Original address: https://www.cloudcrossing.xyz/post/43/
1 Map set
1.1 Overview of Map Collections
Map is an object that maps keys and values one by one. It can get values by keys. A mapping cannot contain duplicate keys; each key can be mapped to at most one value.
What's the difference between Map and Collection?
- A: Map stores elements in the form of key-value pairs. Keys are unique and values can be repeated.
- B: Collection stores separate elements
- Subinterface Set element unique
- Subinterface List elements can be repeated
- C: Data structure
- The data structure of a Map set is valid only for keys, independent of values
- The data structure of Collection sets is valid for elements
1.2 Map Collection Function
- A: Add functionality
- V put(K key, V value): Add elements
- If the key is stored for the first time, it stores the element directly and returns NULL
- If the key is not stored for the first time, replace the previous value with the value and return the replaced value.
- V put(K key, V value): Add elements
- B: Delete function
- void clear(): Remove all key-value pair elements
- V remove(Object key): Delete key-value pair elements according to the specified key and return the deleted value
- C: Judgment function
- boolean containsKey(Object key): Determines whether a collection contains the specified key
- boolean containsValue(Objecy value): Determines whether a collection contains a specified value
- boolean isEmpty(): Determines whether the collection is empty
- D: Acquisition function
- Set < Map. Entry < K, V > entrySet (): Returns a collection of key-to-value objects
- V get(Object key): Gets the value of the specified key
- Set < K > keySet (): Gets the collection of all keys in the collection
- Collection < V > values (): Gets the collection of all values in the collection
- int size(): Returns the logarithm of key-value pairs in a collection
import java.util.HashMap; import java.util.Map; public class MapDemo { public static void main(String[] args) { // Creating Collection Objects Map<String, String> map = new HashMap<String, String>(); // Additive elements // V put(K key,V value): Add elements // System.out.println ("put:"+map.put ("article", "Mayi Terra Cotta"); //NULL // System.out.println ("put:"+map.put ("article", "Yao Di");//Mayili map.put("Deng Chao", "Sun Li"); map.put("Huang Xiaoming", "Angela Baby"); map.put("Jay Chou", "Jolin"); // void clear(): Remove all key-value pair elements // map.clear(); // V remove(Object key): Delete key-value pair elements based on keys and return values // System.out.println ("remove:"+map.remove ("Huang Xiaoming");//Yang Ying // System.out.println ("remove:"+map.remove ("Huang Xiaobo");//null // boolean containsKey(Object key): Determines whether a collection contains the specified key // System.out.println("containsKey:" + map.containsKey("Huang Xiaoming");//true // System.out.println("containsKey:" + map.containsKey("Huang Xiaobo");//false // boolean isEmpty(): Determines whether the collection is empty // System.out.println("isEmpty:"+map.isEmpty()); //int size(): Returns the logarithm of key-value pairs in a collection System.out.println("size:"+map.size()); // Output Collection Name System.out.println("map:" + map); } }
