Kotlin knowledge points Summary - Basic
1, Data type
1.1 definition of basic data type
val anInt: Int = 8 val aLong: Long = 12368172397127391 val aFloat: Float = 2.0F val aDouble: Double = 3.0 val aShort: Short = 127 val maxByte: Byte = Byte.MAX_VALUE //Delay initializing lateinit without assignment lateinit var s:String
1.2 template operator
val arg1: Int = 0 val arg2: Int = 1 val charArray:CharArray= charArrayOf('a','b') println("" + arg1 + " + " + arg2 + " = " + (arg1 + arg2)) println("$arg1 + $arg2 = ${arg1 + arg2}") //Operation results //0 + 1 = 1 //0 + 1 = 1 val rawString: String = """ ${charArray[0]} \t \n \\\\\\$$$ salary """ println(rawString) //Run results (the following is the print of the template operator. No / / comment is added here) Hello "Trump" $salary a \t \n \\\\\\$$$ salary
1.3 class and object initialization
//1. When there is only one constructor, the constructor keyword can be omitted open class MyClass (name:String, sex:String): Any() { //2. The first constructor calls init method by default, and the rest are not called init { println(this.javaClass.name+"Construct method call name:$name sex:$sex") } } class MyClass1(name:String,sex:String):MyClass(name,sex) fun main() { val myClass=MyClass1("zhanglei","male") println(myClass is MyClass1) } //Return results //com.hongshi.test.MyClass1 constructor call name:zhanglei sex: Male //true
1.4. Empty type and smart type conversion
Null type (any type can be null or non null)
val notNull:String = null//Error, cannot be empty val nullable:String?= null//Correct, can be empty notNull.length//Correct. Values that are not empty can be used directly by customers nullable.length//Error, may be empty, unable to get the length directly nullable!!.length//Correct, mandatory nullable cannot be null nullable?.length//Correct. If nullable is null, null is returned
