Output results:
Second timestamp: 1591778815
Haos timestamp: 1591778815967
Nanosecond: 967 million
If the string format is not correct, for example, it is modified to 2020-06-10T08:46:55.967, Java. Net will be thrown time. format. Datetimeparseexception exception, as shown in the following figure:
2. LocalDate
2.1 get current date
Using LocalDate to get the current date is very simple, as shown below:
LocalDate today = LocalDate.now(); System.out.println("today: " + today);
Output results:
today: 2020-06-10
Without any formatting, the output result is very friendly. If you use Date to output such a format, you have to specify yyyy MM DD in conjunction with SimpleDateFormat for formatting. There will be a bug accidentally. For example, there was a popular bug at the end of last year. I still cut the figure at that time:
These two friends followed me on December 31, 2019, but when I checked it on January 2, 2020, it was displayed as December 31, 2020. Why? When formatting the date, the format is wrong. It should be yyyy / mm / DD, but it is written as yyyy / mm / DD. just that week crosses the new year, it will be displayed as the next year, that is, 2020. At that time, several bloggers wrote articles to analyze the reasons, so I won't explain too much here.
Key points: all of this is said, to everyone, I am newly registered under the official account of Amway.
2.2 date of acquisition
LocalDate today = LocalDate.now(); int year = today.getYear(); int month = today.getMonthValue(); int day = today.getDayOfMonth(); System.out.println("year: " + year); System.out.println("month: " + month); System.out.println("day: " + day);
Output results:
year: 2020
month: 6
day: 10
Getting the month finally returns 1 to 12, unlike Java util. The calendar gets the month and returns 0 to 11. After obtaining, you have to add 1.
2.3 designated date
LocalDate specifiedDate = LocalDate.of(2020, 6, 1); System.out.println("specifiedDate: " + specifiedDate);
Output results:
specifiedDate: 2020-06-01
If the month is determined, it is recommended to use another overloaded method to specify the month by enumeration:
LocalDate specifiedDate = LocalDate.of(2020, Month.JUNE, 1);
2.4 are the comparison dates equal
LocalDate localDate1 = LocalDate.now(); LocalDate localDate2 = LocalDate.of(2020, 6, 10); if (localDate1.equals(localDate2)) { System.out.println("localDate1 equals localDate2"); }
Output results:
localDate1 equals localDate2
2.5 the acquisition date is the day of the week / month / year
LocalDate today = LocalDate.now(); System.out.println("Today:" + today); System.out.println("Today is:" + today.getDayOfWeek()); System.out.println("Today is the third day of the week" + today.getDayOfWeek().getValue() + "day"); System.out.println("Today is the third day of this month" + today.getDayOfMonth() + "day"); System.out.println("Today is the third day of the year" + today.getDayOfYear() + "day");
Output results:
Today:2020-06-11
Today is:THURSDAY
Today is the fourth day of the week
Today is the 11th day of this month
Today is the 163rd day of the year
2.6 judge whether it is a leap year
LocalDate today = LocalDate.now(); System.out.println(today.getYear() + " is leap year:" + today.isLeapYear());
Output results:
2020 is leap year:true
3. LocalTime
3.1 obtaining hours, minutes and seconds
If you use Java util. Date, the code is as follows:
Date date = new Date(); int hour = date.getHours(); int minute = date.getMinutes(); int second = date.getSeconds(); System.out.println("hour: " + hour); System.out.println("minute: " + minute); System.out.println("second: " + second);
Output results:
Note: these methods have expired, so it is strongly not recommended to use them in the project:
If you use Java util. Calendar, the code is as follows:
Calendar calendar = Calendar.getInstance(); // 12 hour system int hourOf12 = calendar.get(Calendar.HOUR); // 24-hour system int hourOf24 = calendar.get(Calendar.HOUR_OF_DAY); int minute = calendar.get(Calendar.MINUTE); int second = calendar.get(Calendar.SECOND); int milliSecond = calendar.get(Calendar.MILLISECOND); System.out.println("hourOf12: " + hourOf12); System.out.println("hourOf24: " + hourOf24); System.out.println("minute: " + minute); System.out.println("second: " + second); System.out.println("milliSecond: " + milliSecond);
Output results:
**Note: * * there are two options when obtaining hours: one returns the hours in 12 hour system and the other returns the hours in 24-hour system. Because it is 8 p.m., calendar Get (calendar. Hour) returns 8, while calendar Get (calendar. Hour_of_day) returns 20.
If you use Java time. Localtime, the code is as follows:
LocalTime localTime = LocalTime.now(); System.out.println("localTime:" + localTime); int hour = localTime.getHour(); int minute = localTime.getMinute(); int second = localTime.getSecond(); System.out.println("hour: " + hour); System.out.println("minute: " + minute); System.out.println("second: " + second);
Output results:
As you can see, LocalTime has only time and no date.
