Garbage collector and memory allocation strategy
Garbage collector and garbage collection algorithm
preface
I interviewed interns in April because I saw the JVM garbage collector and recycling algorithm in advance and answered this question. Ha ha, the interviewer directly told me that I could prepare three aspects. Today I want to review and share with you.
Various regions of Java runtime memory. For the three areas of program counter, virtual machine stack and local method stack, its life cycle is related to the relevant threads, which are born and died with the threads. And the memory allocation and recycling of these three areas are deterministic, because when the method ends or the thread ends, the memory will be recycled naturally with the thread. Memory allocation and recycling focus on Java heap and method area.
1, How to judge whether an object is "dead" (key)
1.1 reference counting method
The algorithm described by reference counting is: add a reference counter to the object. Whenever there is a place to reference it, the counter will be + 1; When the reference fails, the counter is - 1; The object whose counter is 0 at any time can no longer be used, that is, the object is "dead". The implementation of reference counting method is simple and the judgment efficiency is relatively high. It is a good algorithm in most cases. For example, Python language uses reference counting for memory management. However, in the mainstream JVM, reference counting method is not used to manage memory. The main reason is that reference counting method can not solve the problem of circular reference of objects
Example: observe the circular reference problem
public class Test { public Object instance = null; private static int _1MB = 1024 * 1024; private byte[] bigSize = new byte[2 * _1MB]; public static void testGC() { Test test1 = new Test(); Test test2 = new Test(); test1.instance = test2; test2.instance = test1; test1 = null; test2 = null; // Force jvm garbage collection System.gc(); 19 } public static void main(String[] args) { testGC(); 22 } }
The results are as follows:
[GC (System.gc()) 6092K->856K(125952K), 0.0007504 secs]
It can be seen from the results that the GC log contains "6092k - > 856k (125952k)", which means that the virtual machine does not recycle the two objects because they refer to each other. The JVM reference method is not used to determine whether the JVM is alive or not.
1.2 reachability analysis algorithm
As mentioned above, Java does not use the reference counting method to judge whether the object is "dead", but uses the "reachability analysis" to judge whether the object is alive (C#, Lisp - the earliest language using dynamic memory allocation).
The core idea of this algorithm is: starting from a series of objects called "GC Roots", search downward from these nodes, and the search path is called "reference chain". When an object is not connected to GC Roots (it is not reachable from GC Roots to this object), it is proved that this object is unavailable.
The following figure is an example:
Although objects Object5-Object7 are associated with each other, they are inaccessible to GC Roots, so they will be judged as recyclable objects.
In the Java language, objects that can be used as GC Roots include the following:
1. Objects referenced in virtual machine stack (local variable table in stack frame)
2. Objects referenced by class static attributes in the method area
3. Objects referenced by constants in the method area
4. Objects referenced by JNI(Native method) in the local method stack
In jdk1 2 before, the definition of reference in Java was very traditional: if the value stored in the data of reference type represents the starting address of another memory, it is said that this memory represents a reference. This definition is somewhat narrow. Under this definition, an object has only two states: referenced or not referenced.
We hope to describe this kind of object: when there is enough memory space, it can be saved in memory; If the memory space is still very tight after garbage collection, you can discard these objects. Cache objects in many systems fit this scenario.
In jdk1 After 2, Java expanded the concept of reference and divided it into four types: strong reference, soft reference, weak reference and phantom reference. The strength of these four types of references decreases in turn.
1. Strong reference: strong reference refers to a reference that is common in program code, such as "Object obj = new Object()". As long as the strong reference still exists, the garbage collector will never recycle the referenced object instance.
2. Soft reference: soft reference is used to describe some useful but not necessary objects. For objects associated with soft references, these objects will be included in the recycling scope for the second recycling before the system is about to overflow memory. If there is still not enough memory for this recovery, a memory overflow exception will be thrown. In jdk1 After 2, the SoftReference class is provided to implement soft reference.
