preface
- Maven is a project construction and management tool, which can be used to build and manage JAVA projects.
- Maven uses the Project Object Model (POM) to manage the project.
- Maven's main work is to parse some XML documents and manage the life cycle and plug-ins.
- Maven is designed to delegate the main responsibilities to a group of Maven plug-ins, which can affect the Maven life cycle and provide access to the target.
0x01 Maven environment configuration
1. JDK installation and system requirements
Maven is a Java based tool, so the first thing to do is to install JDK (Baidu). System requirements
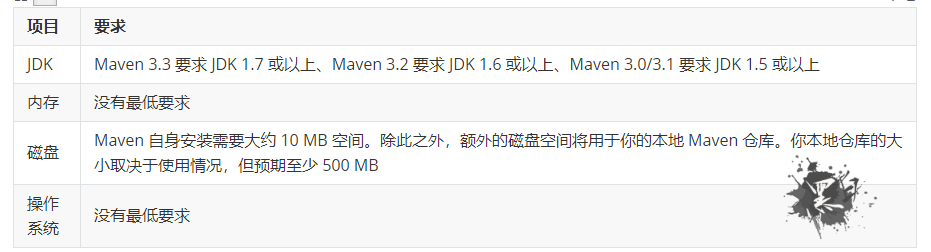
2. Maven installation and environment variable configuration
Mevan Download: http://maven.apache.org/download.cgi

Download Maven package and decompression path on the corresponding platform
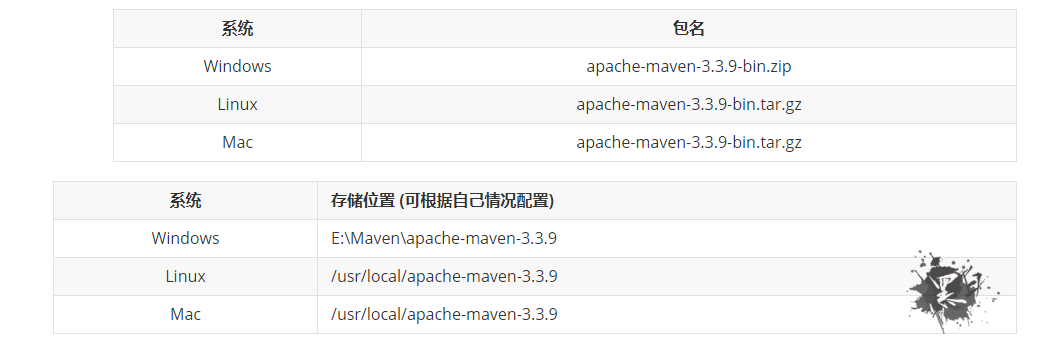
Set Maven environment variable
Windows
New system variable: MAVEN_HOME, variable value: e: maven apache-maven-3.3 nine
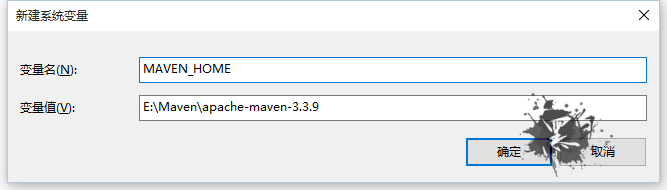
Edit system variable Path and add variable value:;% MAVEN_HOME%bin
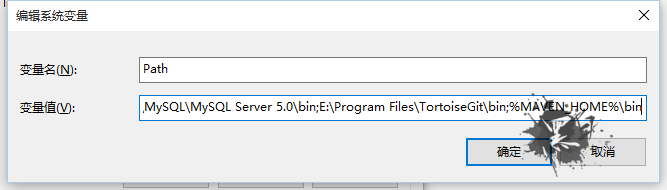
Linux
Download and extract:
# wget http://mirrors.hust.edu.cn/apache/maven/maven-3/3.3.9/binaries/apache-maven-3.3.9-bin.tar.gz # tar -xvf apache-maven-3.3.9-bin.tar.gz # sudo mv -f apache-maven-3.3.9 /usr/local/
Edit the / etc/profile} sudo vim /etc/profile and add the following code at the end of the file:
export MAVEN_HOME=/usr/local/apache-maven-3.3.9 export PATH=${PATH}:${MAVEN_HOME}/bin
Save the file and run the following command to make the environment variable effective:
Enter the following command on the console. If you can see the relevant version information of maven, it indicates that Maven has been successfully installed:
# source /etc/profile # mvn -v
Mac
Download and extract:
$ curl -O http://mirrors.hust.edu.cn/apache/maven/maven-3/3.3.9/binaries/apache-maven-3.3.9-bin.tar.gz $ tar -xvf apache-maven-3.3.9-bin.tar.gz $ sudo mv -f apache-maven-3.3.9 /usr/local/
Edit the / etc/profile} sudo vim /etc/profile and add the following code at the end of the file:
export MAVEN_HOME=/usr/local/apache-maven-3.3.9 export PATH=${PATH}:${MAVEN_HOME}/bin
Save the file and run the following command to make the environment variable effective:
$ source /etc/profile
Enter the following command on the console. If you can see the relevant version information of maven, it indicates that Maven has been successfully installed:
$ mvn -v Apache Maven 3.3.9 (bb52d8502b132ec0a5a3f4c09453c07478323dc5; 2015-11-11T00:41:47+08:00) Maven home: /usr/local/apache-maven-3.3.9 Java version: 1.8.0_31, vendor: Oracle Corporation Java home: /Library/Java/JavaVirtualMachines/jdk1.8.0_31.jdk/Contents/Home/jre Default locale: zh_CN, platform encoding: ISO8859-1 OS name: "mac os x", version: "10.13.4", arch: "x86_64", family: "mac"
0x02 Maven introduces external dependencies
Project structure
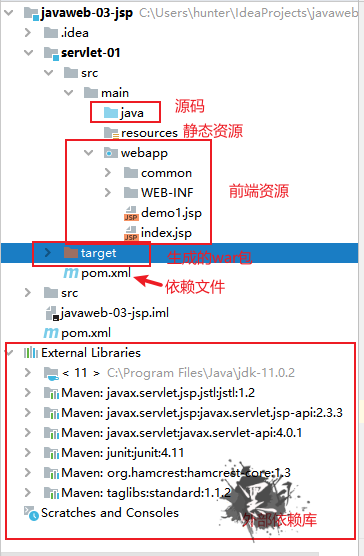
If our project needs to introduce external dependencies, you can use POM Add dependency to XML file
eg:sevlet Dependence of <dependency> <groupId>javax.servlet</groupId> <artifactId>javax.servlet-api</artifactId> <version>4.0.1</version> <scope>provided</scope> </dependency>
Complete POM XML file
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>org.example</groupId> <artifactId>javaweb-03-jsp</artifactId> <packaging>pom</packaging> <version>1.0-SNAPSHOT</version> <modules> <module>servlet-01</module> </modules> <dependencies> <!-- https://mvnrepository.com/artifact/taglibs/standard --> <dependency> <groupId>taglibs</groupId> <artifactId>standard</artifactId> <version>1.1.2</version> </dependency> <!-- https://mvnrepository.com/artifact/javax.servlet/javax.servlet-api --> <dependency> <groupId>javax.servlet</groupId> <artifactId>javax.servlet-api</artifactId> <version>4.0.1</version> <scope>provided</scope> </dependency> <!-- https://mvnrepository.com/artifact/javax.servlet.jsp/javax.servlet.jsp-api --> <dependency> <groupId>javax.servlet.jsp</groupId> <artifactId>javax.servlet.jsp-api</artifactId> <version>2.3.3</version> <scope>provided</scope> </dependency> <!-- https://mvnrepository.com/artifact/javax.servlet.jsp.jstl/jstl --> <dependency> <groupId>javax.servlet.jsp.jstl</groupId> <artifactId>jstl</artifactId> <version>1.2</version> </dependency> </dependencies> </project>
The dependent packages used in the project will be imported and used in the lib file under the WEB-INF folder when generating war
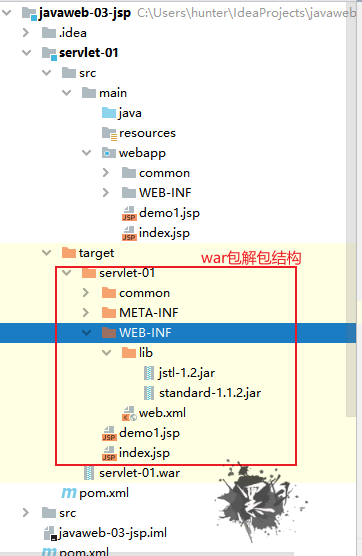
Dependent libraries: https://mvnrepository.com/