import java.util.Collection; import java.util.HashMap; import java.util.Map; import java.util.Set; public class MapDemo2 { public static void main(String[] args) { // Creating Collection Objects Map<String, String> map = new HashMap<String, String>(); // Create and add elements map.put("Deng Chao", "Sun Li"); map.put("Huang Xiaoming", "Angela Baby"); map.put("Jay Chou", "Jolin"); // V get(Object key): Get values based on keys System.out.println("get:" + map.get("Jay Chou")); //Jolin System.out.println("get:" + map.get("Zhou Jie")); // null System.out.println("----------------------"); // Set < K > keySet (): Gets the collection of all keys in the collection Set<String> set = map.keySet(); for (String key : set) { System.out.println(key);//Output all keys } System.out.println("----------------------"); // Collection < V > values (): Gets the collection of all values in the collection Collection<String> con = map.values(); for (String value : con) { System.out.println(value);//Output all values } } }
1.3 Map set traversal
A: Find values by keys:
- A: Get a collection of all keys
- b: traverse the set of keys to get each value
- c: Find values from keys to collections
import java.util.HashMap; import java.util.Map; import java.util.Set; public class MapDemo3 { public static void main(String[] args) { // Creating Collection Objects Map<String, String> map = new HashMap<String, String>(); // Create elements and add them to collections map.put("Yang Guo", "Little dragon maiden"); map.put("Guo Jing", "Huang Rong"); map.put("Yang Kang", "Mu Ni Chi"); // ergodic // Get all the keys Set<String> set = map.keySet(); // Traverse through the set of keys to get each key for (String key : set) { // Find values by keys String value = map.get(key); System.out.println(key + "---" + value); } } }
B: Find keys and values for objects by key values:
- A: Gets a collection of all key-value pairs of objects
- b: traverse the set of key-value pairs of objects to get each key-value pair of objects
- c: Get keys and values based on key-value pairs of objects
import java.util.HashMap; import java.util.Map; import java.util.Set; public class MapDemo4 { public static void main(String[] args) { // Creating Collection Objects Map<String, String> map = new HashMap<String, String>(); // Create elements and add them to collections map.put("Yang Guo", "Little dragon maiden"); map.put("Guo Jing", "Huang Rong"); map.put("Yang Kang", "Mu Ni Chi"); // Gets a collection of all key-value pairs of objects Set<Map.Entry<String, String>> set = map.entrySet(); // Traversing the set of key-value pairs of objects to get each key-value pair of objects for (Map.Entry<String, String> me : set) { // Getting keys and values from key-value pairs of objects String key = me.getKey(); String value = me.getValue(); System.out.println(key + "---" + value); } } }
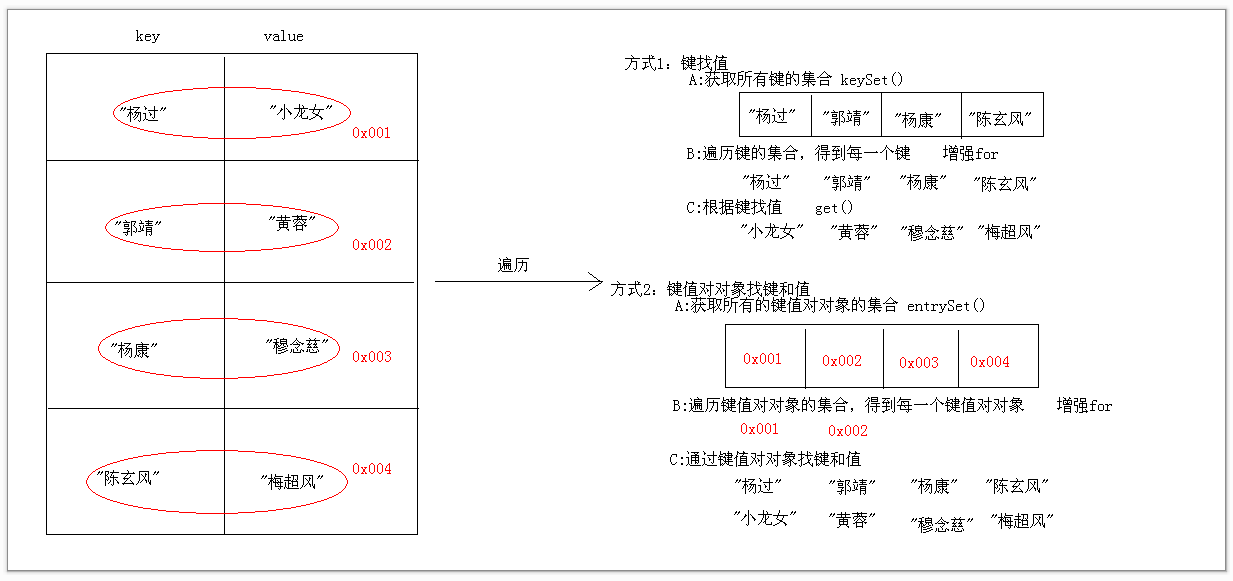
1.4 HashMap collection
HashMap is based on the implementation of the Map interface of the hash table (guaranteeing the uniqueness of keys), allowing null values and null keys (except for asynchronous and null allowed, the HashMap class is roughly the same as Hashtable).
