2 = new File("D:\\desktop\\java\\test\\hzc");
if (!file2.exists()) {
file2.mkdir();//Create a single tier directory
}
file2.delete();
File file3 = new File("D:\\desktop\\java\\test\\hzc\\13\\22");
if (!file3.exists()) {
file3.mkdirs();//Create multi tier directory
}
System.out.println("==========");
String[] s = file2.list();//Output the files and file names in this directory
for (String x:s) {
System.out.println(x);
}
File[] a = file2.listFiles();//Returns an abstract path name array, and outputs the path of the file and the path of the file name in this directory.
for (File x:a
) {
System.out.println(x);
}
}
}
Output a path and traverse all directories and files, no matter how deep the level is
import java.io.File;
public class FileTest {
//Traverse the test files under the desktop, and traverse all directories and files under the test folder. No matter how deep the level is, traverse them all
//Use recursion
public static void main(String[] args) {
File file = new File("D:\\desktop\\java\\test");
new FileTest().test(file);
}
public void test(File file){
if (file.isFile() ) {
System.out.println(file.getAbsolutePath()+"It's a file");
}else{
System.out.println(file.getAbsolutePath()+"It's a folder");
//If it is a folder, there may be subdirectories
File[] file1 = file.listFiles();
if (file1 != null && file1.length>0) {
for (File x:file1) {
test(x);
}
}
}
}
}
-
IO principle and stream classification
-
Java IO principle
- The IO stream is used to process data transmission between devices
- In Java program, the input / output operation of data is carried out in the way of "stream"
- Java.IO package provides various "flow" classes and interfaces to obtain different kinds of data and provide standard methods to input or output data
- input: read external data (data from disk, optical disc and other storage devices) into the program (memory).
- Output: output program (memory) data to disk, optical disc and other storage devices
-
Stream classification
- According to different operation data units, it is divided into byte stream (8 bit) and character stream (16 bit)
- According to the flow direction of data flow, it is divided into input flow and output flow
- According to the different roles of flow, it can be divided into node flow and processing flow
- 1. Byte stream: all files can be read
- **2. It is convenient to read plain text files, which has helped us deal with the problem of garbled code**
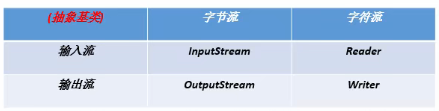
- Java's IO stream involves more than 40 classes, which are actually very regular. They are derived from the following four abstract base classes The names of subclasses derived from these four classes are suffixed with their parent class names
-
File stream
- FileInputStream / FileOutputStream / FileReader / FileWriter-
- Byte input stream (FileInputStream)
import java.io.File;
import java.io.FileInputStream;
public class FileInputStreamDemo {
public static void main(String[] args) {
//1. Pipeline between documents and procedures
try {
// FileInputStream fis = new FileInputStream("D: \ \ desktop \ \ java\test\hh.txt");
//The following method is better, because you can judge whether the file exists. If it does not exist, you do not need to execute the following code to reduce the occurrence of errors
File file = new File("D:\\desktop\\java\\test\\hh.txt");
//Judge whether the file exists and whether the information already exists. The bytecode is not 0
if (file.exists() && file.length()>0 ) {
FileInputStream fileInputStream = new FileInputStream(file);
int ch = 0;
byte[] shu = new byte[10];
//The data read by read() will be converted into int data. When the method reads the last character, it will return - 1, which means the end
// while((ch=fileInputStream.read())!=-1){
// //The output int character needs to be converted into Unicode character
// System.out.print((char)ch);
// }
//It should be noted that the read() method reads one byte by one, while Chinese takes up two bytes, so fileInputStream cannot read Chinese, and the read data will become garbled
while((ch=fileInputStream.read(shu))!=-1){
//The output int character needs to be converted into Unicode character
System.out.print(new String(shu,0,ch));
}
//Remember to turn off the IO stream
fileInputStream.close();
}
} catch (Exception e) {
System.out.println("File not found");
}
}
}
import java.io.FileOutputStream;
public class FileOutputStreamDemo {
public static void main(String[] args) {