3. Weak reference: weak reference is also used to describe non essential objects. But its strength is weaker than that of soft reference. Objects associated with weak references can only survive until the next garbage collection occurs. When the garbage collector starts working, it will recycle the objects associated with only weak references, regardless of whether the current content is sufficient or not. In jdk1 After 2, the WeakReference class is provided to implement weak reference.
4. Virtual reference: virtual reference is also called ghost reference or phantom reference. It is the weakest reference relationship. Whether an object has a virtual reference will not affect its lifetime at all, nor can it be used to obtain an object's real value through virtual reference
Example. The only purpose of setting a virtual reference for an object is to receive a system notification when the object is recycled by the collector. In jdk1 After 2, the phantom reference class is provided to implement the virtual reference.
Survival or death?
Even the unreachable objects in the reachability analysis algorithm are not "must die". At this time, they are temporarily in the "Probation" stage. To declare the true death of an object, it needs to go through the marking process at least twice: if the object finds no reference chain connected to GC Roots after accessibility analysis, it will be marked for the first time and filtered. The filter condition is whether it is necessary to execute the finalize() method. When the object does not cover the finalize() method or the finalize() method has been called by the JVM, the virtual opportunity regards both cases as "unnecessary execution", and the object at this time is the real "dead" object.
If the object is determined to be necessary to execute the finalize() method, the object will be placed in a queue called F-Queue and executed later by a low priority Finalizer thread automatically established by the virtual machine (the execution here refers to the virtual opportunity triggering the finalize() method). The finalize() method is the last chance for the object to escape death. Later, GC will mark the object in the F-Queue for a second time. If the object successfully saves itself in finalize() (it only needs to re establish an association with any object in the reference chain), it will be removed from the collection of "about to be recycled" at the second marking; If the object still doesn't escape at this time, it is basically recycled.
Example: object self rescue
public class Test { public static Test test; public void isAlive() { System.out.println("I am alive :)"); 5 } @Override protected void finalize() throws Throwable { super.finalize(); System.out.println("finalize method executed!"); test = this; 11 } public static void main(String[] args)throws Exception { test = new Test(); test = null; System.gc(); Thread.sleep(500); if (test != null) { test.isAlive(); }else { System.out.println("no,I am dead :("); 21 } // The following code is exactly the same as the above, but this self rescue failed test = null; System.gc(); Thread.sleep(500); if (test != null) { test.isAlive(); }else { System.out.println("no,I am dead :("); 30 } } }
From the above code example, we found that the finalize method was indeed triggered by the JVM, and the object escaped successfully before being collected.
But from the results, we found that two exactly the same code fragments, the result is a successful escape and a failure. This is because the finalize() method of any object will only be called automatically by the system once. If the same object faces a recycling after escaping once, its finalize() method will not be executed again. Therefore, the self rescue action of the second section of code fails.
II Recycling method area (key)
The garbage collection in the method area (permanent generation) mainly collects two parts: discarded constants and useless classes.
Recycling discarded constants is very similar to recycling objects in the Java heap. Take the recycling of literal quantity (direct quantity) in the constant pool as an example. If a String "abc" has entered the constant pool, but no String object in the current system references the "abc" constant of the constant pool, and this literal quantity is not referenced elsewhere, if GC occurs at this time and it is necessary, the "abc" constant will be cleared out by the system
Pool. The symbolic references of other classes (interfaces), methods and fields in the constant pool are similar.
Determining whether a class is a "useless class" is much more complex. A class needs to meet the following three conditions at the same time before it can be regarded as a "useless class":
1. All instances of this class have been recycled (that is, there are no instances of this class in the Java heap)
2. The ClassLoader that loads this class has been recycled
3. The Class object corresponding to this Class is not referenced anywhere else, and the method of this Class cannot be accessed anywhere through reflection
The JVM can recycle useless classes that meet the above three conditions at the same time, which is only "yes" rather than inevitable. In scenarios where reflection and dynamic proxy are widely used, the JVM needs to have the function of class unloading to prevent the overflow of permanent generation.
