15 supplement
14.1 basic summary
14.1.1 data type
It is divided into two categories:
-
Basic type (value type)
- String: any string
- Number: any number
- boolean: true,false
- undefined: undefined
- Null: null
-
Object type (reference type)
- Object: any object
- Array: special object, accessed by subscript
- Function: a special object that can be executed
- Judge data type:
-
typeof returns a string representation of the data type
- You can judge undefined, numeric value, string, Boolean value and function
- null and object, object and array cannot be judged
-
instanceof determines the specific type of the object: array, function...
- A instanceof B: is a an instance of B
-
===(try not to use = =, because = = can do data conversion)
- You can judge undefined and null (because these two types have only one value)
// Basic type var a; console.log(a, typeof a === undefined) // undefined false console.log(a, typeof a, typeof a === 'undefined', a===undefined) // undefined "undefined" true true ---------------------------------------------------------------------------- a = 3 console.log(typeof a === 'number') // true ----------------------------------------------------------------------------- a = 'asdsd' console.log(typeof a === 'string') // true ----------------------------------------------------------------------------- a = true console.log(typeof a === 'boolean') // true ----------------------------------------------------------------------------- a = null console.log(typeof a, a === null) // 'object' true // Object----------------------------------------------------------------------- var b1 { b2: [1,'abc',console.log], b3: function() { console.log('b3') return function() { return 'xfdfds' } } } console.log(b1 instanceof Object, b1 instanceof Array) // true false // Object is a constructor (because new Object()) ------------------------------------------------------------------------------ console.log(typeof b1.b2) // 'object' console.log(b1.b2 instanceof Array, b1.b2 instanceof Object) // true true // Array array is also an object ------------------------------------------------------------------------------ console.log(b1.b3 instanceof Function, b1.b3 instanceof Object) // true true // Function s are also objects console.log(typeof b1.b3, typeof b1.b3 === 'function') // 'function' true console.log(typeof b1.b2[2] === 'function') // true ------------------------------------------------------------------------------ b1.b2[2](4) // This is a function // How to call the output xfds console.log(b1.b3()())
- Difference between undefined and null
- undefined (declared unassigned)
var a console.log(a) //The declaration is not assigned undefined
- Null (defined and assigned, the value is null)
// start var b = null //The initial assignment is null, indicating that it will be assigned as an object // Assign a value when the object is determined b = ['aedxf', 12] // last b = null // Release the object and make the object pointed to by b become a garbage object (recycled by the garbage collector)
- When is a variable assigned null
- Initial assignment, indicating that the assignment is to be an object
- Before completion, make the object a garbage object (collected by the garbage collector)
- Strictly distinguish between variable type and data type
- Type of data
- Basic type
- object type
- Type of variable
- Basic type: data of basic type is saved
- Reference type: saves the address value
14.1.2 data & Variables & Memory
- Memory
-
A small piece of memory stores two things: internally stored data and address values
Simple data types also have addresses, but they don't
-
Memory classification
- Stack: global variable, local variable
- Heaps: objects
The function is in heap space, but the function name (the variable identifying the function) is in stack space
-
Variable (variable name + variable value)
Each variable corresponds to a small memory. The variable name is used to find the corresponding memory, and the variable value is the data stored in the memory
-
Memory, relation, data
Memory the space used to store data
Variable is the identification of memory
-
Problems about assignment and memory
var a = xxx, what is stored in memory of a?
- xxx is the basic data, which is the data saved
- xxx is the object, which saves the address value of the object
- xxx is a variable that stores the memory contents of xxx (which may be basic data or address)
- On the assignment of reference variables
var obj1 = {name: 'Tom'} var obj2 = obj1 obj1.name = 'Jack' console.log(obj2.name) // 'Jack' function fn(obj){ obj.name = 'A' } fn(obj1) //At this time, three variables point to the same object (formal parameter obj of obj1, obj2 and fn) function fn2(obj) { obj = {age:15} //This is a garbage object. After the function is executed, the local variables will be recycled } fn2(a) console.log(a.age) // 15 // The formal parameter obj passed from a to fn2 is the address value of A. at this time, obj stores the address value of A // However, in the function, obj is copied to an address value again, and a does not change
- When js calls a function, whether to pass variable parameters by value or by reference
- Understanding 1: all values (basic value / address value) are passed
- Understanding 2: it may be value passing or reference passing (address value)
var a = 3 function fn(a) { //The formal parameter a here is equivalent to var a in fn, which is independent of the global a a = a+1 } fn(a) //The value passed in here is 3 console.log(a) // 3. The global a variable is printed here
- How does JS engine manage memory
- Memory life cycle
- Allocate small memory space and get the right to use it
- Store data and operate repeatedly
- Free up small memory space
- Free memory
- Local variable: automatically released after function execution
- Object: becomes a garbage object and is subsequently recycled by the garbage collector
- Global variables: not released
var a = 3 var obj = {} //At this time, there are two objects, and the memory space is not released (because it is a global variable) object = null // At this time, two objects, obj, are not released function fn() { var b = {} var c = 4 // The variable is initialized only when the function is called } fn() //After the function is executed, b is automatically released, and the object pointed to by b is collected by the garbage collector at a later time
14.1.3 object
- What is an object
Encapsulation of multiple data
- Why use objects
Unified management of multiple data
- Composition of objects
- Attribute: it is composed of attribute name (string) and attribute value (any type)
- Method: a special attribute (the attribute value is a function)
- Access properties
p.name or p['name']
- When must we use the method of [attribute name]
- The attribute name contains special characters: - space p ['content type'] = 'text/json'
- Uncertain attribute name: the attribute name is a variable
var propName = 'myAge' var age = 18 p[propName] = value
14.1.4 function
- What is a function
- Encapsulation of n statements to achieve specific functions
- Only functions can be executed, and other types of data cannot be executed
- How to call a function
- test(): call directly
- obj.test(): called by object
- new test(): Call new
- test.call/apply(obj): temporarily call the method that makes test obj
var obj = {} function test2() { this.xxx = 'atguigu' } // Assume obj test2() test2.call(obj) // You can call a function as a method that specifies any object console.log(obj.xxx)// atguigu
14.1.5 callback function
- What function is a callback function?
- What you defined, did not (actively) call, but finally executed
- Common callback functions
- dom event callback function: for example, trigger the calling function after clicking
- Timer callback function
- ajax request callback function
- Lifecycle callback function
14.1.6 IIFE (execute function immediately)
Full name: immediately invoked function expression
effect:
- Hidden implementation
- Does not contaminate external (global) namespaces
- Used to encode js module
(function () { var a = 1; function test(){ console.log(++a); } window.$ = function () { //Expose a global function return { test: test } } }) $().test() //1. $is a function 2 After execution, an object is returned
14.2 function object
14.2.1 prototype & prototype chain (implicit prototype chain)
- Combining Shang Silicon Valley and dark horse, mainly remember the inside of the red box. See (4.7 and 7.1) for details. Since all functions are instances of Function, Foo constructor, Object constructor and Function constructor all have one_ proto _ Property points to the Function prototype Object; Because the prototype Object is an empty Object by default, the Function prototype Object is empty_ proto _ Property points to an Object prototype Object
-
How does instanceof judge?
