1 distributed
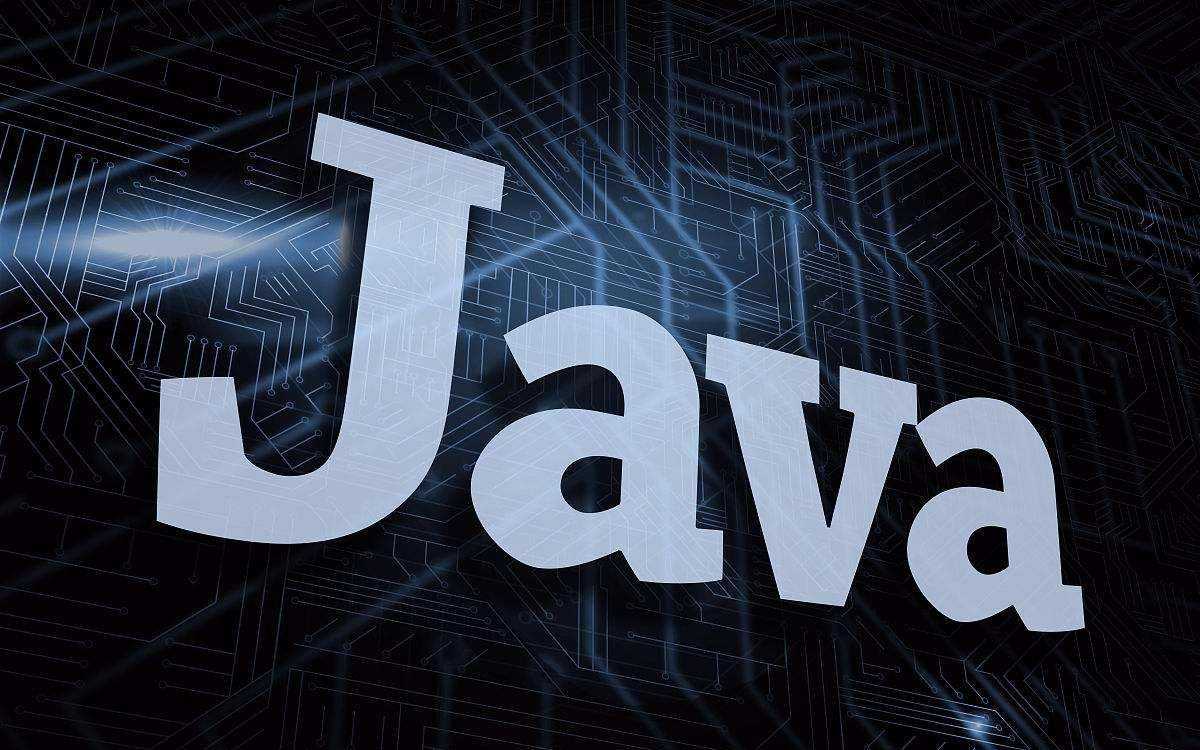
1.1 what is distributed
- A distributed system must be a system composed of multiple nodes. Among them, nodes refer to computer servers, and these nodes are generally not isolated, but interconnected.
- Our nodes are deployed on these connected nodes, and their operations will be coordinated. For users, the distributed system is just a server that provides the services they need. In fact, these services are a distributed system composed of many servers behind it. Therefore, the distributed system looks like a supercomputer.
