Object oriented has three characteristics: Encapsulation, Inheritance and Polymorphism.
This section covers encapsulation.
Procedure 1
class Woman { private String name; private int age; private Man husband; public void setName(String name) { this.name = name; } public String getName() { return name; } public void setAge(int age) { this.age = age; } public int getAge() { return age; } public void setHusband(Man husband) { this.husband = husband; } public Man getHusband() { return husband; } } public class Man { private String name; private int age; private Woman wife; public void setName(String Name) { this.name = Name; } public String getName() { return this.name; } public void setAge(int Age) { this.age = Age; } public int getAge() { return this.age; } public void setWife(Woman wife) { this.wife = wife; } } class Test { public static void main(String[] args) { Woman w = new Woman(); w.setName("Han Meimei"); w.setAge(25); System.out.println("My name is " + w.getName()); System.out.println("My Age is " + w.getAge()); Man m = new Man(); m.setName("Li Lei"); m.setAge(26); m.setWife(w); w.setHusband(m); System.out.println("My husband is " + w.getHusband().getName()); System.out.println(); System.out.println("My name is " + m.getName()); System.out.println("My Age is " + m.getAge()); System.out.println("You don't know whether I have wife or not!"); } }
Operation results:
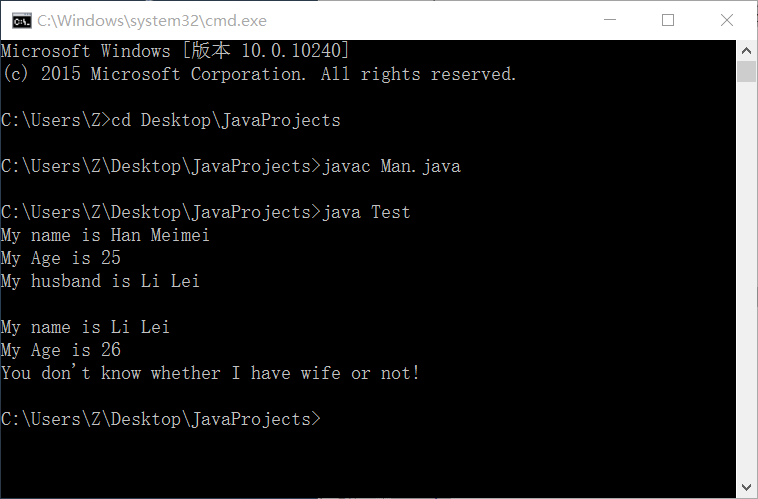
analysis:
Because Li Lei doesn't want others to visit his wife, he encapsulates the wire and does not provide the getWife() method.
(children may laugh when they see it)
Procedure 2
public class J { public static void printf(String str) { System.out.println(str); } public static void cout(String str) { System.out.println(str); } } class JTest { public static void main(String[] args) { J.printf("Hello World!"); J.cout("Hello World!"); } }
Operation results:
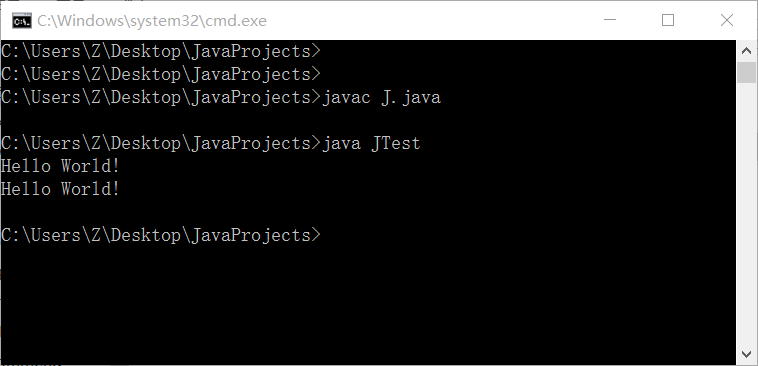
analysis:
Some children may think that printf in C language and cout in C + + are better than those in java System.out.println It should be convenient. So here we also encapsulate printf and cout to achieve the purpose of printing.
(children should be very happy to see here)
Of course, this is just an example. If you really write java code, it is recommended to use the default provided by Java System.out.println .