A instanceof B: returns true if the prototype object of B function is on the prototype chain of a object; otherwise, returns false
-
Interview questions:
// Test 1 function A() {} A.prototype.n = 1; var b = new A(); A.prototype = { n: 2, m: 3 } var c = new A(); console.log(b.n, b.m, c.n, c.m); // 1 undefined 2 3 -------------------------------------------------------------------- // Test 2 var F = function(){} Object.prototype.a = function(){ console.log('a()') } Function.prototype.b = function(){ console.log('b()') } var f = new F(); f.a() // a() f.b() // f.b is not a function F.a() // a() F.b() // b()
14.2.2 execution context and execution context stack
- Global execution context
-
Determine the window as the global execution context before executing the global code
-
Preprocess global data
- The global variable defined by var = = > undefined is added as the attribute of window
- The global function declared by function = = > is assigned (fun) and added as the method of window
- This = = = > assignment (window)
-
Start executing global code
- Function execution context
- Before calling the function and preparing to execute the function body, create the corresponding function execution context object
- Preprocess local data
- Formal parameter variable = = > assignment (argument) = = > attribute added as execution context
- Arguments = = = > assignment (argument list), added as the attribute of the execution context
- The local variable defined by var = = > undefined is added as the attribute of the execution context
- The function declared by function = = > (fun) is added as the method of execution context
- This = = = > assignment (object calling the function)
- Start executing function body code
- Execution Context Stack
- Before executing the global code, the JS engine will create a stack to store and manage all execution context objects
- After the global execution context (window) is determined, it is added to the stack (stack pressing)
- After the function execution context is created, it is added to the stack (stack pressing)
- After the current function is executed, the object at the top of the stack is removed (out of the stack)
- When all the code is executed, only window is left in the stack
- Interview questions
// 1. What to output in turn 2 Several execution contexts are generated in the whole process console.log('global begin:' + i) var i = 1 foo(1) function foo(i) { if(i == 4) { return; } console.log('foo() begin:' + i); foo(i + 1); console.log('foo() end:' + i); } console.log('global() end:' + i); 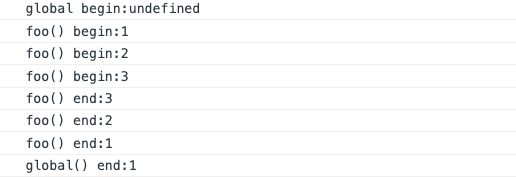 // Five execution contexts were generated
When the function declaration is the same as the variable name, the function declaration is still a function declaration and will not be overwritten before the variable assignment; When a variable is assigned, the function declaration is overwritten by the same variable.
// Test question 1: execute variable promotion first, and then function promotion (in doubt) function a() {} var a; console.log(typeof a) // 'function' ------------------------------------------------------------ // Test question 2 if(!(b in window)) { var b = 1; } console.log(b); // undefined ------------------------------------------------------------ // Test question 3 var c = 1; function c(c) { console.log(c); } c(2); // c is not a function
14.2.3 scope and scope chain
- Difference 1
- Outside the global scope, each function will create its own scope, which has been determined when the function is defined. Not when a function is called
- The global execution context is created immediately after the global scope is determined and before js code is executed
- The function execution context is created before the function body code is executed when the function is called
- Difference 2
- The scope is static. As long as the function is defined, it will always exist and will not change
- The execution context is dynamic. It is created when the function is called and will be automatically released when the function is called
- contact
- The context (object) is subordinate to the scope
- Global context = = > global scope
- Function context = = > corresponding function usage domain
- Interview questions
// Test question 1: how much output var x = 10; function fn() { console.log(x); } function show(f) { var x = 20; f(); } show(fn); // 10 // The scope is determined when the function is defined, so there are three scopes: global, fn(), show() // When fn() is called, first find X in its own scope, and then find it in the global scope, so x = 10 ---------------------------------------------------------------- // Test question 2: output var fn = function () { console.log(fn) } fn() var obj = { fn2: function () { console.log(fn2) } } obj.fn2() // result ƒ () { console.log(fn) } ReferenceError: fn2 is not defined // When fobj When calling fn2(), first look for FN2 in its own scope, and then look for it in the global scope, so an error is reported // To find FN2: this fn2
14.2.4 closure
Combination 11
// Requirement: click a button and prompt "click the nth button" for(var i=0;i<btns.length;i++) { // BTNs is calculated every time length // For (VaR I = 0, length = btns.length; I < length; I + +) will calculate BTNs only once length var btn = btns[i] btn.onclick = function () { alert('The first'+(i+1)+'individual') } } console.log(i); // 4 [i is a global variable] ------------------------------------------------------------ function fn1() { //closure var a=2; function fn2() { console.log(a); } } fn1()
- How to generate closures?
- Closure occurs when a nested inner (child) function references a variable (function) of a nested outer (parent) function
- What is a closure?
- Debugging and viewing with chrome
- Understanding 1: closures are nested internal functions (most people)
- Understanding 2: objects (very few people) containing referenced variables (functions) are recommended
- Note: closures exist in nested inner functions
- Conditions for generating closures?
- Function nesting
- The internal function refers to the data of the external function (variable / function)
- Common closures
- Take a function as the return value of another function
- Pass a function as an argument to another function call
1. Process oriented and object-oriented
1.1 comparison between process oriented and object-oriented
- Process oriented is to analyze the steps needed to solve the problem, and then use functions to realize these steps step by step. When using, you can call them one by one in turn.
- Object oriented is to decompose transactions into objects, and then divide and cooperate among objects.
Process oriented | object-oriented | |
---|---|---|
advantage | Higher performance than object-oriented, suitable for things closely related to hardware, such as process oriented programming adopted by single chip microcomputer. | Easy to maintain, reuse and expand. Due to the characteristics of encapsulation, inheritance and polymorphism of object-oriented, a low coupling system can be designed to make the system more flexible and easier to maintain |
shortcoming | Difficult to maintain, reuse and expand | Lower performance than process oriented |
2. Objects and classes
2.1 object
Object is composed of attributes and methods: it is a collection of unordered key value pairs, which refers to a specific thing
- Attribute: the characteristic of a thing, which is represented by an attribute in an object (a common noun)
- Method: the behavior of things is expressed by means in the object (commonly used verb)
2.1.1 creating objects
//The following code is a review of the object / / create the object literally var LDH = {Name: 'Andy Lau', age: 18} console log(ldh);// Constructor creates object function star (name, age) {this. Name = name; this. Age = age;} Var LDH = new star ('andy Lau ', 18) / / instantiate the object console log(ldh);
The running result of the above two lines of code is:
Type 2.2
- The concept of class is newly added in ES6. You can use the class keyword to declare a class, and then instantiate the object with this class. Class abstracts the common part of an object. It generally refers to a large class object, especially a class, and instantiates a specific object through a class
2.2.1 create class
- Syntax:
//Step 1 use the class keyword class name { // class body } //Step 2 create an instance using the defined class. Pay attention to the new keyword var xx = new name();
- Examples
// 1. Create class create a star class class Star { // The common properties of the class are placed in the constructor constructor(name, age) { this.name = name; this.age = age; } } // 2. Create new object with class var ldh = new Star('Lau Andy', 18); console.log(ldh);
Operation results of the above code:
From the results, we can see that the running result is the same as using the constructor
2.2.2 create and add attributes and methods of class
// 1. Create a class class // 1. Create a class class class Star { // The common attributes of the class are placed in the constructor, which is the constructor or constructor constructor(uname, age) { this.uname = uname; this.age = age; }//------------------------------------------->Note that there is no need to add commas between methods sing(song) { console.log(this.uname + 'sing' + song); } } // 2. Create new object with class var ldh = new Star('Lau Andy', 18); console.log(ldh); // Star {uname: "Andy Lau", age: 18} ldh.sing('Ice rain'); // Andy Lau sings ice rain
Operation results of the above code:
Attention:
- Create a class through the class keyword. We still habitually define the initial capitalization of the class name
- There is a constructor function in the class, which can accept the passed parameters and return the instance object at the same time
- constructor function will automatically call this function as long as new generates an instance. If we don't write this function, the class will automatically generate this function
- There is no need to add comma separation between multiple function methods
- Generate instance new cannot be omitted
- Syntax specification: do not add parentheses after the class name of the created class, parentheses after the class name of the generated instance, and function is not required for the constructor
2.2.3 inheritance of class
- grammar
// Parent class class Father{ } // The subclass inherits the parent class class Son extends Father { }
- Examples
class Father { constructor(surname) { this.surname= surname; } say() { console.log('What's your last name' + this.surname); } } class Son extends Father{ // In this way, the subclass inherits the properties and methods of the parent class } var damao= new Son('Liu'); damao.say(); //The result is that your last name is Liu
Operation results of the above code:
- Subclasses use the super keyword to access the methods of the parent class
//Parent class defined class Father { constructor(x, y) { this.x = x; this.y = y; } sum() { console.log(this.x + this.y); } } //The child element inherits the parent class class Son extends Father { constructor(x, y) { super(x, y); //The constructor in the parent class was called with super } } var son = new Son(1, 2); son.sum(); //The result is 3
be careful:
-
In inheritance, if you instantiate a subclass to output a method, first see whether the subclass has this method. If so, execute the subclass first
-
In inheritance, if there is no method in the subclass, check whether the parent class has this method. If so, execute the method of the parent class (proximity principle)
-
If the subclass wants to inherit the method of the parent class while extending its own method internally, calling the constructor of the parent class by super, super must be called before the subclass this.
