In some applications, the product requirements are as follows: for example, when the user jumps to the route, the user needs to be prompted that the data of the current page has not been saved, and the user needs to be prompted to leave for confirmation.
The general effect is as follows:
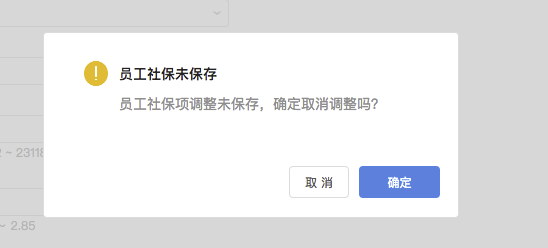
Previous router router practices are as follows:
Routing hook: componentDidMount() { this.props.router.setRouteLeaveHook( this.props.routes[1], this.routerWillLeave() )} Routerwillleave() {return false} ture is to leave, false is to leave
In react router 4:
Using the Prompt component:
import { Prompt } from 'react-router-dom'; .... render(){ return( <Prompt message="Are you sure you want to leave?" when={true}/> ) }
Where message can be:
message: string
Set the prompt when the user leaves the current page.
< prompt message = "are you sure you want to leave? / >message: func
Return function set when the user leaves the current page
<Prompt message={location => (
Are you sue you want to go to ${location.pathname}?
)} />when: bool
By setting certain conditions, you need to decide whether to enable Prompt. When the attribute value is true, you need to enable prevent conversion;
However, if you use this method directly, the default pop-up effect of the page is as follows:
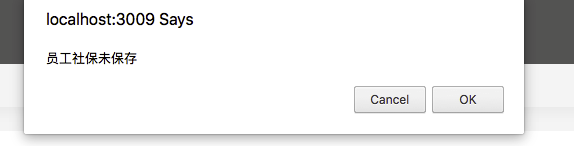
Does not meet our product design requirements.
1. custom
Go directly to demo
import React from 'react' import ReactDOM from 'react-dom' import { Prompt } from 'react-router' import { BrowserRouter as Router, Route, Link } from 'react-router-dom' const MyComponent1 = () => ( <div>Component 1</div> ) const MyComponent2 = () => ( <div>Module two</div> ) class MyComponent extends React.Component { render() { const getConfirmation = (message,callback) => { const ConFirmComponent = () => ( <div> {message} <button onClick={() => {callback(true);ReactDOM.unmountComponentAtNode(document.getElementById('root1'))}}>Determine</button> <button onClick={() => {callback(false);ReactDOM.unmountComponentAtNode(document.getElementById('root1'))}}>cancel</button> </div> ) ReactDOM.render( <ConFirmComponent />, document.getElementById('root1') ) } return ( <Router getUserConfirmation={getConfirmation}> <div> <Prompt message="Are you sure you want to leave?" /> <Link to="/a">Jump component II</Link> <Route component={MyComponent1}/> <Route exact path="/a" component={MyComponent2}/> </div> </Router> ) } } ReactDOM.render( <MyComponent/>, document.getElementById('root') )
See the article for more api introduction of react router