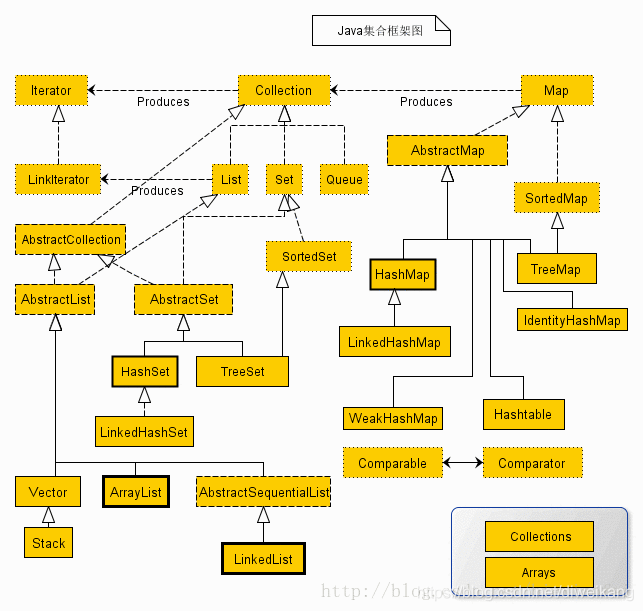
In the above class diagram, the solid line border is the implementation class, such as ArrayList, LinkedList, HashMap, etc., the broken line border is the abstract class, such as AbstractCollection, AbstractList, AbstractMap, etc., and the dotted line border is the interface, such as Collection, Iterator, List, etc
Features of the collection:
Collection is used to store different types of objects (except basic data types), and the storage length is variable.
What is actually stored in the Java collection is only the reference of the object. Each collection element is a reference variable. The actual content is placed in the heap memory or method area, but the basic data type allocates space on the stack memory, and the data on the stack will be retrieved at any time.
Application of common methods of ArrayList
import java.util.ArrayList; import java.util.Arrays; /* ArrayList Application of common methods */ public class Demo1 { public static void main(String[] args){ //ArrayList: the array structure is used. It is slow to add and delete and fast to find ArrayList<Integer> data = new ArrayList<>(); data.add(100); //Adds the specified element to the end of this list data.add(200); data.add(300); data.add(2,121); //Adds the specified element to the specified unknown element System.out.println(data.get(0)); //Gets the element at the specified location // data.clear(); // Delete all elements System.out.println(data.get(2)); data.indexOf(0); //Returns the index of the specified element that appears for the first time in this list, or - 1 if the list does not contain the element. System.out.println(data.get(0)); data.remove(0); //Deletes the element at the specified location in this list System.out.println(data.get(0)); data.set(1,110); //set is used to specify the element to replace the element at the specified position, which is preceded by the position and followed by the element System.out.println(data.get(1)); int i = data.size(); //Returns the elements in this list System.out.println(i); System.out.println(Arrays.toString(data.toArray())); //Returns an array containing all the elements in this list in the appropriate order (from the first element to the last element). } }
Application of Vector common methods
import java.util.Arrays; import java.util.Vector; /* Vector Application of common methods */ public class Demo2 { public static void main(String[] args) { //Vector: the array structure is used. It is slow to add, delete and find Vector<Integer> data = new Vector<>(); data.add(111); data.add(112); //Inserts the specified element into the specified position in this Vector System.out.println(data.get(0)); //Returns the element at the specified position in this Vector. data.add(1,123); //Inserts the specified element into the specified position in this Vector data.add(2,110); System.out.println(data.get(1)); data.remove(1); //Deletes the specified element at the specified location in this Vector System.out.println(data.get(1)); System.out.println(data.hashCode()); //Returns the hash code value of this Vector. data.set(1,125); //Replaces the element at the specified position in this Vector with the specified element System.out.println(data.get(1)); System.out.println(data.size()); //Returns the number of components in this vector. System.out.println(Arrays.toString(data.toArray())); //Returns an array containing all the elements in this Vector in the correct order System.out.println(data.toString()); //Returns the String representation of this Vector, including the String representation of each element. } }
Application of LinkedList common methods
public class Demo3 { public static void main(String[] args) { //LinkedList: it uses a two-way linked list structure. It is fast to add, delete and slow to find LinkedList<Integer> data = new LinkedList<>(); //Inserts the specified element at the specified location in this list. data.add(0,100); //Appends the specified element to the end of this list data.add(110); //Inserts the specified element at the beginning of this list. data.addFirst(222); //Appends the specified element to the end of this list data.addLast(333); //Returns the element at the specified location in this list System.out.println(data.get(0)); //Returns the first element in this list System.out.println(data.getFirst()); //Returns the last element in this list System.out.println(data.getLast()); //Deletes the element at the specified location in this list data.remove(1); //Retrieve and delete the header (first element) of this list data.remove(); //Stack pressing data.push(100); data.push(200); //Bomb stack Integer i = data.pop(); System.out.println(i); //Replaces the element at the specified location with the specified element data.set(1,369); System.out.println(data.get(1)); //Returns the number of elements in this list. System.out.println(data.size()); //Returns an array containing all the elements in this list in the appropriate order (from the first element to the last element). System.out.println(Arrays.toString(data.toArray())); } }
Application of Iterator interface
import java.util.ArrayList; import java.util.Iterator; import java.util.List; import java.util.ListIterator; /* Iterator Application of interface */ public class Demo4 { public static void main(String[] args) { //Iterator //ListIterator ArrayList<Integer> data = new ArrayList<>(); data.add(1); data.add(2); data.add(3); data.add(4); data.add(5); /*Iterator<Integer> iterator = data.iterator(); while (iterator.hasNext()){ Integer i = iterator.next(); System.out.println(i); } //Returns the next element in the list and advances the cursor position iterator.next(); //Removes the last element returned by next() or previous() (optional) from the list. iterator.remove(); System.out.println(data.size());*/ ListIterator<Integer> iterator = data.listIterator(); iterator.add(100); iterator.next(); //Returns the next element in the list and advances the cursor position. iterator.next(); System.out.println(iterator.next()); } }
Collection (the largest interface of a collection) inheritance relationship
Collection is the most basic collection interface. A collection represents a group of objects, that is, the Elements of the collection. Some collections allow the same Elements while others don't, some can sort and others don't. The Java SDK does not provide classes directly inherited from collection. The classes provided by the Java SDK are "sub interfaces" inherited from collection, such as List and Set.
