- 1. Spring overview
- 1. Core container
- 2. Data access / integration
- 3.Web
- 4. Other modules
- Spring download and directory structure
- 2. Spring's core container
- 3. Introduction to spring
- 4. Dependency injection
- Using setter method to implement dependency injection
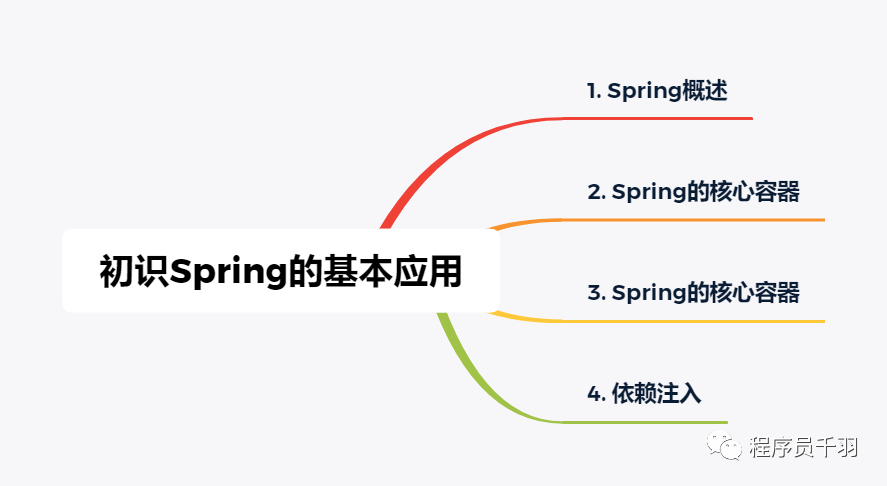
"https://gitee.com/nateshao/ssm/tree/master/100-spring-hello
1. Spring overview
What is Spring?
"Spring is a lightweight open source framework, which takes IoC and AOP aspect oriented programming as the kernel and uses basic JavaBean s to complete the work previously only possible by EJB s.
Three tier architecture
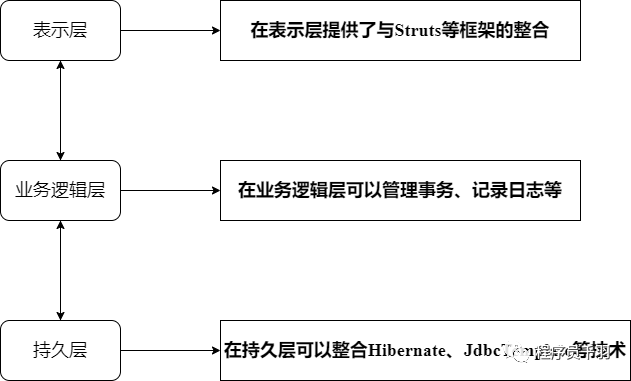
7 advantages of Spring framework
- Non intrusive design
- Convenient decoupling and simplified development
- Support AOP
- Support declarative transactions
- Convenient program testing
- Convenient integration of various excellent frameworks
- Reduce the difficulty of using Java EE API
Spring architecture
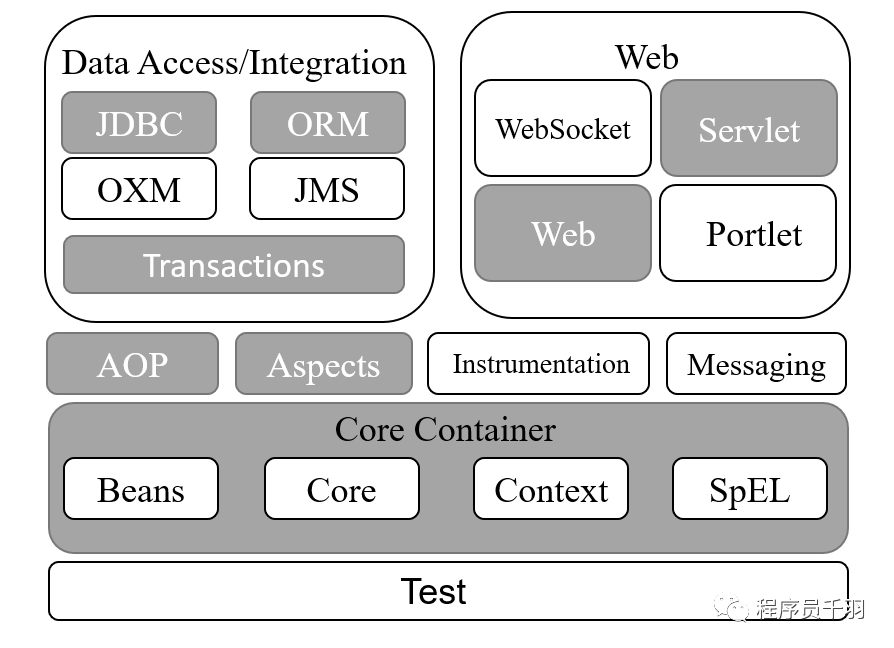
1. Core container
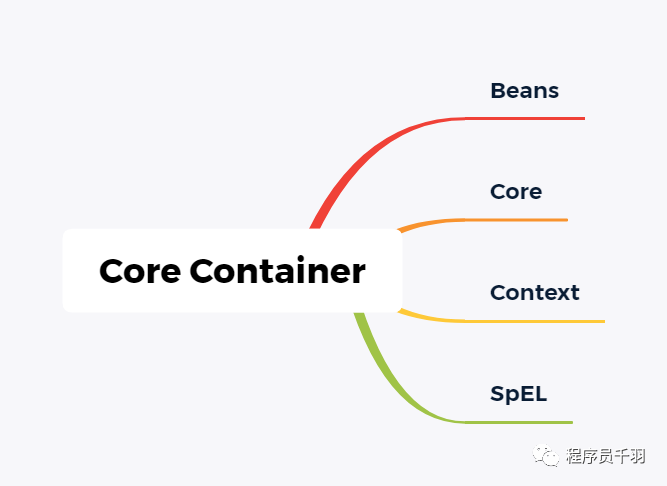
2. Data access / integration
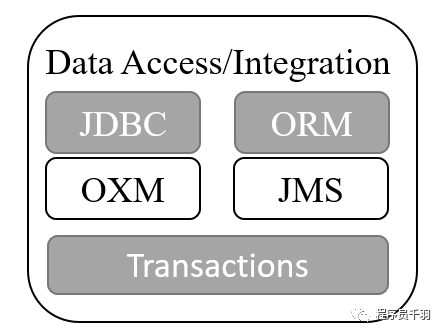