// The parent class has an addition method class Father { constructor(x, y) { this.x = x; this.y = y; } sum() { console.log(this.x + this.y); } } // The subclass inherits the addition method of the parent class and extends the subtraction method at the same time class Son extends Father { constructor(x, y) { // Using super to call the parent class, the constructor super must be called before subclass this, and will report error after this. super(x, y); this.x = x; this.y = y; } subtract() { console.log(this.x - this.y); } } var son = new Son(5, 3); son.subtract(); //2 son.sum();//8
The running result of the above code is:
-
Always pay attention to the direction of this. The common attributes and methods in the class must be used with this
- this in the constructor refers to the instance object from new
- Custom methods generally also point to the instance object from new
- After binding the event, this points to the event source that triggered the event
-
In ES6, there is no variable promotion for a class, so you must define a class before you can instantiate an object through the class
3. tab bar switching of object-oriented version
3.1 functional requirements
- Click the tab bar to switch the effect
- Click the + sign to add tab items and content items
- Click the x sign to delete the current tab item and content item
- Double click the text of tab item or content item to modify the text content
3.2 case preparation
- Get Title element
- Get content element
- Get the x number of the deleted small button
- Create a new js file, define classes, and add required attributes and methods (switch, delete, add, modify)
- Always pay attention to the direction of this
3.3 switching
-
Bind the click event to the obtained title, display the corresponding content area, and store the corresponding index
this.lis[i].index = i; this.lis[i].onclick = this.toggleTab;
-
Use exclusivity to realize the display of only one element
toggleTab() { //Remove all title and content class styles for (VaR I = 0; I < this. LIS. Length; I + +) {this. LIS [i]. Classname = ''; this. Sections [i]. Classname = '';}// Add the active style this. For the current title className = 'liactive'; // Add the active style that. For the current content sections[this.index]. className = 'conactive'; }
3.4 adding
-
Add button + bind click event for
this.add.onclick = this.addTab;
-
Realize the addition of title and content, and do a good job in exclusive treatment
addTab() { that.clearClass(); // (1) Create Li element and section element var random = math random(); Var Li = '< Li class = "liactive" > < span > new tab < / span > < span class = "iconfont icon guanbi" > </ span></li>'; Var section = '< section class = "active" > test' + random + '< / section >'// (2) Append these two elements to the corresponding parent element that ul. insertAdjacentHTML('beforeend', li); that. fsection. insertAdjacentHTML('beforeend', section); that. init(); }
3.5 deletion
-
Bind click event for delete button x of element
this.remove[i].onclick = this.removeTab;
-
Get all of the parent elements of the clicked delete button, and delete the corresponding title and content
removeTab(e) { e.stopPropagation(); // Prevent bubbling and trigger the switching click event of li var index = this parentNode. index; console. log(index); // Delete the corresponding li and section remove() methods according to the index number to directly delete the specified element that lis[index]. remove(); that. sections[index]. remove(); that. init(); // When we delete a li that is not in the selected state, the original selected li remains unchanged. if (document.querySelector('.liactive')) return// When we delete the li in the selected state, we make the previous li in the selected state// Manually invoking our click event does not require the mouse to trigger that lis[index] && that. lis[index]. click(); }
3.6 editing
-
Bind double-click events for elements (title and content)
this.spans[i].ondblclick = this.editTab; this.sections[i].ondblclick = this.editTab;
-
In the selected state of double-click event processing text, modify the internal DOM node to realize the transmission of old and new value values
editTab() { var str = this.innerHTML; // Double click the suppress selected text window getSelection ? window. getSelection(). removeAllRanges() : document. selection. empty(); // alert(11); this. innerHTML = '<input type="text" />'; var input = this. children[0]; input. value = str; input. select(); // The text in the text box is selected. / / when we leave the text box, we give the value in the text box to span input onblur = function() { this.parentNode.innerHTML = this.value; }; // Press enter to send the value in the text box to span input Onkeyup = function (E) {if (e.keycode = = = 13) {/ / manually call the event that the form loses focus. You don't need to leave the mouse to operate this.blur();}}}
Dynamically create elements
- The first method: createElement, innerHTML assignment, appendChild append to the parent element
- The second method (Advanced): insertAdjacentHTML() can directly add string format elements to the parent element
-------Method 1---------var li = document.createElement('li');li.innerHTML = '<span>new tab </span> <span class="iconfont icon-guanbi"></span>';that.ul.appendChild(li);-------Method 2---------var li = '<li class="liactive"><span>new tab </span> <span class="iconfont icon-guanbi"></span></li>';that.ul.insertAdjacentHTML('beforeend', li);
4. Constructors and prototypes
4.1 three ways to create objects - Review
-
Literal mode
var obj = {};
-
new keyword
var obj = new Object();
-
Constructor mode
function Person(name,age){ this.name = name; this.age = age;}var obj = new Person('zs',12);
Four things new does:
- Create a new empty object in memory.
- Let this point to the new object.
- Execute the code in the constructor and add properties and methods to the new object.
- Return the new object (return is not required in the constructor).
4.2 static members and instance members
4.2.1 instance members
Instance members are members added through this inside the constructor. For example, in the following code, uname age sing is an instance member, which can only be accessed through the instantiated object
function Star(uname, age) { this.uname = uname; this.age = age; this.sing = function() { console.log('I can sing'); }}var ldh = new Star('Lau Andy', 18);console.log(ldh.uname);//Instance members can only be accessed through instantiated objects
4.2.2 static members
Static members are members added to the constructor itself. For example, in the following code, sex is a static member. Static members can only be accessed through the constructor
function Star(uname, age) { this.uname = uname; this.age = age; this.sing = function() { console.log('I can sing'); }}Star.sex = 'male';var ldh = new Star('Lau Andy', 18);console.log(Star.sex);//Static members can only be accessed through constructors
4.3 constructor problems
Constructor method is easy to use, but there is a problem of wasting memory.
Each time an object is created, a space is opened up for the functions in it
4.4 constructor prototype
The function allocated by the constructor through the prototype is shared by all objects.
JavaScript stipulates that each constructor has a prototype attribute that points to another Object (prototype Object), and the default value is an empty Object object. Note that this prototype is an Object. All properties and methods of this Object will be owned by the constructor.
We can define those invariant methods directly on the prototype object, so that all instances of the object can share these methods.
Role of prototype: resource sharing
console.log(typeof Date.prototype); // 'object' function Star(uname, age) { this.uname = uname; this.age = age; } Star.prototype.sing = function() { console.log('I can sing'); } var ldh = new Star('Lau Andy', 18); var zxy = new Star('Xue You Zhang', 19); ldh.sing();//I can sing zxy.sing();//I can sing
4.5 object prototype
Objects have a property__ proto__ (implicit prototype) the prototype object (explicit prototype) pointing to the constructor. The reason why our object can use the properties and methods of the constructor prototype object is that the object has__ proto__ The existence of prototypes.