HashMap does not guarantee the order of mappings, especially it does not guarantee that the order will remain constant.
import java.util.HashMap; import java.util.Set; /* * HashMap<String,Student> * Key: String number * Value: Student Student Student Object */ public class HashMapDemo3 { public static void main(String[] args) { // Creating Collection Objects HashMap<String, Student> hm = new HashMap<String, Student>(); // Creating Student Objects Student s1 = new Student("Stephen Chow", 58); Student s2 = new Student("Lau Andy", 55); Student s3 = new Student("Tony Leung", 54); Student s4 = new Student("Carina Lau", 50); // Additive elements hm.put("9527", s1); hm.put("9522", s2); hm.put("9524", s3); hm.put("9529", s4); // ergodic Set<String> set = hm.keySet(); for (String key : set) { Student value = hm.get(key); System.out.println(key + "---" + value.getName() + "---" + value.getAge()); } } }
Like HashSet, HashMap relies on hashCode() and equals() to ensure the uniqueness of keys. So you need to rewrite these two methods of custom classes as key values according to the situation.
import java.util.HashMap; import java.util.Set; /* * HashMap<Student,String> * Key: Student * Requirement: If the member variable values of both objects are the same, they are the same object. * Value: String */ public class HashMapDemo4 { public static void main(String[] args) { // Creating Collection Objects HashMap<Student, String> hm = new HashMap<Student, String>(); // Creating Student Objects Student s1 = new Student("army officer's hat ornaments", 27); Student s2 = new Student("Wang Zhaojun", 30); Student s3 = new Student("Xi Shi", 33); Student s4 = new Student("Yang Yuhuan", 35); Student s5 = new Student("army officer's hat ornaments", 27); // Additive elements hm.put(s1, "8888"); hm.put(s2, "6666"); hm.put(s3, "5555"); hm.put(s4, "7777"); hm.put(s5, "9999"); // ergodic Set<Student> set = hm.keySet(); for (Student key : set) { String value = hm.get(key); System.out.println(key.getName() + "---" + key.getAge() + "---" + value); } } } class Student { ... public int hashCode() {...} public boolean equals(Object obj) {...} } /*Operation results: Wang Zhaojun - 30 - 6666 Mink cicada - 27 - 9999 Yang Yuhuan - 35 - 7777 Xishi - 33 - 5555*/
1.5 LinkedHashMap collection
LinkedHashMap is a hash table and link list implementation of Map interface with predictable iteration order.
import java.util.LinkedHashMap; import java.util.Set; public class demo { public static void main(String[] args) { // Creating Collection Objects LinkedHashMap<String, String> hm = new LinkedHashMap<String, String>(); // Create and add elements hm.put("2345", "hello"); hm.put("1234", "world"); hm.put("3456", "java"); hm.put("1234", "javaee"); hm.put("3456", "android"); // ergodic Set<String> set = hm.keySet(); for (String key : set) { String value = hm.get(key); System.out.println(key + "---" + value); } } } /*Operation results: 2345---hello 1234---javaee 3456---android*/
1.6 TreeMap collection
TreeMap is based on Navigable Map of Red-Black tree. The mapping is sorted according to the natural order of its keys, or according to the Comparator provided when creating the mapping, depending on the construction method used.
Traversal implementation of natural sorting:
import java.util.Set; import java.util.TreeMap; public class TreeMapDemo { public static void main(String[] args) { // Creating Collection Objects TreeMap<String, String> tm = new TreeMap<String, String>(); // Create and add elements tm.put("hello", "Hello"); tm.put("world", "world"); tm.put("java", "Java"); tm.put("world", "World 2"); tm.put("javaee", "Java EE"); // Ergodic set Set<String> set = tm.keySet(); for (String key : set) { String value = tm.get(key); System.out.println(key + "---" + value); } } } /*Operation results: hello---Hello java---Java javaee---Java EE world---World 2*/
Comparator sort traversal implementation:
import java.util.Comparator; import java.util.Set; import java.util.TreeMap; public class TreeMapDemo2 { public static void main(String[] args) { // Creating Collection Objects TreeMap<Student, String> tm = new TreeMap<Student, String>( new Comparator<Student>() { @Override public int compare(Student s1, Student s2) { // Main conditions int num = s1.getAge() - s2.getAge(); // minor criteria int num2 = num == 0 ? s1.getName().compareTo( s2.getName()) : num; return num2; } }); // Creating Student Objects Student s1 = new Student("Pan an", 30); Student s2 = new Student("Liu Xia Hui", 35); Student s3 = new Student("Tang Pahu", 33); Student s4 = new Student("Yanqing", 32); Student s5 = new Student("Tang Pahu", 33); // Storage element tm.put(s1, "Song dynasty"); tm.put(s2, "Yuan Dynasty"); tm.put(s3, "The Ming Dynasty"); tm.put(s4, "Qing Dynasty"); tm.put(s5, "Han Dynasty"); // ergodic Set<Student> set = tm.keySet(); for (Student key : set) { String value = tm.get(key); System.out.println(key.getName() + "---" + key.getAge() + "---" + value); } } } /*Operation results: Pan'an-30--Song Dynasty Yanqing - 32 - Qing Dynasty Tang Bohu-33--Han Dynasty Liu Xiahui-35--Yuan Dynasty*/