1.2 difference between distributed and cluster
- Cluster means that the same system department is on different servers, for example, a login system department is on different servers
- Distributed is that different system departments call each other on different servers
There was only one chef in the small restaurant, who cut vegetables, washed vegetables, prepared materials and fried vegetables. Later, when there were many guests, one chef in the kitchen was busy, so another chef was hired. Both chefs could cook the same dishes. The relationship between the two chefs was cluster. In order to make the chef concentrate on cooking and make the dishes to the extreme, another garnish was hired to be responsible for cutting, preparing and preparing materials. The relationship between the chef and the garnish is distributed, and one garnish can't be busy. Another garnish was hired, and the relationship between the two garnish is set
2. Build distributed projects
Preparation tools: eclipse, VMware with CentOS7 system, zookeeper The most important thing is a three - year - old machine for the elderly
1. First, create a maven project of the parent class. The packaging method is pom
Create a parent Maven project in eclipse, which is packaged as pom Why create a maven project with a parent class? Because you want to use this Maven project to manage the versions of various jar packages, you can directly follow the parent class if you want the jar package for the subclass xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.itqf</groupId> <artifactId>sping-parent</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>pom</packaging> <!-- Define all jar Package version --> <properties> <junit.version>4.12</junit.version> <spring.version>4.2.4.RELEASE</spring.version> <mybatis.version>3.2.8</mybatis.version> <mybatis.spring.version>1.2.2</mybatis.spring.version> <mybatis.paginator.version>1.2.15</mybatis.paginator.version> <mysql.version>5.1.32</mysql.version> <slf4j.version>1.6.4</slf4j.version> <jackson.version>2.4.2</jackson.version> <druid.version>1.0.9</druid.version> <httpclient.version>4.3.5</httpclient.version> <jstl.version>1.2</jstl.version> <servlet-api.version>2.5</servlet-api.version> <jsp-api.version>2.0</jsp-api.version> <joda-time.version>2.5</joda-time.version> <commons-lang3.version>3.3.2</commons-lang3.version> <commons-io.version>1.3.2</commons-io.version> <commons-net.version>3.3</commons-net.version> <pagehelper.version>3.4.2-fix</pagehelper.version> <jsqlparser.version>0.9.1</jsqlparser.version> <commons-fileupload.version>1.3.1</commons-fileupload.version> <jedis.version>2.7.2</jedis.version> <solrj.version>4.10.3</solrj.version> <dubbo.version>2.5.3</dubbo.version> <zookeeper.version>3.4.7</zookeeper.version> <zkclient.version>0.1</zkclient.version> <activemq.version>5.11.2</activemq.version> <freemarker.version>2.3.23</freemarker.version> <quartz.version>2.2.2</quartz.version> </properties> <!-- Manage all the information used in the project jar Package, does not really rely on --> <dependencyManagement> <dependencies> <!-- Time operation component --> <dependency> <groupId>joda-time</groupId> Copy code
2. Create a maven aggregation project
2.1 create maven aggregation project and inherit the parent project
Aggregation project: the controller layer and view layer in the project can be separated into a project, and finally run together
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <groupId>com.itqf</groupId> <artifactId>sping-parent</artifactId> <version>0.0.1-SNAPSHOT</version> </parent> <groupId>com.itqf</groupId> <artifactId>sping-manager</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>pom</packaging> <dependencies> <dependency> <groupId>com.itqf</groupId> <artifactId>sping-common</artifactId> <version>0.0.1-SNAPSHOT</version> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.apache.tomcat.maven</groupId> <artifactId>tomcat7-maven-plugin</artifactId> <configuration> <port>8083</port> <path>/</path> </configuration> </plugin> </plugins> </build> <modules> <module>sping-manager-pojo</module> <module>sping-manager-interface</module> <module>sping-manager-service</module> <module>sping-manager-mapper</module> </modules> </project> Copy code
2.2 create maven Module in aggregation project and name it spin manager POJO (entity class layer)
- pojo is a common jar format and does not need to rely on the parent project
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <groupId>com.itqf</groupId> <artifactId>sping-manager</artifactId> <version>0.0.1-SNAPSHOT</version> </parent> <artifactId>sping-manager-pojo</artifactId> </project> Copy code
2.3 create maven Module in aggregation project and name it spin manager mapper (Dao layer)
- In POM Dependent pojo. XML file Because the method of mapper layer returns an entity class object, pojo is needed
- Import dependent jar packages
xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <groupId>com.itqf</groupId> <artifactId>sping-manager</artifactId> <version>0.0.1-SNAPSHOT</version> </parent> <artifactId>sping-manager-mapper</artifactId> <!-- rely on pojo --> <dependencies> <dependency> <groupId>com.itqf</groupId> <artifactId>sping-manager-pojo</artifactId> <version>0.0.1-SNAPSHOT</version> </dependency> <!-- Mybatis --> <dependency> <groupId>org.mybatis</groupId> <artifactId>mybatis</artifactId> <version>${mybatis.version}</version> </dependency> <dependency> <groupId>org.mybatis</groupId> <artifactId>mybatis-spring</artifactId> <version>${mybatis.spring.version}</version> </dependency> <dependency> <groupId>com.github.miemiedev</groupId> <artifactId>mybatis-paginator</artifactId> <version>${mybatis.paginator.version}</version> </dependency> <dependency> <groupId>com.github.pagehelper</groupId> <artifactId>pagehelper</artifactId> <version>${pagehelper.version}</version> </dependency> <!-- MySql --> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>${mysql.version}</version> </dependency> <!-- Connection pool --> <dependency> <groupId>com.alibaba</groupId> <artifactId>druid</artifactId> <version>${druid.version}</version> </dependency> </dependencies> </project> Copy code
2.4 create a spin manager interface in the aggregation project and put all service interfaces into independent projects
- If the method return value in the interface is an entity class, pojo is required Therefore, we rely on pojo xml in pom
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <groupId>com.itqf</groupId> <artifactId>sping-manager</artifactId> <version>0.0.1-SNAPSHOT</version> </parent> <artifactId>sping-manager-interface</artifactId> <dependencies> <dependency> <groupId>com.itqf</groupId> <artifactId>sping-manager-pojo</artifactId> <version>0.0.1-SNAPSHOT</version> </dependency> </dependencies> </project> Copy code
2.5 create spin Manager Service (implementation class of interface) in the aggregation project The packaging method is war
Because the controller layer is separated from the service layer, when running and starting, the controller and service should be published separately with tomcat, and the configuration files required in the aggregation project should be put into the service, so that the configuration files can be loaded and integrated when Tomcat starts
- The service needs an interface, so it depends on the interface spin manager interface
- The service needs pojo and also needs to call mapper, so you can directly rely on mapper, thinking that mapper has relied on pojo (dependency passing)
- The service needs to be managed by spring. Let spring create objects for the service
- service needs dubbo package (dubbo will be introduced later)
- Service needs to use SOA and publish it as a service
configuration file
SqlMapConfig.xml
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd"> <configuration> </configuration> Copy code
db.properties
db.driver=com.mysql.jdbc.Driver db.url=jdbc:mysql://localhost:3306/taotao?characterEncoding=UTF-8 db.username=root db.password=root Copy code
applicationContext-tx.xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:p="http://www.springframework.org/schema/p" xmlns:context="http://www.springframework.org/schema/context" xmlns:mvc="http://www.springframework.org/schema/mvc" xmlns:tx="http://www.springframework.org/schema/tx" xmlns:aop="http://www.springframework.org/schema/aop" xmlns:dubbo="http://code.alibabatech.com/schema/dubbo" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.2.xsd http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-4.2.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-4.2.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-4.2.xsd http://code.alibabatech.com/schema/dubbo http://code.alibabatech.com/schema/dubbo/dubbo.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.2.xsd"> <bean id="txManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager"> <property name="dataSource" ref="dataSource"></property> </bean> <tx:advice id="adviceId" transaction-manager="txManager"> <tx:attributes> <tx:method name="add*" propagation="REQUIRED"/> <tx:method name="save*" propagation="REQUIRED"/> <tx:method name="insert*" propagation="REQUIRED"/> <tx:method name="update*" propagation="REQUIRED"/> <tx:method name="del*" propagation="REQUIRED"/> <tx:method name="find*" propagation="SUPPORTS" read-only="true"/> <tx:method name="get*" propagation="SUPPORTS" read-only="true"/> </tx:attributes> </tx:advice> <aop:config> <aop:advisor advice-ref="adviceId" pointcut="execution(* com.itqf.service..*.*(..))"/> </aop:config> </beans> Copy code
