filter
Basic Usage
Filters are commonly used for text formatting and can be bound to the v-bind attribute. They are added to the tail of js and called by the "pipe character". They can only be used in Vue 2.0.
For example: < p > the value of message is {{message | capitalize} < / P > use the pipe character to call the capitalize filter to format the contents of the parameters before the pipe character
- The filter is essentially a function and must be defined under the filters node
- There must be a return value in the filter
- val in the filter function parameter is the value before the pipe character
<div id="app"> <p>message The value of is {{ message | capitalize}}</p> </div> <script src="./lib/vue.js"></script> <script> const vm = new Vue({ el:"#app", data:{ message: "hello,vue" }, filters:{ capitalize(val){ //val is a value before the pipe character console.log(val) // The charAt method receives the index value, indicating that the characters corresponding to the index are intercepted const first = val.charAt(0).toUpperCase() //The slice method of the string can intercept the string from the specified index const other = val.slice(1) // There must be a return value in the filter return first + other } } }) </script>
Tips
In the above example, we use the slice() method to intercept the string from the specified index. In the brand list case, we use a splice() method to delete / add items to or from the array. The two methods look very similar. Let's distinguish them.
- slice(start,end): returns the selected element from the existing array and a new array
- start is required to specify the starting position. A positive number means from front to back. If it is negative, it means from back to front, and - 1 means the last element
- End is optional. If it is not specified, it represents all elements from start to the end of the array
- Returns a subarray without changing the original array
- Splice (index, number, Item1, Item2...): add or delete items to or from the array, and return the deleted items
- index is required and specifies where to add or delete
- number is required, indicating the quantity to be deleted. If it is 0, the item will not be deleted
- item1,item2... Are optional to add new items to the array
- The original array will be changed and the deleted items will be returned
**Difference: * * slice() is often used to cut strings, and splice() is used to delete a segment of elements in an array
**Association: * * substr(start,num) and substring(start,end) are two methods often used to intercept strings
Private and global filters
Private filter: the filter defined under the filters node can only be used in the el area controlled by the current vm instance
Global filters: using vue Filter (name, function), independent of each vm instance, can be shared among multiple vue instances
- If the global filter has the same name as the private filter, the private filter is called according to the proximity principle
<div id="app"> <p>message The value of is {{ message | capitalize}}</p> </div> <div id="app2"> <p>message The value of is {{ message | capitalize}}</p> </div> <script src="./lib/vue.js"></script> <script> //Using Vue Filter() defines the global filter Vue.filter('capitalize',function(str){ const first = str.charAt(0).toUpperCase() const other = str.slice(1) //Private has the same name as the whole, and the principle of proximity gives priority to private return first + other+' ------' }) const vm = new Vue({ el:"#app", data:{ message: "hello,vue" }, filters:{ capitalize(val){ //val is a value before the pipe character console.log(val) // The charAt method receives the index value, indicating that the characters corresponding to the index are intercepted const first = val.charAt(0).toUpperCase() //The slice method of the string can intercept the string from the specified index const other = val.slice(1) // There must be a return value in the filter return first + other } } }) const vm2 = new Vue({ el:'#app2', data:{ message:"vue.js hello message2" } }) </script>
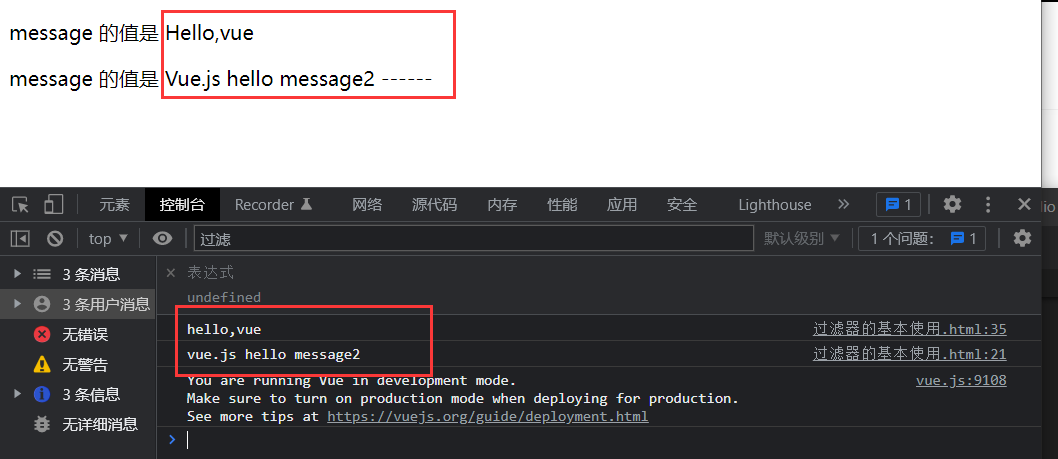
Other uses of filters
-
Calling multiple filters consecutively: {{message | filterA | filterB}} finally returns the result of filterB processing
-
Since the essence of the filter is a js function, it can receive parameters: {{message | filter a (arg1, arg2)}}
-
In the formal parameter list of the filter handler function:
- The first parameter is always the value to be processed before the pipe character
- Starting from the second parameter, it is the parameter when calling the filter
For example: Vue filter('filterA',(msg,arg1,arg2) =>{.....})
Listener
Basic Usage
The watch listener is used to monitor data changes and perform specific operations on data changes. Data changes will automatically trigger the listener.
