- After using static import, you can omit the class name to access static members (member variables, methods, nested classes)
Classic usage scenarios for static import:
import static java.lang.Math.PI; public class Main { public static void main(String[] args) { System.out.println(2 * PI * 10); System.out.println(2 * PI * 20); } }
Using static import correctly can eliminate some duplicate class names and improve code readability;
Excessive use of static import will make readers confused about the class in which static members are defined;
Recommendation: use static import with caution.
Initialization of member variables
===========================================================================
The compiler will automatically set the initial value for uninitialized member variables;
How to manually provide initial values for instance variables?
-
In the statement
-
In the construction method
-
In initialization block
The compiler copies the initialization block to the header of each constructor (the initialization block is executed every time an instance object is created)
How to manually provide initial values for class variables?
-
In the statement
-
In static initialization block
Execute static initialization block when a class is initialized;
When a class is actively used for the first time, the JVM initializes the class;
Initialization block, static initialization block
public class Person { static { // Static initialization block System.out.println("static block"); } { // Initialization block System.out.println("block"); } public Person() {} public Person(int age) {} public static void main(String[] args) { new Person(); // static block // block new Person(20); // block } }
A more complex example:
public class Person { static { System.out.println("Person static block"); } { System.out.println("Person block"); } public Person() { System.out.println("Person constructor"); }
public class Student extends Person {
static { System.out.println("Student static block"); } { System.out.println("Student block"); } public Student() { System.out.println("Student constructor"); }
}
**Execution sequence**: Parent static block -> Subclass static block -> Parent code block -> Parent constructor -> Subclass code block -> Subclass constructor
public static void main(String[] args) {
new Student(); // Person static block // Student static block // Person block // Person constructor // Student block // Student constructor
}
[](https://gitee.com/vip204888/java-p7) Singleton Pattern ========================================================================================== If a class is designed in singleton mode, only one instance of this class can be created during the running of the program. **Hungry Han single case mode**: Like a starving man, he directly created the only instance. (thread safe)
/*
- Hungry Han single case mode
*/
public class Rocket {
private static Rocket instance = new Rocket(); private Rocket(){} public static Rocket getInstance() { return instance; }
}
**Lazy singleton mode**: Like a lazy man, you can create instances only when you need them. (thread unsafe)
/*
- Lazy singleton mode
*/
public class Rocket {
private static Rocket instance = null; private Rocket(){} public static Rocket getInstance() { if (instance == null) { instance = new Rocket(); } return instance; }
}
[](https://gitee.com/vip204888/java-p7)final, Constant ===================================================================================== cover `final` Decorated classes:**Cannot be subclassed or inherited** cover `final` Modification method:**Cannot be overridden** cover `final` Modified variables:**Only one assignment can be made** Constant writing: * `public static final double PI = 3.14159265358979323846;` * `private static final int NOT_FOUND = - 1;` If will**Basic type**or**A string is defined as a constant**,also**The value can be determined at compile time**: * **The compiler replaces constant names everywhere with constant values**(be similar to C Macro replacement for language) * be called**Compile-time constant **( compile-time constant) For example,**It will be replaced at compile time**:
public class Main {
static final int A = 123456; static final String B = "HELLO"; public static void main(String[] args) { System.out.println(A); // At compile time, it is directly replaced with the following // System.out.println(123456); System.out.println(B); // At compile time, it is directly replaced with the following // System.out.println("HELLO"); }
}
In this case,**The value cannot be determined at compile time and will not be replaced**:
public class Main {
static int NUMBER = getNum(); static int getNum() { int a = 10; int b = 20; return a + b * 2 + 6; } public static void main(String[] args) { System.out.println(NUMBER); // Will not be replaced }
}
[](https://gitee.com/vip204888/java-p7) Nested Class ==================================================================================== * **Nested class**: A class defined in another class; * A class outside a nested class is called:**External class**(Outer Class) * The outermost outer class is called:**Top class**(Top-level Class)
public class OuterClass {/ / top class
// Static Nested Class static class StaticNestedClass { } // Non static nested class (inner class) class InnerClass { }
}
[](https://gitee.com/vip204888/java-p7) Inner Class ----------------------------------------------------------------------------------- **Inner class**: Not by `static` Decorated nested classes,**Non static nested class** Like instance variables and instance methods, internal classes are associated with instances of external classes: * You must first create an external class instance, and then create an internal class instance with an external class instance * Inner class cannot be defined except**Compile-time constant **Any other than static member **Inner class and outer class**: * The inner class has direct access to all members in the outer class (even the `private`) * External classes can directly access member variables and methods of internal class instances (even `private`) Internal class example: first company `company` To have employees `employee`,take `employee` Set to `company` Internal class of, and `company` Can access `employee` Member variables and methods (including`private`),`employee` Can access `company` All members (including `private`).