JDBC: it provides an abstract layer of JDBC, which greatly reduces the coding of database operations in the development process. ORM: provides integration layer support for popular object relational mapping API s, including JPA, JDO and Hibernate. 0XM: provides an abstract layer implementation that supports object / XML Mapping, such as JAXB, Castor, XML Beans, JiBX, and XStream. JMS: refers to the Java messaging service, including the features of using and generating information. Since version 4.1, it supports the integration with the spring message module. Transactions: support programming harmony for implementing special interfaces and all POJO classes Ming style transaction management.
3.Web
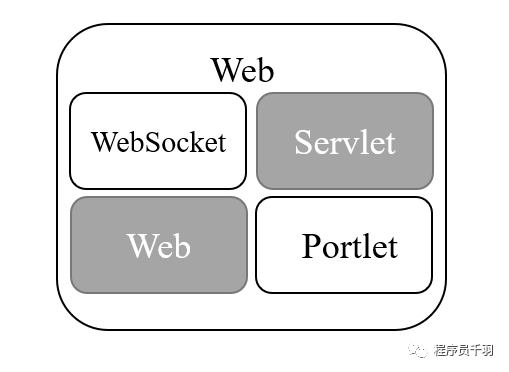
WebSocket: Spring4.0, which provides the implementation of websocket and SockJS, as well as the support for STOMP. Servlet: also known as spring webmvc module, it contains Web programs implemented by spring model view controller (MVC) and REST Web Services. Web: it provides basic web development integration features, such as multi file upload, using servlet listener to initialize IoC container and web application context. Portlet: it provides MVC implementation in portlet environment, similar to the function of servlet module.