III Garbage collection algorithm (key)
3.1 mark clear algorithm
The "mark clear" algorithm is the most basic collection algorithm. The algorithm is divided into "marking" and "clearing" stages: firstly, mark all objects to be recycled, and uniformly recycle all marked objects after marking (see Chapter 3.1.2 for the marking process). The subsequent collection algorithms are based on this idea and improve its shortcomings.
There are two main shortcomings of the "mark clear" algorithm:
1. Efficiency: the efficiency of marking and removal is not high
2. Space problem: after the mark is cleared, a large number of discontinuous memory fragments will be generated. Too many space fragments may lead to the inability to find enough continuous memory and have to trigger another garbage collection in advance when large objects need to be allocated during program operation.
3.2 replication algorithm (new generation recycling algorithm)
The "copy" algorithm is to solve the efficiency problem of "mark clean". It divides the available memory into two equal sized blocks according to its capacity, and only one of them is used at a time. When this memory needs garbage collection, the objects still alive in this area will be copied to another one, and then the used memory area will be cleaned up at one time. The advantage of this is that the memory of the whole half area is recycled every time. When allocating memory, there is no need to consider complex situations such as memory fragments. You only need to move the pointer on the top of the heap and allocate it in order. This algorithm is simple and efficient. The execution flow of the algorithm is as follows:
Current commercial virtual machines (including HotSpot use this collection algorithm to recycle the new generation)
98% of the objects in the Cenozoic era are "living and dying", so it is not necessary to divide the memory space according to the ratio of 1:1. Instead, the memory (Cenozoic memory) is divided into a larger Eden space and two smaller survivor spaces. Eden and one of them are used every time
One Survivor (two Survivor areas, one is called From area and the other is called To area). When recycling, copy the surviving objects in Eden and Survivor To another Survivor space at one time, and finally clean up Eden and the Survivor space just used.
When the Survivor space is not enough, it needs to rely on other memory (old age) for allocation guarantee.
The default size ratio of Eden to Survivor in HotSpot is 8:1, which means Eden: Survivor from: Survivor to = 8:1:1. Therefore, the available memory space of each new generation is 90% of the capacity of the whole new generation, and the remaining 10% is used to store the objects that survive after recycling.
The replication algorithm process implemented by HotSpot is as follows:
1. When Eden area is full, Minor gc will be triggered for the first time To copy the living objects To Survivor From area; When miner GC is triggered again in Eden area, Eden area and From area will be scanned and garbage collected in the two areas. Objects that survive this collection will be directly copied To to area and Eden and From areas will be cleared.
2. When Minor gc occurs again in Eden, the Eden and To areas will be garbage collected, the surviving objects will be copied To the From area, and the Eden and To areas will be emptied.
3. Some objects will be copied back and forth in the From and To areas, and exchanged 15 times (determined by the JVM parameters)
MaxTenuringThreshold determines that this parameter is 15 by default), and if it still survives, it will be saved to the old age
3.3 marking sorting algorithm (older generation recycling algorithm)
The replication collection algorithm will perform more replication operations when the object survival rate is high, and the efficiency will become low. Therefore, the replication algorithm can not be used in the elderly generation.
According to the characteristics of the elderly generation, a new algorithm called "tag sorting algorithm" is proposed. The marking process is still the same as the "mark clear" process, but the subsequent steps are not to directly clean up recyclable objects, but to move all living objects to one end, and then directly clean up the memory outside the end boundary.
The flow chart is as follows:
3.4 generation collection algorithm
At present, JVM garbage collection adopts the "generational collection" algorithm. This algorithm has no new idea, but divides the memory into several blocks according to the different life cycle of the object.