//Null in kotlin does not belong to the basic data type. Add "? After the data type Allow null return fun getName():String?{ return null } fun main() { val name= getName() //type1 if (name!=null){ println(name.length) } println(name?.length)//Blank writing //type2: if (name==null){ return } println(name.length) val name= getName() ?: return//Empty trinocular expression }
Type conversion
//as type (child parent) //as? Safe type forced conversion, conversion failure returns null val child: Child? = parent as? Child
Intelligent type conversion (compiler derivation, away from useless type conversion)
open class Parent() open class Child():Parent(){ fun getString(){ } } fun main(args: Array<String>) { val parent: Parent = Parent() if (parent is Child){ //Only type conversions are allowed parent.getString() } }
as new import package can be alias
import com.hongshi.test2.Test2 as Test2
2, Array
2.1 definition method
val arrayOfInt: IntArray = intArrayOf(1,3,5,7) val arrayOfChar: CharArray = charArrayOf('H', 'e','l','l','o','W','o','r','l','d') val arrayOfString: Array<String> = arrayOf("I", "yes", "Code farmer") fun main(args: Array<String>) { println(arrayOfChar) println(arrayOfChar.joinToString("")) println(arrayOfChar.joinToString()) println(arrayOfInt.slice(1..3)) //In addition, the array size println(arrayOfInt.size) //Array changes the specified value arrayOfInt[1]=111 //Array traversal for(item in arrayOfInt){ println(item) } } //Operation results //HelloWorld //HelloWorld //H, e, l, l, o, W, o, r, l, d //[3, 5, 7]
Comparison of assignment between customized data and ordinary data
val helloWorldArray: Array<Char> = arrayOf('H', 'e', 'l', 'l', 'o', 'W', 'o', 'r', 'l', 'd') val helloWorldCharArray: CharArray = charArrayOf('H', 'e', 'l', 'l', 'o', 'W', 'o', 'r', 'l', 'd')