-
All listeners are defined under the watch node
-
The listener is essentially a function. To monitor which data changes, just use the data name as the method name
-
Formal parameters can be passed in, with the new value first and the old value last
<div id="app"> <input type="text" v-model.lazy="username"> </div> <script src="./lib/vue.js"></script> <script> const vm = new Vue({ el: "#app", data:{ username: "" }, watch:{ username(newVal , oldVal){ console.log("username undergo changes", newVal, oldVal) } } }) </script>
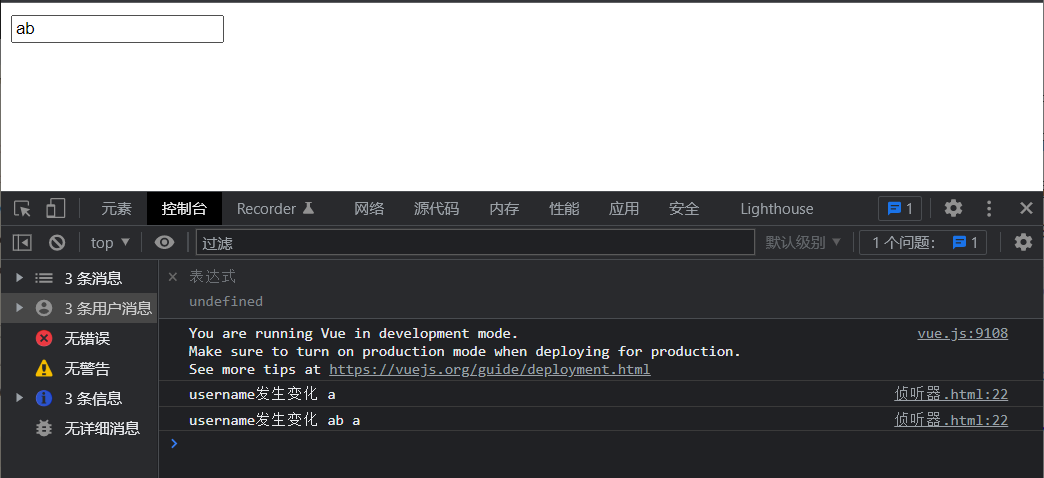
Format of listener
- Method format listener
- Disadvantage 1: it cannot be triggered automatically when you first enter the web page. It cannot be triggered until the data changes
- Disadvantage 2: if you listen to an object, if the properties in the object change, the listener will not be triggered
- Object format listener
- Advantage 1: the listener can be triggered automatically through the immediate option
- Advantage 2: through the deep option, the listener can deeply listen for the changes of each attribute in the object
Advantage 1: immediate option
Method format listener | Object format listener |
---|---|
![]() | ![]() |
![]() | ![]() |
Advantage 2: deep option - enable deep listening. As long as any attribute in the object changes, the listener of the object will be triggered
Method format listener | Object format listener |
---|---|
![]() | ![]() |
![]() | ![]() |
Implement listening for changes in sub attributes of objects
When using this method, it should be noted that if the sub attributes of the listening object change, it must be wrapped with a layer of single quotation marks
watch:{ 'info.username'(newVal,oldVal){ console.log(newVal,oldVal) } }
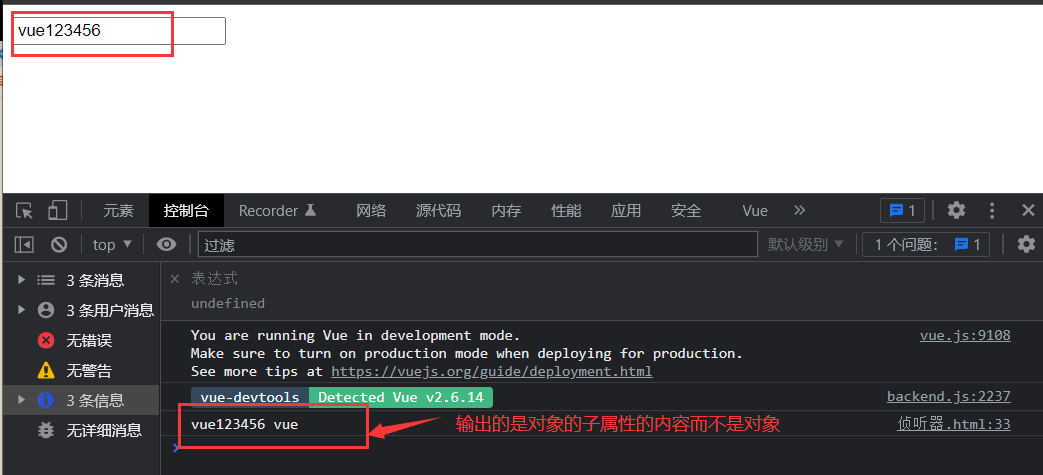
Syntax summary of listener in object format
// Object format listener username:{ handler(newVal, oldVal){ ...... }, //The function of immediate is to control whether the listener is automatically triggered once. The default value is false immediate:true, //The function of deep is to enable deep listening. As long as any attribute of the object changes, the listener of the object will be triggered deep:true }