- Add the Java configuration class EruptConfig of Erupt, subject to the package of starting MallTinyApplication, and configure the package scanning path;
/** * Created by macro on 2021/4/13. */ @Configuration @ComponentScan({"xyz.erupt","com.macro.mall.tiny"}) @EntityScan({"xyz.erupt","com.macro.mall.tiny"}) @EruptScan({"xyz.erupt","com.macro.mall.tiny"}) public class EruptConfig { } Copy code
- Create an erupt database in MySQL, and then run the project using the startup class. The following table will be automatically created in the erupt database;
- After the project is started successfully, you can directly access the login page. The default account password is erupt:erupt, and the project access address is: http://localhost:8080/
- After logging in successfully, we will jump to the project home page. We can find that we don't write a line of front-end code, but have complete permission management and dictionary management functions. Isn't it great!
Implement single table CRUD
CRUD operation can be quickly completed by defining an entity class with core annotations @ Erupt and @ EruptField. Let's take commodity brand management as an example.
- CRUD can be completed with only one entity class without Controller, Service and Dao. First, we create the entity class PmsBrand;
@Erupt(name = "Commodity brand") @Table(name = "pms_brand") @Entity public class PmsBrand { @Id @GeneratedValue(generator = "generator") @GenericGenerator(name = "generator", strategy = "native") @Column(name = "id") @EruptField private Long id; @EruptField( views = @View(title = "Brand name"), edit = @Edit(title = "Brand name",notNull=true,search = @Search(vague = true)) ) private String name; @EruptField( views = @View(title = "Brand initials"), edit = @Edit(title = "Brand initials",notNull=true) ) private String firstLetter; @EruptField( views = @View(title = "brand LOGO"), edit = @Edit(title = "brand LOGO", type = EditType.ATTACHMENT, attachmentType = @AttachmentType(type = AttachmentType.Type.IMAGE)) ) private String logo; @EruptField( views = @View(title = "Large map of brand area"), edit = @Edit(title = "Large map of brand area", type = EditType.ATTACHMENT, attachmentType = @AttachmentType(type = AttachmentType.Type.IMAGE)) ) private String bigPic; @EruptField( views = @View(title = "Brand story"), edit = @Edit(title = "Brand story") ) private String brandStory; @EruptField( views = @View(title = "sort"), edit = @Edit(title = "sort") ) private Integer sort; @EruptField( views = @View(title = "Show"), edit = @Edit(title = "Show") ) private Boolean showStatus; @EruptField( views = @View(title = "Brand manufacturer"), edit = @Edit(title = "Brand manufacturer") ) private Boolean factoryStatus; private Integer productCount; private Integer productCommentCount; } Copy code
- After the item is created successfully, restart the item and add a level-1 menu called commodity in menu maintenance;
- Then add a secondary menu called brand management, pay attention to select the menu type and superior menu, and enter the class name PmsBrand whose type value is entity class;
- After the menu is added successfully, refresh the page and the complete brand management function appears. Let's try to add it;
- If you look at the query list page again, you can find that we have converted the fields of the entity class into different input controls through the @ Edit annotation, such as text box, picture upload box, radio box and value box.
Core notes
Several core notes of Erupt can be learned by comparing the code in PmsBrand!
@Erupt
-
Name: function name
-
desc: function description
@EruptField
-
views: table presentation configuration
-
Edit: edit item configuration
-
sort: front-end display order. The smaller the number, the higher the number
@View
-
title: table column name
-
desc: table column description
-
Type: the data display form. The default is AUTO. You can infer by yourself according to the attribute type
-
show: show
@Edit
-
title: table column name
-
desc: table column description
-
Type: edit type. The default value is AUTO. You can infer from the attribute type
-
show: show
-
notNull: is it required
-
Search: whether search is supported. search = @Search(vague = true) will enable advanced query policy
Of course, Erupt's functions are far more than that. It also integrates many practical system functions, including scheduled tasks, code generator, system monitoring and NoSQL support.
Timed task erupt job
Through the scheduled task function, we can define the scheduled task in the code, and then operate the task in the graphical interface, which is somewhat similar to that mentioned earlier PowerJob How you feel!
- First, we need to be in POM Add erupt job dependency in XML;
<!--Timed task erupt-job--> <dependency> <groupId>xyz.erupt</groupId> <artifactId>erupt-job</artifactId> <version>${erupt.version}</version> </dependency> Copy code
- Then in application Add mail configuration in YML (otherwise an error will be reported when starting);
spring: mail: username: xxxxxx@qq.com password: 123456 host: smtp.exmail.qq.com port: 465 properties: mail.smtp.ssl.auth: true mail.smtp.ssl.enable: true mail.smtp.ssl.required: true Copy code
- Then create a scheduled task implementation class JobHandlerImpl and add the scheduled task execution code in the exec method;
/** * Created by macro on 2021/4/13. */ @Service @Slf4j public class JobHandlerImpl implements EruptJobHandler { @Override public String exec(String code, String param) throws Exception { log.info("The scheduled task has been executed, code:{},param:{}",code,param); return "success"; } } Copy code
- Then restart the application, add a scheduled task in task maintenance, and execute it every 5 seconds;
- After adding successfully, the scheduled task starts to execute. Click the Log button in the task list to view the execution log.