4. Other modules
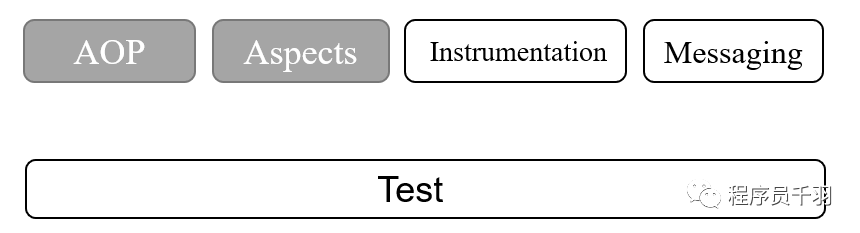
AOP: it provides an aspect oriented programming implementation, which allows to define method interceptors and pointcuts, and separate the code according to functions to reduce coupling.
Aspects: provides integration with AspectJ, a powerful and mature aspect oriented programming (AOP) framework.
Instrumentation: it provides the support of class tools and the implementation of class loader, which can be used in a specific application server.
Messaging: Sring4.0, which provides support for messaging architecture and protocols.
Test: provides support for unit testing and integration testing.
Spring download and directory structure
The jar package required for spring development is divided into two parts: Spring Framework package and third-party dependency package.
Download address: http://repo.spring.io/simple/libs-release-local/org/springframework/spring/4.3.6.RELEASE/
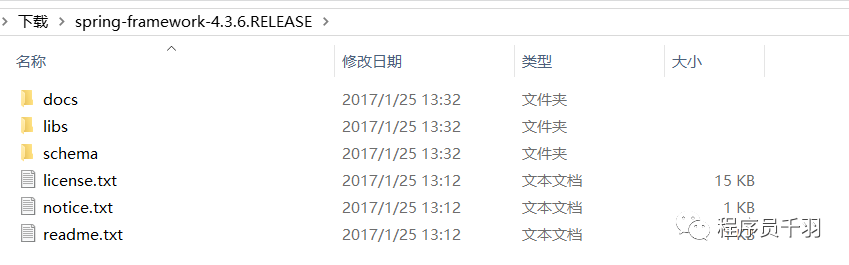
The docs folder contains API documents and development specifications
The libs folder contains JAR packages and source code
The schema folder contains the schema files required for development
Open the libs directory to see 60 JAR files, as follows:
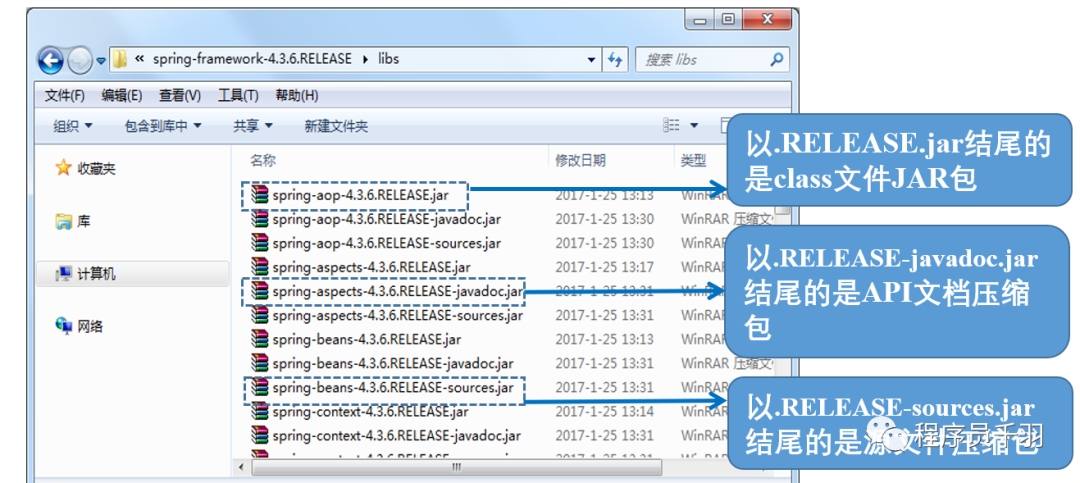
There are four Spring basic packages in the libs directory, which correspond to the four modules of the Spring core container.
