1,Cookie
1.1. What is a Cookie?
- Cookie translates to cookie.
- Cookie is a technology that the server notifies the client to save key value pairs.
- After the client has a Cookie, each request is sent to the server.
- The size of each Cookie cannot exceed 4kb
1.2. How to create cookies
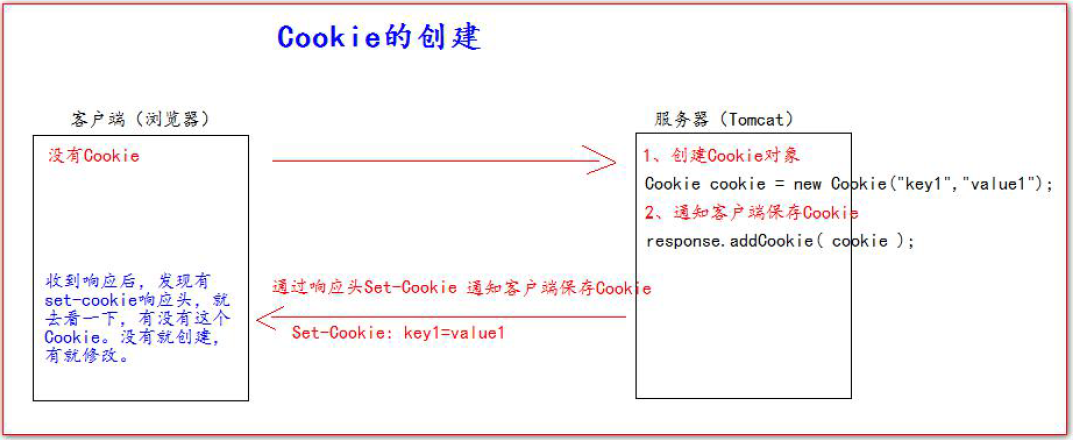
protected void createCookie(HttpServletRequest req, HttpServletResponse resp) throws ServletException,
IOException {
//1 create Cookie object
Cookie cookie = new Cookie("key4", "value4");
//2 notify the client to save the Cookie
resp.addCookie(cookie);
//1 create Cookie object
Cookie cookie1 = new Cookie("key5", "value5");
//2 notify the client to save the Cookie
resp.addCookie(cookie1);
resp.getWriter().write("Cookie Created successfully");
}
1.3 how does the server get cookies
- The server only needs one line of code to get the client's Cookie: req getCookies():Cookie[]
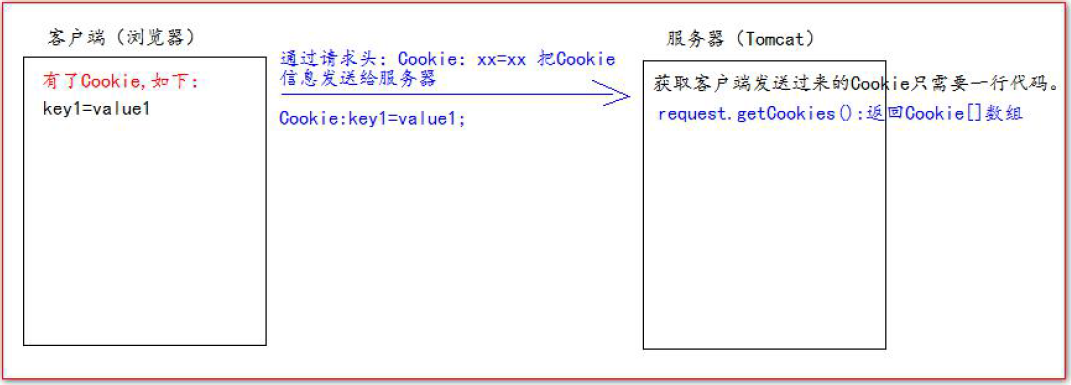
public class CookieUtils {
/**
* Finds a Cookie object with the specified name
* @param name
* @param cookies
* @return
*/
public static Cookie findCookie(String name , Cookie[] cookies){
if (name == null || cookies == null || cookies.length == 0) {
return null;
}
for (Cookie cookie : cookies) {
if (name.equals(cookie.getName())) {
return cookie;
}
}
return null;
}
}
protected void getCookie(HttpServletRequest req, HttpServletResponse resp) throws ServletException,
IOException {
Cookie[] cookies = req.getCookies();
for (Cookie cookie : cookies) {
// The getName method returns the key (name) of the Cookie
// The getValue method returns the value of the Cookie
resp.getWriter().write("Cookie[" + cookie.getName() + "=" + cookie.getValue() + "] <br/>");
}
Cookie iWantCookie = CookieUtils.findCookie("key1", cookies);
// for (Cookie cookie : cookies) {
// if ("key2".equals(cookie.getName())) {
// iWantCookie = cookie;
// break;
// }
// }
// If it is not equal to null, it indicates that a value has been assigned, that is, the required Cookie has been found
if (iWantCookie != null) {
resp.getWriter().write("Found what you need Cookie");
}
}
1.4 modification of Cookie value
- Scheme I:
- First create a Cookie object with the same name (key) to modify
- In the constructor, a new Cookie value is assigned at the same time.
