public static void main(String[] args) throws InterruptedException { Thread t1 = new Thread(()->{ while (true) { if (Thread.currentThread().isInterrupted()) { System.out.println("Interrupt!"); break; } try { Thread.sleep(2000); } catch (InterruptedException e) { System.out.println("Interrupted When Sleep"); Thread.currentThread().interrupt(); } Thread.yield(); } }); t1.start(); Thread.sleep(1000); t1.interrupt(); } ``` 4. If in sleep When the thread is interrupted, the program will throw an exception and enter exception handling. stay catch In this sentence, since the interrupt has been caught, we can immediately exit the thread, but we don't do so. because**Perhaps in this code, subsequent processing must be carried out to ensure the consistency and integrity of data.**Therefore, it was implemented interrupt()Method interrupts itself again and sets the interrupt flag bit. The only way to do this is to check isInterrupted(),To find that the current thread has been interrupted. You can try catch of interrupt Comment out for verification. 5. Thread.sleep()Method throws an exception due to an interrupt. In this case, it will**Clear the interrupt flag. If it is not processed, it will not be interrupted at the beginning of the next cycle. Therefore, in exception handling, set the interrupt flag bit again.**
-
wait and notify
===================
-
wait and notify are not methods in the Thread class, but in the Object class, which means that any Object can call these two methods.
-
If a thread calls the wait() method, it will be counted into the waiting queue of the object object. There may be multiple threads in this waiting queue because the system runs multiple threads waiting for the same object at the same time. When notify() is called, it will randomly select a thread from the waiting queue and wake it up. But this choice is not fair. It is not that the thread waiting first will be selected first. This choice is completely random.
-
The notifyAll() method wakes up all threads in the waiting queue.
-
Both wait() and notify() methods must be included in the corresponding synchronized statement. Both wait() and notify() need to obtain a monitor of the target object first.
-
After the wait() method is executed, the monitor will be released. When the notify() method is re executed, * * the first thing to do is not to continue to execute the subsequent code, but to try to retrieve the monitor of the object** If it is temporarily unavailable, the thread must also wait for the monitor. When the monitor is successfully obtained, it can continue to execute in a real sense.
-
The difference between the wait() method and sleep() method is that wait releases the lock of the object, while sleep does not release the lock.
-
-
Suspend and resume
========================
-
The suspended thread must wait until the resume operation before continuing to specify
-
However, it has been marked as an abandoned method and is not recommended. Because suspend() will not release any resources while causing the thread to pause. At this time, any thread that wants to access the lock temporarily used by it will be implicated, resulting in failure to run normally. The suspended thread cannot continue operation until the resume() operation is performed on the corresponding thread. However, if the resume operation is executed before suspend, it is difficult for the suspended thread to continue.
-
If you want to implement suspend and resume, you can use wait and notify.
-
-
Wait for the thread to join and yield
==========================
-
The input of a thread may be very dependent on the output of one or more other threads. Therefore, this thread needs to wait for the dependent thread to finish executing before it can continue executing.
-
public final void join() throws InterruptedException public final synchronized void join(long millis) throws InterruptedException
-
The first join() method means to wait indefinitely. It will block the current thread until the target thread has finished executing.
-
The second join() gives a maximum waiting time. If the target thread is still executing after the given time, the current thread will continue to execute because it can't wait.
-
Join means to join. Therefore, if a thread wants to join another thread, the best way is to wait for it to go together.
-
public class JoinMain { public volatile static int i = 0; public static class AddThread extends Thread { @Override public void run() { for (i = 0; i < 1000000; i++); } } public static void main(String[] args) throws InterruptedException { AddThread at = new AddThread(); at.start(); at.join(); System.out.println(i); } }
-
In the main function, if you don't have to wait for AddThread with join(), the i you get is likely to be 0 or a very small number. Because AddThread has not finished executing, the value of i has been output. However, after using the join method, it means that the main thread is willing to wait for the completion of AddThread execution and move forward with AddThread. Therefore, when the join() returns, AddThread execution has been completed, so i is always 1000000;
-
The essence of join is to make the calling thread wait() on the current thread object instance.