- spring-core-4.3.6.RELEASE.jar It contains the core tool classes of the Spring framework. Other Spring components need to use the classes in this package.
- spring-beans-4.3.6.RELEASE.jar The JAR package used by all applications contains all classes related to accessing configuration files, creating and managing beans, and performing control inversion or dependency injection operations.
- spring-context-4.3.6.RELEASE.jar It provides extended services on basic IoC functions and supports many enterprise level services
- spring-expression-4.3.6.RELEASE.jar Defines the expression language of Spring.
2. Third party dependency package
When developing with Spring, in addition to using its own JAR package, the core container of Spring also needs to rely on Commons JAR package for logging.
Download address: http://commons.apache.org/proper/commons-logging/download_logging.cgi
2. Spring's core container
"Overview: Spring container is responsible for controlling the relationship between programs, not directly controlled by program code. Spring provides us with two core containers, BeanFactory and ApplicationContext, which will be briefly introduced in this section.
When creating a BeanFactory instance, you need to provide detailed configuration information of the containers managed by Spring. This information is usually managed in the form of XML files. The syntax for loading configuration information is as follows:
BeanFactory beanFactory = new XmlBeanFactory(new FileSystemResource("F:/applicationContext.xml"));
Tip: this loading method is not widely used in actual development. Readers can understand it.
ApplicationContext is a sub interface of BeanFactory and another commonly used Spring core container. It is organized by org springframework. context. The ApplicationContext interface definition not only includes all functions of BeanFactory, but also adds support for internationalization, resource access, event propagation, etc. Two methods are usually used to create an ApplicationContext interface instance, as follows:
1. Create through ClassPathXmlApplicationContext
ApplicationContext applicationContext = new ClassPathXmlApplicationContext(String configLocation);
ClassPathXmlApplicationContext will find the specified XML configuration file from the classPath, find and load it, and complete the instantiation of ApplicationContext.
2. Create through FileSystemXmlApplicationContext
ApplicationContext applicationContext = new FileSystemXmlApplicationContext(String configLocation);
FileSystemXmlApplicationContext will find the specified XML configuration file from the specified file system path (absolute path), find and load it, and complete the instantiation of ApplicationContext.
In the Java project, the ApplicationContext container will be instantiated through the ClassPathXmlApplicationContext class. In the Web project, the instantiation of the ApplicationContext container will be completed by the Web server.
When the Web server instantiates the ApplicationContext container, it usually uses the ContextLoaderListener. This method only needs to be implemented on the Web Add the following code to XML:
<context-param> <param-name>contextConfigLocation</param-name> <param-value> classpath:spring/applicationContext.xml </param-value> </context-param> <listener> <listener-class> org.springframework.web.context.ContextLoaderListener </listener-class> </listener>
After creating the spring container, you can get the beans in the spring container. Spring usually uses the following two methods to obtain Bean instances:
Object getBean(String name); According to the container Bean of id or name To get the specified Bean,Cast is required after obtaining. <T> T getBean(Class<T> requiredType); Get according to the type of class Bean Examples of. Since this method is generic, the Bean No cast is required after that.
