The topics are as follows:
Give you an integer X. if x is a palindrome integer, return true; Otherwise, false is returned. Palindromes are integers that are read in the same positive order (from left to right) and reverse order (from right to left). For example, 121 is palindrome, not 123.

To judge whether a number is a palindrome number, the first idea is to convert it into a string, and then judge whether it is the same by reversing the string, for example:
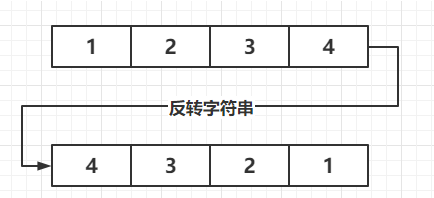
If the string after inversion is different, it is not a palindrome number.
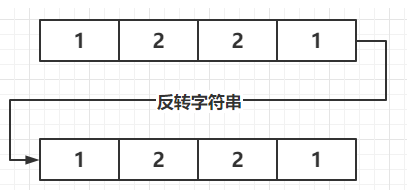
If the number after inversion is the same, it is the palindrome number. The resulting code is as follows:
public class Solution { public static void main(String[] args) { System.out.println(isPalindrome(121)); } public static boolean isPalindrome(int x) { // Convert a number to a string String str = String.valueOf(x); // Reverse it String newStr = new StringBuilder(str).reverse().toString(); return str.equals(newStr); } }
Submit to LeetCode:
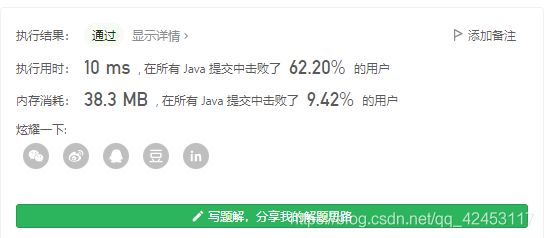
At the end of the topic, an advanced requirement is also put forward:
Advanced: can you solve this problem without converting integers to strings?
How do you do this without strings? In fact, it is also very simple. You can directly reverse the number by calculating. Take 1234 as an example. First, we need to obtain the single digit 4 of the number. How to obtain it? Balance 10:

Next, get the ten digit 3, divide 1234 by 10 to get the number 123, and then let 123 find the remainder of 10 to get 3:
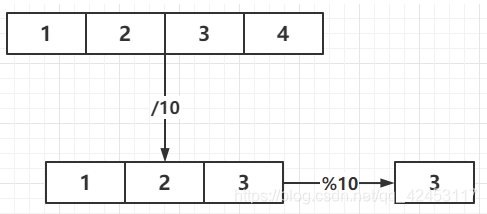
By analogy, you can get each digit in the number:
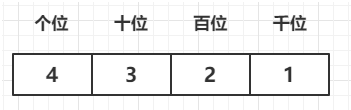
Then multiply each bit by the corresponding carry. Since we want to reverse, we have to reverse it. 4 is regarded as thousands, 3 as hundreds, 2 as tens, and 1 as a bit:
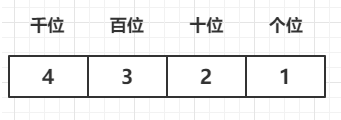
Thus, the reversed number is obtained: 4 * 1000 + 3 * 100 + 2 * 10 + 1 = 4321.
The code is as follows:
public class Solution { public static void main(String[] args) { System.out.println(isPalindrome(121)); } public static boolean isPalindrome(int x) { int num = x; int result = 0; // Reverse number while (x > 0) { result *= 10; result += x % 10; // Get every bit x /= 10; } return num == result; } }
However, there is a small loophole in this program, that is, the cross-border problem. When a number is reversed and greater than the maximum value of int, the program will make an error:
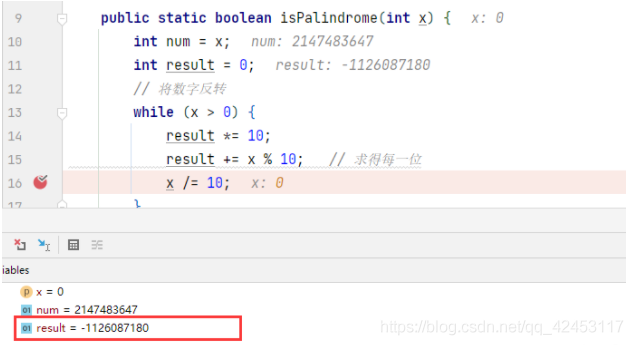
At this time, because the result exceeds the maximum value that int can represent, it has become a negative value. It can never be equal to the input value, so the program cannot accurately judge whether the input value is a palindrome number.
In order to solve this problem, we can not reverse all the numbers, but reverse half of them. Because of the nature of palindromes, we only need to know that half of them are the same, then it must be palindromes.
We need to discuss it in two cases. The first is the number of odd length. Take 12321 as an example:

