Checking of Check Agreement
I found that in the last article I forgot to check my agreement. Although there is no agreement, it still needs to be done.
Find the corresponding area in register.html:
<div class="form-group"> <label> <input type="checkbox" name="aggree" value="1"> I agree to the agreement. </label> </div>
Its name is aggree. We continue to interrupt the breakpoint to test where it went during transmission.

Click the registration button after entering content on the page
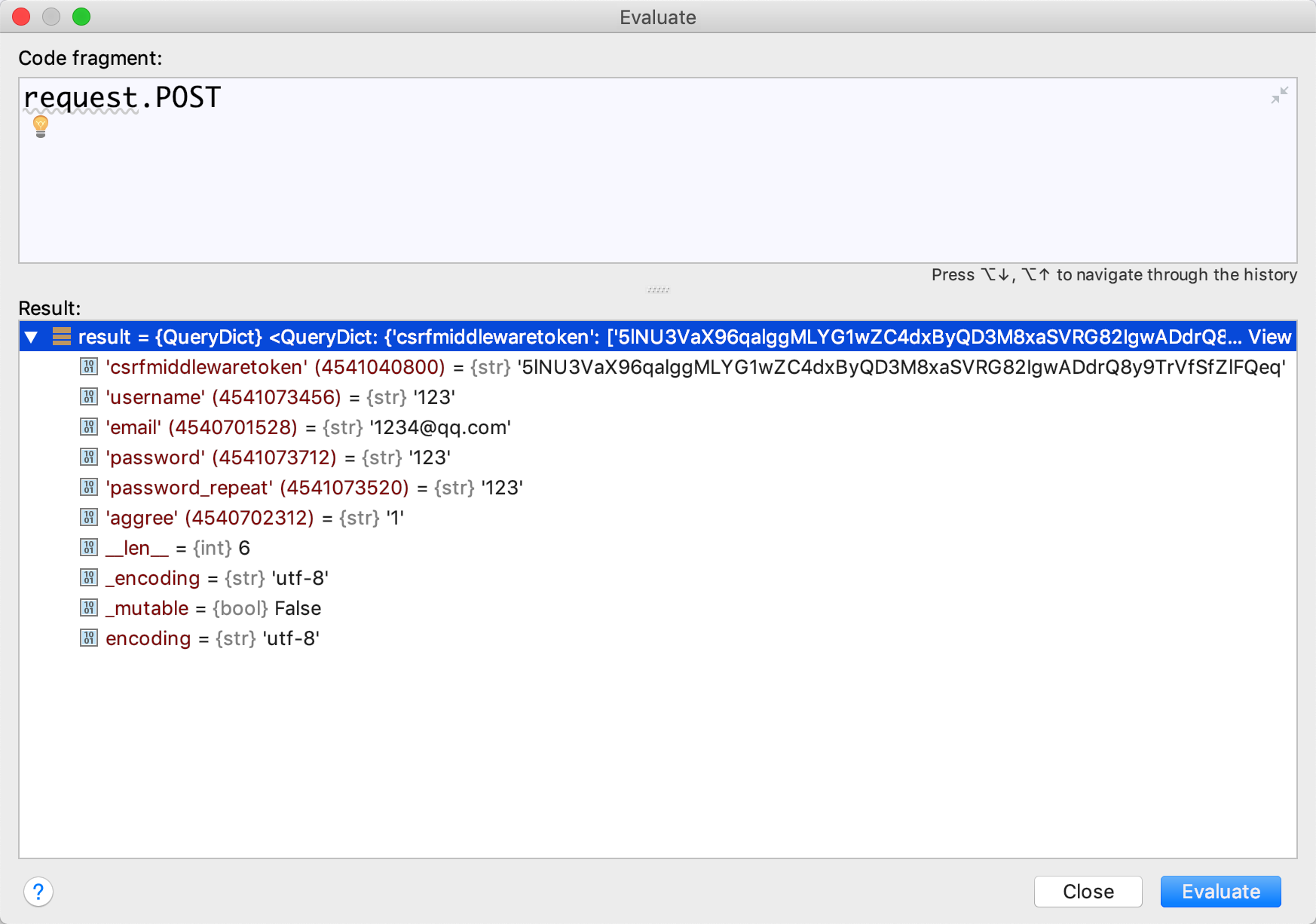
