1. Method definition and call
- So what is the method?
A method is essentially a block of code - So what is the significance of the method?
After defining a method, you can call it repeatedly in different positions, so you don't need to write the same piece of code repeatedly.
1. Method definition and calling syntax
//Method definition
public static Enlarge return value method name(Parameter type parameter) {
Method body code;
return Return value;
}
//Method call
Return value variable = Method name(Argument);
- When defining a method, you can have no parameters, but if so, you must specify the parameter type
- When defining a method, the return value can be null. If not, the return value type is written as void
- The parameters when defining a method are called formal parameters, and the parameters when calling a method are called arguments
- The code defining the method can be written anywhere in the class, but it must be written in the class
2. Method calling process
- When defining a method, the method will not be executed, and the method will only be executed when calling this method
- When the method is called, the value of the actual parameter will be assigned to the formal parameter. After the parameter is passed, the method body code will be executed
- When the method encounters a return statement, the execution of the method body will end. After the execution of the called method is completed, return to the location of the method call to continue execution
3. Method of finding the sum of factorials
- So let's write a method to calculate the factorial and call it to calculate the sum of factorials of num
public Test {
public static void main(String[] args) {
int num = 5;
int sum = 0;
for(int i = 1;i <= num;i++) {
sum += fac(i);
}
System.out.println(num+" The sum of the factorials of is "+sum!)
}
public static int fac(int num) {
int result = 1;
for(int i = 0;i < n;i++) {
result *= i;
}
return result;
}
}
//Operation results
5 The sum of the factorials of is 153!
4. Relationship between formal parameter and argument
- Swap two integer variables
public class Test {
public static void main(String[] args) {
int num1 = 10;
int num2 = 20;
swap(num1,num2);
System.out.println("num1 = "+num1+" num2 = "+num2);
}
public static void swap(int num1,int num2) {
int tmp = num1;
num1 = num2;
num2 = tmp;
}
}
//Operation results
num1 = 10 num2 = 20
- As can be seen from the above code, simply calling to exchange the values of two numbers cannot be successfully exchanged; This is because for simple basic types, formal parameters are copies of arguments, that is, value passing calls.
- Then we solve this problem with the parameters of reference type
public class Test{
public static void main(String[] args) {
int num1 = 10;
int num2 = 20;
int[] array = {num1,num2};
swap(array);
System.out.println("num1 = "+array[0]+" num2 = "+array[1]);
}
public static void swap(int[] array) {
int tmp = array[0];
array[0] = array[1];
array[1] = tmp;
}
}
//Operation results
num1 = 20 num2 = 10
2. Overloading of methods
- When two methods have the same method name and different parameter numbers or parameter types (the same or different return values are OK), we call it method overloading.
class Test {
public static void main(String[] args) {
int a = 10;
int b = 20;
int ret = add(a, b);
System.out.println("ret = " + ret);
double a2 = 10.5;
double b2 = 20.5;
double ret2 = add(a2, b2);
System.out.println("ret2 = " + ret2);
double a3 = 10.5;
double b3 = 10.5;
double c3 = 20.5;
double ret3 = add(a3, b3, c3);
System.out.println("ret3 = " + ret3);
}
public static int add(int x, int y) {
return x + y;
}
public static double add(double x, double y) {
return x + y;
}
public static double add(double x, double y, double z) {
return x + y + z;
}
}
- When two methods have the same method name, the same parameters, but different return values, they are not overloaded
3. Method recursion
1. The concept of recursion
- A method invokes the method itself in the execution process, which is called "recursion".