We get the number of the reverse half length:
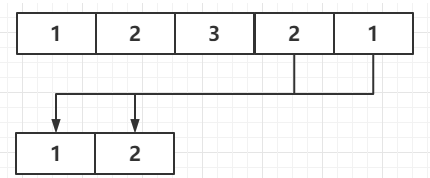
Comparing it with the number of half the length before inversion, it is found that it is 12, indicating that 12321 is a palindrome number.
If it is a number of even length, take 1221 as an example:

Still get the number of half the length after inversion:
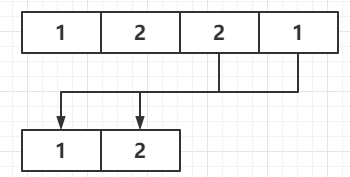
Compare it with the number that reverses the first half of the length.
So the key is how to cut and obtain numbers? Let's discuss the odd length and even length numbers together:

First, let it find the remaining 10 to get the last digit:
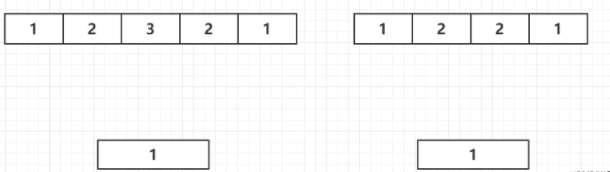
Then divide the original number by 10 to round off the last digit:
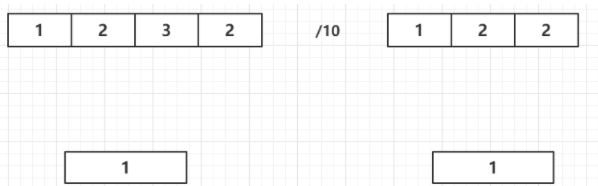
Find the remaining 10 to get the last bit:
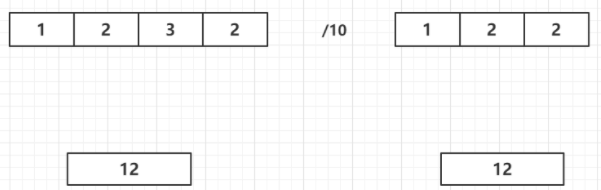
Divide the original number by 10 and round off the last digit:
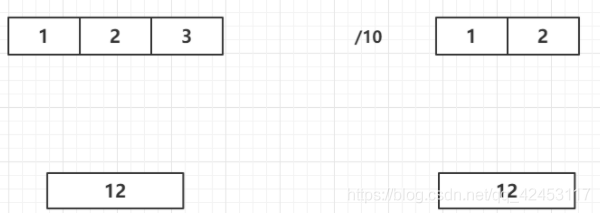
The operation should be stopped here, because the number of even length cases has obtained a number of half the length. For even number cases, directly compare whether the newly generated number is equal to the original number; For the case of odd length, although half the length is obtained, the length in the original number is 3, so we should obtain it again:
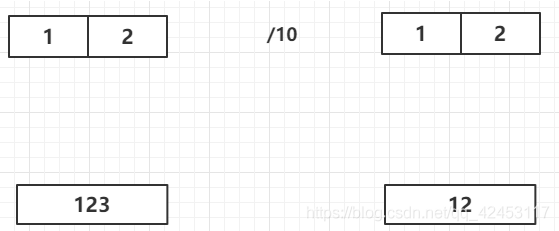
It can be concluded that the termination condition of the cycle is that when the original number is less than or equal to the newly generated number, for odd numbers, we need to remove the last digit and compare it with the original number, so divide the newly generated number by 10 and compare it.
To sum up, the code is:
public class Solution { public static void main(String[] args) { System.out.println(isPalindrome(12321)); } public static boolean isPalindrome(int x) { // Negative numbers must not be palindromes if (x < 0 || (x % 10 == 0 && x != 0)) { // If the last digit of the number is 0, if it is a palindrome number, the first digit of the number must be 0, and the number satisfying the situation is only 0 // Therefore, if the last bit is 0, but the number is not 0, false will be returned directly return false; } int newNum = 0; // Stop the loop when the original number is less than or equal to the newly generated number while (x > newNum) { newNum *= 10; newNum += x % 10; x /= 10; } // Compare whether the newly generated number is equal to the original number return x == newNum || x == newNum / 10; } }
Submit to LeetCode:
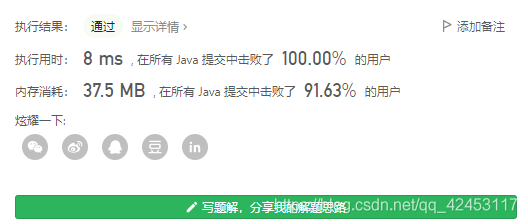