You can see the aggree field in the figure. Similarly, test the transmission content after unchecking:
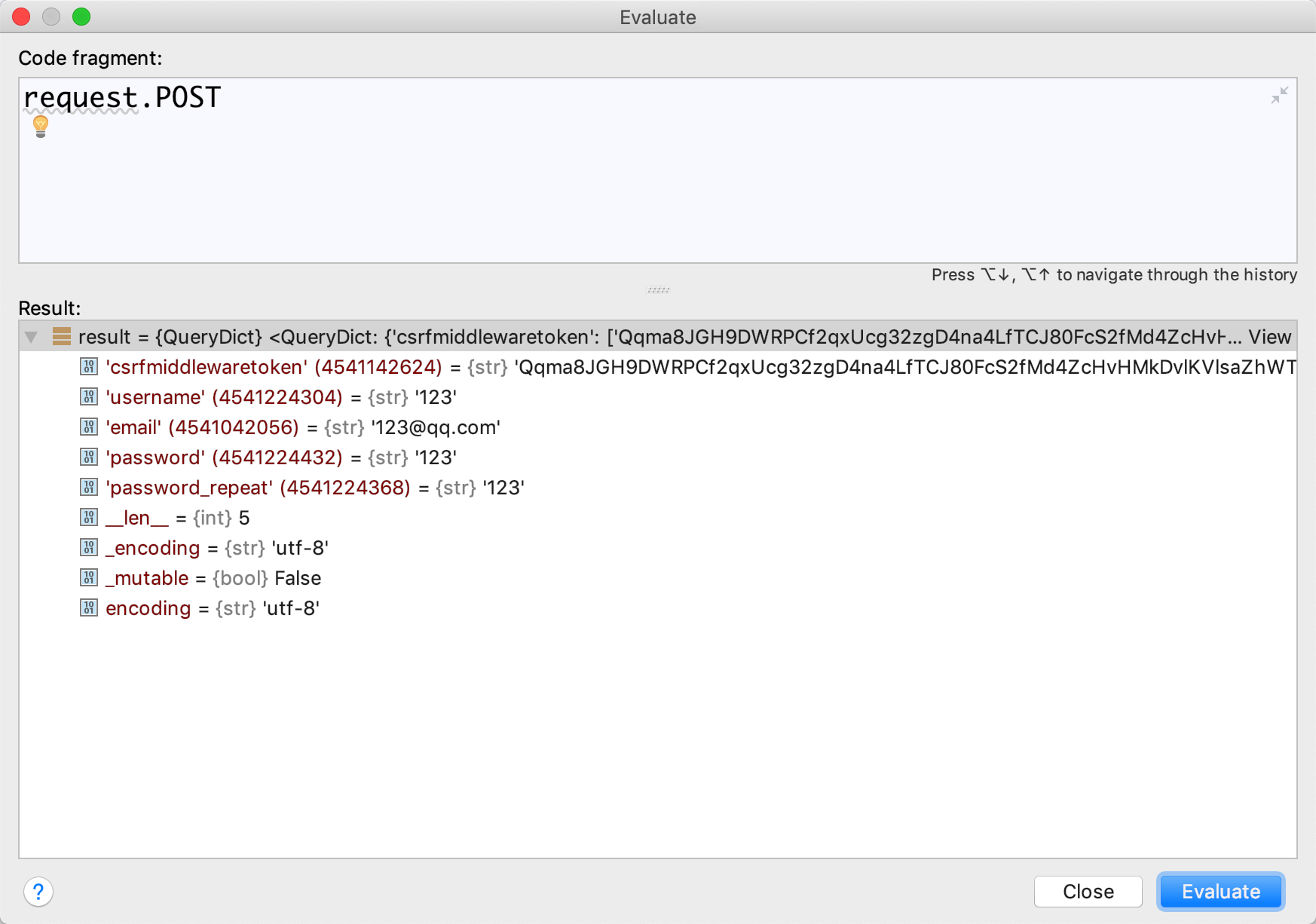
You can see that there is no field at all. So our judgment is whether this field is good or not. Add a little bit of fault-tolerance to the dictionary get
if not request.POST.get('aggree'): return to_json_data(errno=Code.AGGREE,errmsg=error_map[Code.AGGREE])
Above we have completed the preliminary registration function. But there are many bug s visible to the naked eye. These questions were revised in the subsequent self-test.