Generally, Java heap is divided into new generation and old generation. In the new generation, a large number of objects die in each garbage collection, and only a few survive. Therefore, we use the replication algorithm; In the elderly generation, the object has high survival rate and there is no additional space to allocate and guarantee it, so the "mark clean" or "mark tidy" algorithm must be adopted.
Interview question: do you know about Minor GC and Full GC? Is there any difference between these two GCS
1.Minor GC is also called Cenozoic GC: it refers to the garbage collection in Cenozoic. Because most Java objects have the characteristics of being born in the morning and dying in the evening, minor GC (using replication algorithm) is very frequent, and generally the recycling speed is relatively fast.
2.Full GC, also known as older generation GC or major GC: refers to garbage collection in the old age. Major GC appears, often accompanied by Minor GC at least once (not absolutely, there is a direct policy selection process of full GC in the Parallel Scavenge collector). Major GC is generally 10 times slower than Minor GC.
IV garbage collector
If the collection algorithm we mentioned above is the methodology of memory recycling, then the garbage collector is the specific implementation of memory recycling. The collector described below is based on jdk1 For the HotSpot virtual machine behind the G1 collector of 7, all collectors contained in this JVM are shown in the following figure:
The figure above shows seven kinds of collectors acting on different generations. If there is a connection between the two collectors, they can be used together. The area indicates whether it belongs to the new generation collector or the old generation collector. Before talking about specific collectors, let's clarify three concepts:
Parallel: multiple garbage collection threads work in parallel, and the user thread is still waiting
Concurrent: it means that the user thread and the garbage collection thread execute at the same time (not necessarily in parallel, but may execute alternately). The user program continues to run, while the garbage collection program is on another CPU.
Throughput: it is the ratio of the CPU time used to run user code to the total CPU consumption time.
Throughput running user code time running user code time garbage collection time
For example, the virtual machine runs for 100 minutes in total, and garbage collection takes 1 minute, so the throughput is 99%.
4.1 serial collector (new generation collector, serial GC)
Serial collector is the most basic and oldest collector. It was once (before JDK 1.3.1) the only choice for the new generation collection of virtual machines.
characteristic:
This collector is a single threaded collector, but its meaning of "single thread" does not only mean that it will only use one CPU or one collection thread to complete garbage collection. More importantly, when it collects garbage, it must suspend all other work lines until it stops the world
Application scenario:
Serial collector is the default new generation collector for virtual machines running in Client mode.
Advantages:
For the single thread garbage collector, it is more efficient than other single thread garbage collectors, because it has no single thread interaction. In fact, so far: it is still the default new generation collector for virtual machines running in Client mode
4.2 parnew collector (new generation collector, parallel GC)
ParNew collector is actually a multi-threaded version of Serial collector. In addition to using multiple threads for garbage collection, other behaviors include all control parameters available to Serial collector, collection algorithm, Stop The World, object allocation rules, recycling strategy, etc., which are exactly the same as Serial collector. In terms of implementation, these two collectors also share a lot of code.
characteristic:
Multithreaded version of Serial collector
Application scenario:
ParNew collector is the preferred new generation collector among many virtual machines running in Server mode.
As the preferred collector of Server, there is a very important reason that has nothing to do with performance: except for the Serial collector, at present, only it can work with the CMS collector.
During JDK 1.5, HotSpot launched a garbage collector that can almost be regarded as epoch-making in strong interactive applications - CMS collector. This collector is the first real concurrent collector in HotSpot virtual machine. It realizes the garbage collection line and user thread working at the same time for the first time.
Unfortunately, as the collector of the older generation, CMS cannot work with the new generation collector Parallel Scavenge that already exists in JDK 1.4.0. Therefore, when using CMS to collect the old age in JDK 1.5, the new generation can only choose one of the ParNew or Serial collectors.
Comparative analysis:
Compared with Serial collector:
The ParNew collector will never have a better effect than the Serial collector in a single CPU environment. Even due to the overhead of thread interaction, the collector can not be guaranteed to surpass the Serial collector in a two CPU environment implemented by hyper threading technology.
