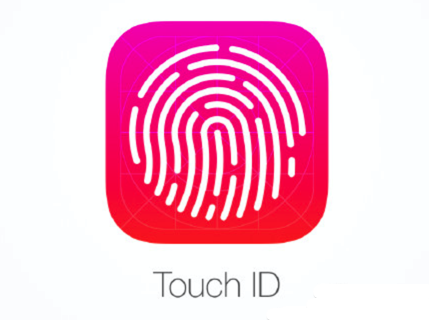
Fingerprint unlocking is becoming more and more popular, mainly for its convenience. Before using it, you should know how to use it.
Introduction to API
Using the fingerprint function, we must use the library of the system. The Library of fingerprint is Local Authentication. framework, so I will introduce the function of all the files in this library.
We opened the Local Authentication. framework library and found four. h files in it.
- LAContext.h - LAError.h - LAPublicDefines.h - LocalAuthentication.h
1,LocalAuthentication.h
There are two lines to introduce the header file. Obviously, this is the class we want to call when we introduce the fingerprint library. We can introduce #import <Local Authentication/Local Authentication.h> in the file.
2,LAPublicDefines.h
This is the definition of some macro definitions, very simple to understand.
3,LAError.h
In fact, this class is not difficult. It is an enumeration which lists all possible error types. Each error type has a comment. Let me translate it for you.
LAErrorAuthenticationFailed //Failure of three consecutive fingerprint verification may result in blurred fingerprints or incorrect fingerprints LAErrorUserCancel //The user canceled the validation and clicked the Cancel button. LAErrorUserFallback //User cancels validation and clicks the Enter Password button LAErrorSystemCancel //The system cancels authorization, such as other APP entry LAErrorPasscodeNotSet //Fingerprint verification cannot start/fail because the device has no password set LAErrorTouchIDNotAvailable //Device TouchID is not available, such as not open LAErrorTouchIDNotEnrolled //Fingerprint verification could not be started because no fingerprints were entered (password set) LAErrorTouchIDLockout //Device TouchID is locked because there are too many failures LAErrorAppCancel //The application cancels authentication, and APP calls the - (void)invalidate method to invalidate the LAContext LAErrorInvalidContext //The instantiated LAContext object fails, and this error message pops up when the evaluation... Method is called again.
4,LAContext.h
This class is the most important core part.
The first thing you see is an enumeration of LAPolicy, which contains two
LAPolicy Device Owner Authentication WithBiometrics (iOS 8 or more available): This represents verification only with fingerprints. When the first fingerprint fails, the "Enter password" button will appear, and the title and function of the input password can be customized; when the third fingerprint fails, the pop-up window disappears; when the verification is started again, there are two opportunities. If both fail, the fingerprint verification lock will no longer pop-up the verification window. Until the password is entered to unlock the fingerprint (the screen can be locked back in and unlocked by entering the password).
LAPolicy Device Owner Authentication (iOS 9 or above): This means that you can use fingerprints or passwords to verify, preferring fingerprints. The first fingerprint failure, there will be a "input password" button, the title of the input password can be customized, but the function can not be customized, but must enter the system password (lock screen password); the third verification failure, pop-up window disappeared, pop-up input system password interface; if five successive fingerprint failure, fingerprint lock, at this time only pop-up input password interface, Until the password is successfully unlocked.
Comparisons of two verification methods:
Similarity: Five consecutive failures in validation result in lock-in
Differences: The former can be customized to input password function, while the latter is fixed input system password function.
The former LAPolicy Device Owner Authentication WithBiometrics is commonly used
Next is the instance method, which creates the instance object: LAContext *context = [LAContext alloc] init]; and calls it with context.
/* This method checks whether the touch ID is available for the current device and returns a BOOL value. policy: This is the above enumeration of the two verification methods, generally using the former. error: The type of error can be referred to in LAError.h. */ - (BOOL)canEvaluatePolicy:(LAPolicy)policy error:(NSError * __autoreleasing *)error __attribute__((swift_error(none)));
/* This is the way to start verifying fingerprints. policy: This is the above enumeration of the two verification methods, generally using the former. localizedReason: The message on the fingerprint verification box is "verify the fingerprint of an existing mobile phone by the Home key" (can't be empty or crash) reply: A block returns the result of fingerprint verification. Success: success is YES, failure: success is NO, and error of error type is returned. Also refer to the type in LAError.h. */ - (void)evaluatePolicy:(LAPolicy)policy localizedReason:(NSString *)localizedReason reply:(void(^)(BOOL success, NSError * __nullable error))reply;
//Used to abolish the instance object context - (void)invalidate NS_AVAILABLE(10_11, 9_0);
The following two enumerations and three methods are generally not used, so we will not introduce them here.