Code generator erupt generator
If you find handwriting entity classes troublesome, you can also use the code generator in Erupt.
- In POM Add the dependency of erupt generator in XML;
<!-- Code generator erupt-generator --> <dependency> <groupId>xyz.erupt</groupId> <artifactId>erupt-generator</artifactId> <version>${erupt.version}</version> </dependency> Copy code
- In the code generation menu, we can directly add tables and fields as in Navicat to generate entity class code;
- During the addition process, we can find that Erupt supports many editing types, up to 30;
- After adding successfully, click the code preview button of the list item to directly generate the code and copy it to your own project.
System monitoring erupt monitor
By using Erupt's system monitoring function, we can view the server configuration, Redis cache usage and online user information.
- In POM Add erupt monitor dependency in XML;
<!--Server monitoring erupt-monitor--> <dependency> <groupId>xyz.erupt</groupId> <artifactId>erupt-monitor</artifactId> <version>${erupt.version}</version> </dependency> Copy code
- Redis needs to be used, so it needs to be in application Add redis configuration in YML and enable the redis storage function of Session;
spring: redis: host: localhost # Redis server address database: 1 # Redis database index (0 by default) port: 6379 # Redis server connection port password: 123456 # Redis server connection password (blank by default) timeout: 3000ms # Connection timeout erupt: # Enable redis mode to store session s. The default is false. After enabling, add redis configuration in the configuration file redisSession: true Copy code
- Through the service monitoring menu, you can view the CPU, memory and Java virtual machine information of the server;
- Redis information, command statistics and Redis Key statistics can be viewed through the cache monitoring menu;
- Through the online user menu, you can view the online user information and force the user to exit!
NoSQL data source erupt mongodb
Erupt supports a variety of data sources, including MySQL, Oracle, PostgreSQL, H2, and even MongoDB. Let's experience the support functions of MongoDB.
- In POM Add erupt mongodb dependency in XML;
<!--NoSQL data source erupt-mongodb--> <dependency> <groupId>xyz.erupt</groupId> <artifactId>erupt-mongodb</artifactId> <version>${erupt.version}</version> </dependency> Copy code
- Since MongoDB needs to be used, it should be in application Add MongoDB configuration in YML;
spring: data: mongodb: host: localhost # Connection address of mongodb port: 27017 # Connection port number of mongodb database: erupt # mongodb connected database Copy code
- Take a simplified version of commodity management as an example, or a familiar routine, add a PmsProduct entity class;
/** # summary From basic to advanced and then to actual combat, this paper goes from shallow to deep MySQL Speak clearly and plainly, this should be the best I have seen so far MySQL I believe that if you read this note carefully, you will be able to solve both the problems encountered at work and the questions asked by the interviewer! **Important thing: you need to get the full version MySQL Please forward the learning notes+After attention[Click here for free](https://gitee.com/vip204888/java-p7) to free download** **MySQL50 Arrangement of high frequency interview questions:** artifactId> <version>${erupt.version}</version> </dependency> Copy code
- Since MongoDB needs to be used, it should be in application Add MongoDB configuration in YML;
spring: data: mongodb: host: localhost # Connection address of mongodb port: 27017 # Connection port number of mongodb database: erupt # mongodb connected database Copy code
- Take a simplified version of commodity management as an example, or a familiar routine, add a PmsProduct entity class;
/** # summary From basic to advanced and then to actual combat, this paper goes from shallow to deep MySQL Speak clearly and plainly, this should be the best I have seen so far MySQL I believe that if you read this note carefully, you will be able to solve both the problems encountered at work and the questions asked by the interviewer! **Important thing: you need to get the full version MySQL Please forward the learning notes+After attention[Click here for free](https://gitee.com/vip204888/java-p7) to free download** **MySQL50 Arrangement of high frequency interview questions:** 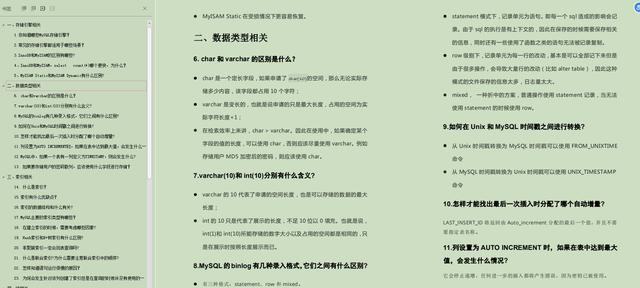