Before ES6, programmers can directly operate explicit prototypes, not implicit prototypes
__proto__Object prototype and prototype object prototype Is equivalent console.log(ldh._prop_ === Star.prototype); ------ true __proto__The significance of object prototype is to provide a direction or a route for the object search mechanism, but it is a non-standard attribute. Therefore, this attribute can not be used in actual development. It only points to the prototype object internally prototype
**Search rules for methods: * * first, check whether there is a method on the object. If so, execute the method on the object. If not, because there is__ proto__ For the existence of, go to the constructor prototype object prototype to find the method.
4.6 constructor
Object prototype( __proto__)And constructors( prototype)There is a property in the prototype object constructor Properties, constructor We call it a constructor because it refers back to the constructor itself. constructor It is mainly used to record which constructor the object refers to. It can make the prototype object point to the original constructor again. In general, the method of the object is set in the prototype object of the constructor. If there are methods of multiple objects, we can assign values to the prototype object in the form of objects, but this will overwrite the original content of the constructor prototype object, so that the modified prototype object constructor It no longer points to the current constructor. At this point, we can add one to the modified prototype object constructor Point to the original constructor.
If we modify the original prototype object and assign an object to the prototype object, we must manually use the constructor to refer back to the original constructor, such as:
function Star(uname, age) { this.uname = uname; this.age = age; } // In many cases, we need to manually use the constructor attribute to refer back to the original constructor //Star.prototype.sing = function() { // console.log('I can sing '); //}; //Star.prototype.movie = function() { // console.log('I can play movies'); //}; //The following writing method causes the constructor property of the prototype object to be overwritten by the object without referring back to the constructor Star Star.prototype = { // If we modify the original prototype object and assign an object to the prototype object, we must manually use the constructor to refer back to the original constructor // Manually set the pointer back to the original constructor constructor: Star, sing:function() { console.log('I can sing'); }, movie:function() { console.log('I can act in movies'); } } var zxy = new Star('Xue You Zhang', 19); console.log(zxy);
As a result of the above code operation, set the constructor attribute as shown in the figure:
If the constructor property is not set, as shown in the figure:
Summary:
- Prototype (explicit prototype): each constructor has a prototype attribute, which points to the prototype Object of the constructor. The default value is an empty Object object.
- __ proto__ (implicit prototype): each instance object has one__ proto __ Property, pointing to the prototype object of the constructor. The default value is the prototype property value of the constructor.
- Constructor: instance object prototype__ proto__ Both the constructor and prototype prototype objects have an attribute constructor attribute, which points to the constructor.
- Relationship:
1. The prototype attribute of the constructor points to the constructor prototype object.
2. The instance object is created by the constructor__ proto__ Property points to the prototype object of the constructor.
3. The constructor attribute of the prototype object of the constructor points to the constructor, and the constructor attribute of the prototype of the instance object also points to the constructor.
4.7 prototype chain
Each instance object has another__ proto__ Property that points to the prototype object of the constructor. The prototype object of the constructor is also an object. There are also__ proto__ Attribute, so looking up layer by layer forms the prototype chain. (the search method is based on the implicit prototype chain, that is, proto, rather than the explicit prototype chain)
4.8 triangular relationship between constructor instance and prototype object
1.Constructor prototype Property points to the constructor prototype object 2.Instance objects are created by constructors,Instance object__proto__Property points to the prototype object 3 of the constructor.Constructor prototype object constructor Property points to the constructor,Prototype of instance object constructor Property also points to the constructor
4.9 prototype chain and member search mechanism
Any object has a prototype object, that is, the prototype attribute. Any prototype object is also an object, and the object has__ proto__ Attributes, looking up layer by layer, form a chain, which we call prototype chain;
- When accessing the properties (including methods) of an object, first find out whether the object itself has the property.
- If not, find its prototype (that is, the prototype object pointed to by proto).
- If not, find the prototype of the prototype Object (the prototype Object of the Object).
- This class pushes until the Object is found (null).
4.10 this point in prototype object
Both this in the constructor and this in the prototype object point to the instance object we created from new
function Star(uname, age) { this.uname = uname; this.age = age;}var that;Star.prototype.sing = function() { console.log('I can sing'); that = this;}var ldh = new Star('Lau Andy', 18);// 1. In the constructor, this refers to the object instance ldhconsole log(that === ldh);// true// 2. This in the prototype object function refers to the instance object LDH
[the external link image transfer fails. The source station may have an anti-theft chain mechanism. It is recommended to save the image and upload it directly (img-bdvezhrs-164597313178) (/ users / yinyuting / learning / front-end learning / stage III: javascript web page programming materials / 05 JavaScript advanced materials / 04 JavaScript advanced materials / JavaScript advanced _day02 (subsection 3-6) / 4-notes / images/img6.png)]
4.11 extend built-in methods for arrays through prototypes
You can extend and customize the original built-in object through the prototype object.
Note: array and string built-in objects cannot override the operation array Prototype = {}, can only be array prototype. XXX = function() {}.
Array.prototype.sum = function() { var sum = 0; for (var i = 0; i < this.length; i++) { sum += this[i]; } return sum; }; var arr = [1,2,3];arr.sum();var arr1 = new Array(11,22,33);arr1.sum();//At this point, the sum () method already exists in the array object. You can always use the array sum() to calculate the data
5. Succession
es6 did not provide extensions inheritance before. Inheritance can be realized through constructor + prototype object simulation, which is called composite inheritance.
5.1 call()
Call this function and modify the this point when the function runs
fun.call(thisArg, arg1, arg2, ...)// thisArg: currently calls the object / / arg1 of the function this, other parameters passed by arg2.
- call() can call a function
- call() can modify the direction of this. When using call(), parameter 1 is the modified direction of this, parameter 2, parameter 3... Use commas to separate the connection
function fn(x, y) { console.log(this); console.log(x + y); } var o = { name: 'andy' }; fn.call(o, 1, 2);//When the function is called, this points to the object o,
[the external link image transfer fails. The source station may have an anti-theft chain mechanism. It is recommended to save the image and upload it directly (img-kxk2bfbg-164597313178) (/ users / yinyuting / learning / front-end learning / stage III: javascript web page programming materials / 05-JavaScript advanced materials / 04-JavaScript advanced materials / JavaScript advanced _day02 (sections 3-6) / 4-notes / images/img10.png)]
5.2 borrowing the constructor to inherit the attributes in the parent constructor
Core principle: point this of the parent type to this of the child type through call(), so that the child type can inherit the attributes of the parent type.