Login function
Now let's write the login function, but before writing, write down the page after login, or where to log in?
Write a simple index.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Interface Test Platform</title> </head> <body> </body> </html>
First dig a hole, then replace the specific content.
First implement the post request for LoginView
We need the following steps to complete a login:
- Get the parameters returned by the front end
- Calibration parameter
- User login to set session information
- Return front end
Because CSRF middleware is used for post transfer checking, add {% csrf_token%} to the login page
Join the same location as the registration, inside the form formcsrf check
Get the parameters returned by the front end
The following is the writing of the back-end code.
The first is to obtain the content of the front-end transmission as registered:
def post(self,request): try: json_data = request.POST if not json_data: return to_json_data(errno=Code.PARAMERR, errmsg="The parameter is empty. Please re-enter it.") dict_data = json.loads(json_data.decode('utf8')) except Exception as e: logging.info('error message:\n{}'.format(e)) return to_json_data(errno=Code.UNKOWNERR, errmsg=error_map[Code.UNKOWNERR])
Also use breakpoints to view the contents of the transmissions
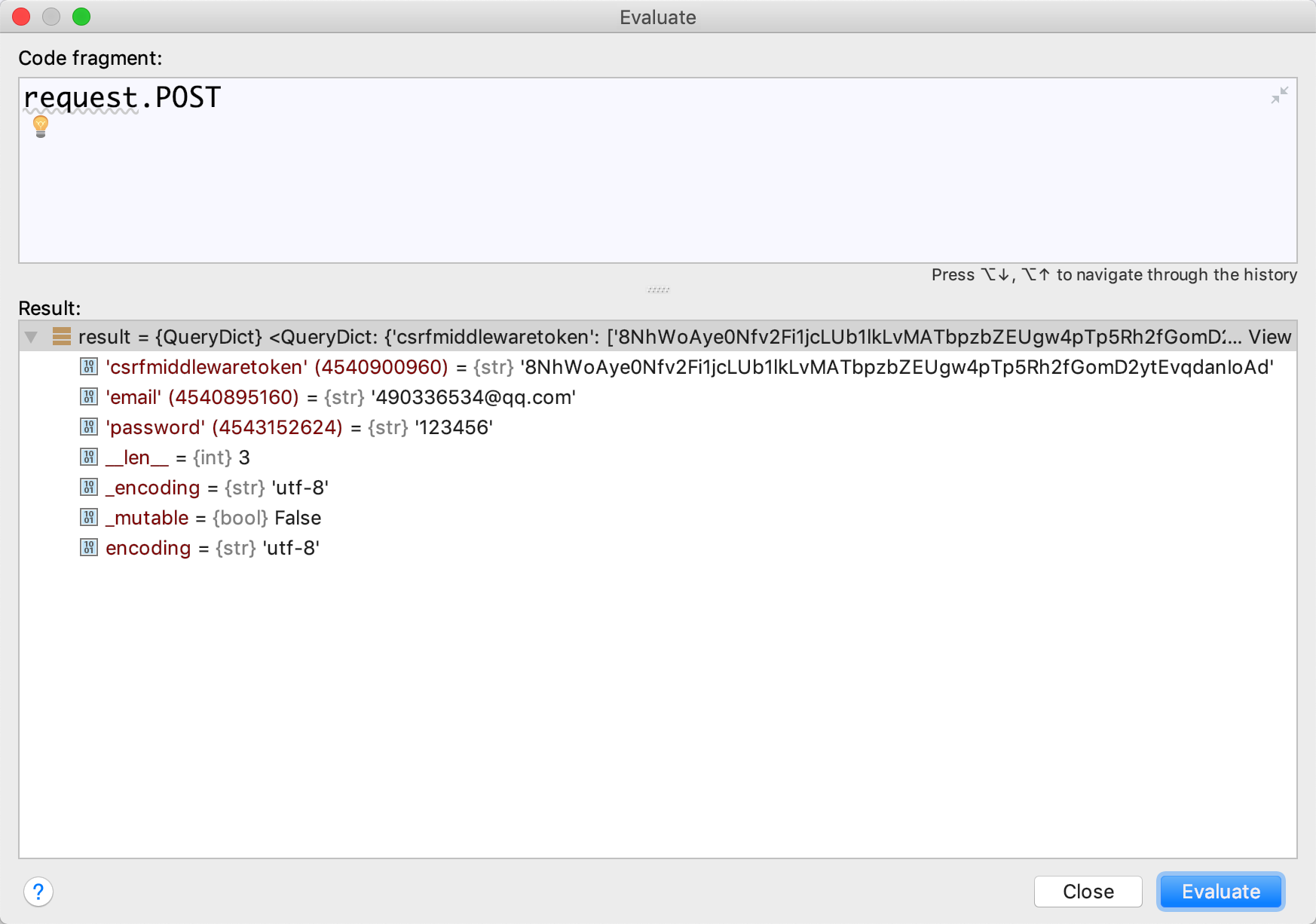
Calibration parameter
Looks like it's okay, then check the content. Need to compare with database
form checking is also used this time.