- Call response addCookie( Cookie );
// Scheme I:
// 1. First create a Cookie object with the same name to modify
// 2. In the constructor, a new Cookie value is assigned at the same time.
Cookie cookie = new Cookie("key1","newValue1");
// 3. Call response addCookie( Cookie ); Notify client to save changes
resp.addCookie(cookie);
- Scheme II:
- Find the Cookie object to be modified first
- Call the setValue() method to assign a new Cookie value.
- Call response Addcookie() notifies the client to save the modification
// Scheme II:
// 1. Find the Cookie object to be modified first
Cookie cookie = CookieUtils.findCookie("key2", req.getCookies());
if (cookie != null) {
// 2. Call the setValue() method to assign a new Cookie value.
cookie.setValue("newValue2");
// 3. Call response Addcookie () notifies the client to save the modification
resp.addCookie(cookie);
}
1.5 Cookie life control
- The life control of cookies refers to how to manage when cookies are destroyed (deleted)
- setMaxAge()
- A positive number that expires after the specified number of seconds
- A negative number indicates that the Cookie will be deleted as soon as the browser is closed (the default value is - 1)
- Zero indicates that the Cookie is deleted immediately
/**
* Set Cooie to survive for 1 hour
* @param req
* @param resp
* @throws ServletException
* @throws IOException
*/
protected void life3600(HttpServletRequest req, HttpServletResponse resp) throws ServletException,
IOException {
Cookie cookie = new Cookie("life3600", "life3600");
cookie.setMaxAge(60 * 60); // The Cookie was deleted after one hour. invalid
resp.addCookie(cookie);
resp.getWriter().write("A one hour survival has been created Cookie");
}
/**
* Delete a Cookie now
* @param req
* @param resp
* @throws ServletException
* @throws IOException
*/
protected void deleteNow(HttpServletRequest req, HttpServletResponse resp) throws ServletException,
IOException {
// Find the Cookie object you want to delete first
Cookie cookie = CookieUtils.findCookie("key4", req.getCookies());
if (cookie != null) {
// Call setMaxAge(0);
cookie.setMaxAge(0); // It means to delete it immediately without waiting for the browser to close
// Call response addCookie(cookie);
resp.addCookie(cookie);
resp.getWriter().write("key4 of Cookie Has been deleted");
}
}
/**
* Default session level Cookie
* @param req
* @param resp
* @throws ServletException
* @throws IOException
*/
protected void defaultLife(HttpServletRequest req, HttpServletResponse resp) throws ServletException,
IOException {
Cookie cookie = new Cookie("defalutLife","defaultLife");
cookie.setMaxAge(-1);//Set survival time
resp.addCookie(cookie);
}
1.6 setting of effective Path of Cookie
- The path attribute of cookies can effectively filter which cookies can be sent to the server. Which do not send.
- The path attribute is effectively filtered by the requested address.
- CookieA path = / Project path
- CookieB path = / Project path / abc
- The request address is as follows:
- http://ip:port/ Project path / a.html
- CookieA send
- CookieB does not send
- http://ip:port/ Project path / abc/a.html
protected void testPath(HttpServletRequest req, HttpServletResponse resp) throws ServletException,
IOException {
Cookie cookie = new Cookie("path1", "path1");
// Getcontextpath() = = = > > > get the project path
cookie.setPath( req.getContextPath() + "/abc" ); // ===>>>>/ Project path / abc
resp.addCookie(cookie);
resp.getWriter().write("Created a with Path Path Cookie");
}
2. Session session
2.1. What is a Session
- Session is an interface (HttpSession).
- A Session is a Session. It is a technology used to maintain an association between a client and a server.
- Each client has its own Session.
- In the Session session, we often use it to save the information after the user logs in.
2.2. How to create a Session and obtain (id number, new or not)
- How to create and get a Session. Their API s are the same.
