Binary threshold processing
The source code of this section is in the file examples / filtering / binarythresholdimagefilter cxx
Yes.
This example illustrates the use of binary threshold image filter. This filter passes through as shown in Figure 6-1
The pixel values are changed under the rules shown to convert an image into a binary image. Users need to specify two thresholds: upper threshold and lower threshold, that is, two brightness values: internal and external. For each pixel in the input image, the upper and lower thresholds are used to compare with the pixel value. If the pixel value is within the range defined by [lower threshold, upper threshold], the output pixel is specified as InsideValue
; Otherwise, the output pixel is specified as OutsideValue
. Threshold processing is usually used as the last operation of a segmentation path.
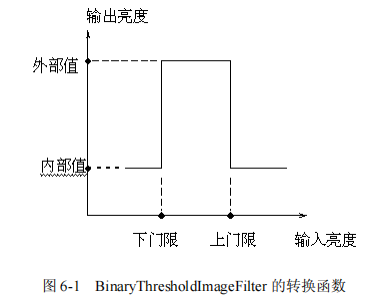
//The first step to use itk::BinaryThresholdImageFilter is to include its header file: #include "itkBinaryThresholdImageFilter.h" //The next step is to determine the pixel type of the input and output images: typedef unsigned char InputPixelType; typedef unsigned char OutputPixelType; //Now use pixel type and dimension to define the types of input and output images respectively: typedef itk::Image< InputPixelType, 2 > InputImageType; typedef itk::Image< OutputPixelType, 2 > OutputImageType; //Instantiate the filter type using the input and output image types defined above: typedef itk::BinaryThresholdImageFilter< InputImageType, OutputImageType > FilterType; //Also instantiate an itk::ImageFileReader class to read images from a file (refer to Chapter 7 for more information on reading and writing data): typedef itk::ImageFileReader< InputImageType > ReaderType; //Instantiate an itk::ImageFileWriter to write the output image to a file: typedef itk::ImageFileWriter< InputImageType > WriterType; //Both filters and reader s are created by calling their New() method and pointing the results to itk::SmartPointers: ReaderType::Pointer reader = ReaderType::New( ); FilterType::Pointer filter = FilterType::New( ); //The image obtained by the reader is passed to BinaryThresholdImageFilter as input: filter->SetInput( reader->GetOutput( ) ); //The SetOutsideValue() method defines the brightness value pointing to pixels whose brightness value is outside the upper and lower threshold range. //The SetInsideValue() method defines the brightness value pointing to pixels whose brightness value is within the upper and lower threshold range: inside the threshold range. filter->SetOutsideValue( outsideValue ); filter->SetInsideValue( insideValue ); //The SetLowerThreshold() and setuperthreshold() methods define the brightness range of the input image that will be converted to InsideValue. Note that the types of upper and lower threshold values are the pixel types of the input image, while the types of InsideValue and OutsideValue are the pixel types of the output image. filter->SetLowerThreshold( lowerThreshold ); filter->SetUpperThreshold( upperThreshold ); //Trigger the operation of the filter by calling Update(). If the output of the filter is passed as an input to the following wave filter, Update( )Any later filter in the transfer path will be called to directly trigger the update of this filter. filter->Update( );
This figure shows the limitations of this filter in realizing segmentation. These limitations are in noisy images and similar to MRI
The situation is particularly obvious in the image of lack of spatial uniformity caused by field bias.
The following classes provide similar functionality:
• itk::ThresholdImageFilter
Threshold processing summary
The source code of this section is in the file examples / filtering / thresholdimagefilter cxx
Yes.
This example illustrates itk::ThresholdImageFilter
Usage of. This filter can convert the brightness level of an image in three different ways.
The first one: the user defines a single threshold. Any pixel whose pixel value is below this threshold will be replaced by a user-defined value, which is called OutsideValue here
. Pixels with pixel values above this threshold will remain unchanged. As shown in the figure
6-3 illustrates this type of threshold processing.