- Recursively find the factorial of n
public static void main(String[] args) {
int n = 5;
int result = fac(n);
System.out.println(n+" The factorial of is:"+result);
}
public static int fac(int n) {
if(n == 1) {
return 1;
}
return n*fac(n-1);
}
//Operation results
5 Factorial of: 120
2. Recursive execution process
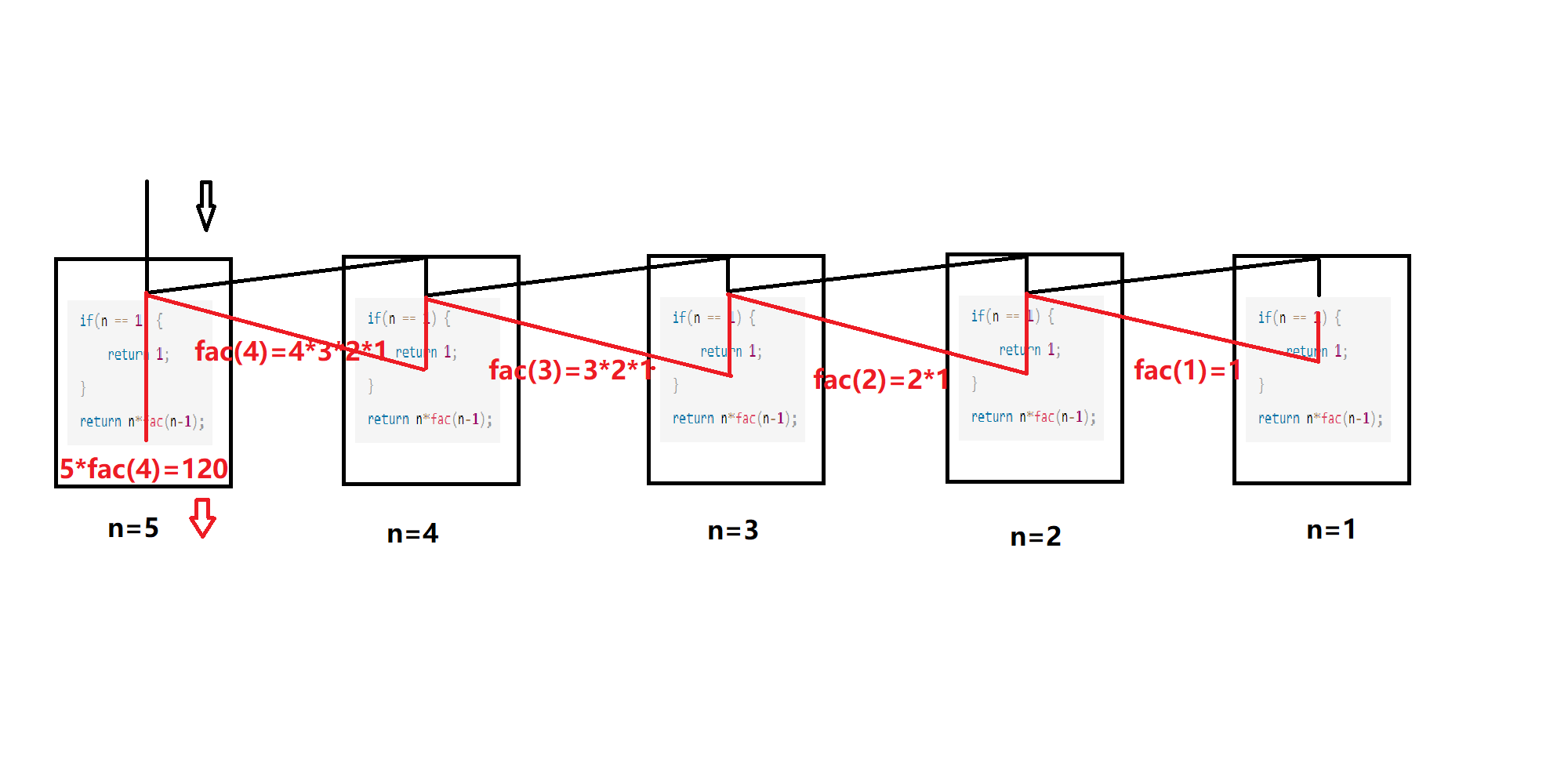
- As for "stack", when each method is called, there will be a "stack"
- Every time a method is called, there will be a "stack frame", which contains the parameters and other information called