4. LocalDateTime
4.1 get current time
LocalDateTime localDateTime = LocalDateTime.now(); System.out.println("localDateTime:" + localDateTime);
Output results:
localDateTime: 2020-06-11T11:03:21.376
4.2 obtain the hour, minute and second of month, day and year
LocalDateTime localDateTime = LocalDateTime.now(); System.out.println("localDateTime: " + localDateTime); System.out.println("year: " + localDateTime.getYear()); System.out.println("month: " + localDateTime.getMonthValue()); System.out.println("day: " + localDateTime.getDayOfMonth()); System.out.println("hour: " + localDateTime.getHour()); System.out.println("minute: " + localDateTime.getMinute()); System.out.println("second: " + localDateTime.getSecond());
Output results:
4.3 add days / hour
LocalDateTime localDateTime = LocalDateTime.now(); System.out.println("localDateTime: " + localDateTime); LocalDateTime tomorrow = localDateTime.plusDays(1); System.out.println("tomorrow: " + tomorrow); LocalDateTime nextHour = localDateTime.plusHours(1); System.out.println("nextHour: " + nextHour);
Output results:
localDateTime: 2020-06-11T11:13:44.979
tomorrow: 2020-06-12T11:13:44.979
nextHour: 2020-06-11T12:13:44.979
LocalDateTime also provides methods to add year, week, minute and second, which are not listed here:
4.4 days / hour reduction
LocalDateTime localDateTime = LocalDateTime.now(); System.out.println("localDateTime: " + localDateTime); LocalDateTime yesterday = localDateTime.minusDays(1); System.out.println("yesterday: " + yesterday); LocalDateTime lastHour = localDateTime.minusHours(1); System.out.println("lastHour: " + lastHour);
Output results:
localDateTime: 2020-06-11T11:20:38.896
yesterday: 2020-06-10T11:20:38.896
lastHour: 2020-06-11T10:20:38.896
Similarly, LocalDateTime also provides methods to reduce juvenile, week, minute and second, which are not listed here:
4.5 the acquisition time is the day of the week / year
LocalDateTime localDateTime = LocalDateTime.now(); System.out.println("localDateTime: " + localDateTime); System.out.println("DayOfWeek: " + localDateTime.getDayOfWeek().getValue()); System.out.println("DayOfYear: " + localDateTime.getDayOfYear());
Output results:
localDateTime: 2020-06-11T11:32:31.731
DayOfWeek: 4
DayOfYear: 163
5. DateTimeFormatter
Java. Net has been introduced in JDK8 time. format. DateTimeFormatter is used to handle date formatting. In Alibaba java development manual, it is also recommended to use DateTimeFormatter instead of SimpleDateFormat.
5.1 formatting LocalDate
LocalDate localDate = LocalDate.now(); System.out.println("ISO_DATE: " + localDate.format(DateTimeFormatter.ISO_DATE)); System.out.println("BASIC_ISO_DATE: " + localDate.format(DateTimeFormatter.BASIC_ISO_DATE)); System.out.println("ISO_WEEK_DATE: " + localDate.format(DateTimeFormatter.ISO_WEEK_DATE)); System.out.println("ISO_ORDINAL_DATE: " + localDate.format(DateTimeFormatter.ISO_ORDINAL_DATE));
Output results:
If the format provided cannot meet your needs, you can also customize the format as before:
LocalDate localDate = LocalDate.now(); System.out.println("yyyy/MM/dd: " + localDate.format(DateTimeFormatter.ofPattern("yyyy/MM/dd")));
Output results:
yyyy/MM/dd: 2020/06/11
5.2 formatting LocalTime
