catalogue
1, Download and installation of Jquery
3, DOM object and Jquery wrapper set object
1. Attributes of operation elements
3. Contents of operation elements
(1). Append child element before
(2). Append child element after
(3). Append sibling element before
(4). Append sibling element after
JQuery is a multi browser compatible javascript script library. The core idea is to write less and do more. Using jQuery will greatly improve the efficiency of writing javascript code, help developers save a lot of work, and make the written code more elegant and robust. At the same time, the rich jQuery plug-ins on the network also make our work become "with jQuery, everything is so easy."
JQuery was released by American John Resig in barcamp in New York in January 2006, attracting many javascript experts from all over the world. It was developed by a team led by Dave Methvin. Today, jQuery has become the most popular javascript framework, with more than 55% of the world's top 10000 most visited websites using jQuery.
1, Download and installation of Jquery
1. Download and version
There are two versions of jQuery available for download:
- Production version - used in actual websites and has been streamlined and compressed.
- Development version - for testing and development (uncompressed, readable code)
Both versions are available from jQuery.com Download.
2. Installation
Introduce in the page:
<head> <script src="jquery.js"></script> </head>
If you don't want to download and store jQuery, you can also reference it through CDN (content distribution network).
Both Google and Microsoft have jQuery on their servers.
<head> <script src="http://ajax.googleapis.com/ajax/libs/jquery/1.8.0/jquery.min.js"> </script> </head>
Or:
<head> <script src="http://ajax.aspnetcdn.com/ajax/jQuery/jquery-1.8.0.js"> </script> </head>
3. Advantages
(1). Provides powerful functions
(2). Resolved browser compatibility issues
(3). Implement rich UI and plug-ins
(4). Script knowledge to correct errors
2, Jquery core
The $symbol represents a reference to the jQuery object in jQuery, and "jQuery" is the core object.
Through this object, you can get the jQuery object and call the methods provided by jQuery. Only jQuery objects can call the methods provided by jQuery.
3, DOM object and Jquery wrapper set object
The original DOM object only has the methods and properties provided by the DOM interface, and the objects obtained through JS code are DOM objects. The object obtained through jquery is the jquery wrapper set object, which is called jquery object for short. Only jquery objects can use the methods provided by jquery.
1.DOM object
Get DOM objects in JavaScript. DOM objects have only limited properties and methods:
let div = document.getElementById("testDiv") let div = document.getElementsByTagName("div")[0]
2.Jquery wrapper set object
It can be said to be an extension of DOM objects. In the world of jQuery, all objects, whether one or a group, are encapsulated into a jQuery wrapper set. For example, get a jQuery wrapper set containing elements:
let jqueryObject = $("#testDiv") let j = jqueryObject[0]
3.DOM object to jQuery object
To convert a DOM object into a jQuery object, you only need to wrap it with the $() method
let div = document.getElementById("testDiv") let jqueryDiv = $(div)
4.jQuery object to DOM object
To convert a jQuery object to a DOM object, you only need to take the array in the element
//The first way is to get the jQuery object let jqueryDiv = jQuery("#testDiv") //The second way is to get the jQuery object jqueryDiv = $("testDiv") //Convert the obtained jQuery object into a DOM object let dom = jqueryDiv[0]
The object obtained by traversing the jQuery object array is a DOM object, which can be converted into a jQuery object through $()
4, jQuery selector
Like using js to operate Dom, it is a very frequent operation to obtain node objects in documents. iQuery provides a simple way for us to find and locate elements, which is called jQuery Selector. Generally speaking, Selector selector is "a string representing special semantics". As long as the Selector string is passed into the above method, different Dom objects can be selected and returned in the form of jQuery wrapper set.
jQuery selectors are mainly divided into "selection" and "filtering" according to their functions. And it is used together. The specific classification is as follows.
1. Foundation selector
If there are multiple IDS with the same name in the id selector, the first one shall prevail
2. Hierarchy selector
3. Form selector
5, jQuery Dom operation
jQuery also provides the operation of HTML nodes, and is optimized on the basis of native JS, which is more convenient to use.
Commonly used to operate from several aspects to find elements (selector has been implemented); Create node objects; Access and set the values and properties of node objects; Add nodes; Delete node; Delete, add, modify and set the CSS style of the node. Note: the following operations are only applicable to jQuery objects.
