1, Overview
- 1. exit() function and_ The exit () function is used to terminate the process.
- 2. Return is a C language keyword. It is mainly used for the return of a sub function and identifies the end of the sub function.
2, Function description
when the program executes to the exit() function or_ When using the exit() function, the process will unconditionally stop all remaining operations, clear various data structures, and terminate the operation of the process.
however, there are differences between the two functions. The function call is shown in Figure 2.1 below.
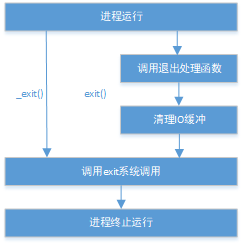
return means to turn the program flow from the called function to the calling function, and bring the value of the expression back to the calling function to return the function value. When returning, it can be attached with a return value, which is specified by the parameters behind return.
to sum up:
return: the end of the function is the end of the function level, and exit() and_ eixt() is the end of the system level.
however, it should be noted that the whole process ends when the return statement is executed in the main function.
it can be seen from figure 2.1_ The exit() function is used to directly stop the process, clear the memory space it uses, and clear its various data structures in the kernel; The exit() function wraps these bases and adds an operation before exiting.
exit() function and_ The biggest difference of the exit () function is that before terminating the current process, the exit () function checks which files are opened by the process and writes the contents of the file buffer back to the file, which is the expression of "cleaning the IO buffer" in the figure.
in the standard function library of Linux, there is an operation called "buffered IO(buffered I/O)", which is characterized by a buffer in memory corresponding to each open file.
several records will be read continuously each time the file is read, so that the next time the file is read, it can be read directly from the buffer in the memory; Similarly, each time a file is written, it is only written to the buffer in memory. When certain conditions are met (such as reaching a certain number or encountering specific characters), the contents of the buffer are written to the file at one time.
this technology greatly increases the speed of file reading and writing, but it also brings some troubles to programming. For example, some data that are thought to have been written to the file are actually stored in the buffer because they do not meet specific conditions. It is used at this time_ The exit() function closes the process directly, and the data in the buffer will be lost. Therefore, if you want to ensure the integrity of the data, you'd better use the exit() function.
if main() is in a recursive program, exit() will still terminate the program, but return will transfer control to the previous level of the recursion, and return will not terminate the program until the first level. Another difference between return and exit () is that even if exit () is invoked even in functions other than main (), it will terminate the whole process.
3, Syntax of exit() function and exit() function
1. exit() function and_ The prototype of the exit () function and the header file
#include <stdlib.h> void exit(int status);
#include <unistd.h> void _exit(int status);
2. Exit() function and_ Arguments to the exit() function
status is an integer parameter that can be used to pass the status at the end of the process. Generally speaking, exit(0) indicates that the program exits normally, exit(1) or exit(-1) indicates that the program exits abnormally.
standard C has EXIT_SUCCESS and EXIT_FAILURE two macros, defined in the header file stdlib.h:
/* We define these the same for all machines. Changes from this to the outside world should be done in `_exit'. */ #define EXIT_FAILURE 1 /* Failing exit status. */ #define EXIT_SUCCESS 0 /* Successful exit status. */
it is recommended to use exit(EXIT_SUCCESS) to indicate normal exit and exit(EXIT_FAILURE) to indicate abnormal exit during actual programming, which is more convenient for code reading and understanding.
in actual programming, you can use the wait() system call to receive the return value of the child process, so as to carry out different processing according to different return values.
2. Exit() function and_ Usage of exit() function
exit() function and_ The exit () function is two simple and serious functions, and return is also a serious keyword. The following three example programs are sufficient.
1). Usage of exit() function
#include <stdio.h> #include <stdlib.h> int main(int argc, const char *argv[]) { /* The standard output stream stdout is a line buffer. It is actually written to the terminal only when the "\ n" line feed character is encountered. */ printf("Using exit...\n"); /* The next output does not output a newline character */ printf("This is the content in buffer."); exit(0); /* Call the exit function to test */ printf("After the exit...\n"); return 0; }
after simple compilation and operation, you can see the operation effect, as shown in Figure 3.1 below.

2), _ Usage of exit() function
#include <stdio.h> #include <unistd.h> int main(int argc, const char *argv[]) { /* The standard output stream stdout is a line buffer. It is actually written to the terminal only when the "\ n" line feed character is encountered. */ printf("Using exit...\n"); /* The next output does not output a newline character */ printf("This is the content in buffer."); _exit(0); /* Call_ exit function test */ printf("After the _exit...\n"); return 0; }
after simple compilation and operation, you can see the operation effect, as shown in Figure 3.2 below.
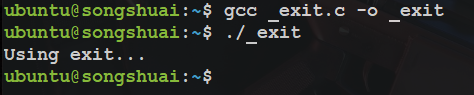
3) use of return
#include <stdio.h> #include <stdlib.h> // for EXIT_SUCCESS int return_test(int num) { if (num == 0) { return 0; /* If num is 0, the recursion is about to end */ } else { /* Returns the sum of itself and the next number */ return num + return_test(num - 1); } } int main(int argc, const char *argv[]) { /* Define a test variable with an initial value of 100 */ int num = 100; printf("Testing return...\n"); /* Call the test function to realize the addition of 0-100 */ int cnt = return_test(num); /* Test execution position and output results */ printf("cnt = %d\n", cnt); /* return halfway through the program */ return EXIT_SUCCESS; printf("After the return...\n"); return 0; }
after simple compilation and operation, you can see the operation effect, as shown in Figure 3.3 below.
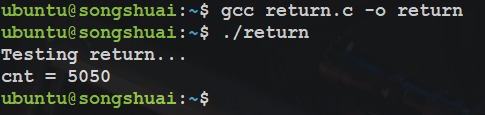
well, it's not easy to summarize and write without much nonsense. If you like this article or it's useful to you, please use your little hands to help you praise it. Of course, it's better to pay attention to a wave. Well, that's it. Mmda (* ~ 3)( ε  ̄ *).
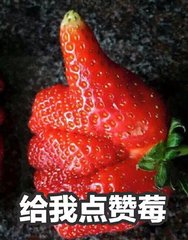
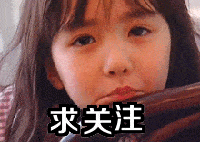
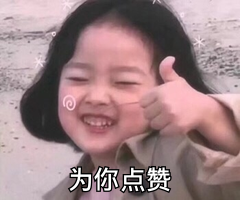