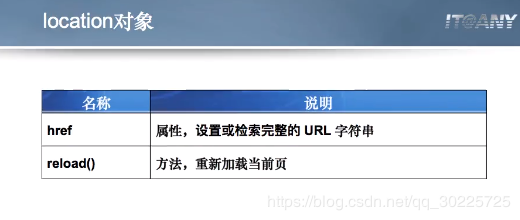
< a class = "BTN BTN SM BTN red hole attention" id = "btnattent" target = "blank" > more free teaching articles < font color = "blue" size = "2" > please pay attention here < / font ></a>
Get the url of the address bar

Change the address to realize page Jump:

Definition and Usage
<font color="red">
Refresh the address url of the address bar
The reload() method is used to reload the current document.
grammar
location.reload(force) description
If the method does not specify a parameter, or if the parameter is false, it uses the HTTP header if modified since to detect whether the document on the server has changed. If the document has changed, reload() downloads the document again. If the document does not change, the method loads the document from the cache. This is exactly the same as when the user clicks the browser's refresh button.
If the parameter of this method is set to true, no matter what the last modification date of the document is, it will bypass the cache and download the document from the server again. This is exactly the same as holding down the Shift key when the user clicks the browser's refresh button.
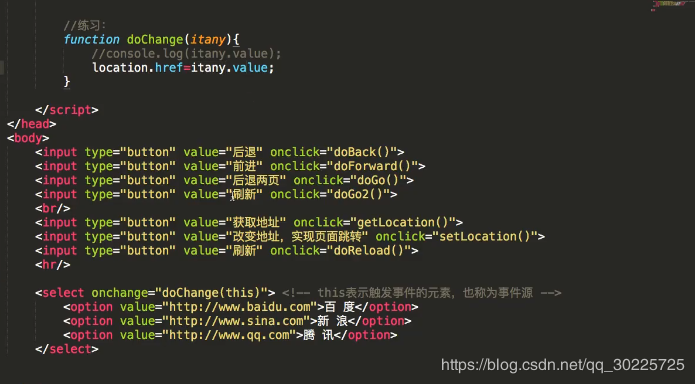
Source code:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Document</title> <script type="text/javascript"> var timer; function start(){ timer=setTimeout(callback,2000); //One time timer, execute the callback function in 2 seconds } function callback(){ console.log("Timer starts!"); } var timer2; function start2(){ timer2=setInterval(callback,2000); //Periodic timer to perform the callback function every 2 seconds } function stop(){ clearTimeout(timer); //clearInterval(timer); //clearInterval() can stop both one-time and periodic timers } function stop2(){ clearInterval(timer2); } //Exercise: output welcome to wbs16073 every second //Mode 1, use setInterval() function start3(){ timer=setInterval(show,1000); } var i=1; function show(){ console.log(i+".welcome to wbs16073"); i++; } //Mode 2, use setTimeout() /*function start4(){ show(); } var i=1; function show(){ console.log(i+".welcome to wbs16073"); i++; timer=setTimeout(show,1000); //After each execution, turn on a one-time timer }*/ function stop3(){ clearInterval(timer); } function stop4(){ clearTimeout(timer); } function start5(){ setTimeout(function(){ alert("This is an anonymous function!"); },2000); } </script> </head> <body> <input type="button" value="setTimeout" onclick="start()"> <input type="button" value="setInterval" onclick="start2()"> <br/> <input type="button" value="clearTimeout" onclick="stop()"> <input type="button" value="clearInterval" onclick="stop2()"> <br/> <input type="button" value="Mode 1" onclick="start3()"> <input type="button" value="Mode 2" onclick="start4()"> <br/> <input type="button" value="Stop mode 1" onclick="stop3()"> <input type="button" value="Stop mode 2" onclick="stop4()"> <br/> <input type="button" value="Using anonymous functions" onclick="start5()"> </body> </html>