However, with the increase of the number of CPU s that can be used, it is still very beneficial for the effective utilization of system resources during GC.
4.3Parallel Scavenge collector (new generation collector, parallel GC)
characteristic:
Parallel Scavenge collector is a new generation collector. It is also a collector using replication algorithm and a parallel multithreaded collector.
The Parallel Scavenge collector uses two parameters to control throughput:
20: Maxgcpausemillis controls the maximum garbage collection pause time
20: Gcratio sets the throughput directly
Intuitively, as long as the maximum garbage collection pause time is smaller, the throughput is higher, but GC The reduction of pause time is at the expense of throughput and Cenozoic space. For example, the original 10 second collection, with a pause of 100 milliseconds, has now become a 5-second collection, with a pause of 70 milliseconds. While the pause time decreases, the throughput also decreases. Application scenario: The shorter the pause time, the more suitable it is for programs that need to interact with users. Good response speed can improve the user experience, and high throughput can be used efficiently CPU Time, complete the operation task of the program as soon as possible, which is mainly suitable for the operation in the background without too many interactive tasks. Comparative analysis: Parallel Scavenge collector VS CMS Equal collector: Parallel Scavenge The characteristic of collector is that its focus is different from other collectors, CMS The focus of the wait collector is to minimize the pause time of user threads during garbage collection Parallel Scavenge The throughput of the collector is a goal that can be controlled( Throughput). Because it is closely related to throughput, Parallel Scavenge Collectors are also often referred to as "throughput first" collectors. Parallel Scavenge collector VS ParNew Collector: Parallel Scavenge Collector and ParNew An important difference between collectors is that they have an adaptive adjustment strategy. GC Adaptive adjustment strategy: Parallel Scavenge The collector has one parameter- XX:+UseAdaptiveSizePolicy . When this parameter is turned on, there is no need to manually specify the size of the new generation Eden And Survivor The proportion of the area, the age of the object promoted to the old age and other detailed parameters. The virtual opportunity collects performance monitoring information according to the operation of the current system, and dynamically adjusts these parameters to provide the most appropriate pause time or maximum throughput. This adjustment method is called GC Adaptive adjustment strategy( GC Ergonomics). Note: the work flow chart is the same as ParNew ## 4.4Serial Old collector (older generation collector, serial GC) characteristic: Serial Old yes Serial The older version of the collector, which is also a single threaded collector, uses tags-Sorting algorithm. Application scenario: Client pattern Serial Old The main significance of the collector is also to Client Virtual machine usage in mode. Server pattern If in Server In mode, it has two main uses: one is in JDK 1.5 And previous versions Parallel Scavenge The collector is used together, and another use is as CMS The backup plan of the collector occurs during concurrent collection Concurrent Mode Failure Used when. 