-
if (millis == 0) { while (isAlive()) { wait(0); } }
-
As you can see, it calls the thread to wait on the current thread object. After execution, the waiting thread will * * call notifyAll() * * to notify all waiting threads to continue execution before exiting.
-
Therefore, it should be noted that methods such as wait() or notify() should not be used on the Thread object in the application, because it is likely to affect the work of the system API or be affected by the system API.
-
public static native void yield(); ```
- yield() this is a static method. Once executed, it causes the current thread to give up the CPU. After the current thread gives up the CPU, it will compete for CPU resources, but whether it can be allocated again is not certain.
-
-
volatile and Java Memory Model (JMM)
================================= 1. **Java Memory models are developed around atomicity, orderliness and visibility.** 2. Java Tell the virtual machine what special operations or keywords are used. Pay special attention to this place,**The optimization target instruction cannot be changed at will**. **volatile**Is one of them. 3. volatile: Volatile, unstable. 4. When volatile To declare a variable is to tell the virtual machine. This variable is most likely to be modified by some threads. To ensure that all threads within the scope of the application can "see" after this variable is modified. Virtual machine must adopt some special means to ensure the visibility of this variable. 5. volatile No**Replace lock. Nor can we guarantee the atomicity of some operations. volatile No guarantee i++Atomic operation.** 6. **volatile It can ensure the visibility and order of data.**
- Daemon thread
======================= 1. **Daemon thread**It is a special thread and the guardian of the system. It silently completes some systematic services in the background. For example, garbage collection threads, JIT Threads can be understood as daemon threads.
- thread priority
====== 1. Java Use 1~10 Indicates thread priority.**The higher the number, the higher the priority.******
- Synchronization method and synchronization block
========= 1. **Thread synchronization** -------- 1. Because multiple threads of the same process share the same storage space, it not only brings convenience, but also brings the problem of access conflict. In order to ensure the correctness of data access in the method, a lock mechanism is added during access**synchronized,**When a thread obtains the exclusive lock of an object and monopolizes resources, other threads must wait and decide whether to lock it after use. However, there are the following problems: 1. Holding a lock by one thread will cause all other threads that need the lock to hang. 2. In multi-threaded competition, locking and releasing locks will lead to more context switching and scheduling delays, resulting in performance problems 3. If a high priority thread waits for a low priority thread to release the lock, it will lead to priority inversion and performance problems. 2. keyword**synchronized**The function of is to realize the synchronization between threads. Its job is to lock the synchronized code, so that each time, only one thread can enter the synchronization block, so as to ensure the safety between threads. 