All classes that implement the Collection interface must provide two standard constructors: the parameterless constructor is used to create an empty Collection; A constructor with a Collection parameter is used to create a new Collection, which has the same elements as the incoming Collection. The latter constructor allows the user to copy a Collection.
(1) List: it is orderly and can store duplicate contents
(2) Set: it is out of order and cannot store duplicate contents, so the duplicate contents are distinguished by hashCode() and equals()
(3) Queue: queue interface
(4) SortedSet: you can sort the data in the set
Map interface
Map is a container that associates key objects with value objects, and a value object can be a map, and so on, so as to form a multi-level mapping. For key objects, like Set, key objects in a map container are not allowed to be repeated in order to maintain the consistency of search results; If two key objects are the same, there is a problem when you want to get the value object corresponding to the key object. Maybe you don't get the value object you want, which will cause confusion. Therefore, the uniqueness of the key is very important and conforms to the nature of the Set. Of course, during use, the value object corresponding to a key may change. At this time, the value object will correspond to the key according to the last modified value object. There is no uniqueness requirement for value objects. You can map any number of keys to a value object without any problem (but it may be inconvenient for you to use. You don't know which key corresponds to the value object).
Please note that Map does not inherit the Collection interface, and Map provides the mapping from key to value. A Map cannot contain the same key, and each key can only Map one value. The Map interface provides views of three sets. The contents of the Map can be regarded as a set of key sets, a set of value sets, or a set of key value mappings.
Note: since the object as a key in the Map will determine the position of the corresponding value by calculating its hash function, any object as a key must implement hashCode and equals methods.
Subclasses that implement the Map interface:
——Hashtable: hashtable inherits the dictionary < K, V > class, implements the Map interface, and implements a hash table with key value mapping. Any non null object can be used as a key or value. Is synchronized.
——HashMap: HashMap is similar to Hashtable, except that HashMap is asynchronous and allows nulls, that is, null value and null key.
However, when a HashMap is regarded as a Collection (the values() method can return a Collection), the iterative sub operation time overhead is proportional to the capacity of the HashMap. Therefore, if the performance of iterative operations is very important, do not set the initialization capacity of HashMap too high or the load factor too low.
——LinkedHashMap: it is a subclass of HashMap. If the order of output and input are the same, LinkedHashMap can be used. Both Key and Value can be null; Duplicate Key will overwrite and duplicate Value is allowed; Non thread safe; Orderly.
——TreeMap: the bottom layer is a binary tree data structure. The thread is out of sync. It can be used to sort the keys in the map collection.
Set interface
The Set interface places additional conventions that exceed the contracts of all constructors inherited from the Collection interface, and are located in the add, equals and hashCode methods of the contract. For convenience, declarations of other inheritance methods are also included here. (the specifications attached to these declarations are customized for the Set interface, but they do not contain any other provisions.)
Note: if you use a mutable object as a set element, you must be very careful. If you change the value of an object in a way that affects the equals comparison when the object is an element in the collection, the behavior of the collection is not specified. A special case of this prohibition is that it is not allowed to include a set as an element.
Method application:
Variables and types | method | describe |
---|---|---|
boolean | add(E e) | Optionally, add the specified element to this collection if it does not already exist. |
boolean | addAll(Collection<? extends E> c) | If all elements in the specified collection do not already exist (optional), add them to the collection. |
void | clear() | Optionally, delete all elements from the collection. |
boolean | contains(Object o) | Returns true if this set contains the specified element. |
boolean | containsAll(Collection<?> c) | Returns true if the collection contains all the elements of the specified collection. |
static Set | copyOf(Collection<? extends E> coll) | Returns the unmodifiable Set containing the element of the given Collection. |
boolean | equals(Object o) | Compares the specified object with this set for equality. |
int | hashCode() | Returns the hash code value of this set. |
boolean | isEmpty() | Returns true if the collection does not contain any elements. |
Iterator | iterator() | Returns the iterator of the element in this set. |
static Set | of() | Returns a non modifiable set containing zero elements. |
static Set | of(E e1, E e2, E e3, E e4, E e5, E e6) | Returns an immutable set of six elements. |
static Set | of(E e1, E e2, E e3, E e4, E e5, E e6, E e7) | Returns a non modifiable set of seven elements. |
boolean | remove(Object o) | If present, removes the specified element from the collection (optional). |
boolean | removeAll(Collection<?> c) | Optionally, delete all elements contained in the specified collection from this collection. |
boolean | retainAll(Collection<?> c) | Keep only the elements in this collection that are included in the specified collection (optional). |
int | size() | Returns the number of elements (cardinality) in this collection. |
Object[] | toArray() | Returns an array containing all elements in this set. |
.