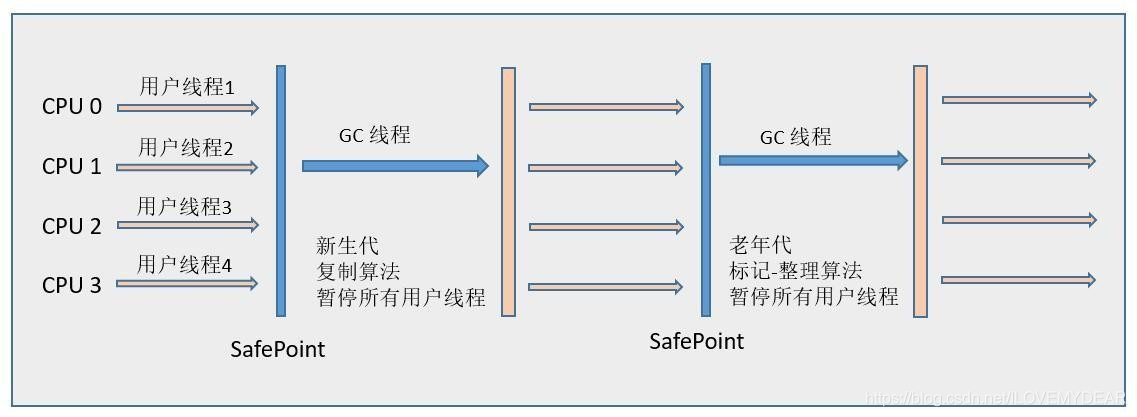 ## 4.5 parallel old collector (older generation collector, parallel GC) characteristic: Parallel Old yes Parallel Scavenge An older version of the collector, using multithreading and "tagging"-Sorting algorithm. Application scenario: Focus on throughput and CPU Priority can be given to resource sensitive occasions Parallel Scavenge plus Parallel Old Collector. This collector is in JDK 1.6 China began to provide, before that, the Cenozoic Parallel Scavenge The collector has been in an awkward state. The reason is that if the Cenozoic chooses Parallel Scavenge Collector, older generation Serial Old There is no alternative to the collector (Parallel Scavenge The collector cannot communicate with CMS The collector works together). Due to the old generation Serial Old The "drag" of the collector on the application performance of the server uses Parallel Scavenge The collector may not be able to maximize the throughput in the overall application. Due to the single thread collection, it can not make full use of many servers CPU In the environment where the older generation is large and the hardware is relatively advanced, the throughput of this combination does not even have to be improved ParNew plus CMS The combination of "awesome". until Parallel Old After the collector appears, "The "throughput first" collector finally has a more veritable application portfolio. 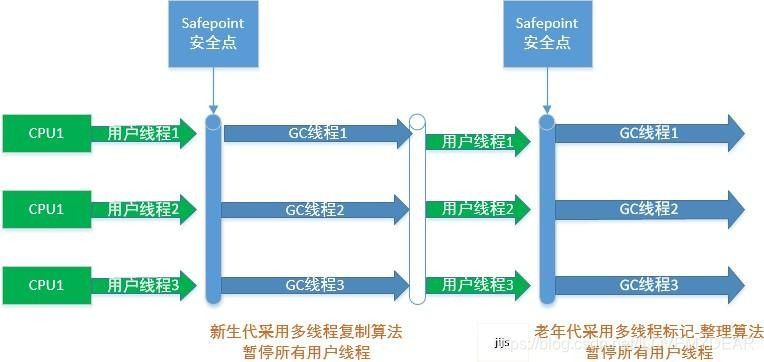 ## 4.6CMS collector (older generation collector, concurrent GC) characteristic: CMS(Concurrent Mark Sweep)A collector is a collector that aims to obtain the shortest recovery pause time. At present, a large part of Java Applications focus on Internet sites or B/S On the server side of the system, such applications pay special attention to the response speed of the service, hoping that the system pause time will be the shortest, so as to bring better experience to users. CMS The collector is very suitable for this kind of application. CMS The collector is implemented based on the "mark clear" algorithm. Its operation process is more complex than the previous collectors. The whole process is divided into four steps: Initial marking( CMS initial mark) The initial mark is just a mark GC Roots Objects that can be directly related to are fast and need“ Stop The World". Concurrent marking( CMS concurrent mark) The concurrent marking phase is GC Roots Tracing The process of. Relabel( CMS remark) The re marking stage is to correct the marking record of the part of the object whose marking changes due to the continuous operation of the user program during concurrent marking. The pause time of this stage is generally slightly longer than that of the initial marking stage, but it is much shorter than that of concurrent marking, so it is still necessary“ Stop The World". Concurrent cleanup( CMS concurrent sweep) The concurrent purge phase purges objects. Since the collector threads of the most time-consuming concurrent marking and concurrent clearing process in the whole process can work with user threads, in general, CMS The memory reclamation process of the collector is executed concurrently with the user thread. advantage: CMS It is an excellent collector. Its main advantages have been