public class Company {
private String name; public Company(String name) { this.name = name; } public void fire(Employee e) { // External classes can directly access member variables (including private) of internal class instances System.out.println(name + " fire " + e.no); } public class Employee { private int no; public Employee(int no) { this.no = no; } public void show() { // The inner class can directly access all members (including private) in the outer class System.out.println(name + " : " + no); } } public static void main(String[] args) { Company c = new Company("Google"); Employee e = c.new Employee(17210224); e.show(); c.fire(e); }
}
**Details of internal classes**: If so**Homonymous variable**,The default access is internal, and the external access needs to be specially pointed out.
public class OuterClass {
private int x = 1; // External class variable x public class InnerClass { private int x = 2; // Internal class variable x public void show() { // Default access internal System.out.println(x); // 2 System.out.println(this.x); // 2 // This is required to access the variable with the same name of the external class System.out.println(OuterClass.this.x); // 1 } } public static void main(String[] args) { new OuterClass().new InnerClass().show(); }
}
### []( https://gitee.com/vip204888/java-p7 )Internal class memory distribution
public class Person {
private int age; public class Hand { private int weight; } public static void main(String[] args) { // You must have a Person object before you can create a Hand object Person p1 = new Person(); Hand h1 = p1.new Hand(); Person p2 = new Person(); Hand h2 = p2.new Hand(); }
}
stay `Hand` Before the class is released,`Person` Class will not be released `Hand` (pointing) 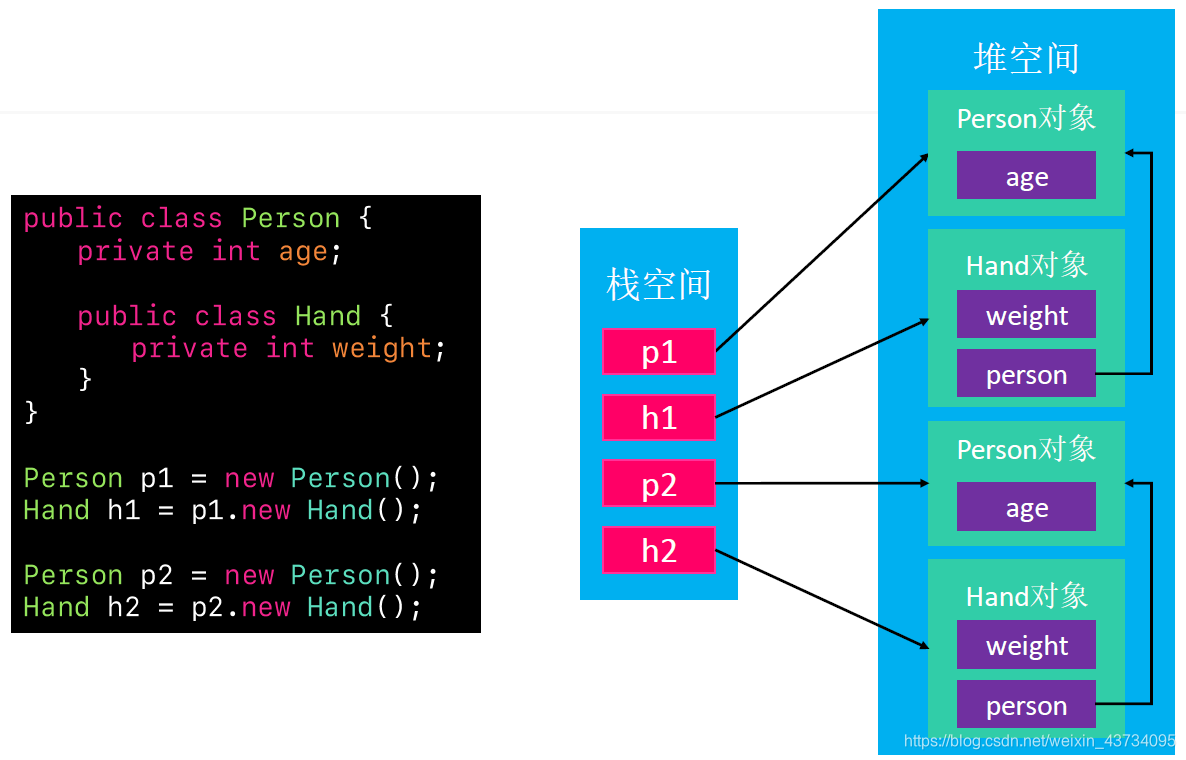 [](https://gitee.com/vip204888/java-p7) Static Nested Class --------------------------------------------------------------------------------------------- **Static Nested Class **: cover `static` Embellished**Nested class**; Static nested classes in**Behavior**It is a top-level class, but the defined code is written in another class; Compared with general top-level classes, static nested classes have some more special permissions * Members in external classes can be accessed directly (even if declared as `private`)
public class Person {
private int age; private static int count = 1; private static void run() { System.out.println("Person - run"); } public static class Car { // Static Nested Class public void test() { Person person = new Person(); // Static nested classes can directly access members (including private) in external classes System.out.println(person.age); // 0 Person.count = 1; Person.run(); // Person - run System.out.println(count); // 1 run(); // Person - run } }
}
public static void main(String[] args) { Person p = new Person(); // Use of static nested classes Person.Car c = new Person.Car(); // If you previously imported person Car; Can be used directly; // Car c = new Car(); c.test(); } ``` [](https://gitee.com/vip204888/java-p7) what is the use of nested classes? ----------------------------------------------------------------------------- If class A Only in class C Internally, consider adding classes A Nest to class C Medium; * Better encapsulation * More simplified packages * Enhance readability and maintainability If class A You need to access classes frequently C of**Non public member**,Consider adding classes A Nest to class C Medium; * In addition, you can add classes as needed A Hide from the public if necessary**Frequent access to non-public instance members**,It is designed as an internal class (non static nested class), otherwise it is designed as a static nested class; * If you have to C Instance to create A Instance, you can A Designed as C Inner class of [](https://gitee.com/vip204888/java-p7) Local Class =================================================================================== **Local class**: Defined in**Code block**Classes in (can be defined in methods for In circulation if Statement (medium) A local class cannot define a division**Compile-time constant **Any other than `static` Members; Local classes can only access `final` perhaps **Effective`final`** Local variables of; * from Java 8 Start, if**The local variable is not assigned a second time**,It's considered yes**Effective`final`** A local class can directly access all members in an external class (even if declared as `private`) * Local classes can directly access instance members (instance variables and instance methods) in