1. Title
Give you the root node root of the binary tree and an integer targetSum representing the target sum. Judge whether there is a path from the root node to the leaf node in the tree. The sum of all node values on this path is equal to the target and targetSum.
A leaf node is a node that has no children.
Example 1:
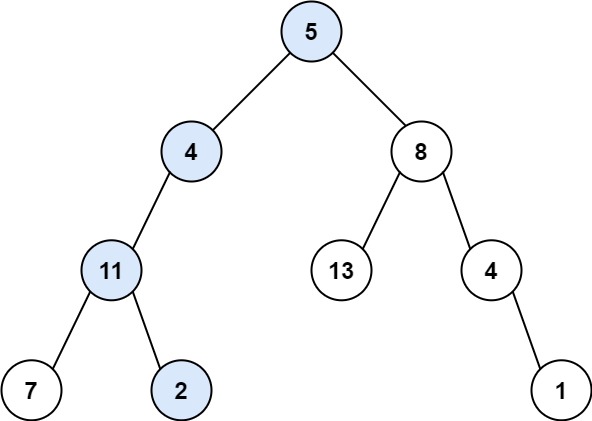
Input: root = [5,4,8,11,null,13,4,7,2,null,null,null,1], targetSum = 22 Output: true
Example 2:
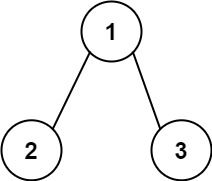
Input: root = [1,2,3], targetSum = 5 Output: false
Example 3:
Input: root = [1,2], targetSum = 0 Output: false
Tips:
- The number of nodes in the tree is in the range [0, 5000]
- -1000 <= Node.val <= 1000
- -1000 <= targetSum <= 1000
2. Train of thought
(recursive) O ( n ) O(n) O(n)
Given a binary tree and a sum, judge whether there is a path from the root node to the leaf node, and the sum of all numbers on the path is equal to sum.
Example:
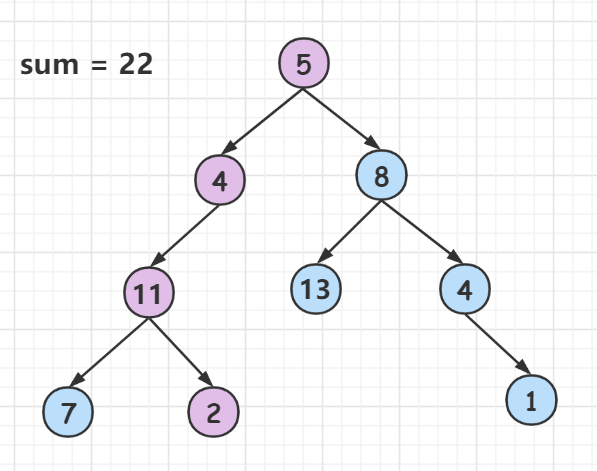
As shown in the example, there is a path [5, 4, 11, 2] whose sum is equal to sum. Let's explain the practice of recursion.
We go from the root node to the leaf node. For each node, let sum subtract the value of the current node. When we go to a leaf node, if the sum value is 0, it means that we have found a path from the root node to the leaf node, and the sum of all numbers on the path is equal to sum.
Recursive boundary:
- 1. Recursion to an empty node directly returns false.
- 2. Recurse to the leaf node to determine whether sum is 0.
The specific process is as follows:
- 1. Go from root node to leaf node.
- 2. Use sum to subtract the value of the current node, that is, sum - = root - > val.
- 3. If the current node is a leaf node, judge whether sum is 0 and return the corresponding result.
- 4. Recurse the left and right subtrees of the current node.
Implementation details:
There is no need to trace back in the recursive process of this problem, because our sum is value transfer. When recursing different paths, the sum values maintained by different paths do not affect each other.
Time complexity analysis: O ( n ) O(n) O(n), where n n n is the number of nodes in the tree. Each node is traversed only once.
3. c + + code
/** * Definition for a binary tree node. * struct TreeNode { * int val; * TreeNode *left; * TreeNode *right; * TreeNode() : val(0), left(nullptr), right(nullptr) {} * TreeNode(int x) : val(x), left(nullptr), right(nullptr) {} * TreeNode(int x, TreeNode *left, TreeNode *right) : val(x), left(left), right(right) {} * }; */ class Solution { public: bool hasPathSum(TreeNode* root, int sum) { if(!root) return false; sum -= root->val; if(!root->left && !root->right) return !sum; //Leaf node to judge whether sum is 0 else return hasPathSum(root->left,sum) || hasPathSum(root->right,sum); //Recursive left and right subtree } };
4. java code
/** * Definition for a binary tree node. * public class TreeNode { * int val; * TreeNode left; * TreeNode right; * TreeNode() {} * TreeNode(int val) { this.val = val; } * TreeNode(int val, TreeNode left, TreeNode right) { * this.val = val; * this.left = left; * this.right = right; * } * } */ class Solution { public boolean hasPathSum(TreeNode root, int sum) { if(root == null) return false; sum -= root.val; if(root.left == null && root.right == null) return sum == 0;//Leaf node to judge whether sum is 0 else return hasPathSum(root.left,sum) || hasPathSum(root.right,sum);//Recursive left and right subtree } }
Original title link: 112. Path sum