reflected in its name: concurrent collection and low pause. Disadvantages: CMS Collector pair CPU Resources are very sensitive In fact, concurrent design oriented programs are right CPU Resource sensitive. In the concurrency phase, although it will not cause the user thread to pause, it will occupy part of the thread (or CPU Resources), which slows down the application and reduces the total throughput. CMS The number of recycle threads started by default is( CPU quantity+3)/ 4,That is, when CPU When there are more than 4, the garbage collection thread shall not be less than 25 during concurrent recycling%of CPU Resources, and with CPU Decrease with the increase of quantity. But when CPU Less than 4 (For example, when there are two, CMS The impact on user programs may become great. CMS The collector cannot process floating garbage CMS The collector is unable to process floating garbage and may“ Concurrent Mode Failure"Failure leads to another Full GC Generation of. because CMS In the concurrent cleanup phase, the user thread is still running. With the program running, new garbage will continue to be generated. This part of garbage appears after the marking process, CMS They can't be disposed of in the current collection, so they have to wait for the next one GC Clean it up when necessary. This part of garbage is called "floating garbage". It is also because the user thread still needs to run in the garbage collection stage, so enough memory space needs to be reserved for the user thread CMS Like other collectors, collectors cannot wait until the old age is almost completely filled before collecting. They need to reserve some space for program operation during concurrent collection. If CMS This occurs when the memory reserved during operation cannot meet the needs of the program“ Concurrent Mode Failure"Failed, then the virtual machine will start the backup plan: temporarily enabled Serial Old The collector is used to re collect the old garbage, so the pause time is very long. CMS The collector generates a lot of space debris CMS It is a collector based on the "mark clear" algorithm, which means that a large number of space debris will be generated at the end of the collection. When there is too much space debris, it will bring great trouble to the allocation of large objects. There is often a lot of space left in the old age, but it is unable to find enough continuous space to allocate the current object, so it has to be triggered in advance Full GC. 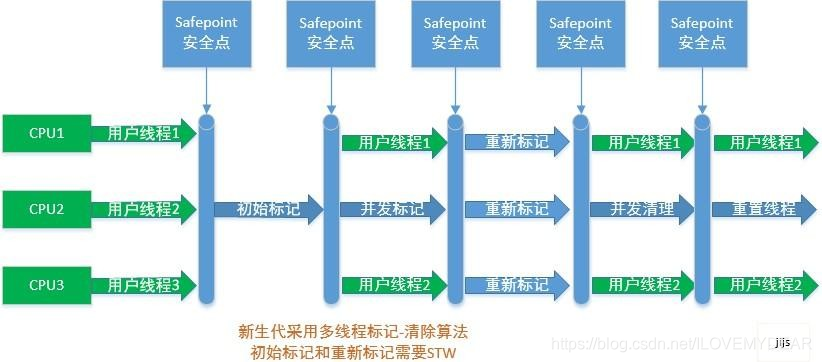 ## 3.7 G1 collector (the only garbage collector in the whole area) G1(Garbage First)Garbage collector is used in heap memory In most cases, put heap Divided into many, many region Block, and then garbage collect it in parallel. G1 After clearing the memory space occupied by the instance, the garbage collector will also compress the memory. G1 Garbage collector recycling region Not at all STW,But based on most garbage Priority recycling(Overall, it is based on"sign-arrangement"Algorithm, from local(Two region between)be based on"copy"algorithm) Strategy for region For garbage collection. in any case, G1 The algorithm used by the collector means The results are as follows: 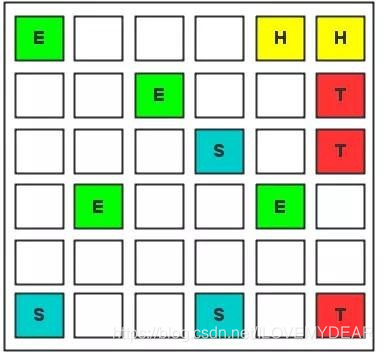 One region May belong to Eden,Survivor perhaps Tenured Memory area. In the figure E