public float refund(E item) { return 0; }
}
FruitShop fruitShop = new FruitShop<>(); // This shop sells apples
FruitShop stringShop = new FruitShop<>(); // This fruit shop sells mobile phones??? Of course not logical
Because of our store generics E If there is no restriction, it will lead to mistakes. Let's put restrictions on it:
public class FruitShop implements Shop{
@Override public E buy() { return null; } @Override public float refund(E item) { return 0; }
}
Now this fruit shop can only sell Fruit perhaps Fruit Subclasses of, such as Apple,Instead of selling mobile phones. #### []( https://gitee.com/vip204888/java-p7 )Generic method You declared generic methods yourself Generic class indicates the specific type of the generic when instantiating the class; Generic method refers to the specific type of generic when calling the method. We created a method for our apple store that supports exchanging other fruits for apples:
public class FruitShop implements Shop{
@Override public E buy() { return null; } @Override public float refund(E item) { return 0; } public <T extends Fruit> E exchange(T item){ return null; }
}
//Use
FruitShop appleShop = new FruitShop<>();
Apple apple = appleShop.exchange(new Banana());
//Type derivation, can be omitted
Apple apple = appleShop.exchange(new Banana());
### []( https://gitee.com/vip204888/java-p7 )Type erase The advantage of generics is that type checking and type conversion are performed at compile time, and generics are valid only at compile time. Generics are in JDK 1.5 If you do not do generic erasure, then JVM It is necessary to correspond so that the generic information can be read and verified correctly; In addition, in order to be compatible with old programs, you need to be a program that does not support generics API Add a set of generics in parallel API. At runtime, the generic parameters at the time of declaration will be erased, and the generics at the place of use will be replaced by the parent class of the generics, such as`<E extends Fruit>`,Use at this time`E`The place will be Fruit Replace. ### []( https://gitee.com/vip204888/java-p7 )Covariance and inversion of generics Definition: if A,B Represents the type,`f()`Represents a constructor of type,`Type1≤Type2`express Type1 yes Type2 Subtypes of,`Type1≥Type2`express Type1 yes Type2 Supertype of; * `f()`It's covariance( covariant)Yes, when`A≤B`happen now and then`f(A)≤f(B)`Establishment; * `f()`It's inverter( contravariant)When`A≤B`happen now and then`f(B)≤f(A)`Establishment; * `f()`Is constant( invariant)Yes, when`A≤B`When the above two formulas are not true, that is`f(A)`And`f(B)`There is no inheritance relationship between them. For any two different types Type1 and Type2,Whatever the relationship between them, a given generic class `G<T>`,`G<Type1>` and `G<Type2>` It doesn't matter, that is Java In, generics are invariant while arrays are covariant. You can verify the following:
ArrayList list = new ArrayList(); // Compiler error
//It can be successfully compiled and used normally.
Number[] arr = new Integer[5];
arr[0] = 1;
//However, there are some problems. This array accepts Number, and other subclasses will not report errors at compile time, but at run time. Generics eliminate this error at its root
stay Java In generics, covariance and inversion can be supported, but there will be great limitations: * `? extends T`(Upper boundary wildcard)Implement covariant relationships, representing`?`Is inherited from`T`Any subtype of. It also represents a constraint relationship, which can only provide data and cannot receive data. * `? super T`(Lower boundary wildcard)Implement inverse relationships, representing`?`yes`T`Any parent type of. It also represents a constraint relationship, which can only receive data and cannot provide data. Covariant can only provide data and cannot receive data, which means that after instantiating a generic class, you can only call the methods in the class whose return value is generic, but not the methods whose parameters are generic. Inversion is just the opposite. So with such restrictions,`?`How should it be applied in our actual development?