1. Attributes of operation elements
(1). Get properties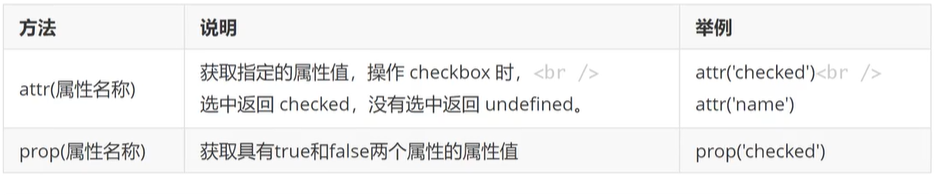
Classification of attributes:
- Inherent attributes: attributes inherent in the element itself (id, name, class, style)
- The return values are Boolean attributes: checked, selected, disabled
- Custom attribute: user defined attribute
The difference between attr and prop:
- If it is an intrinsic attribute, both attr() and prop() methods can get it
- If it is a custom attribute, attr() can get it, while prop() cannot get it
- If the return value is a Boolean attribute, if the attribute is set, attr() returns a specific value and prop() returns true; If the attribute is not set, attr() returns undefined and prop() returns false
<input type="checkbox" name="ch" checked="checked" id="aa" abc="aabbcc"/>aa <input type="checkbox" name="ch" id="bb">bb <script> //get attribute //Inherent attribute let name = $("#aa").attr("name") console.log(name); //ch let name2 = $("#aa").prop("name") console.log(name2); //ch //The return value is the attribute of Boolean (the element sets the attribute) let ck1 = $("#aa").attr("checked") console.log(ck1); //checked let ck2 = $("#aa").prop("checked") console.log(ck2); //true //The return value is the attribute of Boolean, (the element has no attribute set) let ck3 = $("#bb").attr("checked") console.log(ck3); //undefined let ck4 = $("#bb").prop("checked") console.log(ck4); //false //Custom properties let abc1 = $("#aa").attr("abc") console.log(abc1); let abc2 = $("#aa").prop("abc") console.log(abc2); </script>
(2). set a property
attr("attribute name", "attribute value")
prop("attribute name", "attribute value")
//Inherent attribute $("#aa").attr("value","1") $("#bb").attr("value","3") //The return value is a Boolean property $("#bb").attr("checked","checked") $("#bb").prop("checked",false) //Custom properties $("#aa").attr("uname","admin") //$("#aa"). Prop ("uage", 18) / / prop can't get custom attribute
(3). Remove Attribute
removeAttr("property name")
$("#aa").removeAttr("checked")
2. Style of operation element
css():
Set a single style: CSS (specific style name, style value)
Set multiple styles: CSS (specific style name): style value; specific style name: style value)
3. Contents of operation elements
html(), html("html content"), text(), text("text content") all operate on non form elements
html("html content") can be styled in the content;
Using text("text content") can only recognize the text content, and the label effect is not recognized
val(), val("value") operation form elements
Form elements:
text box, password box, radio box, checkbox, hidden field, textarea, drop-down box select
Non form elements:
div, span, h1~h6, table, tr, td, li, p, etc
4. Create element
Creating elements in jQuery is very simple. You can use the core function directly
//Create element let p = "<p>This is a P label</p>" console.log($(p));
5. Add elements
(1). Append child element before
Specifies the element Prepend (content) appends the first element inside the specified element. The content can be a string, html element or jQuery object
$(content) Prependto (specified element) appends the content to the front of the specified element content, which can be a string, html element or jQuery object
(2). Append child element after
Specifies the element Append (content) appends an element to the last side inside the specified element. The content can be a string, html element or jQuery object
$(content) Appendto (specified element) appends the content to the end of the specified element content, which can be a string, html element or jQuery object
(3). Append sibling element before
before() appends content before the specified element
(4). Append sibling element after
after() appends the specified element
Note:
When adding an element, if the element itself does not exist (the new element), the element will be appended to the specified location
If the element itself exists (existing element), the original element will be cut directly to the specified position
6. Delete element
Specifies the element remove()
Specifies the element empty()
7. Traverse elements
each()
$(selector).each(function(index,element)): traversing elements
Parameter function is the callback function during traversal
index is the sequence number of traversal elements, starting from 0.
Element is the current element, which is the dom element
6, jQuery event
1.ready() loading event
The ready() preload event is executed after the dom structure of the page is loaded, similar to the onLoad() event
ready() can write more than one and execute in order
Syntax: $(document) ready(function(){})
Abbreviation: $(function() {})
<head> <meta charset="UTF-8"> <title>Document</title> <script src="./jquery-3.6.0.js"></script> <script> $(function(){ console.log("ready Load event..."); }) $(document).ready(function(){ //Get element console.log($("#p1")); }) </script> </head> <body> <p id="p1">text</p> </body>
2.bind() binding event
(1). Bind single event
bind binding
$("element") bind("event type", function() {})
<body> <div id="test" style="cursor: pointer;">Click to view famous quotes</div> </body> <script> /*Bind single event*/ $("#test").bind("click",function(){ console.log("Nothing is difficult in the world"); }) </script>
Direct binding
$("element") Event name (function() {})
<body> <div id="test" style="cursor: pointer;">Click to view famous quotes</div> </body> <script> // Direct binding $("#test").click(function(){ console.log("Nothing is difficult in the world"); }) </script>
1. Determine which elements to bind events for
Get element
2. What event (event type) is the binding sound
First parameter: type of event
3. Actions triggered by corresponding events
Second parameter: function
(2). Bind multiple events
bind binding
1. Bind the same function for multiple events at the same time
Specifies the element bind("event type 1, event type 2...", function(){})
<body> <button type="button" id="btn1">Button 1</button> </body> <script> //Bind multiple events $("#btn1").bind("click mouseout",function(){ console.log("Button 1..."); }) </script>
2. Bind multiple events for the element and set corresponding functions
Specifies the element bind("event type", function()){
}). bind("event type", function()){
})
<body> <button type="button" id="btn2">Button 2</button> </body> <script> //2. Bind multiple events for the element and set corresponding functions $("#btn2").bind("click",function(){ console.log("Button 2 was clicked"); }).bind("mouseout",function(){ console.log("Button 2 is removed"); }) </script>
3. Bind multiple events for the element and set corresponding functions
Specifies the element bind({
"Event type": function() {},
"Event type": function() {}
})
<body> <button type="button" id="btn3">Button 3</button> </body> <script> //3. Bind multiple events for the element and set corresponding functions $("#btn3").bind({ "click":function(){ console.log("Button 3 was clicked"); }, "mouseout":function(){ console.log("Button 3 is removed"); } }) </script>
Direct binding
Specifies the element Event name (function){
}). Event name (function){
})
<body> <button type="button" id="btn4">Button 4</button> </body> <script> //Direct binding $("#btn4").click(function(){ console.log("Button 4 was clicked"); }).mouseout(function(){ console.log("Button 4 is removed"); }) </script>