The second: the user defines a specific threshold, and all pixels with pixel values above this threshold will be replaced by OutsideValue. Pixels with pixel values below this threshold will remain unchanged. As shown in Figure 6-4
As shown in.
The third one: two thresholds defined by the user. All pixels whose pixel values are within the range defined by these two thresholds will remain unchanged, and pixels whose pixel values are outside this range will be specified as OutsideValue
, as shown in the figure
6-5
As shown in.
Next, select one of the three operation modes of the filter:
(1)ThresholdBelow( )
(2)ThresholdAbove( )
(3)ThresholdOutside( )
//The first step in using this filter is to include its header file: #include "itkThresholdImageFilter.h" //Then we must determine the pixel type of the image to use. The filter is templated based on a single image type, because the algorithm only determines the pixel values outside the specified range and remains unchanged to transfer the remaining values. typedef unsigned char PixelType; //Use pixel types and dimensions to define images: typedef itk::Image< PixelType, 2 > ImageType; //Instantiate the filter using the previously defined image type: typedef itk::ThresholdImageFilter< ImageType > FilterType; //Also instantiate an itk::ImageFileReader class to read images from a file: typedef itk::ImageFileReader< ImageType > ReaderType; //Instantiate an itk::ImageFileWriter to write the output image to a file: typedef itk::ImageFileWriter< ImageType > WriterType; //Both filters and reader s are created by calling their New() method and pointing the results to itk::SmartPointers: ReaderType::Pointer reader = ReaderType::New( ); FilterType::Pointer filter = FilterType::New( ); //The image obtained by the reader is passed as input to itk::ThresholdImageFilter: filter->SetInput( reader->GetOutput( ) ); SetOutsideValue( )The method defines the brightness values pointing to pixels whose brightness values are outside the upper and lower threshold ranges: filter->SetOutsideValue( 0 ); //The second mode of operation can be achieved by calling thresholdlow(). //The thresholdlow() method defines the brightness value of the pixel to be changed to OutsideValue in the input image: filter->ThresholdBelow( 180 ); //Trigger the operation of the filter by calling Update(). filter->Update( ); //The second operation mode can be realized by calling ThresholdAbove(). filter->ThresholdAbove( 180 ); filter->Update( ); //The third mode of operation can be achieved by calling ThresholdOutside(). filter->ThresholdOutside( 170,190 ); filter->Update( ); 108
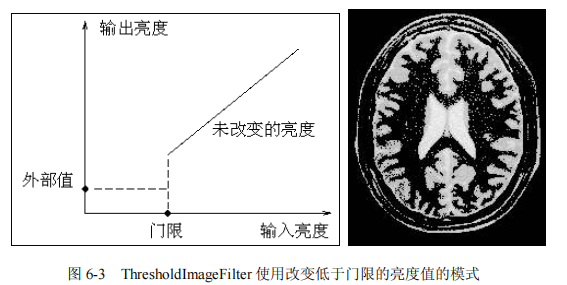
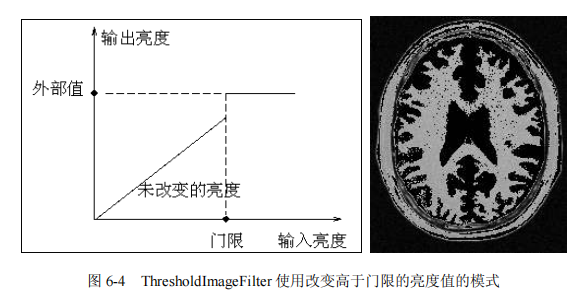
The example in this section also illustrates the limitations of this filter in realizing segmentation. These limitations are particularly obvious in noisy images and images similar to MRI, which lack spatial uniformity due to field bias.
The following classes provide similar functionality:
• itk::BinaryThresholdImageFilter