external classes only if they are defined in the instance related code block Local class example: ``` public class TestLocalClass { private int a = 1; private static int b = 2; private static void test1() {} private void test2() {} public void test3() { int c = 2; class LocalClass { static final int d = 4; void test4() { System.out.println(a + b + c + d); test1(); test2(); } } new LocalClass().test4(); } } ``` [](https://gitee.com/vip204888/java-p7) Abstract Class and Interface ==================================================================================================== [](https://gitee.com/vip204888/java-p7) abstract class ---------------------------------------------------------------------- **Abstract method**: cover `abstract` Modified instance method * Only method declaration, no method implementation (there is no curly bracket after the parameter list, but a semicolon) * Can't be `private` Permission (because the purpose of defining abstract methods is for subclasses to implement) * It can only be defined in abstract classes and interfaces **abstract class**: cover `abstract` Modified class * Abstract methods can be defined * You cannot instantiate, but you can customize the constructor * A subclass must implement all abstract methods in the abstract parent class (unless the subclass is also an abstract class) * Like a non abstract class, you can define member variables, constants, nested types, initialization blocks, non abstract methods, and so on That is to say,**Abstract classes can also define no abstract methods at all** Common usage scenarios: * Extract the public implementation of the subclass into the abstract parent class, and define the subclass as an abstract method that must be implemented separately example: ``` public abstract class Shape { protected double area; protected double girth; public double getArea() { return area; } public double getGirth() { return girth; } public void show() { calculate(); System.out.println(area + "_" + girth); } protected abstract void calculate(); }
public class Rectangle extends Shape {
private double width; private double height; public Rectangle(double width, double height) { super(); this.width = width; this.height = height; } @Override protected void calculate() { area = width * height; girth = (width + height) * 2; }
}
public class Circle extends Shape { private double radius; public Circle(double radius) { this.radius = radius; } @Override protected void calculate() { double half = Math.PI * radius; area = half * radius; girth = half * 2; } }
public static void main(String[] args) {
Rectangle rectangle = new Rectangle(10, 20); rectangle.show(); Circle circle = new Circle(30); circle.show();
}
[](https://gitee.com/vip204888/java-p7) Interface -------------------------------------------------------------------------------- **API**(Application Programming Interface) * **Application programming interface**,A set of functions provided for developers to call (without providing source code) **Java Interface in**: * A collection of method declarations * Used to define specifications and standards **What can be defined in the interface**: * **Abstract method (can be omitted) abstract)** * **Constant (can be omitted) static,final)** * **Nested Type ** * from Java 8 You can define:**Default method(`default`)**,**Static method** The above can be defined implicitly public Therefore, it can be omitted public keyword * from Java 9 You can define:**private method** * Cannot customize construction methods, define (static) initialization blocks, and instantiate **Interface details**: A class can pass `implements` Keyword implements one or more interfaces * A class that implements an interface must implement all abstract methods defined in the interface unless it is an abstract class * If a class implements the same abstract method in multiple interfaces, it only needs to implement this method once * `extends` and i`mplements` Can be used together,`implements` Must be written in `extends` Behind * When the method signatures in the parent class and interface are the same, the return value type must also be the same An interface can be `extends` Keyword inherits one or more interfaces * When the method signatures in multiple parent interfaces are the same, the return value type must also be the same [](https://gitee.com/vip204888/java-p7) comparison between abstraction and interface (how to choose) --------------------------------------------------------------------------------- The purpose of abstract classes and interfaces is still