There are 8 arrays of basic data types (excluding the array customized by the wrapper class)
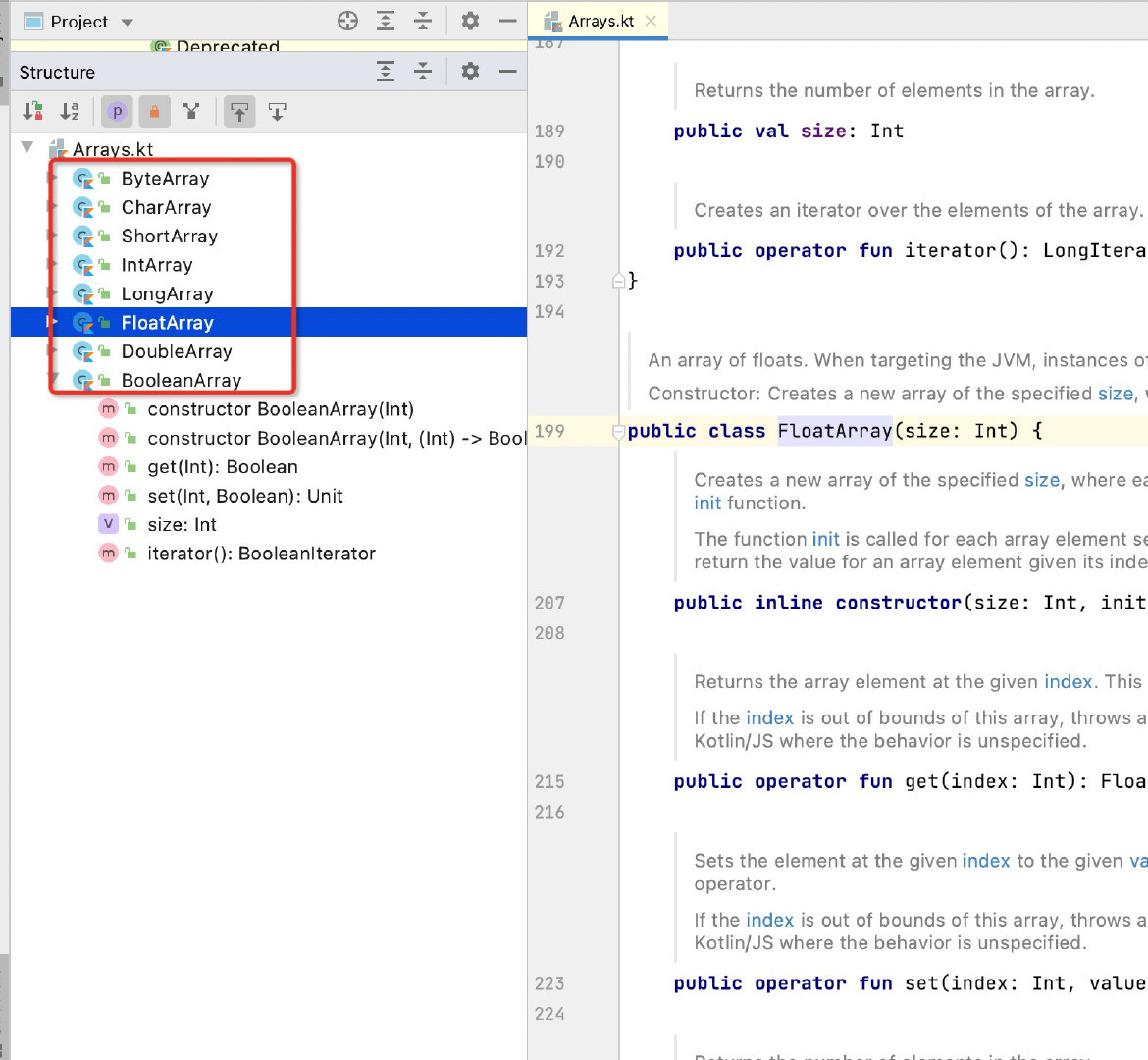
2.2 array processing method
2.2.1 slice usage
val str:String="HelloWorld" fun main() { println(str.slice(0..1))//[0,1] println(str.slice(0 until 1))//[0,1) } //Return results //He //H
2.2.2. String receive string array initialization
val helloWorldCharArray: CharArray = charArrayOf('H', 'e', 'l', 'l', 'o', 'W', 'o', 'r', 'l', 'd') fun main() { println(String(helloWorldCharArray)) } //Return results //HelloWorld
2.2.3 traversal
val arrayOfInt: IntArray = intArrayOf(1,3,5,7) //Type1: for each loop for (item in arrayOfInt){ println(item) } //type2:array extension method forEach loop arrayOfInt.forEach { println(it) }
What's in forEach is actually a lambda expression, Click to jump to the advanced writing method of lambda expression
3, Program structure
3.1 constants and variables (val,var)
Runtime constant
val str
Compiler constant (equivalent to java final)
const val str
3.2 function
3.2.1 basic writing method
//The default return value is Unit, which is equivalent to java void fun main(args: Array<String>) { //Basic data type conversion val arg1 = args[0].toInt() val arg2 = args[1].toInt() println("$arg1 + $arg2 = ${sum(arg1, arg2)}") } //Return value: Int fun sum(arg1: Int, arg2: Int): Int{ return arg1 + arg2 //Return result: 1 + 2 = 3 }
The input parameters of the main function can be entered here or through the command line
kotlin com.TestKt.kt 1 2
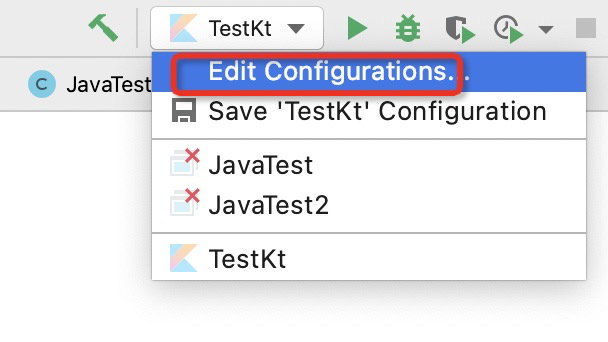
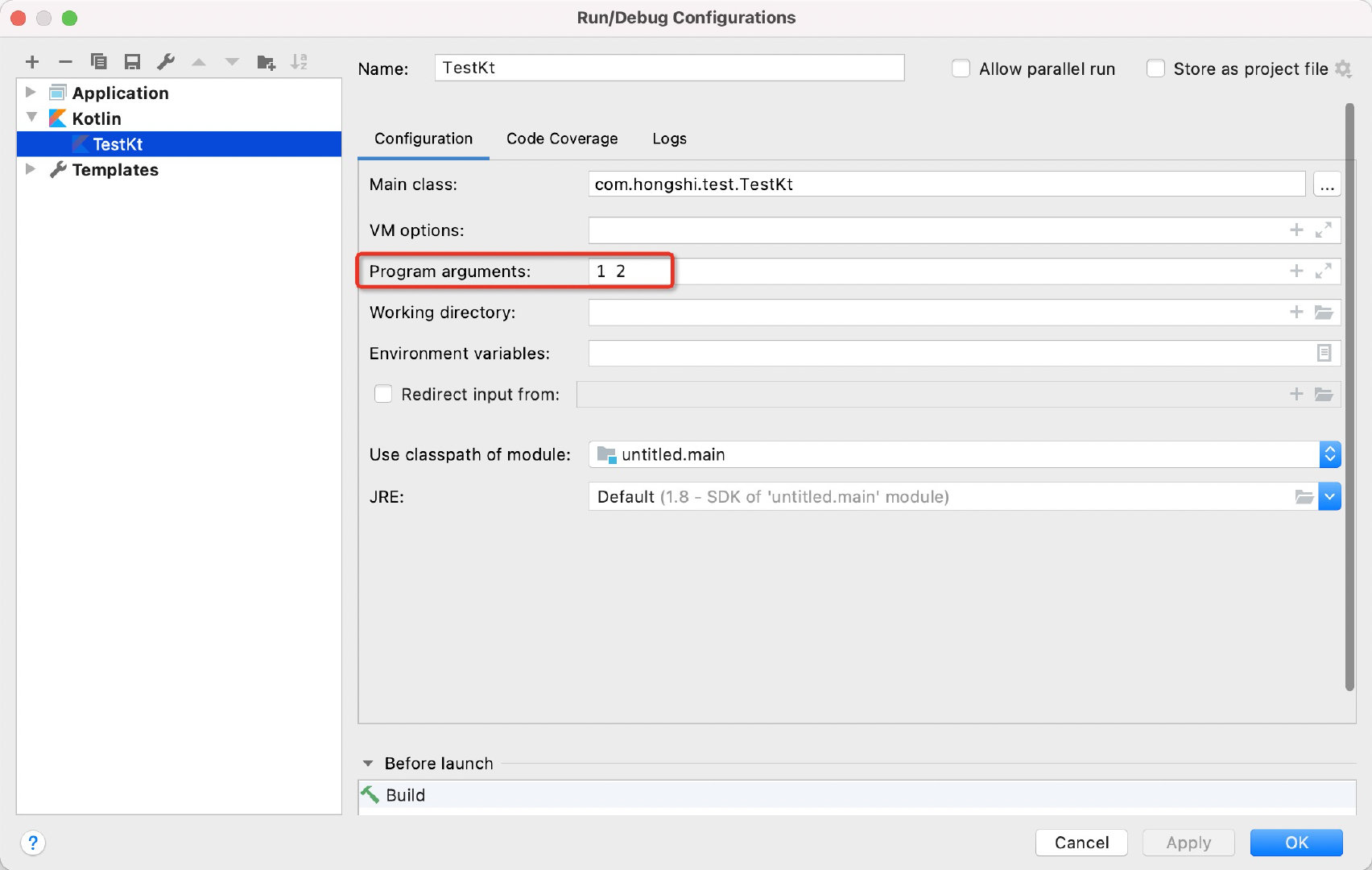
3.2.2 simplified and anonymous functions
//The sum function is abbreviated as fun sum(a:Int,b:Int)=a+b