1.7 Map Set Exercises
1. The difference between Hashtable and HashMap?
- Hashtable: Thread safe and inefficient. Null keys and null values are not allowed
- HashMap: Threads are insecure and efficient. Allow null keys and null values
import java.util.Hashtable; public class HashtableDemo { public static void main(String[] args) { Hashtable<String, String> hm = new Hashtable<String, String>(); hm.put("it001", "hello"); // hm.put(null, "world"); //Error-reporting NullPointerException // hm.put("java", null); //Error NullPointerException System.out.println(hm); } }
2. Do all interfaces such as List, Set, Map inherit sub-Map interfaces?
- List, Set s do not inherit from the Map interface, they inherit from the Collection interface
- The Map interface itself is a top-level interface
3. There is a string "aababcabcdabcde", which obtains the required result of the number of occurrences of each letter in the string: a(5)b(4)c(3)d(2)e(1).
Analysis:
- A: Define a string (which can be improved to keyboard entry)
- B: Define a TreeMap collection
- Key: Character
- Value: Integer
- C: Converting strings to character arrays
- D: Traverse the character array to get each character
- E: Take the character you just got as the key to find the value in the collection and see the return value.
- null: If the key does not exist, the character is used as the key and 1 is stored as the value.
- Not null: To indicate that the key exists, add the value to 1, and then rewrite to store the key and value
- F: Define string buffer variables
- G: Traverse the set, get keys and values, and splice them as required.
- H: Convert string buffer to string output
import java.util.Scanner; import java.util.Set; import java.util.TreeMap; public class TreeMapDemo { public static void main(String[] args) { // Define a string String line = "aababcabcdabcde"; // Define a TreeMap collection TreeMap<Character, Integer> tm = new TreeMap<Character, Integer>(); //Converting strings to character arrays char[] chs = line.toCharArray(); //Traverse the character array to get each character for(char ch : chs){ //Take the character you just got as the key to find the value in the collection and see the return value. Integer i = tm.get(ch); //null: If the key does not exist, the character is used as the key and 1 is stored as the value. if(i == null){ tm.put(ch, 1); }else { //Not null: To indicate that the key exists, add the value to 1, and then rewrite to store the key and value i++; tm.put(ch,i); } } //Define string buffer variables StringBuilder sb= new StringBuilder(); //Traverse the set, get keys and values, and splice them as required. Set<Character> set = tm.keySet(); for(Character key : set){ Integer value = tm.get(key); sb.append(key).append("(").append(value).append(")"); } //Converting String Buffer to String Output String result = sb.toString(); System.out.println("result:"+result); } }
4.HashMap Nested HashMap
import java.util.HashMap; import java.util.Set; public class HashMapDemo2 { public static void main(String[] args) { // Creating Collection Objects HashMap<String, HashMap<String, Integer>> czbkMap = new HashMap<String, HashMap<String, Integer>>(); // Creating the Basic Class Collection Object HashMap<String, Integer> jcMap = new HashMap<String, Integer>(); // Additive elements jcMap.put("Chen Yu Lou", 20); jcMap.put("Gao Yue", 22); // Adding basic classes to large collections czbkMap.put("jc", jcMap); // Creating Collective Objects of Employment Classes HashMap<String, Integer> jyMap = new HashMap<String, Integer>(); // Additive elements jyMap.put("Li Jie", 21); jyMap.put("Cao Shi Lei", 23); // Adding basic classes to large collections czbkMap.put("jy", jyMap); //Ergodic set Set<String> czbkMapSet = czbkMap.keySet(); for(String czbkMapKey : czbkMapSet){ System.out.println(czbkMapKey); HashMap<String, Integer> czbkMapValue = czbkMap.get(czbkMapKey); Set<String> czbkMapValueSet = czbkMapValue.keySet(); for(String czbkMapValueKey : czbkMapValueSet){ Integer czbkMapValueValue = czbkMapValue.get(czbkMapValueKey); System.out.println("\t"+czbkMapValueKey+"---"+czbkMapValueValue); } } } }
5.HashMap nested ArrayList