a little similar. How to choose? When to choose**abstract class**? * stay**Closely related classes**Share code between * Need to divide public Access rights outside * Instance variables and non variables need to be defined final Static variable When to choose**Interface**? * **Unrelated classes**Implement the same method * just**Define behavior**,It doesn't care who has achieved the behavior * The type you want to implement**multiple inheritance** [](https://gitee.com/vip204888/java-p7) interface upgrade (default method, static method) ===================================================================================== If the interface needs to be upgraded, such as adding new abstract methods, it will lead to significant code changes, and the classes that previously implemented the interface will have to be changed If you want to upgrade the interface without changing the previous implementation class, you can Java 8 At first, there are two options: * **Default method**(Default Method) * **Static method**(Static Method) [](https://gitee.com/vip204888/java-p7) Default Method --------------------------------------------------------------------------------------- * use `default` Modify default method * The default method can only be an instance method **Use of default methods**: When there is a default method in the interface implemented by a class, the class can: * **Do nothing**,Follow the default implementation of the interface * Redefine the default method,**cover**Implementation of default method * Redeclare the default method and declare the default method as an abstract method (this class must be an abstract class) When there is a default method in the parent interface inherited by an interface, the interface can: * **Do nothing**,Follow the default implementation of the interface * Redefine the default method,**cover**Implementation of default method * Redeclare the default method and declare the default method as an abstract method Simple example:`Eatable` There are default methods in,`Dog`Do nothing,`Cat` Override the default method.
public interface Eatable {
// Default method default void eat(String name) { System.out.println("Eatable - eat - " + name); }
}
// A new method is added to the Eatable interface, but it does not affect the Dog class public class Dog implements Eatable {}
//Methods are added in the Eatable interface, which can be overridden in Cat classes
public class Cat implements Eatable {
@Override public void eat(String name) { Eatable.super.eat(name); System.out.println("Cat - eat - " + name); }
}
public static void main(String[] args) { Dog dog = new Dog(); dog.eat("bone"); // Eatable - eat - bone Cat cat = new Cat(); cat.eat("fish"); // Eatable - eat - fish // Cat - eat - fish } ``` If the non abstract method defined by the parent class is the same as the default method of the interface, the method of the parent class will eventually be called(**Proximity principle**): ``` public class Animal { public void run() { System.out.println("Animal - run"); } }
public interface Runnable {
default void run() { System.out.println("Runnable - run"); }
}
public class Dog extends Animal implements Runnable {}
public static void main(String[] args) {
Dog dog = new Dog(); dog.run(); // Both the inherited parent class and the implemented interface have run() methods, which call the parent class by default // Animal - run
}
If the abstract method defined by the parent class is the same as the default method of the interface, the child class is required to implement this abstract method * Can pass `super` Keyword calls the default method of the interface
public interface Runnable {
default void run() { System.out.println("Runnable - run"); }
}
public abstract class Animal { public void run() {} }
public class Dog extends Animal implements Runnable {
@Override public void run() { // The run method of the parent class is the same as the run method in the interface. It is required to implement the abstract method of the parent class Runnable.super.run(); // You can call the default method of the interface through super System.out.println("Dog - run"); // Runnable - run // Dog - run }
}
If the default method defined by the (parent) interface is the same as that defined by other (parent) interfaces, the subtype is required to implement this default method: Example:`Runnable` and `Walkable` Defined in two parent interfaces**Default method**All `run()`,`Testable` If you inherit two parent classes, you are required to implement the default method `run()` ,`Dog` Class is the same.
