[JavaScript Title brushing] simple implementation of array, string and ArrayList
Compared with other languages, JavaScript strings have nothing special. They are immutable. Each update will create a new string, which is the same as most programming languages.
Array has some interesting features compared with other programming languages: it is dynamic, its length is not fixed, and it does not need special declarations. These special lines actually prefer array list to array list.
However, JavaScript arrays also have two special functions:
-
It can directly modify the length of the array by operating the length attribute of the array. This feature should also be considered unique in the programming language:
const arr = []; // Directly modify the array length arr.length = 10;
-
Arrays in JavaScript can store multiple types of data, rather than specifying that only a single type of data can be stored
const arr = []; arr.push(1); arr.push('hello world'); arr;
Large O of array
In view of the large number of JavaScript engines and the different implementation of each browser, there is no absolute answer to this question. from Big O of JavaScript arrays On the Q & a section of Stack Overflow, there is a post posted last year, which explains that V8, for example, currently uses hashtable and array list to implement JavaScript array.
The large O of hashtable and array list are as follows:
-
increase
On average O ( n ) O(n) O(n), subscript in the array i i i this position inserts a value that represents the need to move i + 1 i+1 All values after i+1.
-
change
O ( 1 ) O(1) O(1) to access any known subscript i i i can almost access this address directly.
-
check
As mentioned earlier, by knowing the subscript i i i visit almost all of them O ( 1 ) O(1) O(1).
Deleting an element is a special operation. According to this post, using delete array[index] may reconstruct the performance of the entire array, so it may be worse than the conventional deletion operation.
Another Stack Overflow discussion Deleting array elements in JavaScript - delete vs splice More points are mentioned in. Using delete array[index] will delete the prototype in the array, modify the attributes of the object, and will not actually delete the elements in the array:
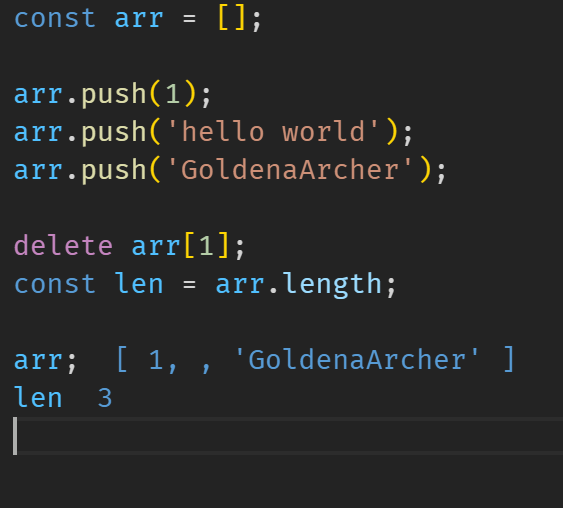