- Define a parent constructor first
- Define a sub constructor
- The child constructor inherits the properties of the parent constructor (using the call method)
// 1. Parent constructor function Father(uname, age) { // this points to the object instance of the parent constructor this.uname = uname; this.age = age; } // 2 . Sub constructor function Son(uname, age, score) { // this points to the object instance of the sub constructor 3 Use the call method to realize that the child inherits the attribute of the parent Father.call(this, uname, age); this.score = score; } var son = new Son('Lau Andy', 18, 100); console.log(son);
5.3 borrowing prototype object inheritance method
- Define a parent constructor first
- Define a sub constructor
- The child constructor inherits the properties of the parent constructor (using the call method)
// 1. Parent constructor function Father(uname, age) { // this points to the object instance of the parent constructor this.uname = uname; this.age = age; } Father.prototype.money = function() { console.log(100000); }; // 2 . Sub constructor function Son(uname, age, score) { // this points to the object instance of the child constructor Father.call(this, uname, age); this.score = score; } // Son.prototype = Father.prototype; If the child prototype object is modified, the parent prototype object will change with it Son.prototype = new Father(); // If you modify the prototype object in the form of an object, don't forget to use the constructor to refer back to the original constructor Son.prototype.constructor = Son; // This is a special method of the sub constructor Son.prototype.exam = function() { console.log('Children have exams'); } var son = new Son('Lau Andy', 18, 100); console.log(son);
The result of the above code is shown in the figure:
6.ES5 new method
Array.isArray(arr);
console.log(typeof arr);
6.1 array method forEach traverses the array
arr.forEach(function(value, index, array) { //Parameter 1: array element. / / parameter 2: index of array element. / / parameter 3: current array}) / / it is equivalent to that the for loop traversed by the array has no return value
6.2 array method filter array
- Returns a new array directly
var arr = [12, 66, 4, 88, 3, 7]; var newArr = arr.filter(function(value, index,array) { //Parameter 1: array element. / / parameter 2: index of array element. / / parameter 3: current array return value > = 20;}); console. log(newArr);// [66,88] / / the return value is a new array
6.3 array method some
some Find whether there are elements in the array that meet the conditions var arr = [10, 30, 4]; var flag = arr.some(function(value,index,array) { //Parameter 1: array element / / parameter 2: index of array element / / parameter 3: current array return value < 3;}); console. log(flag);// False the return value is a Boolean value. As long as an element satisfying the condition is found, the loop will be terminated immediately
The difference between filter and some: filter returns an array and returns all elements that meet the conditions; Some returns a Boolean value and terminates the search when the first element that meets the condition is found
6.4 array method map
Pass all members of the array into the parameter function in turn, and then form a new array of execution results each time to return.
The original array will not be changed
var numbers = [1, 2, 3];var res = numbers.map(function (n) { return n + 1;});
6.5 array method every
Execute the given function for each item of the array. If each item of the function returns true, the final result will be true; As long as the return value of one item is false, the final result is false. And none of the following elements will continue to execute the function;
The original array is not affected;
var arr = [20,13,11,8,0,11];var arr1 = arr.every(function(value){ return value>10;})
6.6 screening commodity cases
-
Define array object data
var data = [{ id: 1, pname: 'millet', price: 3999 }, { id: 2, pname: 'oppo', price: 999 }, { id: 3, pname: 'glory', price: 1299 }, { id: 4, pname: 'Huawei', price: 1999 }, ];
-
Use forEach to traverse the data and render it to the page
data.forEach(function(value) { var tr = document.createElement('tr'); tr.innerHTML = '<td>' + value.id + '</td><td>' + value.pname + '</td><td>' + value.price + '</td>'; tbody.appendChild(tr); });
-
Filter data by price
-
Get the search button and bind the click event for it
search_price.addEventListener('click', function() {});
-
Use filter to filter out the price information entered by the user
search_price.addEventListener('click', function() { var newDate = data.filter(function(value) { //start.value is the start interval / / end Value is the end interval return value. price >= start.value && value. price <= end.value; }); console. log(newDate); });
-
Re render the filtered data to the table
-
Encapsulate the logic of rendering data into a function
function setDate(mydata) { // First clear the data in the original tbody innerHTML = ''; mydata. forEach(function(value) { var tr = document.createElement('tr'); tr.innerHTML = '<td>' + value.id + '</td><td>' + value.pname + '</td><td>' + value.price + '</td>'; tbody.appendChild(tr); }); }
-
Re render filtered data
search_price.addEventListener('click', function() { var newDate = data.filter(function(value) { return value.price >= start.value && value.price <= end.value; }); console.log(newDate); // Render the filtered object to the page setDate(newDate);});
-
-
Filter by product name
-
Get the product name entered by the user
-
Bind the click event for the query button, and filter the entered product name and this data
search_pro.addEventListener('click', function() { var arr = []; data.some(function(value) { if (value.pname === product.value) { // console.log(value); arr.push(value); return true; // return must be followed by true}})// Render the obtained data to the page setDate(arr);})
-
-
6.7 differences between some and forEach and filter
- If the only element in the array is queried, some method is more appropriate. If return true is encountered in some, the traversal is terminated, and the iteration efficiency is higher
- In forEach, return will not terminate the iteration
- In the filter, return will not terminate the iteration
6.8 trim method removes spaces at both ends of the string
var str = ' hello ';console.log(str.trim()) //hello remove the spaces at both ends var STR1 = 'he l o'; console. Log (str.trim()) / / he l o remove spaces at both ends
6.9 get the attribute name of the object
Object. Keys (object) gets the property name in the current object, and the return value is an array
var obj = { id: 1, pname: 'millet', price: 1999, num: 2000};var result = Object.keys(obj);console.log(result)//[id,pname,price,num]
6.10 Object.defineProperty
Object.defineProperty() sets or modifies properties in an object
Object.defineProperty(obj,prop,descriptor)// obj: required, target object / / prop: required, the name of the attribute to be defined or modified / / descriptor: required, the attribute of the target attribute / / descriptor is written in the form of an object {}. The following attributes Value: the value of the modified or added attribute, The default is undefined writable:true/false, / / if the value is false, the property value cannot be modified enumerable: false,//enumerable if the value is false, traversal is not allowed. Configurable: false / / configurable if false, this attribute is not allowed to be deleted. Can the attribute be deleted or can the attribute be modified again
7. Function definition and call
7.1 definition of function
-
Mode 1 function declaration mode function keyword (named function)
function fn(){}
-
Mode 2 function expression (anonymous function)
var fn = function(){}
-
Mode 3 new Function()
-
All parameters in Function must be in string format
-
The third method is inefficient and inconvenient to write, so it is less used
-
All functions are instances (objects) of functions
-
Functions also belong to objects
var f = new Function('a', 'b', 'console.log(a + b)');f(1, 2);var fn = new Function('Parameter 1','Parameter 2'..., 'Function body')console.log(fn instanceof Object); // true
-
All functions have two properties: implicit prototype and explicit prototype
Supplement (Continued):
7.2 function call
/* 1. Ordinary function */ function fn() { console.log('The peak of life'); } fn(); /* 2. Object method */ var o = { sayHi: function() { console.log('The peak of life'); } } o.sayHi(); /* 3. Constructor*/ function Star() {}; new Star(); /* 4. Binding event function*/ btn.onclick = function() {}; // Click the button to call this function /* 5. Timer Functions */ setInterval(function() {}, 1000); This function is called once a second automatically by the timer /* 6. Execute function immediately (self calling function)*/ (function() { console.log('The peak of life'); })();
8.this
8.1 this point inside the function
These points of this are determined when we call the function. The different calling methods determine the different points of this
It usually points to our caller
8.2 change the internal this direction of the function
Handle the problem of this pointing inside the function, commonly used: bind(), call(), apply()
8.2.1 call method
The call() method calls an object. It is simply understood as the way to call a function, but it can change the this point of the function
Application scenario: often do inheritance
var o = { name: 'andy'} function fn(a, b) { console.log(this); console.log(a+b)};fn(1,2)// At this time, this refers to the window, and the running result is 3FN Call (O, 1,2) / / at this time, this refers to object o, the parameters are separated by commas, and the running result is 3
The running result of the above code is:
8.2.2 apply method
The apply() method calls a function. It is simply understood as the way to call a function, but it can change the this point of the function.