Indicates that region belong to Eden Memory area Domain, S Indicates belonging Survivor Memory area, T Indicates belonging Tenured Memory area. The blank in the figure indicates unused memory space. G1 The garbage collector also adds a new memory area called Humongous Memory area, as shown in the figure H Block. This memory area is mainly used to store large objects-That is, the size exceeds one region Size 50%Object. ### Young generation garbage collection stay G1 In the garbage collector, the younger generation garbage collection process uses the replication algorithm. hold Eden District and Survivor Copy objects from the area to the new Survivor Area. As shown below: 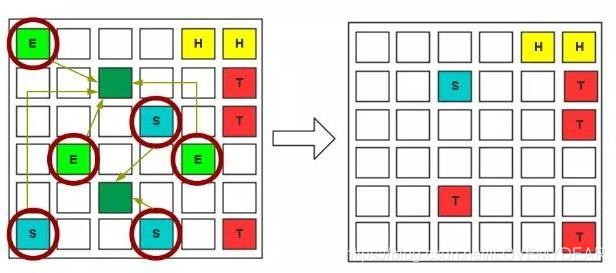 ### Elderly garbage collection For the garbage collection of the elderly generation, G1 The garbage collector is also divided into four stages, basically the same as CMS The garbage collector is the same, but slightly different: Initial marking(Initial Mark)stage - with CMS Of garbage collector Initial Mark The same stage, G1 You also need to pause the execution of the application. It will mark all reachable objects in the first child node of the root object starting from the root object. however G1 Of the garbage collector Initial Mark Phase is with minor gc Together. That is, in G1 You don't have to be like CMS In that way, the execution of the application is suspended separately to run Initial Mark Stage, but in G1 trigger minor gc The old generation will be replaced at the same time Initial Mark I did. Concurrent marking(Concurrent Mark)stage - At this stage G1 Do things with CMS Same. but G1 At the same time, I also did one more thing, that is, if Concurrent Mark What were found during the phase Tenured region If the survival rate of objects in is very small or almost no objects survive, then G1 It will be recycled at this stage without waiting for later clean up Phase. This too Garbage First The origin of the name. meanwhile,At this stage, G1 Accounting for each region The survival rate of the object is convenient for the later clean up Phase use. Final marking(CMS Medium Remark stage) - At this stage G1 Do things with CMS equally, But the algorithm is different, G1 Using a method called SATB(snapshot-at-the-begining)Our algorithm can Remark Phase faster marking Reachable object. Filter recycling(Clean up/Copy)stage - stay G1 Yes, No CMS Corresponding in Sweep Phase. Instead, it has one Clean up/Copy Stage, in this stage,G1 Those who have a low survival rate will be selected region For recycling, this stage is also the same as minor gc Co occurring,As shown in the figure below: 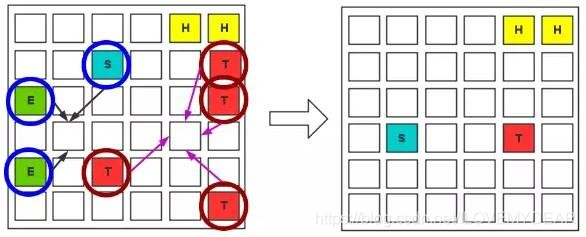 G1(Garbage-First)It is a garbage collector for server-side applications. HotSpot The mission given to it by the development team is that it can be replaced in the future JDK 1.5 Published in CMS Collector. If your app pursues low pauses, G1 Can be used as an option; If your application pursues throughput, G1 It does not bring particularly obvious benefits. # summary <font color=#999aaa > tip: here is a summary of the article: For example, the above is what we will talk about today. This article only briefly introduces it pandas Use of, and pandas It provides a large number of functions and methods that enable us to process data quickly and conveniently.