// Two enumerations LACredentialType LAAccessControlOperation // Three methods - (BOOL)setCredential:(nullable NSData *)credential type:(LACredentialType)type NS_AVAILABLE(10_11, 9_0) __WATCHOS_AVAILABLE(3.0) __TVOS_UNAVAILABLE; - (BOOL)isCredentialSet:(LACredentialType)type NS_AVAILABLE(10_11, 9_0) __WATCHOS_AVAILABLE(3.0) __TVOS_UNAVAILABLE; - (void)evaluateAccessControl:(SecAccessControlRef)accessControl operation:(LAAccessControlOperation)operation localizedReason:(NSString *)localizedReason reply:(void(^)(BOOL success, NSError * __nullable error))reply NS_AVAILABLE(10_11, 9_0) __WATCHOS_AVAILABLE(3.0) __TVOS_UNAVAILABLE;
There are five other attributes
// You can set the title of the fingerprint cartridge "Enter password" button. If not set or set to nil, the default "Enter password" will be displayed. If set to @", the cartridge will no longer display the button. @property (nonatomic, nullable, copy) NSString *localizedFallbackTitle;
// You can set the title of the "Cancel" button of the fingerprint cartridge (iOS 10.0 or above is available). If it is not set to nil or set to @ ", the default"Cancel"will be displayed. @property (nonatomic, nullable, copy) NSString *localizedCancelTitle NS_AVAILABLE(10_12, 10_0);
// Maximum number of fingerprint attempts (iOS 8.3 - iOS 9.0 available) @property (nonatomic, nullable) NSNumber *maxBiometryFailures NS_DEPRECATED_IOS(8_3, 9_0) __WATCHOS_UNAVAILABLE __TVOS_UNAVAILABLE;
// This can detect changes in your fingerprint database, adding or deleting fingerprints will respond accordingly (iOS 9.0 or more is available) @property (nonatomic, nullable, readonly) NSData *evaluatedPolicyDomainState NS_AVAILABLE(10_11, 9_0) __WATCHOS_UNAVAILABLE __TVOS_UNAVAILABLE;
// The time interval between two fingerprints is opened to determine whether fingerprint unlocking is needed for the second time. @property (nonatomic) NSTimeInterval touchIDAuthenticationAllowableReuseDuration NS_AVAILABLE(NA, 9_0) __WATCHOS_UNAVAILABLE __TVOS_UNAVAILABLE;
II. Usage
1. First, the dependency framework Local Authentication. framework is introduced.
#import <LocalAuthentication/LocalAuthentication.h>
2. Integrated fingerprint unlocking method
- (void)evaluateAuthenticate { //iOS 8 or above supports fingerprint identification interface if ([[UIDevice currentDevice].systemVersion floatValue] < 8) { NSLog(@"I won't support it TouchID (Version must be higher than iOS 8.0 To be able to use)"); return; } //Create LAContext LAContext *context = [[LAContext alloc] init]; context.localizedFallbackTitle = @"Enter your password"; NSError *Error = nil; //Judging Equipment Support Status if ([context canEvaluatePolicy:LAPolicyDeviceOwnerAuthenticationWithBiometrics error:&Error]) { //Support fingerprint verification [context evaluatePolicy:LAPolicyDeviceOwnerAuthenticationWithBiometrics localizedReason:@"Please verify the fingerprint of the existing mobile phone" reply:^(BOOL success, NSError *error) { if (success) { //Verification is successful and the main thread processes the UI [[NSOperationQueue mainQueue] addOperationWithBlock:^{ NSLog(@"Fingerprint Verification Successful"); }]; } else { NSLog(@"Validation failure == %@", error.localizedDescription); switch (error.code) { case LAErrorSystemCancel:{ NSLog(@"The system revokes authorization, for example APP cut-in"); } break; case LAErrorUserCancel:{ NSLog(@"The user canceled the validation and clicked the Cancel button."); } break; case LAErrorUserFallback:{ NSLog(@"User cancels validation and clicks the Enter Password button"); [[NSOperationQueue mainQueue] addOperationWithBlock:^{ //User chooses to enter password and switch main thread processing }]; } break; case LAErrorAuthenticationFailed:{ NSLog(@"Failure of three consecutive fingerprint verification may result in blurred fingerprints or incorrect fingerprints"); } break; case LAErrorTouchIDLockout:{ NSLog(@"equipment TouchID Locked, because there are too many failures"); } break; default:{ NSLog(@"equipment TouchID Not available..."); [[NSOperationQueue mainQueue] addOperationWithBlock:^{ //In other cases, switch the main thread processing }]; } break; } } }]; } else { //TouchID is not supported on this device NSLog(@"I won't support it TouchID == %@", Error.localizedDescription); switch (Error.code) { case LAErrorTouchIDNotEnrolled:{ NSLog(@"Fingerprint verification could not be started because no fingerprints were entered"); } break; case LAErrorPasscodeNotSet:{ NSLog(@"Fingerprint verification cannot be started because the device has no password set"); } break; case LAErrorTouchIDLockout:{ NSLog(@"equipment TouchID Locked, because there are too many failures"); } break; default:{ NSLog(@"equipment TouchID Not available..."); } break; } } }
3. demo
Here's one I packaged. demo Welcome to consult!
If you have any questions, please leave a message!