Often related to application scenario: array
fun.apply(thisArg,[argsArray]) // thisArg: the value of this specified when the fun function runs // argsArray: the value passed must be included in the array
var o = { name: 'andy' } function fn(a, b) { console.log(this); console.log(a+b) }; fn()// At this time, this points to the window, and the running result is 3 fn.apply(o,[1,2])//At this time, this refers to the object o, and the parameter is passed through the array. The running result is 3 ------------------Main application--------------------- var arr = [1,66,3,99,4]; var max = Math.max.apply(Math, arr); //Get the maximum value of the array
8.2.3 bind method
The bind() method will not call the function, but it can change the internal this point of the function and return the new function generated after the original function changes this
If you just want to change the point of this and don't want to call this function, you can use bind
Application scenario: do not call the function, but also want to change the point of this
var o = { name: 'andy' }; function fn(a, b) { console.log(this); console.log(a + b); }; var f = fn.bind(o, 1, 2); //f here is the new function returned by bind f();//Calling the new function this points to the object o parameters, separated by commas -------------------------------------------------------- // 1. The original function will not be called, and the this point inside the original function can be changed // 2. The returned function is the new function generated after the original function changes this // 3. If we don't need to call some functions immediately, but want to change the internal this point of this function, use bind at this time // 4. We have a button. When we click it, we disable it and turn it on after 3 seconds var btn1 = document.querySelector('button'); btn1.onclick = function() { this.disabled = true; // this is the btn button // var that = this; setTimeout(function() { // that.disabled = false; // this in the timer function points to window this.disabled = false; // At this time, this in the timer function points to btn }.bind(this), 3000); // this refers to the btn object } var btns = document.querySelectorAll('button'); for (var i = 0; i < btns.length; i++) { btns[i].onclick = function() { this.disabled = true; setTimeout(function() { this.disabled = false; }.bind(this), 2000); } }
8.2.4 similarities and differences among call, apply and bind
-
Common ground: you can change the direction of this
-
difference:
- Call and apply will call the function and change the internal this point of the function
- The parameters passed by call and apply are different. The parameters passed by call are separated by commas and that passed by apply are array
- bind will not call the function. You can change the internal this point of the function
-
Application scenario
- call often does inheritance
- apply is often associated with arrays For example, with the help of mathematical objects to achieve the maximum and minimum value of the array
- bind does not call the function, but also wants to change the point of this For example, change the direction of this inside the timer
function Person(color) { this.color = color; this.setColor = function(color) { return this.color; }}var test = p.setColor;test(); // This points to window ------------------------------------------------------------------------------- function fun1() {function fun2() {console.log (this);} fun2();} fun2(); // This points to window
9. Strict mode
9.1 what is strict mode
In addition to providing normal mode, JavaScript also provides strict mode. The strict mode of ES5 is a way to adopt restrictive JavaScript variants, that is, to run JS code under strict conditions.
Strict mode is only supported in browsers above IE10, and will be ignored in older browsers.
Strict mode makes some changes to the normal JavaScript semantics:
1. Eliminate some unreasonable and imprecise aspects of Javascript syntax and reduce some strange behaviors.
2. Eliminate some unsafe places in code operation and ensure the safety of code operation.
3. Improve the efficiency of the compiler and increase the running speed.
4. Some grammars that may be defined in the future version of ECMAScript are disabled to pave the way for the new version of Javascript in the future. For example, some reserved words such as class, enum, export, extends, import and super cannot be used as variable names
9.2 turn on strict mode
Strict mode can be applied to the whole script or individual functions. Therefore, when using strict mode, we can divide it into two cases: script enabling strict mode and function enabling strict mode.
-
Scenario 1: enable strict mode for script
-
Some script scripts are in strict mode and some script scripts are in normal mode, which is not conducive to file merging. Therefore, the whole script file can be placed in an anonymous function that is executed immediately. In this way, one scope is created independently without affecting others
Script script file.(function (){ //In the current self calling function, the strict mode is enabled. Besides the current function, the normal mode is "use strict"; var num = 10; function fn() {}})();// Or < script > "use strict"// The current script tag has strict mode enabled < / script > < script > // The current script tag does not turn on strict mode < / script >
-
-
Case 2: turn on strict mode for function
-
To enable strict mode for a function, you need to set "use strict"; (or 'use strict';) The declaration precedes all statements in the body of the function.
function fn(){ "use strict"; return "123";} //The current fn function has strict mode on
-
9.3 changes in strict mode
Strict mode has made some changes to the syntax and behavior of Javascript.
9.3.1 variable provisions
- In normal mode, if a variable is assigned without declaration, it is a global variable by default Strict mode prohibits this usage. Variables must be declared with the var command before they are used
- It is strictly forbidden to delete declared variables. For example, delete x; The grammar is wrong
9.3.2 this pointing problem
-
Previously, this in the global function points to the window object
-
In strict mode, this in the function in the global scope is undefined
-
In normal mode, the constructor can be called without adding new. When the normal function is used, this points to the global object window
-
In strict mode, if the constructor does not add a new call, this points to undefined. If the assignment is made, an error will be reported
-
The constructor instantiated by new points to the created object instance.
-
The timer this still points to window
-
Event, object, or point to the caller
9.3.3 function variation
- Functions cannot have arguments with duplicate names
- Function must be declared first The new version of JavaScript will introduce "block level scope" (introduced in ES6). In order to be consistent with the new version, it is not allowed to declare functions in non function code blocks
'use strict' num = 10 console.log(num)//Use undeclared variables after strict mode -------------------------------------------------------------------------------- var num2 = 1; delete num2;//Strict mode does not allow deletion of variables -------------------------------------------------------------------------------- function fn() { console.log(this); // In strict mode, this in the function in the global scope is undefined } fn(); --------------------------------------------------------------------------------- function Star() { this.sex = 'male'; } Star(); console.log(window.sex); //In normal mode, this in the ordinary constructor points to window and outputs' male '‘ // In strict mode, if the constructor does not add a new call, this points to undefined. If you assign a value to it, an error will be reported --------------------- var ldh = new Star(); console.log(ldh.sex); ---------------------------------------------------------------------------------- setTimeout(function() { console.log(this); //In strict mode, the timer this still points to window }, 2000); ---------------------------------------------------------------------------------- function fn(a, a) { console.log(a + a); //In strict mode, duplicate names are not allowed for parameters in a function }; fn(1, 2); ---------------------------------------------------------------------------------- function baz() { function eit() {} //legitimate }
More stringent model requirements reference
10. Higher order function
Higher order functions are functions that operate on other functions. They receive functions as parameters or output functions as return values.
At this point fn is a higher-order function
Function is also a data type, which can also be passed to another parameter as a parameter. The most typical is as a callback function.
Similarly, functions can also be passed back as return values
11. Closure
11.1 review of the scope of variables
Variables can be divided into two types according to different scopes: global variables and local variables.
- Global variables can be used inside functions.
- Local variables cannot be used outside a function.
- When the function is executed, the local variables in this scope will be destroyed.
11.2 what is closure
A closure is a function that has access to a variable in the scope of another function. A simple understanding is that one scope can access local variables inside another function. The function of this local variable is the closure function
11.3 function of closure
Action: extend the action range of the variable.
