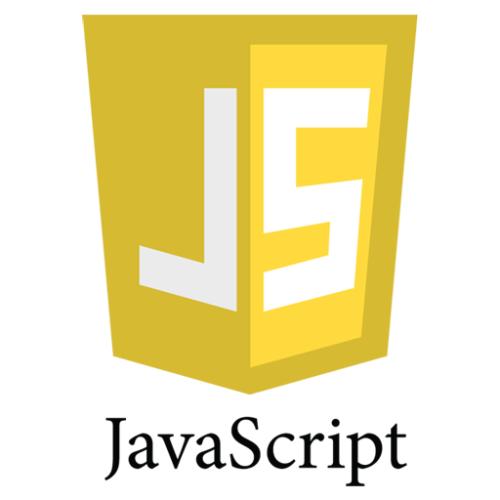
Using JavaScript technology to process statistics in browsers has the best dissemination effect
Functions are blocks of code designed to perform specific tasks.It has an entry and an exit while implementing custom operations.The so-called entrance refers to the various parameters that a function carries. Through this entrance, we can substitute the parameter values of a function into a subroutine for computer processing. The so-called exit refers to the function values of a function, which are calculated by the computer and brought back to the program calling it.
In program design, some commonly used function modules are often written as functions and placed in the function library for users to choose from.Being good at using functions can reduce the amount of work required to repeat programming segments.
In short, a JS function is a small piece of code that accomplishes a common function, while a JS method is a javascript function called through an object.That is to say, methods are functions, but special functions that belong to specified objects.When you bind a function to an object, it becomes a method of an object.
Everything in JS is an object, and an object is data that has properties and methods.However, JS objects are created by functions, and JS functions themselves are objects."Method is a function and object is a function". The purpose and meaning of function in JS is a little..
Starting from "JS function is a small piece of code that can complete a common function", this paper introduces several common definitions of JS function.After mastering the basic technology of JS function, we will understand the function as a method customized by a statistical object (project) in the process of statistical data processing.
1. General Functions
[Back] I. Basic GrammarFunction function name (parameter 1, parameter 2, parameter 3) { Code to execute Return return value }
The JavaScript function syntax parses as follows:
- JavaScript functions are defined by the function keyword followed by the function name and parentheses ()
- Function names can contain letters, numbers, underscores, and dollar symbols
- Parentheses can include comma-separated parameters
- Code executed by the function is placed in curly brackets {}
- The return value of a function is specified by the return keyword
II. Parameterless Functions
console.clear(); function test1() { return "Hello World!"; } //Executing the function returns "Hello World!" and assigns it to the variable str var str = test1(); console.log(str);
III. Functions with parameters
//Calculate the sum of any two numbers function oSum(x, y) { return x+y; } var mySum = oSum(15, 85); console.log(mySum); //100
IV, parameter default settings
//Setting default values for two parameters in a summation function console.clear(); function oSum(x = 50, y = 50) { return x+y; } var mySum = oSum(15, 55); console.log(mySum); //70 mySum = oSum(15); console.log(mySum); //65 mySum = oSum(); console.log(mySum); //100
V. Parameters are functions
console.clear(); function oSum(x, y) {return x+y;} var mySum = oSum(oSum(45, 75), 100); console.log(mySum); //220
VI, Object {} Assigning Default Values for Parameter-Deconstruction
console.clear(); function oSum({x = 10, y = 20}) { return x+y; } console.log(oSum({})); //30, error calling oSum() directly here console.log(oSum({x:100})); //120 console.log(oSum({y:100})); //110 console.log(oSum({x:100,y:200})); //300 console.log(oSum({y:50,x:20})); //70
//Code fault tolerance is better console.clear(); function oSum({x = 10, y = 20} = {}) { return x+y; } console.log(oSum()); //30 console.log(oSum({})); //30 console.log(oSum({x:100})); //120 console.log(oSum({y:100})); //110 console.log(oSum({x:100,y:200})); //300 console.log(oSum({y:50,x:20})); //70
2. Function arguments object
[Back]In JavaScript, parameters are represented as an array inside a function, and arguments is an array object inside a function that is dedicated to storing arguments.The object of the function arguments is used to detect and reset parameters while the function is running.
I,arguments.length-Number of function parameters
console.clear(); function fn(a,b,c){ console.log(arguments.length); //3 return a+b+c; } console.log(fn(1,2,3)); //6
II, arguments[] - Function output and settings
console.clear(); function fn(a,b,c){ console.log(arguments[0]); //1 console.log(arguments[1]); //2 console.log(arguments[2]); //3 arguments[0] = 5; //Reset parameter a=5 return a+b+c; } console.log(fn(1,2,3)); //10
III. arguments to Array
The arguments object is not a real array; it is similar to an array, but it has no array properties other than the length attribute and index elements.For example, it does not have a pop method.But it can be converted to a real array, and the full array method can be used once converted to a real array
console.clear(); function fn(a, b, c) { var arr = Array.from(arguments); //Rotate Array console.log(arr); //Output Array return a + b + c; } console.log(fn(1, 2, 3)); //6
3. Anonymous functions
[Back]Anonymous functions, as the name implies, refer to functions without names. They are used frequently in actual development and are the focus of learning JS well.