3. Introduction to spring
In IEDA, create a Web project named 100 Spring Hello, copy the four basic packages of Spring and the JAR package of Commons logging to the lib directory and publish them to the classpath.
pom.xml
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.nateshao</groupId> <artifactId>100-spring-hello</artifactId> <version>1.0-SNAPSHOT</version> <name>100-spring-hello</name> <!-- FIXME change it to the project's website --> <url>http://www.example.com</url> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <maven.compiler.source>1.8</maven.compiler.source> <maven.compiler.target>1.8</maven.compiler.target> </properties> <dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.11</version> <scope>test</scope> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>5.3.8</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-beans</artifactId> <version>5.3.8</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-core</artifactId> <version>5.3.8</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-expression</artifactId> <version>5.3.8</version> </dependency> <dependency> <groupId>commons-logging</groupId> <artifactId>commons-logging</artifactId> <version>1.2</version> </dependency> </dependencies> <build> <pluginManagement><!-- lock down plugins versions to avoid using Maven defaults (may be moved to parent pom) --> <plugins> <!-- clean lifecycle, see https://maven.apache.org/ref/current/maven-core/lifecycles.html#clean_Lifecycle --> <plugin> <artifactId>maven-clean-plugin</artifactId> <version>3.1.0</version> </plugin> <!-- default lifecycle, jar packaging: see https://maven.apache.org/ref/current/maven-core/default-bindings.html#Plugin_bindings_for_jar_packaging --> <plugin> <artifactId>maven-resources-plugin</artifactId> <version>3.0.2</version> </plugin> <plugin> <artifactId>maven-compiler-plugin</artifactId> <version>3.8.0</version> </plugin> <plugin> <artifactId>maven-surefire-plugin</artifactId> <version>2.22.1</version> </plugin> <plugin> <artifactId>maven-jar-plugin</artifactId> <version>3.0.2</version> </plugin> <plugin> <artifactId>maven-install-plugin</artifactId> <version>2.5.2</version> </plugin> <plugin> <artifactId>maven-deploy-plugin</artifactId> <version>2.8.2</version> </plugin> <!-- site lifecycle, see https://maven.apache.org/ref/current/maven-core/lifecycles.html#site_Lifecycle --> <plugin> <artifactId>maven-site-plugin</artifactId> <version>3.7.1</version> </plugin> <plugin> <artifactId>maven-project-info-reports-plugin</artifactId> <version>3.0.0</version> </plugin> </plugins> </pluginManagement> </build> </project>
In the main/java directory, create a com nateshao. IOC package, create the interface UserDao in the package, and then define a say() method in the interface.
package com.nateshao.ioc; /** * @date Created by Shao Tongjie on 2021/10/13 20:58 * @WeChat official account programmers * @Personal website www.nateshao.com cn * @Blog https://nateshao.gitee.io * @GitHub https://github.com/nateshao * @Gitee https://gitee.com/nateshao * Description: */ public class UserDaoImpl implements UserDao { public void say() { System.out.println("userDao say hello World !"); } }
On COM nateshao. Under the IOC package, create the implementation class UserDaoImpl of the UserDao interface. This class needs to implement the say() method in the interface and write an output statement in the method.
package com.nateshao.ioc; /** * @date Created by Shao Tongjie on 2021/10/13 20:58 * @WeChat official account programmers * @Personal website www.nateshao.com cn * @Blog https://nateshao.gitee.io * @GitHub https://github.com/nateshao * @Gitee https://gitee.com/nateshao * Description: */ public class UserDaoImpl implements UserDao { public void say() { System.out.println("userDao say hello World !"); } }
In the main/resources directory, create the Spring configuration file ApplicationContext XML and create a Bean with id userDao in the configuration file.
applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd"> <bean id="userDao" class="com.nateshao.ioc.UserDaoImpl"/> </beans>
On COM nateshao. Under the IOC package, create the test class TestIoC and write the main() method in the class. In the main() method, you need to initialize the Spring container, load the configuration file, and then get the userDao instance (the Java object) through the Spring container, and finally call the say() method in the instance.
