java lazy development: mybatis generator generates the corresponding entity class, xml Mapper file, interface and help class according to the table design in the database.
Take spring-tool-suite-3.9.2.RELEASE as an example:
install
Introduction package
<dependency> <groupId>org.mybatis.generator</groupId> <artifactId>mybatis-generator-core</artifactId> <version>1.3.6</version> </dependency>
Create mybatis generator configuration file
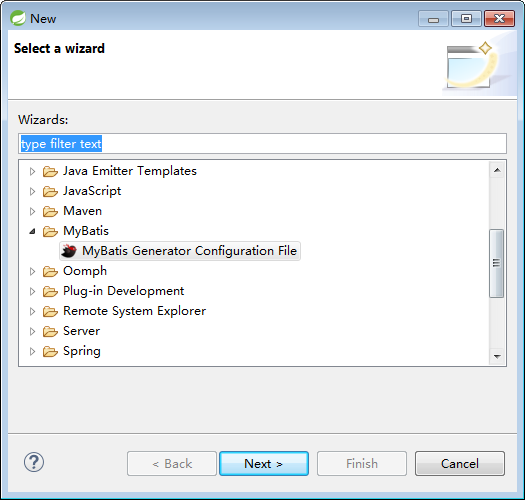
Modify mybatis generator configuration file
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE generatorConfiguration PUBLIC "-//mybatis.org//DTD MyBatis Generator Configuration 1.0//EN" "http://mybatis.org/dtd/mybatis-generator-config_1_0.dtd"> <generatorConfiguration> <context id="prod"> <!-- RowBounds pagination --> <plugin type="org.mybatis.generator.plugins.RowBoundsPlugin" /> <plugin type="org.mybatis.generator.plugins.CaseInsensitiveLikePlugin" /> <plugin type="org.mybatis.generator.plugins.SerializablePlugin" /> <commentGenerator> <property name="suppressDate" value="true" /> <property name="suppressAllComments" value="true" /> </commentGenerator> <!-- jdbc Connect --> <jdbcConnection driverClass="com.mysql.jdbc.Driver" connectionURL="jdbc:mysql://192.168.1.206/testOne" userId="root" password="1234" /> <javaTypeResolver> <property name="forceBigDecimals" value="false" /> </javaTypeResolver> <!-- targetProject:generate PO Location of class --> <javaModelGenerator targetPackage="com.lazy.develop.generator.entity" targetProject="lazyDevelop"> <!-- enableSubPackages:Whether to let schema As suffix of package --> <property name="enableSubPackages" value="false" /> <!-- Spaces before and after values returned from the database are cleaned up --> <property name="trimStrings" value="true" /> <property name="comments" value="true"/> </javaModelGenerator> <!-- targetProject:mapper Location of map file generation --> <sqlMapGenerator targetPackage="com.lazy.develop.generator.mapper" targetProject="lazyDevelop"> <property name="enableSubPackages" value="false" /> </sqlMapGenerator> <!-- targetPackage: mapper Location of interface generation --> <javaClientGenerator type="XMLMAPPER" targetPackage="com.lazy.develop.generator.dao" targetProject="lazyDevelop"> <property name="enableSubPackages" value="false" /> </javaClientGenerator> <!-- Specify database tables --> <table schema="" tableName="user" domainObjectName="User" enableCountByExample="false" enableUpdateByExample="false" enableDeleteByExample="false" enableSelectByExample="false" selectByExampleQueryId="false"> <generatedKey column="id" sqlStatement="MySql" identity="true"/> </table> </context> </generatorConfiguration>
The construction of the project will not be written. It will be Ok if it is integrated into its own framework
Function
If you have plug-ins in your editor, just right-click the mybatis generator configuration file to run it. If you do, run the following main method:
package com.mybatis.test; import org.mybatis.generator.api.ShellRunner; public class App { public static void main(String[] args) { args = new String[] { "-configfile", "src\\main\\resources\\generatorConfig.xml.xml", "-overwrite" }; ShellRunner.main(args); } }