import java.util.ArrayList; import java.util.HashMap; import java.util.Set; public class HashMapIncludeArrayListDemo { public static void main(String[] args) { // Creating Collection Objects HashMap<String, ArrayList<String>> hm = new HashMap<String, ArrayList<String>>(); // Create element set 1 ArrayList<String> array1 = new ArrayList<String>(); array1.add("Lv Bu"); array1.add("Zhou Yu"); hm.put("Romance of the Three Kingdoms", array1); // Create element set 2 ArrayList<String> array2 = new ArrayList<String>(); array2.add("Linghu Chong"); array2.add("Lin Ping Zhi"); hm.put("Laughing and fighting", array2); // Create element set 3 ArrayList<String> array3 = new ArrayList<String>(); array3.add("Guo Jing"); array3.add("Yang Guo"); hm.put("Divine eagle", array3); //Ergodic set Set<String> set = hm.keySet(); for(String key : set){ System.out.println(key); ArrayList<String> value = hm.get(key); for(String s : value){ System.out.println("\t"+s); } } } }
6.ArrayList nested HashMap
import java.util.ArrayList; import java.util.HashMap; import java.util.Set; public class ArrayListIncludeHashMapDemo { public static void main(String[] args) { // Creating Collection Objects ArrayList<HashMap<String, String>> array = new ArrayList<HashMap<String, String>>(); // Create element 1 HashMap<String, String> hm1 = new HashMap<String, String>(); hm1.put("Zhou Yu", "Little Joe"); hm1.put("Lv Bu", "army officer's hat ornaments"); // Add elements to array array.add(hm1); // Create element 1 HashMap<String, String> hm2 = new HashMap<String, String>(); hm2.put("Guo Jing", "Huang Rong"); hm2.put("Yang Guo", "Little dragon maiden"); // Add elements to array array.add(hm2); // Create element 1 HashMap<String, String> hm3 = new HashMap<String, String>(); hm3.put("Linghu Chong", "Ren yingying"); hm3.put("Lin Ping Zhi", "Yue Ling Shan"); // Add elements to array array.add(hm3); // ergodic for (HashMap<String, String> hm : array) { Set<String> set = hm.keySet(); for (String key : set) { String value = hm.get(key); System.out.println(key + "---" + value); } } } }
2 Collections class
2.1 Overview of Collections Classes
Collections classes are tool classes that operate on collections, and their member methods are static methods.
What's the difference between Collection and Collections?
- Collection: is the top-level interface of a single-column collection, with sub-interfaces List and Set.
- Collections: A tool class for set operations, with methods for sorting and dichotomizing collections
2.2 Common methods
- Public static < T > void sort (List < T > list): Sorting is natural by default.
- Public static < T > int binary Search (List <?> list, T key): Binary lookup
- Public static < T > T Max (Collection <?> coll): maximum
- Public static void reverse (List <?> list): inversion
- Public static void shuffle (List <?> list): Random permutation
import java.util.Collections; import java.util.List; import java.util.ArrayList; public class CollectionsDemo { public static void main(String[] args) { // Creating Collection Objects List<Integer> list = new ArrayList<Integer>(); // Additive elements list.add(30); list.add(20); list.add(50); list.add(10); list.add(40); System.out.println("list:" + list); // Public static < T > void sort (List < T > list): Sorting is natural by default. // Collections.sort(list); // System.out.println("list:" + list); // [10, 20, 30, 40, 50] // Public static < T > int binary Search (List <?> list, T key): Binary lookup // System.out.println("binarySearch:" + Collections.binarySearch(list, 30)); // System.out.println("binarySearch:" + Collections.binarySearch(list, 300)); //-6 // Public static < T > T Max (Collection <?> coll): maximum // System.out.println("max:"+Collections.max(list)); // Public static void reverse (List <?> list): inversion // Collections.reverse(list); // System.out.println("list:" + list); //Public static void shuffle (List <?> list): Random permutation Collections.shuffle(list); System.out.println("list:" + list); } }
2.3 Collections exercises
1.Collections can sort elements of basic wrapper classes for ArrayList. Can storing custom objects be sorted?