// The scope outside fn can access the local variables inside fn function fn() { var num = 10; function fun() { console.log(num); } return fun; } var f = fn(); //Similar to var f = function fun() {console. Log (Num);} Because the return value of fn is the function fun f();
Disadvantages: after the function is executed, the local variables in the function are not released, and the memory occupation time will become longer; Easy to cause memory leakage
Solution: it can be released in time without closing
11.4 closure cases
- The index number of the current li is obtained by means of closure
for (var i = 0; i < lis.length; i++) { // Four immediate execution functions are created using the for loop // The immediate execution function also becomes a small closure, because any function in the immediate execution function can use its i variable (function(i) { lis[i].onclick = function() { console.log(i); } })(i); } // Because the scope is formed inside the function immediately, console Log (i) will only use the i within this scope and will not be affected by the external for loop i
- - 3 seconds after applying the closure of all elements
for (var i = 0; i < lis.length; i++) { (function(i) { setTimeout(function() { console.log(lis[i].innerHTML); }, 3000) })(i); }
- Closure application - calculate taxi price
/*requirement analysis The starting price of a taxi is 13 (within 3 kilometers), and then 5 yuan will be added for every additional kilometer The user can calculate the taxi price by entering the mileage If there is congestion, the total price will be charged 10 yuan more*/ var car = (function() { var start = 13; // Starting price local variable var total = 0; // Total price local variable return { // Normal total price price: function(n) { if (n <= 3) { total = start; } else { total = start + (n - 3) * 5 } return total; }, // Cost after congestion yd: function(flag) { return flag ? total + 10 : total; } } })(); console.log(car.price(5)); // 23 console.log(car.yd(true)); // 33
11.5 cases (thinking questions)
var name = "The Window"; var object = { name: "My Object", getNameFunc: function() { return function() { return this.name; }; } }; console.log(object.getNameFunc()()) // "The Window" -----------------Disassemble------------------ var f = object.getNameFunc(); var f = function() { return this.name; } f(); //this points to window ----------------------------------------------------------------------------------- var name = "The Window"; var object = { name: "My Object", getNameFunc: function() { var that = this; return function() { return that.name; }; } }; console.log(object.getNameFunc()()) //My Object -----------------Disassemble------------------ var f = object.getNameFunc(); var f = function() { return that.name; //obeject.name } f();
12. Recursion
12.1 what is recursion
Recursion: if a function can call itself internally, then the function is a recursive function. Simple understanding: the function calls itself internally. This function is a recursive function
Note: the function of recursive function is the same as that of loop. Because recursive function is prone to "stack overflow", the exit condition return must be added.
12.2 finding factorial of 1~n by recursion
//Using recursive function to find factorial 1 * 2 * 3 * 4 * of 1~n n function fn(n) { if (n == 1) { //End condition return 1; } return n * fn(n - 1); } console.log(fn(3));
12.3 finding Fibonacci sequence by recursion
// Using recursive function to find Fibonacci sequence (rabbit sequence) 1, 1, 2, 3, 5, 8, 13, 21 // The user can input a number n to calculate the rabbit sequence value corresponding to this number // We only need to know the first two items (n-1, n-2) of N entered by the user to calculate the sequence value corresponding to n function fb(n) { if (n === 1 || n === 2) { return 1; } return fb(n - 1) + fb(n - 2); } console.log(fb(3));
12.4 traversing data using recursion
// If we want to enter the id number, we can return the data object var data = [{ id: 1, name: 'household electrical appliances', goods: [{ id: 11, gname: 'Refrigerator', goods: [{ id: 111, gname: 'Haier' }, { id: 112, gname: 'Beautiful' }, ] }, { id: 12, gname: 'Washing machine' }] }, { id: 2, name: 'Clothes & Accessories' }]; //1. Use forEach to traverse every object inside function getID(json, id) { var o = {}; json.forEach(function(item) { // console.log(item); // 2 array elements if (item.id == id) { // console.log(item); o = item; return o; // 2. We want to get the data of the inner layer 11 and 12. We can use recursive functions // There should be an array of goods in it, and the length of the array is not 0 } else if (item.goods && item.goods.length > 0) { o = getID(item.goods, id); } }); return o; }
12.5 light copy and deep copy
-
Shallow copy: copy only one layer, and copy only references at deeper object level
-
Deep copy: copy multiple layers, and each level of data will be copied
-
Object.assign(target,... source) es6 new methods can be copied
var obj = { id: 1, name: 'andy', msg: { age: 18 } }; var o = {}; ------------------Shallow copy------------- // The copy is the address of msg // for (var k in obj) { // //K is the attribute name obj[k] attribute value // o[k] = obj[k]; // } // o.msg.age = 20; Object.assign(o, obj); o.msg.age = 20; console.log(obj); ------------------Deep copy------------- // Encapsulation function function deepCopy(newobj, oldobj) { for (var k in oldobj) { // Determine which data type our attribute value belongs to // 1. Get the attribute value oldobj[k] var item = oldobj[k]; // 2. Judge whether the value is an array if (item instanceof Array) { newobj[k] = []; deepCopy(newobj[k], item) } else if (item instanceof Object) { // 3. Judge whether this value is an object newobj[k] = {}; deepCopy(newobj[k], item) } else { // 4. It belongs to simple data type newobj[k] = item; } } }
13. Regular expression overview
13.1 what is a regular expression
Regular Expression is a pattern used to match character combinations in a string. In JavaScript, regular expressions are also objects.
Regular table is usually used to retrieve and replace the text that conforms to a certain pattern (rule), such as verification form: the user name form can only enter English letters, numbers or underscores, and the nickname input box can enter Chinese (matching). In addition, regular expressions are often used to filter out some sensitive words in the page content (replacement), or get the specific part we want from the string (extraction), etc.
Other languages also use regular expressions. At this stage, we mainly use JavaScript regular expressions to complete form verification.
13.2 characteristics of regular expressions
- Flexibility, logic and functionality are very strong.
- It can quickly achieve the complex control of string in a very simple way.
- For people who have just come into contact, it is more obscure and difficult to understand. For example: ^ \ w+([-+.]\w+)*@\w+([-.]\w+)*.\w+([-.]\w+)*$
- The actual development is generally to directly copy the written regular expression However, it is required to use regular expressions and modify them according to the actual situation For example, user name: / ^ [a-z0-9#-]{3,16}$/
14. Use of regular expressions in js
14.1 creation of regular expressions
In JavaScript, you can create a regular expression in two ways.
Method 1: create by calling the constructor of RegExp object
var regexp = new RegExp(/123/); console.log(regexp);
Method 2: create regular expressions with literal values
var rg = /123/;
14.2 testing regular expressions
The test() regular object method is used to detect whether the string conforms to the rule. The object will return true or false, and its parameter is the test string.
var rg = /123/; console.log(rg.test(123));//Whether 123 appears in the matching character, and the result is true console.log(rg.test('abc'));//Whether 123 appears in the matching character. If it does not appear, the result is false
15. Special characters in regular expressions
15.1 composition of regular expressions
A regular expression can consist of simple characters, such as / abc /, or a combination of simple and special characters, such as / ab*c /. Among them, special characters are also called metacharacters, which are special symbols with special significance in regular expressions, such as ^, $, + and so on.
There are many special characters. You can refer to:
jQuery Manual: regular expressions
[regular test tool]< http://tool.oschina.net/regex )
15.2 boundary character
The boundary character (position character) in regular expression is used to indicate the position of the character. There are mainly two characters
Boundary character | explain |
---|---|
^ | Represents the text that matches the beginning of the line (starting with who) |
$ | Represents the text that matches the end of the line (with whom) |
If ^ and $are together, it means that it must be an exact match.
var rg = /abc/; // Regular expressions do not need quotation marks, whether numeric or string // /abc / as long as abc is included, the string returns true console.log(rg.test('abc')); console.log(rg.test('abcd')); console.log(rg.test('aabcd')); console.log('---------------------------'); var reg = /^abc/; console.log(reg.test('abc')); // true console.log(reg.test('abcd')); // true console.log(reg.test('aabcd')); // false console.log('---------------------------'); var reg1 = /^abc$/; // Exact matching requires abc string to meet the specification console.log(reg1.test('abc')); // true console.log(reg1.test('abcd')); // false console.log(reg1.test('aabcd')); // false console.log(reg1.test('abcabc')); // false
15.3 character class
Character class means that there are a series of characters to choose from, as long as you match one of them. All optional characters are placed in square brackets.