applicationContext-dao.xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:p="http://www.springframework.org/schema/p" xmlns:context="http://www.springframework.org/schema/context" xmlns:mvc="http://www.springframework.org/schema/mvc" xmlns:dubbo="http://code.alibabatech.com/schema/dubbo" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.2.xsd http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-4.2.xsd http://code.alibabatech.com/schema/dubbo http://code.alibabatech.com/schema/dubbo/dubbo.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.2.xsd"> <context:property-placeholder location="classpath:resource/*.properties"/> <bean id="dataSource" class="com.alibaba.druid.pool.DruidDataSource"> <property name="driverClassName" value="${db.driver}"></property> <property name="url" value="${db.url}"></property> <property name="username" value="${db.username}"></property> <property name="password" value="${db.password}"></property> <property name="maxActive" value="10"></property> <property name="minIdle" value="5"></property> </bean> <bean class="org.mybatis.spring.SqlSessionFactoryBean"> <property name="dataSource" ref="dataSource"></property> <property name="configLocation" value="classpath:mybatis/SqlMapConfig.xml"></property> </bean> <bean class="org.mybatis.spring.mapper.MapperScannerConfigurer"> <property name="basePackage" value="com.itqf.mapper"></property> </bean> </beans> Copy code
applicationContext-service.xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:p="http://www.springframework.org/schema/p" xmlns:context="http://www.springframework.org/schema/context" xmlns:mvc="http://www.springframework.org/schema/mvc" xmlns:dubbo="http://code.alibabatech.com/schema/dubbo" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.2.xsd http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-4.2.xsd http://code.alibabatech.com/schema/dubbo http://code.alibabatech.com/schema/dubbo/dubbo.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.2.xsd"> <context:component-scan base-package="com.itqf.service"></context:component-scan> </beans> Copy code
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <groupId>com.qianfeng</groupId> <artifactId>sping-manager</artifactId> <version>0.0.1-SNAPSHOT</version> </parent> <artifactId>sping-manager-service</artifactId> <packaging>war</packaging> <dependencies> <dependency> <groupId>com.qianfeng</groupId> <artifactId>sping-manager-interface</artifactId> <version>0.0.1-SNAPSHOT</version> </dependency> <dependency> <groupId>com.qianfeng</groupId> <artifactId>sping-manager-mapper</artifactId> <version>0.0.1-SNAPSHOT</version> </dependency> <!-- Spring --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-beans</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-webmvc</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-jdbc</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-aspects</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-jms</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context-support</artifactId> <version>${spring.version}</version> </dependency> </dependencies> </project> Copy code
Final engineering structure
3 publish the service using dubbo
Think first?
Shopping mall websites like Taobao Jingdong can log in not only from the PC but also from the mobile phone. So how is it realized? Write two controller s? It certainly won't. If anyone is idle looking for something to do, then SOA (Service-Oriented Architecture) needs to be used When we write a project using distributed, many systems will call each other. If we call back and forth, the code structure will be very chaotic Here we use dubbo to manage and solve the problem that there are too many publishing services
What is SOA
SOA is a design method, which contains multiple services, and the cooperation between services will eventually provide a series of functions. A service usually exists in the operating system process in a separate form. Services communicate through network calls rather than in-process calls.
For example, you now have many services: news services (providing news release, viewing, modification and deletion), Order services (order addition, order modification, order viewing, order deletion, etc.) financial services (revenue, expenditure, statistics, etc.) employee services (add, modify, view, statistics) attendance services (sign in, sign out, export, statistics, etc.) sales services (sales reporting, sales statistics.)
Advantages and disadvantages of SOA
dubbo
What is Dubbo (the management tool of resource scheduling and Governance Center)
With the development of the Internet and the continuous expansion of the scale of website applications, the conventional vertical application architecture can no longer cope with it. The distributed service architecture and mobile computing architecture are imperative. There is an urgent need for a governance system to ensure the orderly evolution of the architecture.
- Single application architecture
- When the website traffic is very small, only one application is needed to deploy all functions together to reduce deployment nodes and costs.
- At this time, the data access framework (ORM) used to simplify the workload of addition, deletion, modification and query is the key.
- Vertical application architecture
- When the number of visits increases gradually, the acceleration brought by a single application increases and the machine decreases. Split the application into several unrelated applications to improve efficiency.
- at this time, the Web framework (MVC) for accelerating front-end page development is the key.
