Status of multithreading:
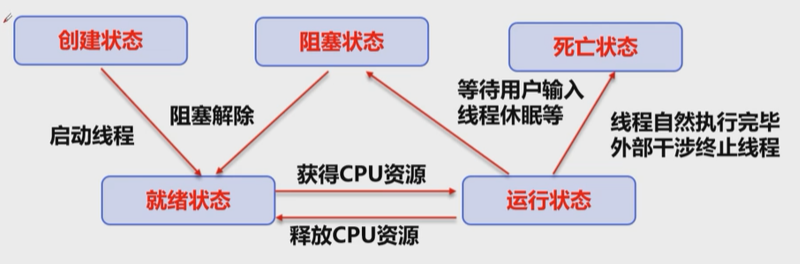
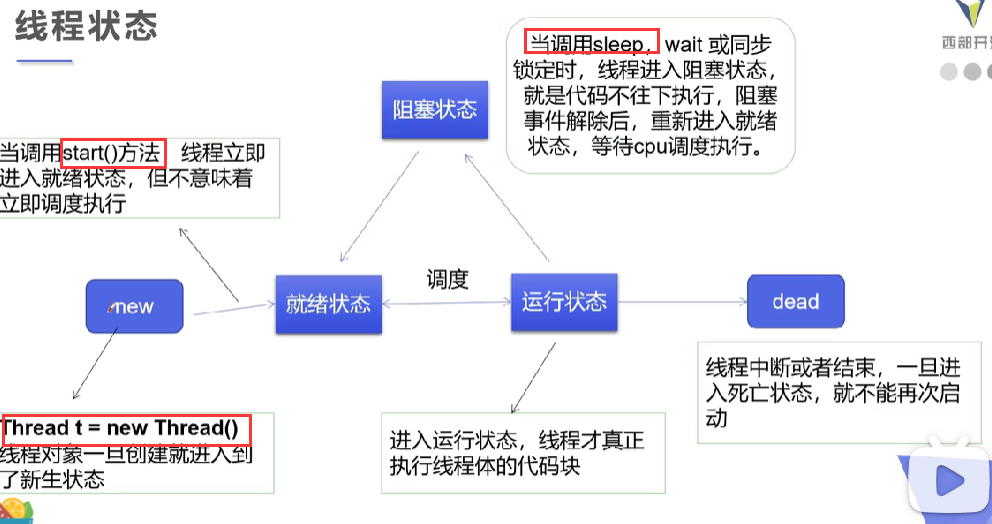
1. Thread stop
- It is recommended that the thread stop normally - > utilization times, and dead loop is not recommended
- It is recommended to use flag bit - > set a flag bit
- Do not use outdated methods such as stop or destroy, or methods not recommended by JDK
Custom stop, you can see that threa stops, but the mian function is still running
package statue; /*Test stop 1. It is recommended that the thread be stopped normally -- "utilization times", and dead loop is not recommended 2.It is recommended to set a flag bit with flag bit -- 3.Do not use outdated methods such as stop or destroy, or methods not recommended by JDK * */ public class TestStop implements Runnable{ //Set an identification bit boolean flag=true; @Override public void run() { int i=0; while (flag){ System.out.println("run Thread"+ i++); } } //2 set a public method, stop the thread and convert the flag public void stop(){ this.flag=false; } public static void main(String[] args) { TestStop testStop=new TestStop(); new Thread(testStop).start(); for (int i = 0; i < 1000; i++) { System.out.println("main"+i); if (i==900){ testStop.stop(); System.out.println("run Stop!"); } } } }
2. Sleep thread
- sleep can simulate network delay and amplify the occurrence of problems.
- Application examples: multi thread ticket selling, countdown and obtaining system time.
- Every object has a lock, and sleep will not release the lock.
package statue; import java.text.SimpleDateFormat; import java.util.Date; public class TestSleep { public static void main(String[] args) throws InterruptedException { try { tenDown();//count down } catch (InterruptedException e) { e.printStackTrace(); } //Print current system time Date startTime= new Date(System.currentTimeMillis()); while (true) { Thread.sleep(1000); System.out.println(new SimpleDateFormat("HH:MM:SS").format(startTime)); startTime=new Date(System.currentTimeMillis()); //Update time } } public static void tenDown() throws InterruptedException { int num=3; while (true) { Thread.sleep(1000); System.out.println(num--); if (num<=0){ break; } } } }
result
3 2 1 16:02:508 16:02:529
3. Comity
- Comity thread, which suspends the thread currently executing, but does not block
- Change the program from running state to ready state
- cpu rescheduling, but comity is not necessarily successful. It depends on the mood of cpu
- Comity and competition at the same time. For example, currently A is running and B is ready. After comity, A exits the running state. At this time, A and B compete for resources at the same time. If A runs, comity is unsuccessful.