LocalTime localTime = LocalTime.now(); System.out.println(localTime); System.out.println("ISO_TIME: " + localTime.format(DateTimeFormatter.ISO_TIME)); System.out.println("HH:mm:ss: " + localTime.format(DateTimeFormatter.ofPattern("HH:mm:ss")));
Output results:
14:28:35.230
ISO_TIME: 14:28:35.23
HH:mm:ss: 14:28:35
5.3 formatting LocalDateTime
LocalDateTime localDateTime = LocalDateTime.now(); System.out.println(localDateTime); System.out.println("ISO_DATE_TIME: " + localDateTime.format(DateTimeFormatter.ISO_DATE_TIME)); System.out.println("ISO_DATE: " + localDateTime.format(DateTimeFormatter.ISO_DATE));
Output results:
2020-06-11T14:33:18.303
ISO_DATE_TIME: 2020-06-11T14:33:18.303
ISO_DATE: 2020-06-11
6. Type conversion
6.1 Instant to Date
In JDK8, the from() method is added to Date to convert Instant to Date. The code is as follows:
Instant instant = Instant.now(); System.out.println(instant); Date dateFromInstant = Date.from(instant); System.out.println(dateFromInstant);
Output results:
2020-06-11T06:39:34.979Z
Thu Jun 11 14:39:34 CST 2020
6.2 Date to Instant
In JDK8, toInstant method is added to Date to convert Date into Instant. The code is as follows:
Date date = new Date(); Instant dateToInstant = date.toInstant(); System.out.println(date); System.out.println(dateToInstant);
Output results:
Thu Jun 11 14:46:12 CST 2020
2020-06-11T06:46:12.112Z
6.3 Date to LocalDateTime
Date date = new Date(); Instant instant = date.toInstant(); LocalDateTime localDateTimeOfInstant = LocalDateTime.ofInstant(instant, ZoneId.systemDefault()); System.out.println(date); System.out.println(localDateTimeOfInstant);
Output results:
Thu Jun 11 14:51:07 CST 2020
2020-06-11T14:51:07.904
6.4 Date to LocalDate
Date date = new Date(); Instant instant = date.toInstant(); LocalDateTime localDateTimeOfInstant = LocalDateTime.ofInstant(instant, ZoneId.systemDefault()); LocalDate localDate = localDateTimeOfInstant.toLocalDate(); System.out.println(date); System.out.println(localDate);
Output results:
Thu Jun 11 14:59:38 CST 2020
2020-06-11
It can be seen that Date is first converted to Instant, then to LocalDateTime, and then obtain LocalDate through LocalDateTime.
6.5 Date to LocalTime
last
That's all I want to share with you this time
The information is all in -*** My study notes: big factory interview real questions + microservices + MySQL+Java+Redis + algorithm + Network + Linux+Spring family bucket + JVM + study note map***
Finally, share a big gift package (learning notes) of the ultimate hand tearing architecture: distributed + microservices + open source framework + performance optimization
OfInstant.toLocalDate();
System.out.println(date);
System.out.println(localDate);
Output results: > Thu Jun 11 14:59:38 CST 2020 > > 2020-06-11 As you can see,`Date`Yes, convert to`Instant`,Convert to`LocalDateTime`,Then pass`LocalDateTime`obtain`LocalDate`. ### 6.5 Date to LocalTime ## last That's all I want to share with you this time All the information is in——***[My study notes: real interview questions for big factories+Microservices+MySQL+Java+Redis+algorithm+network+Linux+Spring Family bucket+JVM+Study note map](https://gitee.com/vip204888/java-p7)*** Finally, share a big gift bag of the ultimate hand tearing architecture(Study notes): Distributed+Microservices+Open source framework+performance optimization 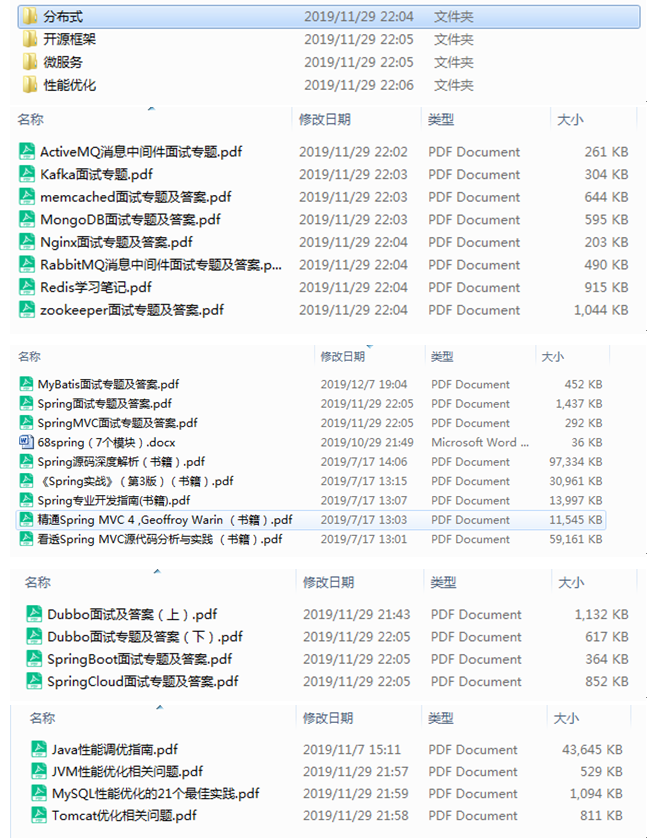