var keys = Object.getOwnPropertyNames(Person.prototype); console.log(keys);//["constructor", "name", "age", "job", "sayHi"] var obj = { 0: "a", 1: "b", 2: "c"}; console.log(Object.getOwnPropertyNames(obj).sort()); // ["0", "1", "2"]
preface
The content of this chapter will be expanded according to the following figure:
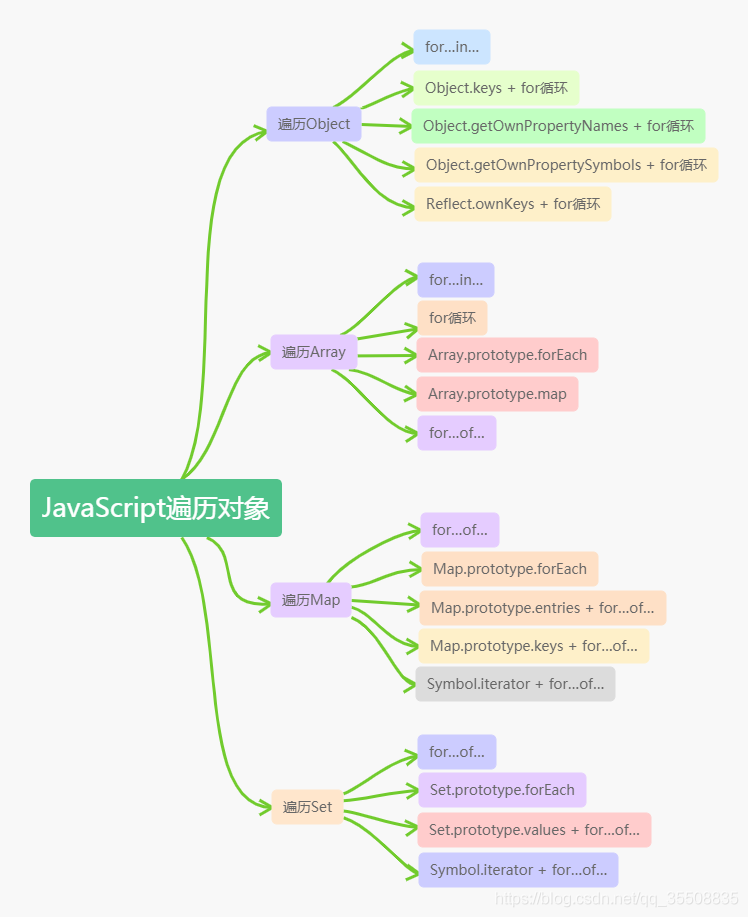
Traversal Object
The most common traversal method of object is to use for... In... But it has some limitations. For example, it can only traverse enumerable attributes. Although object cannot directly use the for loop and forEach, it is reflected ownKeys / Object. getOwnPropertyNames / Object. getOwnPropertySymbols / Object. After the key value set in the object is directly obtained through the conversion of keys and other methods, it can be traversed through the for loop and forEach.
Method | Get property type |
---|---|
Object.keys | Enumerable properties |
Object.getOwnPropertySymbols | Symbol Properties |
Object.getOwnPropertyNames | Enumerable and non enumerable properties |
Reflect.ownKeys | Cannot enumerate attributes and Symbol attributes |
For example, there is an object with three attributes, namely enumerable attribute, non enumerable attribute and Symbol attribute:
let person = { name : 'xiaoming' } person[Symbol('sex')] = 'man'; Object.defineProperty(person, 'age', { value : 18, writable: true, configurable: false, enumerable: false })
Use for... in... To traverse
for(let key in person){ console.log('key : ' + key + ' , value : ' + person[key]); }
Output results:
Use object Keys + for loop traversal
let keys2 = Object.keys(person); for(let index = 0, length = keys2.length; index < length; index++){ let key = keys2[index]; let value = person[key]; console.log('key : ' + key + ' , value : ' + value); }
Output results:
Use object Getownpropertynames + for loop traversal
let names = Object.getOwnPropertyNames(person);
for(let index = 0, length = names.length; index < length; index++){
let key = names[index];
let value = person[key];
console.log('key : ' + key + ' , value : ' + value);
}
Output results:

Use object Getownpropertysymbols + for loop traversal
let symbols = Object.getOwnPropertySymbols(person); for(let index = 0, length = symbols.length; index < length; index++){ let key = symbols[index]; let value = person[key]; typeof key === 'symbol' ? key = Symbol.prototype.toString.call(key) : ''; console.log('key : ' + key + ' , value : ' + value); }
Output results:
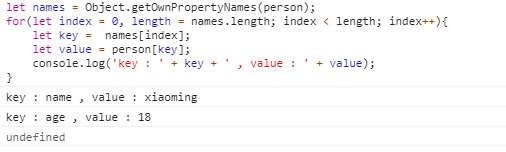
Use reflect Ownkeys + for loop traversal
let keys = Reflect.ownKeys(person); for(let index = 0, length = keys.length; index < length; index++){ let key = keys[index]; let value = person[key]; typeof key === 'symbol' ? key = Symbol.prototype.toString.call(key) : ''; console.log('key : ' + key + ' , value : ' + value); }
Output results:
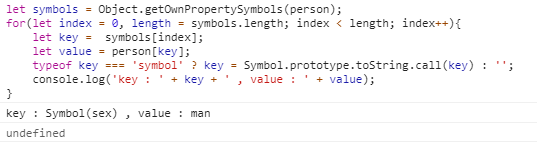
Traverse Array
The for loop can be used to traverse the Array, and the for... in... And for... Of... Can also be used. in the prototype of Array, forEach function and map function can be used to traverse the Array object.
Test data:
let arr = ['item_1', 'item_2', 'item_3', 'item_4', 'item_5'];
Use for... in... To traverse
for(let index in arr){ console.log('index : ' + arr[index]); }
Output results:

Use for loop traversal
f
for(let index = 0, length = arr.length; index < length; index += 1){ console.log('index : ' + arr[index]); }
Output results:
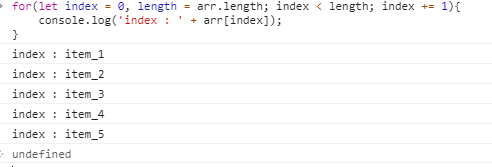
Using array prototype. Foreach traversal
Array.prototype.forEach.call(arr, (value) => { console.log('value : ' + value); })
Output results:

Using array prototype. Map traversal
Array.prototype.map.call(arr, (value) => { console.log('value : ' + value); })
Output results:
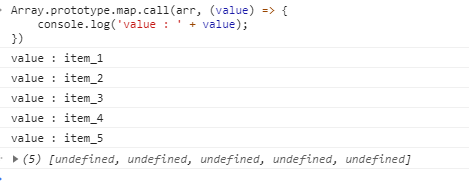
for... of... Traversal
The map method creates a new array based on the data returned after calling the function for each element in the original array.
Use for... of... To traverse
Array.prototype.map.call(arr, (value) => { console.log('value : ' + value); })
Output results:
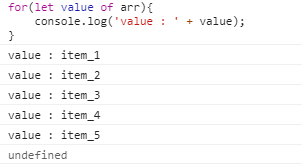
Traverse Map
There are generally two ways to traverse a map. One is to directly use for... Of... Or map prototype. Foreach, the second is through map prototype. entries / Map. prototype. keys / Symbol. The iterator obtains the iterator of the map object, and then traverses the iterator through for... Of.
Test data:
l
et map = new Map(); map.set('key_1', 'value_1'); map.set('key_2', 'value_2'); map.set('key_3', 'value_3'); map.set('key_4', 'value_4'); map.set('key_5', 'value_5');
Object. The keys () method obtains all enumerable instance properties on the object
Receive an object as a parameter and return a string array containing all enumerable properties.
function Person() { } Person.prototype.name = "Tom Holland"; Person.prototype.age = 23; Person.prototype.job = "Spider man"; Person.prototype.sayHi = function() { alert("love you three thousand times"); }; var keys = Object.keys(Person.prototype); console.log(keys);// ["name", "age", "job", "sayHi"] var p1 = new Person(); p1.name = "Robert Downey Jr."; p1.age = 54; var p1keys = Object.keys(p1); console.los(p1keys);//["name", "age"]
-
The for in loop returns all enumerable properties that can be accessed through the object
Includes properties that exist in the instance and properties that exist in the prototype -
Object. The getownpropertynames () method obtains all instance properties, whether enumerable or not (but excluding the properties with Symbol value as name)