- Sure. Natural Sort directly calls Collections.sort(), and comparator sort is implemented by passing anonymous inner classes as parameters into Collections.sort().
- If there are both natural sorting and comparator sorting, comparator sorting is the main method.
import java.util.ArrayList; import java.util.Collections; import java.util.Comparator; import java.util.List; public class CollectionsDemo { public static void main(String[] args) { // Creating Collection Objects List<Student> list = new ArrayList<Student>(); // Creating Student Objects Student s1 = new Student("Brigitte Lin", 27); Student s2 = new Student("Feng Qingyang", 30); Student s3 = new Student("Liu Xiao Qu", 28); Student s4 = new Student("Wu Xin", 29); // Adding Element Objects list.add(s1); list.add(s2); list.add(s3); list.add(s4); // Natural ordering // Collections.sort(list); // Comparator sorting (if there are both natural and comparator sorting, comparator sorting is the main) Collections.sort(list, new Comparator<Student>() { @Override public int compare(Student s1, Student s2) { int num = s2.getAge() - s1.getAge(); int num2 = num == 0 ? s1.getName().compareTo(s2.getName()) : num; return num2; } }); // Ergodic set for (Student s : list) { System.out.println(s.getName() + "---" + s.getAge()); } } }
2. Simulate the shuffling and licensing of Dou Landlord and sort the cards
Analysis:
- A: Create a HashMap collection
- B: Create an ArrayList collection
- C: Create colored arrays and point arrays
- D: Store the number in HashMap from 0 and the corresponding card, and store the number in ArrayList at the same time.
- E: shuffling (shuffling is number)
- F: Licensing (issuing is also a number, in order to ensure that the number is sorted, create a TreeSet collection to receive)
- G: Look at the cards (traverse the TreeSet collection, get the number, and go to the HashMap collection to find the corresponding cards)
import java.util.ArrayList; import java.util.Collections; import java.util.HashMap; import java.util.TreeSet; public class PokerDemo { public static void main(String[] args) { // Create a HashMap collection HashMap<Integer, String> hm = new HashMap<Integer, String>(); // Create an ArrayList collection ArrayList<Integer> array = new ArrayList<Integer>(); // Create colored arrays and point arrays // Define an array of colors String[] colors = { "♠", "♥", "♣", "♦" }; // Define an array of points String[] numbers = { "3", "4", "5", "6", "7", "8", "9", "10", "J", "Q", "K", "A", "2", }; // Store the number in HashMap from 0 and the corresponding card, and store the number in ArrayList at the same time. int index = 0; for (String number : numbers) { for (String color : colors) { String poker = color.concat(number); hm.put(index, poker); array.add(index); index++; } } hm.put(index, "Xiao Wang"); array.add(index); index++; hm.put(index, "king"); array.add(index); // Shuffle cards (shuffle numbers) Collections.shuffle(array); // Licensing (issuing is also a number, in order to ensure that the number is sorted, create a TreeSet collection to receive) TreeSet<Integer> fengQingYang = new TreeSet<Integer>(); TreeSet<Integer> linQingXia = new TreeSet<Integer>(); TreeSet<Integer> liuYi = new TreeSet<Integer>(); TreeSet<Integer> diPai = new TreeSet<Integer>(); for (int x = 0; x < array.size(); x++) { if (x >= array.size() - 3) { diPai.add(array.get(x)); } else if (x % 3 == 0) { fengQingYang.add(array.get(x)); } else if (x % 3 == 1) { linQingXia.add(array.get(x)); } else if (x % 3 == 2) { liuYi.add(array.get(x)); } } // Look at the cards (traverse the TreeSet collection, get the number, and go to the HashMap collection to find the corresponding cards) lookPoker("Feng Qingyang", fengQingYang, hm); lookPoker("Brigitte Lin", linQingXia, hm); lookPoker("Liu", liuYi, hm); lookPoker("A hand", diPai, hm); } // The Function of Writing and Reading Cards public static void lookPoker(String name, TreeSet<Integer> ts, HashMap<Integer, String> hm) { System.out.print(name + "The cards are:"); for (Integer key : ts) { String value = hm.get(key); System.out.print(value + " "); } System.out.println(); } }
3-set summary
3.1 Collection and Map
Collection
- List (ordered, repeatable)
- ArrayList: The underlying data structure is arrays, query fast, add or delete slowly, thread insecurity, high efficiency
- Vector: The underlying data structure is arrays, fast query, slow increment and deletion, thread safety, low efficiency
- LinkedList: The underlying data structure is linked list, which is slow to query, fast to add and delete, thread insecurity and high efficiency.