package statue; public class TestYield { public static void main(String[] args) { MyYield myYield=new MyYield(); new Thread(myYield,"a").start(); new Thread(myYield,"b").start(); } } class MyYield implements Runnable{ @Override public void run() { System.out.println(Thread.currentThread().getName()+"The thread starts executing"); Thread.yield();//Comity System.out.println(Thread.currentThread().getName()+"Thread stop"); } }
4.Join
- Join merge threads. After this thread completes execution, execute other threads. Other threads are blocked
- You can imagine jumping in line
package statue; public class TestJoin implements Runnable{ @Override public void run() { for (int i = 0; i < 10; i++) { System.out.println("thread vip coming"+i); } } public static void main(String[] args) throws InterruptedException { //Start our thread TestJoin testJoin=new TestJoin(); Thread thread =new Thread(testJoin); thread.start(); //Main thread for (int i = 0; i < 60; i++) { if (i==20){ thread.join();//Jump in line } System.out.println("main"+i); } } }
5. Observe thread status
Use: thread State state = thread. getState();
public class TestState { public static void main(String[] args) { Thread thread = new Thread(() -> { for (int i = 0; i < 5; i++) { try { Thread.sleep(1000); } catch (InterruptedException e) { e.printStackTrace(); } } System.out.println("///"); }); //Observation state Thread.State state = thread.getState(); //NEW System.out.println(state); //Observe after startup //Start thread thread.start(); state = thread.getState(); System.out.println(state); //As long as the thread does not terminate, it will always output the state while (state != Thread.State.TERMINATED) { try { Thread.sleep(100); //Update thread status state = thread.getState(); System.out.println(state); } catch (InterruptedException e) { e.printStackTrace(); } } } }
6. Thread priority
getPriority() setPriority(int xxx)
- Set priority before starting
- Maindefault priority 5
- Priority 1-10
package statue; public class TestPriority { public static void main(String[] args) { //Main thread output System.out.println(Thread.currentThread().getName()+"-->"+Thread.currentThread().getPriority()); MyPriority myPriority = new MyPriority(); Thread t1 = new Thread(myPriority ); Thread t2 = new Thread(myPriority ); Thread t3 = new Thread(myPriority ); Thread t4 = new Thread(myPriority ); Thread t5 = new Thread(myPriority ); Thread t6 = new Thread(myPriority ); t1.start(); //Set priority before starting t2.setPriority(3); t2.start(); t3.setPriority(5); t3.start(); t4.setPriority(7); t4.start(); t5.setPriority(Thread.MIN_PRIORITY); t5.start(); t6.setPriority(Thread.MAX_PRIORITY); t6.start(); } } class MyPriority implements Runnable{ @Override public void run() { System.out.println(Thread.currentThread().getName()+"--->"+Thread.currentThread().getPriority()); } }
It can be seen that if the priority is not high, it will be executed first. If the thread priority is low, it just means that the probability of obtaining scheduling is low. It is not that if the priority is low, it will not be called. Look at CPU scheduling
main-->5 Thread-0--->5 Thread-4--->1 Thread-5--->10 Thread-3--->7 Thread-1--->3 Thread-2--->5
7. Daemon thread
- Threads are divided into user threads and daemon threads
- The virtual machine must ensure that the user thread has completed execution
- The virtual machine does not have to wait for the daemon thread to finish executing
- For example, record operation logs in the background, monitor memory, garbage collection and wait...
- The daemon thread will also finish executing after the user thread finishes executing, but the JVM will take some time to stop
- thread.setDaemon(true);// By default, flash is the user thread, and true is the daemon thread
God is watching over you
package statue; public class TestDaemon { public static void main(String[] args) { God god = new God(); You you=new You(); Thread thread = new Thread(god); thread.setDaemon(true);//By default, flash is the user thread, and true is the daemon thread thread.start(); new Thread(you).start(); } } class God implements Runnable{ @Override public void run() { while (true){ System.out.println("God is watching over you"); } } } class You implements Runnable{ @Override public void run() { for (int i = 0; i <36500 ; i++) { System.out.println("Live happily"); } System.out.println("----goodbye!------"); } }