- Distributed service architecture
- When there are more and more vertical applications, the interaction between applications is inevitable. Extract the core business as an independent service, and gradually form a stable service center, so that the front-end application can respond to the changing market demand more quickly.
- At this time, the distributed service framework (RPC) used to improve business reuse and integration is the key.
- Flow computing architecture
- At this time, the pressure of real-time service utilization based on cluster capacity is gradually increasing, and the utilization rate of real-time service based on cluster management is gradually increasing.
- At this time, the resource scheduling and Governance Center (SOA) for improving machine utilization is the key. Construction of Dubbo environment:
Node role description:
- Provider: the service provider that exposes the service.
- Consumer: the service consumer that calls the remote service.
- Registry: Registry for service registration and discovery.
- Monitor: the monitoring center that counts the number and time of service calls.
- Container: service running container.
Description of calling relationship:
- The service container is responsible for starting, loading and running the service provider.
- When a service provider starts, it registers its services with the registry.
- Service consumers subscribe to the services they need from the registry when they start.
- The registry returns the service provider address list to the consumer. If there is any change, the registry will push the change data to the consumer based on the long connection.
- From the provider address list, the service consumer selects one provider to call based on the soft load balancing algorithm. If the call fails, it selects another provider to call.
- Service consumers and providers accumulate call times and call times in memory, and regularly send statistical data to the monitoring center every minute.
Here we mainly come to the registry. We use Zookeeper as the registry
Deploy the registry in linux environment, Zookeeper
- The first step, of course, is to open the virtual machine. I'll do it in CentOS 7
- Get a compressed package of Zookeeper on the Internet. Mine is Zookeeper-3.4.6 tar. gz
- Paste it into the / opt directory and unzip it (jdk environment is required. If there is no jdk environment, install a jdk first)
- Enter the zookeeper-3.4.6 directory and create a folder called data.
- Handle/ Zoo in conf directory_ sample. CFG is renamed zoo cfg
- Modify zoo Data attribute in CFG: dataDir=/opt/zookeeper-3.4.6/data
- Step 7: start zookeeper
- Start:/ zkServer.sh start
- Off:/ zkServer.sh stop
- View status:/ zkServer.sh status
Note that the firewall must be turned off after zookeeper is started!!! That's it
In the ApplicationContext service Add configuration file in XML to publish service
<!-- use dubbo Publishing services --> <!-- Indicate the project where the service is located --> <dubbo:application name="sping-manager"/> <!-- Specify registry adress the address is linux Medium ip Address plus port number,zookeeper The default port number for is 2181 --> <dubbo:registry protocol="zookeeper" address="10.0.117.198:2181" ></dubbo:registry> <!-- Expose services to a port port Is the port number,Select a port that is not occupied --> <dubbo:protocol name="dubbo" port="20888"></dubbo:protocol> <!-- Publishing services,ref yes Spring Container created Service The name of the object --> <dubbo:service interface="com.itqf.service.ItemService" ref="itemServiceImpl" timeout="6000000"></dubbo:service> Copy code
In the POM of service Import package from XML
<!-- dubbo relevant --> <dependency> <groupId>com.alibaba</groupId> <artifactId>dubbo</artifactId> <!-- Exclude dependencies --> <exclusions> <exclusion> <groupId>org.springframework</groupId> <artifactId>spring</artifactId> </exclusion> <exclusion> <groupId>org.jboss.netty</groupId> <artifactId>netty</artifactId> </exclusion> </exclusions> </dependency> <dependency> <groupId>org.apache.zookeeper</groupId> <artifactId>zookeeper</artifactId> </dependency> <dependency> <groupId>com.github.sgroschupf</groupId> <artifactId>zkclient</artifactId> </dependency> Copy code
4. Create a spin manager controller at the same level as the aggregation project Import dependency
contoller needs to use dubbo to access the services published by the service layer To publish using Tomcat server, spring MVC is also needed
xml