- Set (disorder, uniqueness)
- HashSet: The underlying data is a hash table, depending on hashCode() and equals() (automatically generated by IDE)
- Execution order:
- First, determine whether hashCode() is the same
- If so, continue to execute equals(). Return true to indicate that the element is repeated without adding; return false to add directly to the collection
- If not, add directly to the collection
- LinkedHashSet: The underlying data structure is linked list and hash table. The order of elements is guaranteed by linked list and the uniqueness of elements is guaranteed by hash table
- Execution order:
- TreeSet: The underlying data structure is a red-black tree (self-balanced binary tree)
- Guarantee the uniqueness of the element based on whether the return value of the comparison is 0 or not
- Sorting of elements
- Natural Sorting (Element Comparability): Allow the class to which the element belongs to implement the Comparable interface
- Comparator sorting (collections are comparable): Let collections accept a Comparator implementation class object
- HashSet: The underlying data is a hash table, depending on hashCode() and equals() (automatically generated by IDE)
Map (Double Column Set)
- A: The data structure of the Map set is valid only for keys, independent of values
- B: Map sets store elements in the form of key-value pairs, with unique keys and repeatable values.
- HashMap: The underlying data structure is a hash table. Threads are insecure and efficient
- Hash tables rely on hashCode() and equals() (can be generated automatically by IDE)
- Execution order:
- First, determine whether hashCode() is the same
- If so, continue to execute equals(). Return true to indicate that the element is repeated without adding; return false to add directly to the collection
- If not, add directly to the collection
- LinkedHashMap: The underlying data structure is linked list and hash table. The order of elements is guaranteed by linked list and the uniqueness of elements is guaranteed by hash table
- Hashtable: The underlying data structure is a hash table. Thread Safety and Low Efficiency
- Hash tables rely on hashCode() and equals() (can be generated automatically by IDE)
- Execution order:
- First, determine whether hashCode() is the same
- If so, continue to execute equals(). Return true to indicate that the element is repeated without adding; return false to add directly to the collection
- If not, add directly to the collection
- TreeMap: The underlying data structure is a red-black tree. (self-balanced binary tree)
+ Guarantee the uniqueness of the element based on whether the return value of the comparison is 0 or not
+ Sorting of elements
+ Natural Sorting (Element Comparability): Allow the class to which the element belongs to implement the Comparable interface
+ Comparator sorting (collections are comparable): Let collections accept a Comparator implementation class object
3.2 Collection Use Scenarios
- Is it in the form of a key object:?
- Yes, Map
- Do keys need to be sorted:
- Yes: TreeMap
- No: HashMap
- Don't know, just use HashMap
- Do keys need to be sorted:
- No: Collection
- Is the element unique:
- Yes, Set
- Does the element need to be sorted:
- Yes: TreeSet
- No: HashSet
- Don't know, just use HashSet
- Does the element need to be sorted:
- No: List
- Do you want to be safe?
- Yes, Vector
- No: ArrayList or LinkedList
- Add, delete and add: LinkedList
- Multiple queries: ArrayList
- If you don't know, use ArrayList
- If you don't know, use ArrayList
- Do you want to be safe?
- Yes, Set
- Is the element unique:
- Yes, Map
3.3 Common methods and traversal methods of sets
- Collection:
- add()
- remove()
- contains()
- iterator()
- size()
- Traversal:
- Enhanced for
- iterator
- List
- get()
- Traversal:
- Ordinary for
- Set
- Map:
- put()
- remove()
- containskey(),containsValue()
- keySet()
- get()
- value()
- entrySet()
- size()
- Traversal:
- Find values by key
- Find keys and values for objects based on key values