I. Variable Anonymous Functions
console.clear(); var fn = function fn(a,b,c=9) { return a+b+c; } console.log(fn(1,2)); //12 console.log(fn(1,2,3)); //6
II. Anonymous function without name
That is, when a function is declared, it is immediately followed by a parameter.When the Js syntax parses this function, the code inside executes immediately.
console.clear(); console.log(function(a,b){return a+b;}(2,3)); console.clear(); //Parentheses execute anonymous functions (output "Hello Word!") (function (){ console.log("Hello Word!"); })() //Parentheses execute anonymous functions (output Hello Word! lei.) (function (str){ console.log("Hello Word!" +" "+ str +"."); })("lei")
III. Event-bound anonymous functions
Usually when we browse a web page, we enter information through the mouse or keyboard, such as click event when the mouse clicks on a button, or change event when the text information in the text box is changed.In summary, all mouse and keyboard actions of a user are referred to as events, which can be captured by JS code.
console.clear(); //By id or by icon object var logo=document.querySelector("#myLogo"); //Add a click event to the Galaxy Statistics icon in the upper left corner logo.onclick=function(){ console.log("Welcome to Galactic Statistics Studio!"); } logo.onmousemove=function(){ logo.style.cursor="pointer"; console.log("Mouse over image!"); } logo.onmouseout=function(){ logo.style.cursor="default"; console.log("The mouse has left the image!"); }
IV. Object Binding Anonymous Functions as Objects
Earlier, when we introduced JS objects, we mentioned that events are data structures composed of attributes and methods, and that event methods can be established by binding anonymous functions.
console.clear(); var obj={ name:"Carolyn", age:11, fn:function(str){ return "My name is "+this.name+" Siyu"+". I'm "+this.age+" years old now."; } }; console.log(obj.fn("Siyu")); //My name is Carolyn Siyu. I'm 11 years old now.
V. Anonymous function as callback function
What is synchronization and what is asynchronization?
Synchronization means that only one task can be completed at a time.If you have more than one task, you have to queue up, the first task completes, the next one executes, and so on.
Asynchronous means that each task has one or more callback functions. When the previous task is finished, the callback function is executed instead of the latter. The latter task is executed before the previous task is finished, so the execution order of the program is not consistent with the order of the tasks.
JS is single-threaded and cannot be asynchronous in itself. It can be asynchronous through callback functions.The most basic asynchronouss in JS are the setTimeout and setInterval functions.
console.clear(); var interval = setInterval(function(){ console.log("Callback function, which is executed every second"); },1000); //Stop setInterval Calculator by clicking on Galaxy Statistics Chart var logo=document.querySelector("#myLogo"); logo.onclick=function(){ console.log("Stop!"); clearInterval(interval) } setTimeout(function() { console.log("Zhang San") }, 1000 ); setTimeout(function() { console.log("Li Si") }, 2000 ); setTimeout(function() { console.log("King Five") }, 3000 ); //The most basic asynchronous implementation of jS function a() { console.log("implement a function"); setTimeout(function() { console.log("implement a Delay function of function"); },2000); } function b() { console.log("implement b function"); } a(); b();
VI. Anonymous function as return value
console.clear(); function fn(){ return function(){ return "Carolyn"; } } //Calling anonymous functions console.log(fn()()); //Carolyn //perhaps var box=fn(); console.log(box()); //Carolyn
4. Closures and Recursive Functions
[Back]Assuming that function A declares a function B internally, that function B references variables other than function B, and that the return value of function A is a reference to function B, function B is a closure function.
Recursion means that a function calls itself, and when it calls itself, it is itself a closure function and its parameters are out-of-domain variables.
console.clear(); function funA(arg1,arg2) { var i = 0; //Variables of funA scope function funB(step) { i = i + step; //Access funB out-of-scope variable i console.log(i) } return funB; } var allShowA = funA(2, 3); //Called funA arg1=2, arg2=3 allShowA(1);//Called funB step=1, output 1 allShowA(3);//Called funB setp=3, output 4 //accumulation console.clear(); function f(num){ if(num<1){ return 0; }else{ return f(num-1)+num; } } console.log(f(9)); //45 //Factorial console.clear(); function f(num){ if(num<1){ return 1; }else{ return f(num-1)*num; } } console.log(f(4)); //24 //Define recursive methods in objects var obj = { num : 5, fac : function (x) { if (x === 1) { return 1; } else { return x * obj.fac(x - 1); } } }; console.log(obj.fac(5)) /120 //UseArguments.callee function fact(num){ if (num<=1){ return 1; }else{ return num*arguments.callee(num-1); } } console.log(fact(4)); //24
This paper introduces the various uses of JS functions, and combined with the conditions and looping techniques that will follow, LS functions will become extremely powerful.
Tip: The JS script code on this page can be copied and pasted to JS Code Run Window Debugging experience; Text editing shortcuts: Ctrl+A-Select All; Ctrl+C-Copy; Ctrl+X-Cut; Ctrl+V-Paste