<dependencies> <!-- Just rely on the business interface --> <dependency> <groupId>com.qianfeng</groupId> <artifactId>sping-manager-interface</artifactId> <version>0.0.1-SNAPSHOT</version> </dependency> <!-- Spring --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-beans</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-webmvc</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-jdbc</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-aspects</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-jms</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context-support</artifactId> <version>${spring.version}</version> </dependency> <!-- JSP relevant --> <dependency> <groupId>jstl</groupId> <artifactId>jstl</artifactId> </dependency> <dependency> <groupId>javax.servlet</groupId> <artifactId>servlet-api</artifactId> <scope>provided</scope> </dependency> <dependency> <groupId>javax.servlet</groupId> <artifactId>jsp-api</artifactId> <scope>provided</scope> </dependency> <!-- dubbo relevant --> <dependency> <groupId>com.alibaba</groupId> <artifactId>dubbo</artifactId> <!-- Exclude dependencies --> <exclusions> <exclusion> <groupId>org.springframework</groupId> <artifactId>spring</artifactId> </exclusion> <exclusion> <groupId>org.jboss.netty</groupId> <artifactId>netty</artifactId> </exclusion> </exclusions> </dependency> <dependency> <groupId>org.apache.zookeeper</groupId> <artifactId>zookeeper</artifactId> </dependency> <dependency> <groupId>com.github.sgroschupf</groupId> <artifactId>zkclient</artifactId> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.apache.tomcat.maven</groupId> <artifactId>tomcat7-maven-plugin</artifactId> <configuration> <port>8081</port> <path>/</path> </configuration> </plugin> </plugins> </build> Copy code
spingmvc.xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:p="http://www.springframework.org/schema/p" xmlns:context="http://www.springframework.org/schema/context" xmlns:mvc="http://www.springframework.org/schema/mvc" xmlns:tx="http://www.springframework.org/schema/tx" xmlns:aop="http://www.springframework.org/schema/aop" xmlns:dubbo="http://code.alibabatech.com/schema/dubbo" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.2.xsd http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-4.2.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-4.2.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-4.2.xsd http://code.alibabatech.com/schema/dubbo http://code.alibabatech.com/schema/dubbo/dubbo.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.2.xsd"> <context:component-scan base-package="com.itqf.controller"></context:component-scan> <mvc:annotation-driven></mvc:annotation-driven> <bean class="org.springframework.web.servlet.view.InternalResourceViewResolver"> <property name="prefix" value="/WEB-INF/jsp/"></property> <property name="suffix" value=".jsp"></property> </bean> <!--Indicate the project --> <dubbo:application name="sping-manager-controller"/> <!-- Specify registry --> <dubbo:registry protocol="zookeeper" address="10.0.117.198:2181"></dubbo:registry> <!--Call service --> <dubbo:reference interface="com.itqf.service.ItemService" id="itemService"></dubbo:reference> </beans> Copy code
5 create spin common
This is a special place for tools that I need to use. It is used to put some things for public needs; pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <groupId>com.itqf</groupId> <artifactId>sping-parent</artifactId> <version>0.0.1-SNAPSHOT</version> </parent> <groupId>com.itqf</groupId> <artifactId>sping-common</artifactId> <version>0.0.1-SNAPSHOT</version> <dependencies> <!-- Time operation component --> <dependency> <groupId>joda-time</groupId> <artifactId>joda-time</artifactId> <version>${joda-time.version}</version> </dependency> <!-- Apache Tool assembly --> <dependency> <groupId>org.apache.commons</groupId> <artifactId>commons-lang3</artifactId> <version>${commons-lang3.version}</version> </dependency> <dependency> <groupId>org.apache.commons</groupId> <artifactId>commons-io</artifactId> <version>${commons-io.version}</version> </dependency> <dependency> <groupId>commons-net</groupId> <artifactId>commons-net</artifactId> <version>${commons-net.version}</version> </dependency> <!-- Jackson Json Processing kit --> <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-databind</artifactId> <version>${jackson.version}</version> </dependency> </dependencies> </project> Copy code
6 test
Well, such a pseudo distributed project is built. Next, it is very important to install the parent project, the aggregation project of spin manager and spin common into the local warehouse. Otherwise, an error will be reported when starting the project that the parent project or other projects cannot be found
Final engineering drawing:
Summary:
After several hours of hard work, it was finally built. It was going to blow up my old man's machine If there are any mistakes or deficiencies, please don't hesitate