15.3.1 [] square brackets
Indicates that there are a series of characters to choose from, as long as you match one of them
var rg = /[abc]/; // It returns true as long as it contains a, b or c console.log(rg.test('andy'));//true console.log(rg.test('baby'));//true console.log(rg.test('color'));//true console.log(rg.test('red'));//false var rg1 = /^[abc]$/; // Choose one from three. Only the letters a, b or c return true console.log(rg1.test('aa'));//false console.log(rg1.test('a'));//true console.log(rg1.test('b'));//true console.log(rg1.test('c'));//true console.log(rg1.test('abc'));//false ---------------------------------------------------------------------------------- var reg = /^[a-z]$/ //26 English letters. Any letter returns true - indicating the range from a to z console.log(reg.test('a'));//true console.log(reg.test('z'));//true console.log(reg.test('A'));//false ----------------------------------------------------------------------------------- //Character combination var reg1 = /^[a-zA-Z0-9_-]$/; // 26 English letters (both uppercase and lowercase) any letter, 0-9, underline Dash - return true console.log(reg1.test('a'));//true console.log(reg1.test('B'));//true console.log(reg1.test(8));//true console.log(reg1.test('-'));//true console.log(reg1.test('_'));//true console.log(reg1.test('!'));//false ------------------------------------------------------------------------------------ //The addition of ^ inside the inverted square brackets indicates negation. As long as the characters in the square brackets are included, false is returned. var reg2 = /^[^a-zA-Z0-9]$/; console.log(reg2.test('a'));//false console.log(reg2.test('B'));//false console.log(reg2.test(8));//false console.log(reg2.test('!'));//true
15.3.2 quantifier
Quantifier is used to set the number of times a pattern appears.
classifier | explain |
---|---|
* | Repeat 0 or more times |
+ | Repeat 1 or more times |
? | Repeat 0 or 1 times |
{n} | Repeat n times |
{n,} | Repeat n or more times |
{n,m} | Repeat n to m times |
// *It is equivalent to > = 0 appearing 0 or many times. Var reg = / ^ A * $/ console log(reg.test('')); // trueconsole. log(reg.test('a')); // trueconsole. log(reg.test('aaaa')); // True / / + is equivalent to > = 1. It occurs once or many times. Var reg = / ^ A + $/ console log(reg.test('')); // falseconsole. log(reg.test('a')); // trueconsole. log(reg.test('aaaa')); // true// ? Equivalent to 1|0var reg = / ^ a$/ console. log(reg.test('')); // trueconsole. log(reg.test('a')); // trueconsole. log(reg.test('aaaa')); // False / / {3} repeat 3 times var reg = / ^ a {3} $/ console log(reg.test('')); // falseconsole. log(reg.test('a')); // falseconsole. log(reg.test('aaaa')); // falseconsole. log(reg.test('aaa')); // True / / {3,} is equivalent to > = 3var reg = / ^ a {3,} $/ console log(reg.test('')); // falseconsole. log(reg.test('a')); // falseconsole. log(reg.test('aaaa')); // trueconsole. log(reg.test('aaa')); // True / / {3,6} is equivalent to > = 3 & & < = 6var reg = / ^ a {3,6} $/ console log(reg.test('')); // falseconsole. log(reg.test('a')); // falseconsole. log(reg.test('aaaa')); // trueconsole. log(reg.test('aaa')); // trueconsole. log(reg.test('aaaaaaa')); // false
15.3.3 user name form verification
Functional requirements:
- If the user name is valid, the following prompt message is: the user name is valid and the color is green
- If the user name input is illegal, the following prompt message is: the user name does not meet the specification, and the color is red
analysis: - The user name can only be composed of English letters, numbers, underscores or dashes, and the length of the user name is 6 ~ 16 digits
- First, prepare the regular expression pattern / $[a-za-z0-9 -] {6,16}^/
- Validation begins when the form loses focus
- If it meets the regular specification, let the following span tag add the right class
- If it does not conform to the regular specification, let the following span tag add the wrong class
<input type="text" class="uname"> <span>enter one user name</span> <script> // Quantifier is to set the number of times a certain pattern appears var reg = /^[a-zA-Z0-9_-]{6,16}$/; // In this mode, the user can only input English alphanumeric underline and underline var uname = document.querySelector('.uname'); var span = document.querySelector('span'); uname.onblur = function() { if (reg.test(this.value)) { console.log('correct'); span.className = 'right'; span.innerHTML = 'User name format input is correct'; } else { console.log('FALSE'); span.className = 'wrong'; span.innerHTML = 'Incorrect user name format'; } } </script>
15.3.4 bracketed summary
1. Brace quantifier It indicates the number of repetitions
2. Bracketed character set. Matches any character in parentheses
3. Parentheses indicate priority
Regular expression online test
// Bracketed character set Matches any character in parentheses var reg = /^[abc]$/; // A can also be b can also be c can be a | b | c // Brace quantifier It indicates the number of repetitions var reg = /^abc{3}$/; // It just makes c repeat abccc three times console.log(reg.test('abc')); // false console.log(reg.test('abcabcabc')); // false console.log(reg.test('abccc')); // true // Parentheses indicate priority var reg = /^(abc){3}$/; // It makes abcc repeat three times console.log(reg.test('abc')); // false console.log(reg.test('abcabcabc')); // true console.log(reg.test('abccc')); // false
15.4 predefined classes
Predefined classes are shorthand for some common patterns
Case: verify the landline number
var reg = /^\d{3}-\d{8}|\d{4}-\d{7}$/; var reg = /^\d{3,4}-\d{7,8}$/;
Form validation case
//Mobile number verification: / ^ 1 [3 | 4 | 5 | 7 | 8] [0-9] {9} $/; //Verify whether to replace the class name of the element and the content in the element if (reg.test(this.value)) { // console.log('correct '); this.nextElementSibling.className = 'success'; this.nextElementSibling.innerHTML = '<i class="success_icon"></i> Congratulations on your correct input'; } else { // console.log('incorrect '); this.nextElementSibling.className = 'error'; this.nextElementSibling.innerHTML = '<i class="error_icon"></i>Incorrect format,Please input from New '; }
//QQ number verification: / ^ [1-9]\d{4,} $/; //Nickname verification: / ^ [\ u4e00-\u9fa5]{2,8}$/ //Verify whether it passes or not, replace the class name of the element and the content in the element, encapsulate the matching code in the previous step, and call it multiple times function regexp(ele, reg) { ele.onblur = function() { if (reg.test(this.value)) { // console.log('correct '); this.nextElementSibling.className = 'success'; this.nextElementSibling.innerHTML = '<i class="success_icon"></i> Congratulations on your correct input'; } else { // console.log('incorrect '); this.nextElementSibling.className = 'error'; this.nextElementSibling.innerHTML = '<i class="error_icon"></i> Incorrect format,Please input from New '; } } };
//Password verification: / ^ [a-za-z0-9 -]{6,16}$/ //If you enter the password again, you only need to match whether it is consistent with the password value you entered last time
15.5 regular replace
The replace() method can replace a string, and the parameter used to replace can be a string or a regular expression.
stringObject.replace(regexp/substr,replacement)
- The first parameter: the replaced string or regular expression
- Second parameter: replace with the string
- The return value is a new string after replacement
/expression/[switch]
switch (also known as modifier) matches according to what pattern. There are three values:
- g: Global matching
- i: Ignore case
- gi: global match + ignore case
var str = 'andy and red'; var newStr = str.replace('andy', 'baby'); console.log(newStr)//baby and red //andy, which is equivalent here, can be written in a regular expression var newStr2 = str.replace(/andy/, 'baby'); console.log(newStr2)//baby and red //replace all var str = 'abcabc' var nStr = str.replace(/a/,'ha-ha') console.log(nStr) //Ha ha bcabc //Replace all g var nStr = str.replace(/a/g,'ha-ha') console.log(nStr) //Ha ha bc ha ha bc //Ignore case i var str = 'aAbcAba'; var newStr = str.replace(/a/gi,'ha-ha')//"Ha ha ha bc ha ha b ha ha"
Case: filter sensitive words
<textarea name="" id="message"></textarea> <button>Submit</button> <div></div> <script> var text = document.querySelector('textarea'); var btn = document.querySelector('button'); var div = document.querySelector('div'); btn.onclick = function() { div.innerHTML = text.value.replace(/passion|gay/g, '**'); } </script>