try {
//1. Create a water plant
String data = "hello world java";
//Pipe laying procedure to drive letter
//The value of append determines whether the newly added data is overwritten or appended. If it is false, it is overwritten. On the contrary, it is appended
FileOutputStream fis = new FileOutputStream("D:\\desktop\\java\\test\\hzc.txt",false);
//Turn on the tap and drain the water
byte[] bytes= data.getBytes();
fis.write(bytes);
//Turn off the tap
fis.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
Write the information in a file into a new file through code
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
public class IODemo {
public static void main(String[] args) {
//Make a copy of the document
try {
long startTime = System.currentTimeMillis();//Gets the current system time and converts it into milliseconds
System.out.println(startTime);
File file = new File("D:\\desktop\\java\\test\\hh.txt");
if (file.exists() && file.length()>0) {
FileInputStream fis = new FileInputStream(file);
FileOutputStream fos = new FileOutputStream("D:\\desktop\\java\\test\\hzc.txt");
int ch = 0;
while((ch=fis.read())!=-1){
fos.write(ch);
}
fis.close();
fos.close();
long endTime = System.currentTimeMillis();
System.out.println(endTime);
}
} catch (Exception e) {
System.out.println("file does not exist");
}
}
}
-
Buffer stream
- BufferedInputStream / BufferedOutputStream / BufferedReader / BufferedWriter
-
BufferedInputStream
- Use of buffered stream input
import java.io.BufferedInputStream;
import java.io.FileInputStream;
import java.io.InputStream;
public class BufferedInputStreamDemo {
public static void main(String[] args) {
//. water plant (hh.txt)
//. laying pipes
try {
long staryTime = System.currentTimeMillis();
InputStream file = new FileInputStream("D:\\desktop\\java\\test\\hh.txt");
BufferedInputStream bu = new BufferedInputStream(file);
//3. Turn on the tap
//Create an array and store the data you need to input into the array
byte[] cha= new byte[1024];
int len = 0;
while((len=bu.read(cha))!=-1){
System.out.println(len);
}
bu.close();
long endTime = System.currentTimeMillis();
System.out.println("Co consumption"+(endTime-staryTime)+"ms");
} catch (Exception e) {
e.printStackTrace();
}
}
}
// BufferedInputStream stream: byte buffered input stream
//
- Use buffer stream to copy file content
package OOP.IO;
import java.io.*;
public class BufferedOutputStreamDemo {
public static void main(String[] args) {
try {
long statTime = System.currentTimeMillis();
File file1 = new File("D:\\desktop\\java\\test\\hh.txt");
if (file1.exists() && file1.length()>0) {
InputStream file = new FileInputStream("D:\\desktop\\java\\test\\hh.txt");
BufferedInputStream bu = new BufferedInputStream(file);
OutputStream fileOut = new FileOutputStream("D:\\desktop\\java\\test\\hyx.txt");
BufferedOutputStream buff = new BufferedOutputStream(fileOut);
byte[] cha = new byte[1024];
int lea = 0;
//Write the characters in the file into the cha array through read, and return the value to lea
while ((lea = bu.read(cha)) != -1) {
//From subscript 0 to lea characters are written to the buffer byte stream
buff.write(cha,0,lea);
}
bu.close();
buff.close();
long endTime = System.currentTimeMillis();
System.out.println("altogether"+(endTime-statTime)+"ms");
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
-
Conversion flow
- InputStreamReader / OutputStreamWriter
-
Standard input / output stream
-
Print stream
- PrintStream / PrintWriter
-
data stream
- DataInputStream / DataOutputStream
-
Object stream - involves serialization and deserialization
- ObjectInputStream / ObjectOutputStream
-
Random access file stream (random access file stream)
-
Character stream Reader/Writer
-
File character input stream (FileReader)
import java.io.FileReader;
/**
*
*/
public class FileReaderDemo {
public static void main(String[] args) {
try {
long startTime = System.currentTimeMillis();
FileReader fileReader = new FileReader("D:\\desktop\\java\\test\\hh.txt");
char[] car= new char[1024];
int a = 0;
while((a=fileReader.read(car))!=-1){
System.out.println(a);
System.out.print(new String(car,0,a));
}
System.out.println(" ");
long endTime = System.currentTimeMillis();
System.out.println("altogether"+(endTime-startTime)+"ms");
fileReader.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
-
Buffered character input stream (BufferedReader)
-
File character input chu stream (FileWriter)
-
Buffered character output stream (BufferedWriter)