catalogue
1, Map interface
1. Map and Collection
2. Common methods in map interface
3. Map set traversal - keySet method
4. Entry interface
5. Map set traversal - Entry
6. HashMap stores user-defined type key values
2, Object oriented idea of set inheritance system
3, Mutual conversion of array and List
1. Convert Collaction to array
1, Map interface
1. Map and Collection
-
The Collection elements in the Collection are "isolated", and the elements stored in the Collection are stored one by one
-
A collection in a Map. Elements exist in pairs. Each element consists of two parts: key , and value , through which the corresponding value can be found.
-
The Collection in the Collection is called a single column Collection, and the Collection in the Map is called a double column Collection
-
The set {in the Map cannot contain duplicate keys, and the values can be duplicate; Each key can only correspond to one value
-
Common collections in Map are:
-
HashMap collection
The hash table structure is used to store data, and the access order of elements cannot be guaranteed to be consistent. To ensure the uniqueness and non repetition of keys, you need to rewrite the hashCode() method and equals() method of keys. -
LinkedHashMap collection.
The hash table structure + linked list structure is adopted for storing data. Through the linked list structure, the access order of elements can be ensured to be consistent; The hash table structure can ensure the uniqueness and non repetition of the key. The hashCode() method and equals() method of the key need to be rewritten. -
The set in the Map interface has two generic variables < K, V >, which should be given data types when used. The data types of two generic variables < K, V > can be the same or different.
2. Common methods in map interface
-
put(K key, V value): corresponds the specified key to the value and adds it to the collection
-
Method returns the value corresponding to the key
If there is no key to the set, the value of 1. put (null) will be returned if there is no key specified in the set;
2. When using the put method, if the specified key exists in the set, the return value is the value corresponding to the key in the set (the value is the value before replacement), and replace the value corresponding to the specified key with the specified new value.
3.get(Object key): get the value corresponding to the specified key
-
If there is no such key in the collection, null is returned
-
remove(Object key): deletes an element according to the specified key and returns {the value of the deleted element
-
If there is no such key in the collection, null is returned
3. Map set traversal - keySet method
-
keySet method: store all keys in the keySet Set
-
Traversal steps:
-
Call the # keySet method of the map Set and store all keys in the # Set #
-
Traverse the Set set and get all the elements (keys in the Map) in the Set set
-
Call the map set get method to get the value through the key
public class MapDemo { public static void main(String[] args) { Map<String,Integer> map = new HashMap<String,Integer>(); map.put("a", 11); map.put("b", 12); map.put("c", 13); map.put("d", 14); Set<String> set = map.keySet(); Iterator<String> it = set.iterator(); while(it.hasNext()){ String key = it.next(); Integer value = map.get(key); System.out.println(key+"...."+value); } //Enhance for traversal with for(String key : map.keySet()){ Integer value = map.get(key); System.out.println(key+"...."+value); }
4. Entry interface
- When designing the Map class, it provides a nested interface: entry. Entry encapsulates the correspondence of key value pairs into objects. That is, the key value pair object, so that when we traverse the Map collection, we can obtain the corresponding key and corresponding value from each key value pair object
- Entry is a} static internal nested interface provided in the Map interface
- Map.Entry(K, V)
- entrySet() method: used to return all key value pair (Entry) objects in the Map Set in the form of "Set".
5. Map set traversal - Entry
-
Key value pair method: that is to obtain the keys and values in the key value pair (Entry) object through each} key value pair (Entry) object in the collection.
-
Traversal steps:
-
Call the map collection method {entrySet(), and store the mapping relationship objects in the collection into the Set collection
-
Traverse the Set set containing key value pair (Entry) objects to get each key value pair (Entry) object
-
Through the key value pair (Entry) object, use getKey and getValue to obtain the key and value in the Entry object.
Map collections cannot be traversed directly using iterators or {for}. But it can be used after being converted to Set.
public class MapSet { public static void main(String[] args) { Map<Integer,String> map = new HashMap<>(); map.put(1,"a"); map.put(2,"ab"); map.put(3,"abc"); //Call the map Set method entrySet() to store the mapping relationship objects in the Set into the Set Set<Map.Entry<Integer,String>> set = map.entrySet(); //Traverse Set set Iterator<Map.Entry<Integer,String>> it = set.iterator(); while(it.hasNext()){ //Get the elements in the Set collection, that is, the mapping relationship object //it.next is the map Entry object Map.Entry<Integer,String> entry = it.next(); //Through the key value pair (Entry) object, get the key and value in the Entry object with getKey and getValue Integer key = entry.getKey(); String value = entry.getValue(); System.out.println(key+" : "+value); } } //Enhanced for loop for(Map.Entry<Integer,String> entry : map.entrySet()){ System.out.println(entry.getKey()+" : "+entry.getValue()); } }
6. HashMap stores user-defined type key values
- When storing custom objects in HashMap, if the custom objects exist as , key , the , hashCode , and , equals methods of the object must be copied to ensure the uniqueness of the , object
- If you want to ensure that the keys stored in the map are in the same order as the keys taken out, you can use the LinkedHashMap collection to store them.