1. Specify lock object: lock the given object, and obtain the lock of the given object before entering the synchronization code. 2. Direct action on instance method: it is equivalent to locking the current instance. Before entering the synchronization code, you need to obtain the lock of the current instance. 3. Direct action on static methods: it is equivalent to locking the current class. Enter the synchronization code block to obtain the lock of the current class. 3. **synchronized**Except for the guarantee**Thread synchronization,**It can also ensure the communication between threads**Visibility and order.**In terms of visibility, synchronized Can completely replace volatile,It's just not so convenient to use. In terms of order, because synchronized Limit that only one thread can access the synchronization block at a time. No matter how the code in the synchronization block is executed out of order, as long as the serial semantic consistency is guaranteed, the execution result is always the same. 2. Synchronization method ---- 1. Can pass private Keyword to ensure that data objects can only be accessed by methods, so you only need to propose a mechanism for methods, which is synchronized keyword. have**synchronized method**and**synchronized block** 2. synchronized Method controls access to "objects". Each object corresponds to a lock synchronized Methods must obtain the lock of the object calling the method before they can be executed, otherwise the thread will block. Once the method is executed, it will monopolize the lock until the method returns, and the blocked thread can obtain the lock and continue to execute 1. Defect: if a large method is declared as synchronized Will affect efficiency. 3. Synchronization block --- 1. Synchronization block: synchronized(obj){} 2. Obj be called **Synchronization monitor** 1. obj You can make any object,**Shared resources are recommended as synchronization monitors** 2. There is no need to specify a synchronization monitor in the synchronization method, because the synchronization monitor of the synchronization method is this,It's the object itself, or class 3. Synchronization monitor execution 1. The first thread accesses, locks the synchronization monitor, and executes the code in it 2. The second thread is accessed. The return synchronization monitor is locked and cannot be accessed 3. After the first thread is accessed, unlock the synchronization monitor 4. The second thread accesses, finds that the synchronization monitor has no lock, and then locks and accesses
- deadlock
== 1. Multiple threads each occupy some resources, and**Wait for resources occupied by other threads**Can run, resulting in two or more threads waiting for each other's resources to be released and stopping execution. A synchronization block**When you have "locks of more than two objects" at the same time**,A "deadlock" may occur 2. produce**Four necessary conditions for Deadlock:** 1. **Mutually exclusive conditions:**A resource can only be used by one process at a time 2. **Request and hold conditions:**When a process is blocked by a request for resources, it holds on to the obtained resources 3. **Conditions of non deprivation:**The resources obtained by the process cannot be forcibly deprived until they are used up 4. **Cycle waiting conditions:**Several processes form a head to tail cyclic waiting resource relationship 5. Deadlock can be avoided by breaking any one or more of these conditions