//Anonymous function, similar to function pointer in c var sum=fun(a:Int,b:Int)=a+b //If you return the Unit object, you can write the execution statement directly after the equal sign var print=fun(a:Int,b:Int)=printf("asdf")
3.2.3 lambda expression
lambda is an anonymous function
Writing method {[parameter list] - [function body, and the last line is the return value]}
3.2.3.1 common writing
//Common writing var sum={a:Int,b:Int->a+b} //Writing without input parameters var printHello={ println("printHello") } //Writing method with return value (as shown in the figure below) var sum={ println("printHello") 1+2 } //Return results //printHello //3
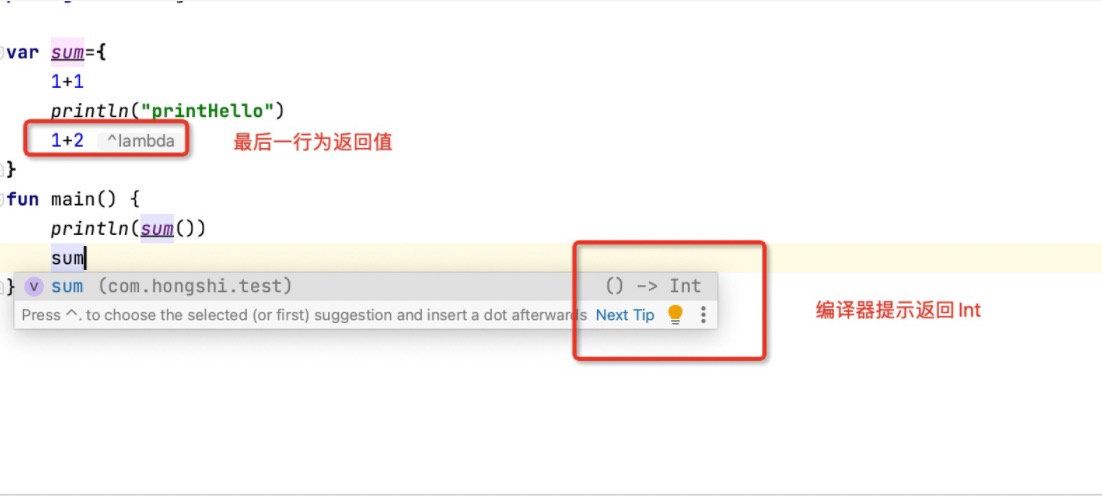
The complete writing method of parameter and return value brought in by lambda expression
val sum = { arg1: Int, arg2: Int -> println("$arg1 + $arg2 = ${arg1 + arg2}") arg1 + arg2 } fun main(args: Array<String>) { // (Array<String>) -> Unit println(sum(1, 3)) //Here, calling () is equivalent to calling the invoke method. Invoke method: the operator in kotlin is overloaded as a method println(sum.invoke(1, 3)) }
3.2.3.2 simplification
lambda expressions can default to it as long as one parameter
val arrayOfInt: IntArray = intArrayOf(1,3,5,7) arrayOfInt.forEach { println(it) } //If there is only one parameter passed in for each, you can not write it and use it instead //You can also add parentheses, which is essentially the function body of a lambda expression arrayOfInt.forEach ({ println(it) })
- Step 1: complete writing of foreach
arrayOfInt.forEach ({ element-> println(element) })
- step 2: add parentheses
Scala: want to solve the problem of insufficient expression ability of Java
Groovy: want to solve the problem that Java syntax is too verbose
Clojure: I want to solve the problem that Java does not have functional programming