2, Object oriented idea of set inheritance system
- Interface: used to specify the functions of the in all sets, which is equivalent to defining the set function standard;
- Abstract class: extract the methods with the same function implementation mode from multiple sets to the abstract class implementation. The specific set is no longer written, but can be inherited and used;
- Concrete classes: inherit abstract classes, implement interfaces, and rewrite all abstract methods to achieve a set with specified functions. Each specific collection class implements the function methods in the interface in different ways according to its own data storage structure.
3, Mutual conversion of array and List
1. Convert Collaction to array
- Use arrays The List obtained by aslist is not allowed to add elements or delete elements, because it is a thread unsafe collection and must be converted to a thread safe collection
- Collections. Synchronized list (list): converts a list into a thread safe collection
- Collections.synchronizedList(set)
- Collections.synchronizedList(map)
public class Supplement01 { public static void main(String[] args) { //int[] a = {1, 2, 3, 4}; //Convert array to collection List<Integer> list = Arrays.asList(1, 2, 3, 4); //Add element, delete element //Use arrays The List obtained by aslist is not allowed to add elements or delete elements //list.add(5); //list.remove(0); //Thread unsafe collection ArrayList<String> list1 = new ArrayList<>(); //Convert thread unsafe collections to thread safe collections //strings is a thread safe collection List<String> strings = Collections.synchronizedList(list1); } } ### last In a word, the interviewer asks questions and questions Redis There are so many knowledge points. The review is not in place and the mastery of knowledge points is not skilled enough, so the interview will be blocked. These Redis Interview knowledge analysis and some of my study notes are shared for your reference **Note to those who need these learning notes:[You can get it for free by poking here](https://docs.qq.com/doc/DSmxTbFJ1cmN1R2dB)** More study notes and interview materials are also shared as follows (all available for free): 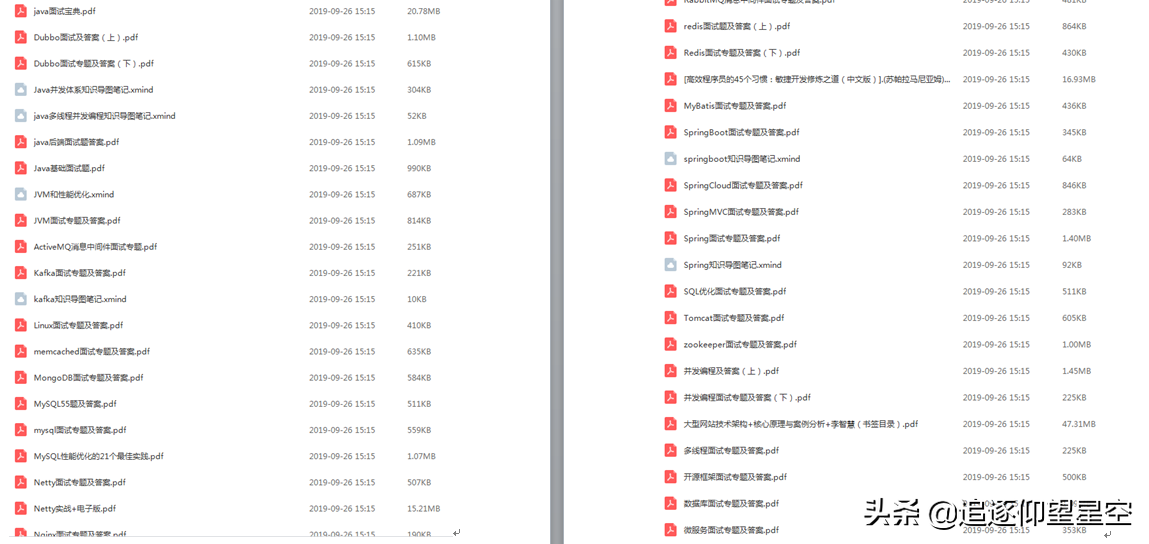 is Interview knowledge analysis and some of my study notes are shared for your reference **Note to those who need these learning notes:[You can get it for free by poking here](https://docs.qq.com/doc/DSmxTbFJ1cmN1R2dB)** More study notes and interview materials are also shared as follows (all available for free): [External chain picture transfer...(img-aOkFZ3ck-1623562623655)]