- Lock
============== 1. java.util.concurrent.locks.Lock Interface is**A tool that controls access to shared resources by multiple threads**. Locks provide exclusive access to shared resources, with only one thread pair at a time Lock Object is locked, and the thread should obtain the lock before accessing the shared resource Lock object 2. ReentrantLock(Reentrant lock), which has synchronized The same concurrency and memory semantics are commonly used in thread safety control ReentrantLock,You can display lock and release lock. 3. 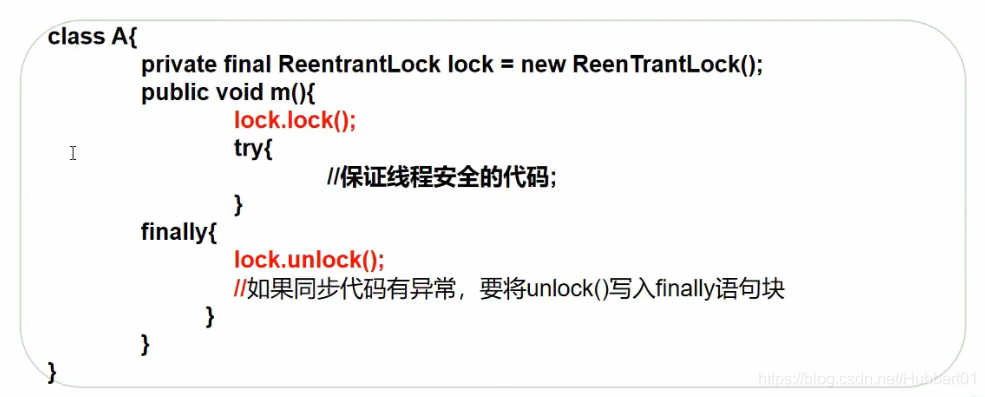
- Synchronized versus Lock
=================== 1. Lock Yes display lock (manually open and close the lock, don't forget to close the lock) synchronized Is an implicit lock, except that the scope is automatically released 2. Lock Only code block locks, synchronized There are code block locks and method locks 3. use Lock Lock, JVM It will take less time to schedule threads for better performance. And it has better scalability 4. Priority order 1. **Lock** \> synchronization**Code block**(Has entered the method body and allocated corresponding resources) > **Synchronization method**((outside the method body)
- Thread collaboration (producer consumer mode)
=============== 1. 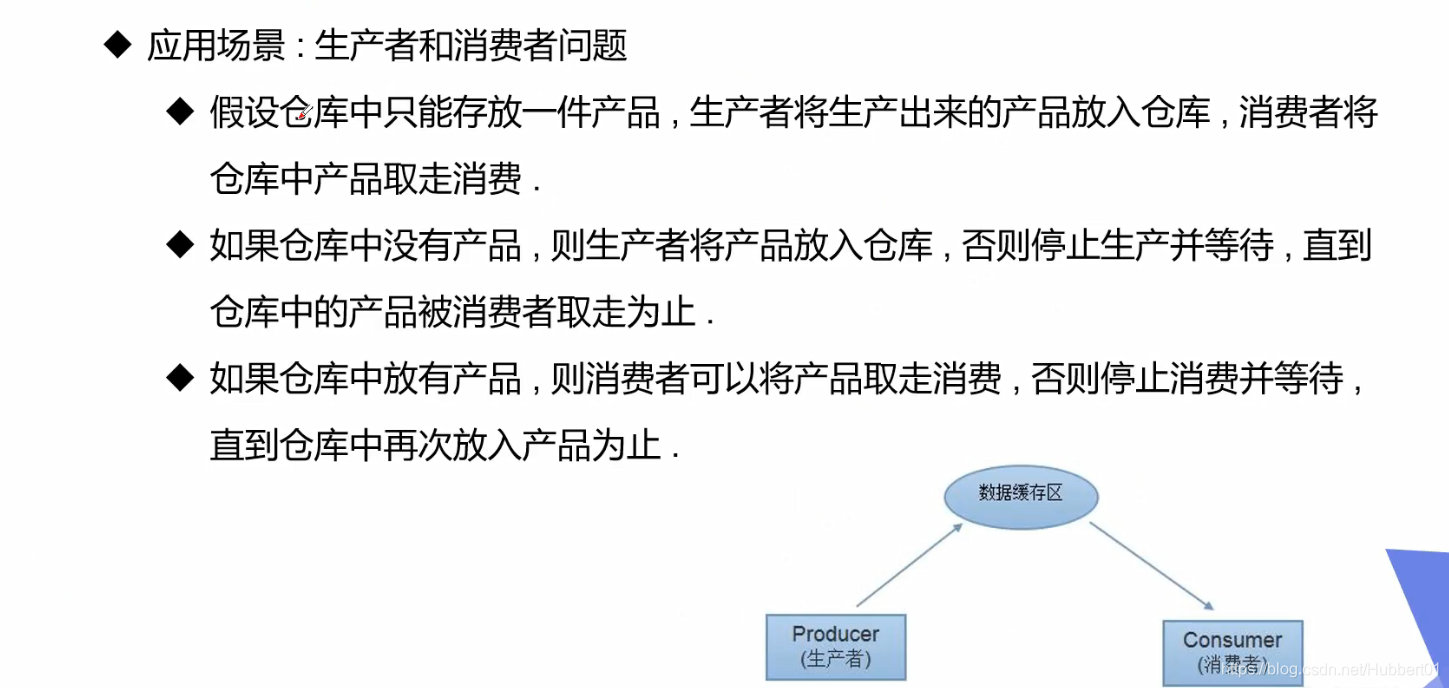
Finally, I share a wave of my interview classic - Java core interview question bank of first-line Internet manufacturers
Here are some of my personal practices. I hope I can provide you with some help:
Click "Java core interview question bank of first-line Internet manufacturers" to get it for free , it has been sorted out for a long time. It is very suitable to review the interview questions, including Java foundation, exception, collection, concurrent programming, JVM, Spring family bucket, MyBatis, Redis, database, middleware MQ, Dubbo, Linux, Tomcat, ZooKeeper, Netty, etc., and will be updated continuously... star!
283 pages of Java advanced core pdf document
Java part: Java foundation, collection, concurrency, multithreading, JVM, design pattern
Data structure algorithm: Java algorithm, data structure
Open source framework: Spring, MyBatis, MVC, netty, tomcat
Distributed part: architecture design, Redis cache, Zookeeper, kafka, RabbitMQ, load balancing, etc
Microservices: SpringBoot, SpringCloud, Dubbo, Docker
There are also source code related reading and learning
34)]
283 pages of Java advanced core pdf document
Java part: Java foundation, collection, concurrency, multithreading, JVM, design pattern
Data structure algorithm: Java algorithm, data structure
Open source framework: Spring, MyBatis, MVC, netty, tomcat
Distributed part: architecture design, Redis cache, Zookeeper, kafka, RabbitMQ, load balancing, etc
Microservices: SpringBoot, SpringCloud, Dubbo, Docker
[external chain picture transferring... (img-KC2iqY3L-1628575496636)]
There are also source code related reading and learning
[external chain picture transferring... (img-qDN5vB9j-1628575496638)]