Kotlin: want to solve Java
arrayOfInt.forEach (){ println(it) }
- step 3: delete parentheses
idea prompt: parentheses are useless and can be deleted
arrayOfInt.forEach { println(it) }
- step 4: final writing: reference
idea prompts "Convert lambda to reference"
The println function name is passed to forEach as a parameter, and println receives Any? Arbitrary parameter
prinln source code:
@kotlin.internal.InlineOnly public actual inline fun println(message: Any?) { System.out.println(message) }
forEach source code:
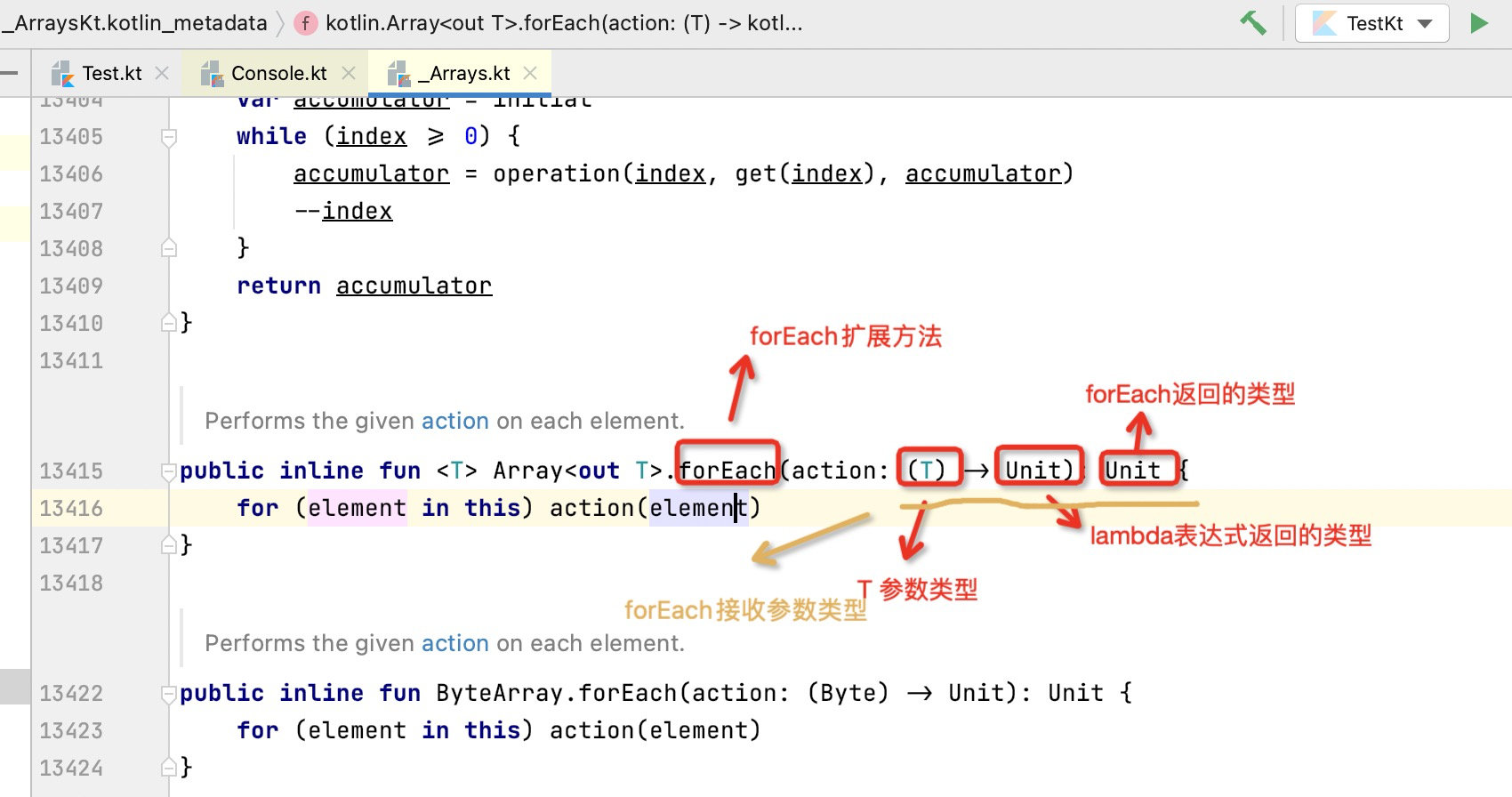
forEach receives parameter T, and Any is equivalent to java Object, which is the parent or indirect parent of Any parameter. println uses the parameter T of forEach through reference.
Functions whose input parameters and return values are consistent with formal parameters can be passed in as arguments by function reference. The final wording is as follows
arrayOfInt.forEach(::println)
3.2.3.3. forEach exit cycle
forEach receives a lambda expression. If it returns directly, it will cause the return of the whole main method
fun main() { val arrayOfInt: IntArray = intArrayOf(1,3,5,7) arrayOfInt.forEach { if (it==5){ return } println(it) } println("end") } //Return result (the lambda expression return cannot be executed to println ("end"), and the lambda function will not) //1 //3
Solution, label foreach @, return@ForEach
Note: ForEach name can be taken arbitrarily
fun main() { val arrayOfInt: IntArray = intArrayOf(1,3,5,7) arrayOfInt.forEach asdf@{ if (it==5){ return@asdf } println(it) } println("end") }
3.2.4 function type