List<? extends Number> list = new ArrayList();
Such usage is not available to us at ordinary times. Generic wildcards can only be used for some scenarios. Take our fruit store for example. We now have a demand to calculate the total weight of fruits in the collection, so we write the following method:
public int totalWeight(List<? extends Fruit> list) {
int total = 0; for(Fruit fruit : list) { total += fruit.weight; } return total;
}
//When used, you can pass in a collection of any Fruit subclasses to this method
List appleList = new ArrayList<>();
totalWeight(appleList);
List bananaList = new ArrayList<>();
totalWeight(bananaList);
Another usage of inversion:
List appleList = new ArrayList();
Of course, this usage is extremely rare and will not be discussed. We add a method to the apple class. The function of this method is to load the collection for the apple:
public class Apple extends Fruit{
public void addToList(List<? super Apple> list) { list.add(this); }
}
So, when we come Apple When we gather, we will Apple Load this collection. At the same time, we can also pass in Fruit Collection of:
List appleList = new ArrayList<>();
List fruitList = new ArrayList<>();
List bananaList = new ArrayList<>();
Apple apple = new Apple();
apple.addToList(appleList); // Compile passed
apple.addToList(fruitList); // Compile passed
apple.addToList(bananaList); // report errors
[](https://gitee.com/vip204888/java-p7) exception ---------------------------------------------------------------------- ### []( https://gitee.com/vip204888/java-p7 )Abnormal system Java The system of exceptions in is a tree structure, and the superclasses of all exceptions are Throwale,It has two subclasses: Error and Exception,Represents error and exception respectively, where exception class Exception It is also divided into runtime exceptions(RuntimeException)And non runtime exceptions, which are very different, also known as do not check exceptions( Unchecked Exception)And check for abnormalities( Checked Exception). 1. Error And Exception * Error It is an error that the program cannot handle, such as OutOfMemoryError,ThreadDeath Wait. When these anomalies occur, Java Virtual machine( JVM)Thread termination is usually selected. * Exception It is an exception that the program itself can handle. This exception is divided into two categories: runtime exception and non runtime exception. These exceptions should be handled as much as possible in the program. 2. Runtime and non runtime exceptions * Runtime exceptions are RuntimeException Class and its subclasses are abnormal, such as NullPointerException,IndexOutOfBoundsException Etc., the program can select capture processing or no processing. * Non runtime exception is RuntimeException Exceptions other than are of type Exception Class and its subclasses are exceptions that must be handled from the perspective of program syntax. If they are not handled, the program cannot be compiled, such as IOException,SQLException And user-defined Exception Abnormal. ### []( https://gitee.com/vip204888/java-p7 )Exception handling * stay try in return After, it will still be executed finally. * finally Statement in return After statement execution return Returns the previously executed. * finally In block return Statement overrides try In block return return. * If finally Not in statement return If the statement overrides the return value, the original return value may be because finally It may change or remain the same(Specifically distinguish between values and references). * When an exception occurs, catch Medium return Execution and when no exception occurs try in return The implementation of is exactly the same. * stay Java If there is no exception in the, try/catch No performance loss. stay Java After the class is compiled, the normal process is separated from the exception handling part. The class will follow an exception table, and each try-catch Row records will be added to this table. When the execution throws an exception, first go to the exception table to find out whether it can be deleted catch,If yes, skip to the starting position of exception handling to start processing. If not, go to the original position return,also copy The exception is referenced to the parent caller, then look at the exception table of the parent call, and so on. Let's take an example:
public int test(){
int i = 1; try { i++; return i; }catch (Exception e){ e.printStackTrace(); }finally { System.out.println("finally"); i++; } return 0;
}
System.out.println(test());
// out ->
finally
2
We see, finally Yes, then i++It should also be executed. Why 2 is returned? We can see by looking at the bytecode
Feeling:
In fact, when I submit my resume, I don't dare to send it to Ali. Because Ali has had three interviews in front of him, he didn't get his resume, so he thought he hung up.
Special thanks to the interviewer on one side for fishing me, giving me the opportunity, and recognizing my efforts and attitude. Comparing my facial Sutra with those of other big men, I'm really lucky. Others 80% strength, I may 80% luck. So for me, I will continue to redouble my efforts to make up for my lack of technology and the gap with the leaders of Keban. I hope I can continue to maintain my enthusiasm for learning and continue to work hard.
I also wish all students can find their favorite offer.
The preparations I made before the interview (review materials for brushing questions and learning notes and learning routes of some big guys) have been sorted into electronic documents, Friends in need can click [like + follow] to get it for free
Yes, why 2 is returned? We can see by looking at the bytecode
# **Feeling:** In fact, when I submit my resume, I don't dare to send it to Ali. Because Ali has had three interviews in front of him, he didn't get his resume, so he thought he hung up. Special thanks to the interviewer on one side for fishing me, giving me the opportunity, and recognizing my efforts and attitude. Comparing my facial Sutra with those of other big men, I'm really lucky. Others 80% strength, I may 80% luck. So for me, I will continue to redouble my efforts to make up for my lack of technology and the gap with the leaders of Keban. I hope I can continue to maintain my enthusiasm for learning and continue to work hard. **I also wish you all can find your heart offer. ** The preparations I made before the interview (review materials for brushing questions and learning notes and learning routes of some big guys) have been sorted into electronic documents,**[Friends in need can [like]+Follow] Click here to get it for free](https://gitee.com/vip204888/java-p7)** 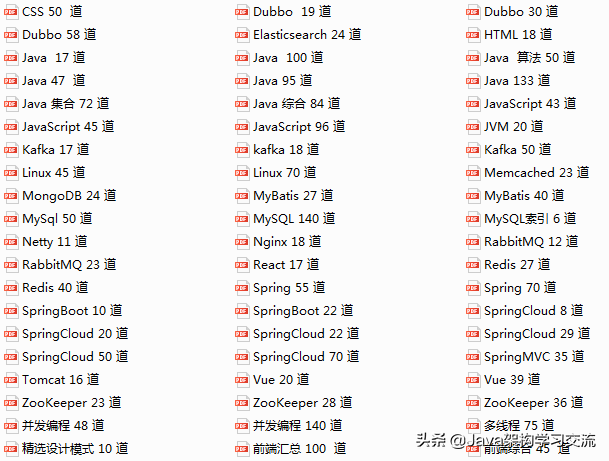