TestIoC.java
package com.nateshao.ioc; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; /** * @date Created by Shao Tongjie on 2021/10/13 21:03 * @Programming time of WeChat official account * @Personal website www.nateshao.com cn * @Blog https://nateshao.gitee.io * @GitHub https://github.com/nateshao * @Gitee https://gitee.com/nateshao * Description: */ public class TestIoC { public static void main(String[] args) { ApplicationContext applicationContext = new ClassPathXmlApplicationContext("applicationContext.xml"); UserDao userDao = (UserDao) applicationContext.getBean("userDao"); userDao.say(); } }
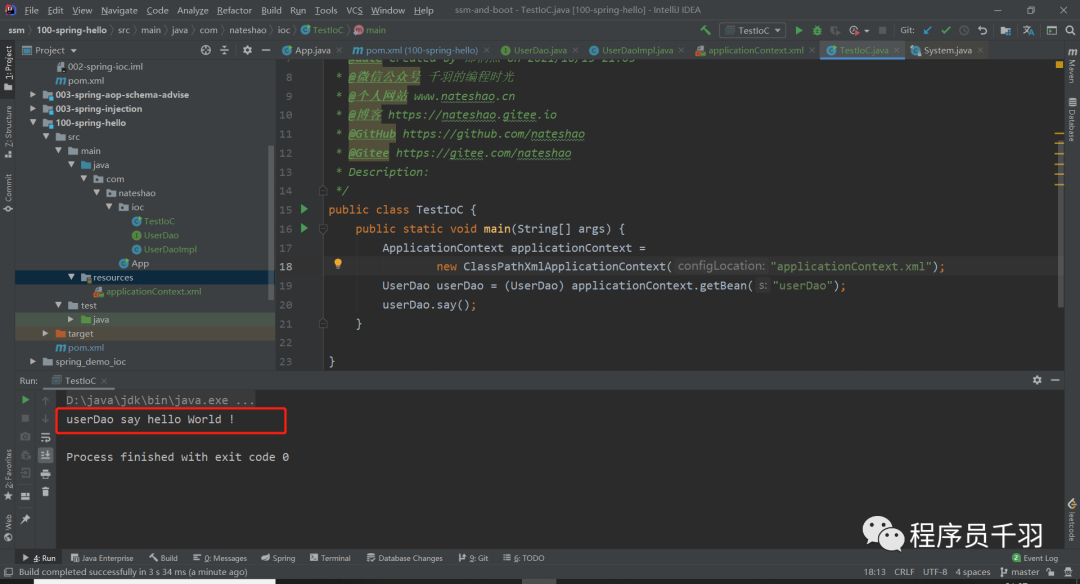
4. Dependency injection
"Concept: the full name of DI is Dependency Injection, which is called Dependency Injection in Chinese. It has the same meaning as control inversion (IoC), but the two terms describe the same concept from two angles.
IOC: after using the Spring framework, the object instance is no longer created by the caller, but by the Spring container. The Spring container will be responsible for controlling the relationship between programs, not directly controlled by the caller's program code. In this way, the control is transferred from the application code to the Spring container, and the control is reversed, which is the control reversal.
DI: from the perspective of Spring container, Spring container is responsible for assigning the dependent object to the caller's member variable, which is equivalent to injecting the caller with its dependent instance, which is Spring's dependency injection.
Using setter method to implement dependency injection
- On COM nateshao. In the IOC package, create the interface UserService and write a say() method in the interface.
UserDao.java
package com.nateshao.ioc; /** * @date Created by Shao Tongjie on 2021/10/13 20:57 * @WeChat official account programmers * @Personal website www.nateshao.com cn * @Blog https://nateshao.gitee.io * @GitHub https://github.com/nateshao * @Gitee https://gitee.com/nateshao * Description: */ public interface UserDao { public void say(); }
2. On COM nateshao. In the IOC package, create the implementation class UserServiceImpl of the UserService interface, declare the userDao attribute in the class, and add the setter method of the attribute.