fun main() { //No parameter, return Unit: () - > Unit var printUsage=fun()= println("asdf") println(printUsage) //Return result: function0 < kotlin Unit> //No parameter, return an integer: () - > int var sum0=fun()=1 println(sum0) //Return result: function0 < Java lang.Integer> //Pass in an integer and return an integer: (int) - > int var sum1=fun(a:Int)=a println(sum1) //Return result: function1 < Java lang.Integer, java. lang.Integer> //Pass in two integers and return an integer: (int, int) - > int var sum2=fun(a:Int,b:Int)=a println(sum2) //Return result: function2 < Java lang.Integer, java. lang.Integer, java. lang.Integer> //Incoming string, Lambda expression, return Boolean: (string, (string) - > string) - > Boolean var sum3=fun(a:String,b:(String)->String)=true println(sum3) //Return result: function2 < Java lang.String, kotlin. jvm. functions. Function1<? super java. lang.String, ? extends java. lang.String>, java. lang.Boolean> //===========Summary: invoke has several parameters called Function //Named function sum type (int, int) - > int fun sum(arg1: Int, arg2: Int): Int{ return arg1 + arg2 //Return result: 1 + 2 = 3 } //Reference to function reference println(::sum) //Return result: function sum (Kotlin reflection is not available). Reflection cannot be used. Use the is keyword to judge println(::sum is (Int,Int)->Int) //Return result: true }
3.3 class members
3.3.1 difference between function and method
In languages such as C++, functions are bits of code that will perform a particular action - but are not associated with an object. functions that are to do with an object are called methods. in java all functions are methods as they are all to do with objects.
Method is located on the object.
Functions are object independent.
For Java, there are only methods.
For C, there are only functions.
For C + +, it depends on whether you are in a class
The summary is in one sentence: the method inside the class and the function outside the class