- request.getSession()
- The first call is to create a Session
- After that, you can get the Session session object that you created earlier.
- isNew()
- Judge whether it is newly created (New)
- true indicates that it has just been created
- false indicates created before getting
- getId()
- Get the Session id value of the Session.
- Each session has an ID number. That is, the ID value. And this ID is unique.
2.3. Access of Session domain data
/**
* Save data to Session
* @param req
* @param resp
* @throws ServletException
* @throws IOException
*/
protected void setAttribute(HttpServletRequest req, HttpServletResponse resp) throws ServletException,
IOException {
req.getSession().setAttribute("key1", "value1");
resp.getWriter().write("Already gone Session Data saved in");
}
/**
* Get the data in the Session domain
* @param req
* @param resp
* @throws ServletException
* @throws IOException
*/
protected void getAttribute(HttpServletRequest req, HttpServletResponse resp) throws ServletException,
IOException {
Object attribute = req.getSession().getAttribute("key1");
resp.getWriter().write("from Session Get out of key1 The data are:" + attribute);
}
2.4. Session lifecycle control
- public void setMaxInactiveInterval(int interval)
- Set the timeout time (in seconds) of the Session. If it exceeds the specified time, the Session will be destroyed.
- When the value is positive, set the timeout length of the Session.
- A negative number means never timeout (rarely used)
- public int getMaxInactiveInterval()
- Gets the timeout of the Session
- public void invalidate()
- Make the current Session timeout immediately invalid.
- The default timeout for Session is 30 minutes.
- Because in the configuration file of Tomcat server, web XML has the following configuration by default, which means that all sessions under the current Tomcat server are configured
<session-config> <session-timeout>30</session-timeout></session-config>
- If you want to modify only the timeout duration of individual sessions. You can use Session Setmaxinactivitinterval (int interval) sets the timeout duration separately.
protected void life3(HttpServletRequest req, HttpServletResponse resp) throws ServletException,
IOException {
// Get the Session object first
HttpSession session = req.getSession();
// Set the timeout after 3 seconds for the current session
session.setMaxInactiveInterval(3);
resp.getWriter().write("current Session Has been set to timeout after 3 seconds");
}
protected void deleteNow(HttpServletRequest req, HttpServletResponse resp) throws ServletException,
IOException {
// Get the Session object first
HttpSession session = req.getSession();
// Let the Session timeout immediately
session.invalidate();
resp.getWriter().write("Session Has been set to timeout (invalid)");
}
2.5 technical insider of association between browser and Session
- Session technology, the bottom layer is actually implemented based on Cookie technology.
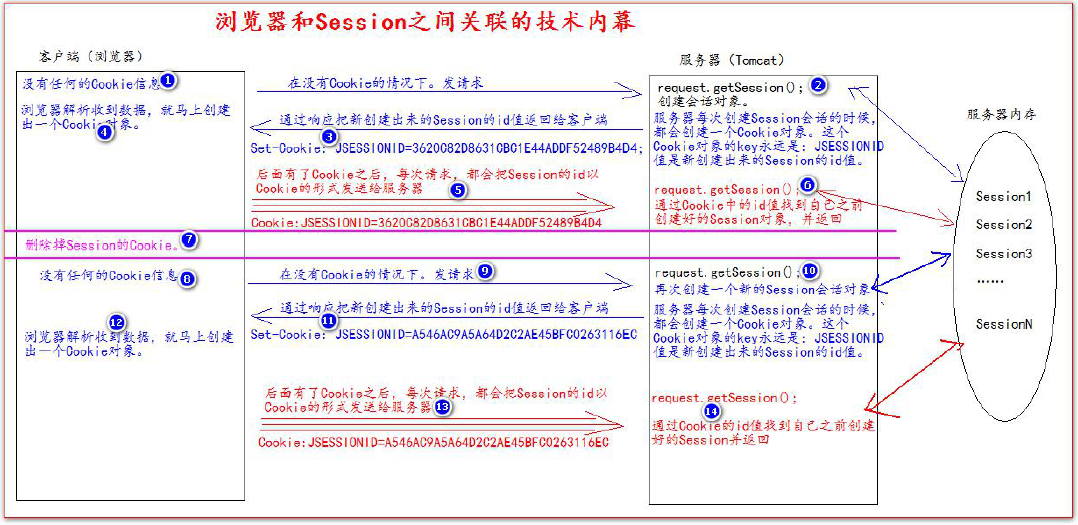