UserServiceImpl.java
package com.nateshao.ioc; /** * @date Created by Shao Tongjie on 2021/10/13 21:10 * @Programming time of WeChat official account * @Personal website www.nateshao.com cn * @Blog https://nateshao.gitee.io * @GitHub https://github.com/nateshao * @Gitee https://gitee.com/nateshao * Description: */ public class UserServiceImpl implements UserService { private UserDao userDao; public void setUserDao(UserDao userDao) { this.userDao = userDao; } @Override public void say() { this.userDao.say(); System.out.println("userService say hello World !"); } }
- In the configuration file ApplicationContext XML, create a Bean with userService id, which is used to instantiate the information of UserServiceImpl class and inject the instance of userDao into userService. applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd"> <bean id="userDao" class="com.nateshao.ioc.UserDaoImpl"/> <!--Add a id by userService Examples of --> <bean id="userService" class="com.nateshao.ioc.UserServiceImpl"> <!-- take id by userDao of Bean Instance injection into userService Instance --> <property name="userDao" ref="userDao" /> </bean> </beans>
- On COM nateshao. In the IOC package, create a test class TestDI to test the program.
package com.nateshao.ioc; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; /** * @date Created by Shao Tongjie on 2021/10/13 21:16 * @Programming time of WeChat official account * @Personal website www.nateshao.com cn * @Blog https://nateshao.gitee.io * @GitHub https://github.com/nateshao * @Gitee https://gitee.com/nateshao * Description: */ public class TestDI { public static void main(String[] args) { ApplicationContext applicationContext = new ClassPathXmlApplicationContext("applicationContext.xml"); UserService userService = (UserService) applicationContext.getBean("userService"); userService.say(); } }
Operation results
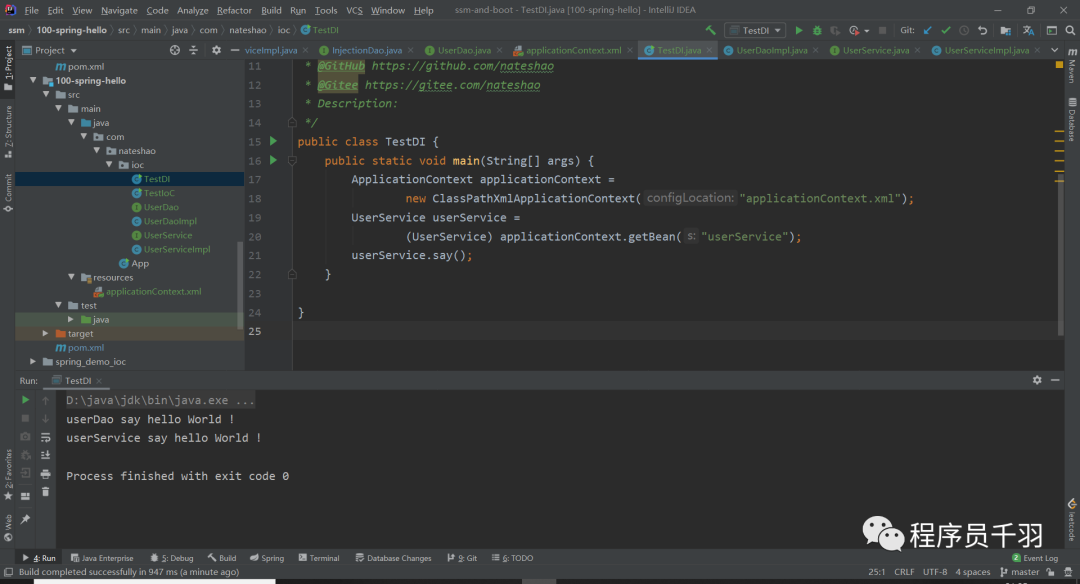
So here, I mainly introduce some basic knowledge of Spring framework, including the concept, advantages, architecture, core container, dependency injection, etc. at the same time, I explain the basic use of Spring through an introductory program.
Through the study here, you can have a preliminary understanding of the Spring framework and its architecture, master the use of the Spring framework, manage the ideas of IoC and DI in the Spring framework, and master the implementation of attribute setter method injection.
a minor question
Please briefly describe the advantages of the Spring framework.
Please briefly describe what is IoC and DI of Spring.
✎ next
What are the ways to instantiate beans?
What is the scope of a Bean?
How many ways can beans be assembled?