In addition, in Chapter 4 of the Red Treasure book JavaScript Advanced Programming Chapter 4 learning notes The optimization of hidden classes has also been mentioned, that is, the JavaScript code interpreted by the V8 engine of Chrome will use hidden classes. When initializing variables, judge whether the same hidden classes can be used. If possible, hidden classes will be shared to optimize them.
Once the prototypes of objects are inconsistent - deleting will clear the prototypes of Array objects, which will cause the copy of multiple Array objects, thus affecting the running efficiency of the code.
Implementing ArrayList using JavaScript
The JavaScript array itself is already very dynamic. Here, use JavaScript to simulate the implementation of ArrayList in Java. By the way, get familiar with the operation of Jest.
I have to say that Jest is really easy to use. This is a simple overview:
In the test cases, I wrote 11 test cases. The results after running yarn test or npm test are as follows:

The following simple report:
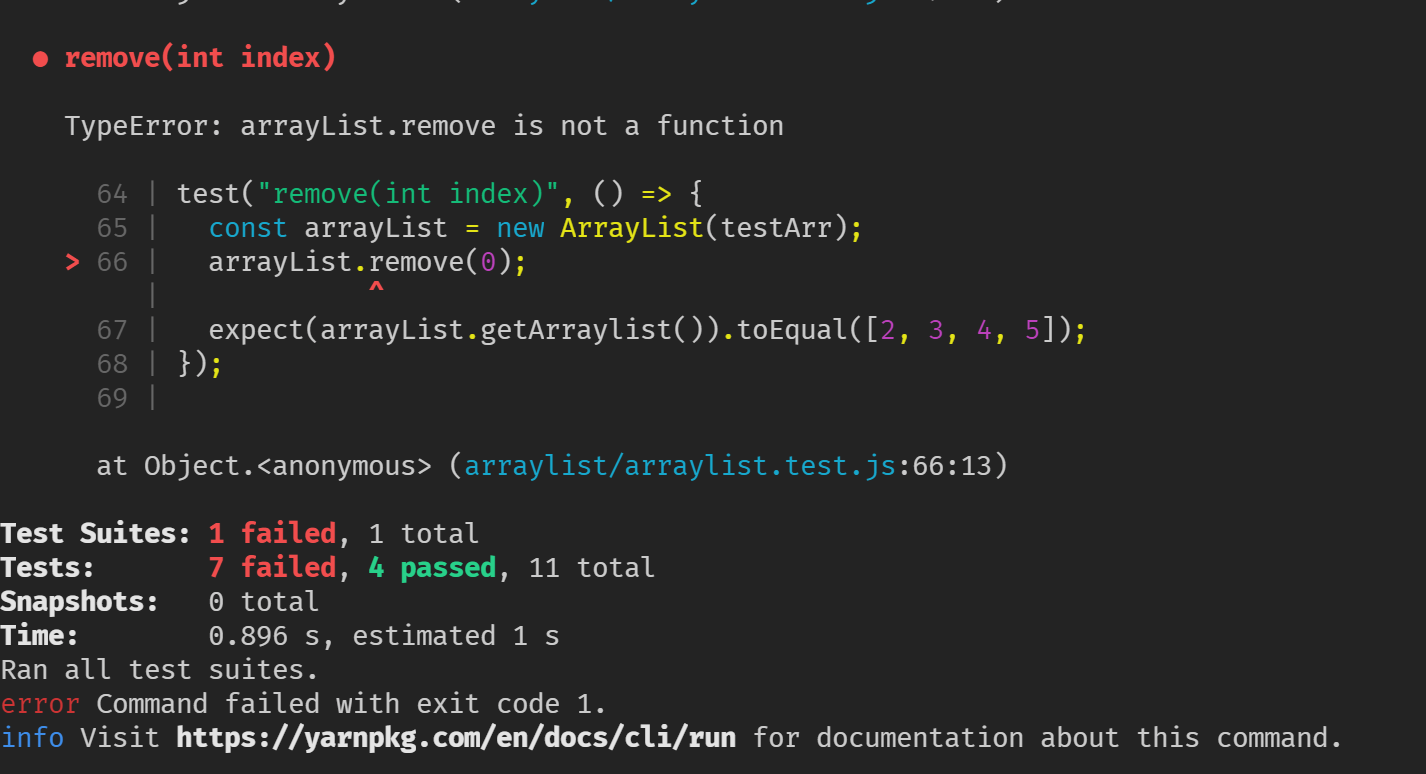
And the command line and HTML version of the test coverage:
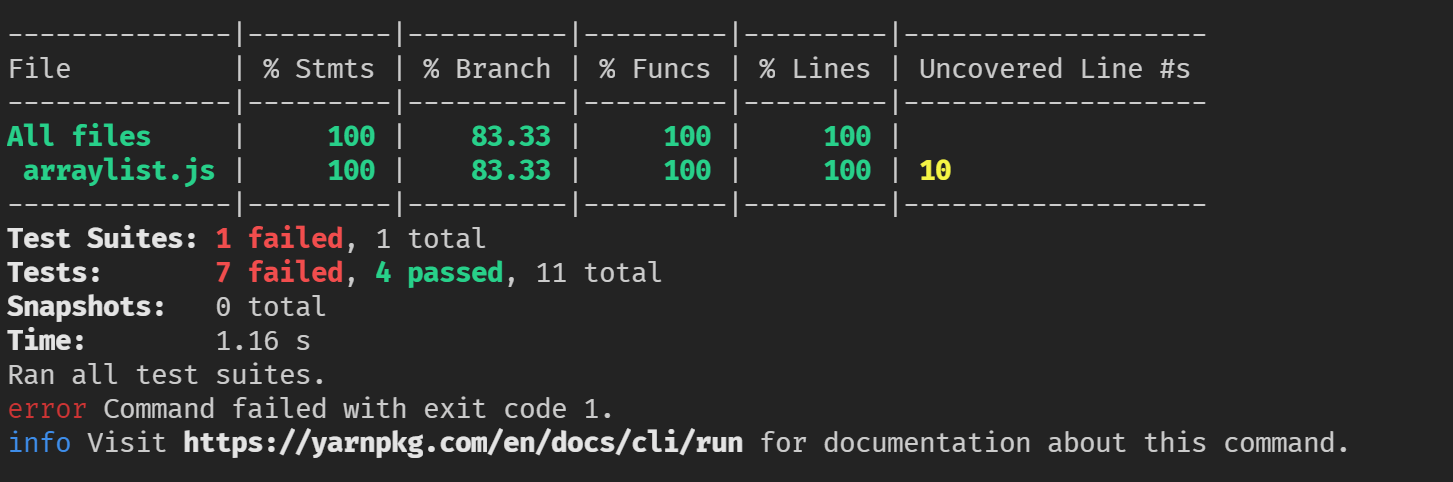

Complete implementation code:
class ArrayList { arraylist = undefined; DEFAULT_CAPACITY = 10; length = 0; constructor(param) { if (param === undefined) { this.arraylist = new Array(this.DEFAULT_CAPACITY); this.length = 0; } else if (typeof param === 'number') { this.arraylist = new Array(param); this.length = param; } else if (typeof param[Symbol.iterator]) { this.arraylist = Array.from(param); this.length = this.arraylist.length; } } getArraylist() { return this.arraylist; } size() { return this.length; } add(element) { this.arraylist[this.length++] = element; } addAll(iterable) { const newArray = Array.from(iterable); this.arraylist.splice(this.length, 0, ...newArray); this.length += newArray.length; } contains(val) { return this.arraylist.includes(val); } clear() { this.arraylist.fill(undefined); this.length = 0; } get(index) { return this.arraylist[index]; } indexOf(target) { return this.arraylist.indexOf(target); } isEmpty() { return this.length === 0; } remove(index) { this.arraylist.splice(index, 1); } } module.exports = ArrayList;
The complete implementation code and test code are here: JavaScript implements basic ArrayList functions
other
Other learning notes about JavaScript arrays written before.
Can't JavaScript foreach use break/continue? In the same way, the for loop does not work