public interface Runnable {
default void run() { System.out.println("Runnable - run"); }
}
public interface Walkable { default void run() { System.out.println("Walkable - run"); } }
//Testable parent interface inherits Runnable parent interface and Walkable parent interface
//They all have the default method run, which requires the Testable interface to implement
public interface Testable extends Runnable, Walkable {
@Override default void run() { Runnable.super.run(); Walkable.super.run(); System.out.println("Testable - run"); }
}
// Dog class implements two interfaces: Runnable and Walkable // They all have the default method run, which requires the Dog class to implement public class Dog implements Runnable, Walkable { @Override public void run() { Runnable.super.run(); Walkable.super.run(); System.out.println("Dog - run"); } }
public static void main(String[] args) {
Dog dog = new Dog(); dog.run(); // Runnable - run // Walkable - run // Dog - run
}
Take another example:
public interface Animal {
default String myself() { return "I am an animal."; }
}
public interface Fire extends Animal {}
public interface Fly extends Animal {
@Override default String myself() { return "I am able to fly."; }
}
public class Dragon implements Fly, Fire {}
public static void main(String[] args) {
Dragon dragon = new Dragon(); System.out.println(dragon.myself()); // I am able to fly.
}
[](https://gitee.com/vip204888/java-p7) Static Method -------------------------------------------------------------------------------------- * Static methods defined in the interface can only be called through the interface name,**Cannot be inherited**;
public interface Eatable {
static void eat(String name) {
last
No matter which company, it attaches great importance to the foundation. Large factories pay more attention to the depth and breadth of technology. The interview is a two-way selection process. Don't interview with a fear mentality, which is not conducive to your own play. At the same time, you should not only focus on the salary, but also see whether you really like the company and whether you can really get exercise.
For the above interview technical points, I also do some information sharing here, hoping to better help you.
Here you can get the following information for free
le, Walkable {
@Override public void run() { Runnable.super.run(); Walkable.super.run(); System.out.println("Dog - run"); }
}
public static void main(String[] args) { Dog dog = new Dog(); dog.run(); // Runnable - run // Walkable - run // Dog - run } ``` Take another example: ``` public interface Animal { default String myself() { return "I am an animal."; } }
public interface Fire extends Animal {}
public interface Fly extends Animal { @Override default String myself() { return "I am able to fly."; } }
public class Dragon implements Fly, Fire {}
public static void main(String[] args) { Dragon dragon = new Dragon(); System.out.println(dragon.myself()); // I am able to fly. } ``` [](https://gitee.com/vip204888/java-p7) Static Method -------------------------------------------------------------------------------------- * Static methods defined in the interface can only be called through the interface name,**Cannot be inherited**; ``` public interface Eatable { static void eat(String name) { # last No matter which company, it attaches great importance to the foundation. Large factories pay more attention to the depth and breadth of technology. The interview is a two-way selection process. Don't interview with a fear mentality, which is not conducive to your own play. At the same time, you should not only focus on the salary, but also see whether you really like the company and whether you can really get exercise. For the above interview technical points, I also do some information sharing here, hoping to better help you. **[Here you can get the following information for free](https://gitee.com/vip204888/java-p7)** [External chain picture transfer...(img-HR7ppDwx-1628596596775)] [External chain picture transfer...(img-Go8TYDXc-1628596596778)] [External chain picture transfer...(img-JqKIveoB-1628596596779)]