jQuery operation CSS
jQuery has several methods for CSS operations. We will learn the following:
addClass() - add one or more classes to the selected element
removeClass() - removes one or more classes from the selected element
toggleClass() - add / delete class switch for the selected element
css() - set or return style properties
Instance style sheet
.important
{
font-weight:bold;
font-size:xx-large;
}
.blue
{
color:blue;
}
jQuery addClass() method
The following example shows how to add a class attribute to different elements. Of course, when adding a class, you can also select multiple elements:
$("button").click(function(){
$("h1,h2,p").addClass("blue");
$("div").addClass("important");
});
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <script src="http://cdn.static.runoob.com/libs/jquery/1.10.2/jquery.min.js"> </script> <script> $(document).ready(function(){ $("button").click(function(){ $("h1,h2,p").addClass("blue"); $("div").addClass("important"); }); }); </script> <style type="text/css"> .important { font-weight:bold; font-size:xx-large; } .blue { color:blue; } </style> </head> <body> <h1>Title 1</h1> <h2>Title 2</h2> <p>This is a paragraph.</p> <p>This is another paragraph.</p> <div>Here are some important texts!</div> <br> <button>Add for element class</button> </body> </html>
Before operation
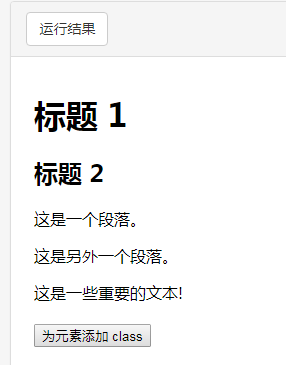
After operation
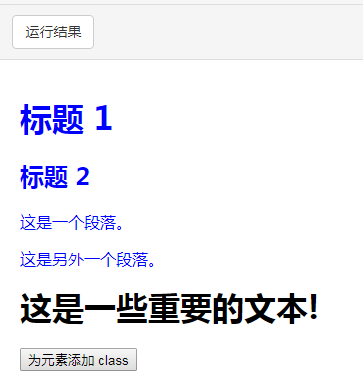
You can specify multiple classes in the addClass() method:
For example, add the "important blue" style at the same time
$("button").click(function(){
$("body div:first").addClass("important blue");
});
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <script src="http://cdn.bootcss.com/jquery/1.10.2/jquery.min.js"> </script> <script> $(document).ready(function(){ $("button").click(function(){ $("body div:first").addClass("important blue"); }); }); </script> <style type="text/css"> .important { font-weight:bold; font-size:xx-large; } .blue { color:blue; } </style> </head> <body> <div id="div1">Here are some texts.</div> <div id="div2">Here are some texts.</div> <br> <button>For the first div Element add class</button> </body> </html>
Before operation
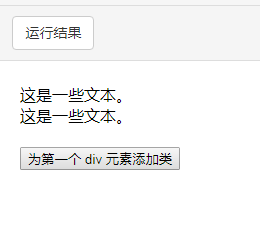
After operation
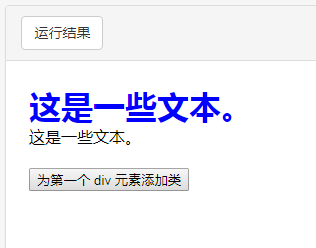
How to delete the specified class attribute in different elements:
Use jQuery removeClass()
$("button").click(function(){$("h1,h2,p").removeClass("blue");});
How to switch between adding and deleting classes for the selected elements
$("button").click(function(){
$("h1,h2,p").toggleClass("blue");
});