class LoginForm(forms.Form): """ login form data """ email = forms.EmailField() password = forms.CharField(label='Password', max_length=20, min_length=6, error_messages={"min_length": "Password length greater than 6", "max_length": "Password length should be less than 20", "required": "Password cannot be empty"} ) remember_me = forms.BooleanField(required=False) def __init__(self, *args, **kwargs): """ :param args: :param kwargs: """ self.request = kwargs.pop('request', None) super(LoginForm, self).__init__(*args, **kwargs) def clean(self): """ :return: """ # 1. Obtain parameters after cleaning cleanded_data = super().clean() user_info = cleanded_data.get('email') passwd = cleanded_data.get('password') hold_login = cleanded_data.get('remember') # 2. Query the database to determine whether the user's account and password are correct. user_queryset = User.objects.filter(Q(email=user_info)) if user_queryset: user = user_queryset.first() if user_queryset.get(password=passwd): # 3. Whether to set user information into the session if hold_login: self.request.session.set_expiry(constants.USER_SESSION_EXPIRES) else: self.request.session.set_expiry(0) # Close the browser and empty it else: raise forms.ValidationError('The user password is incorrect, please re-enter it!') else: raise forms.ValidationError('User account does not exist, please re-enter!')
More important in this code is the clean function
cleanded_data = super().clean() user_info = cleanded_data.get('email') passwd = cleanded_data.get('password') hold_login = cleanded_data.get('remember')
Through these lines of code, you get specific content, such as
Useinfo is the email filled in by the front end.
passwd is the password filled in by the front end.
hold_login is the front-end tick to remember me;
Then query the database for the existence of this email
user_queryset = User.objects.filter(Q(email=user_info))
Check the password if it exists
user_queryset.get(password=passwd)
If the password is correct, record the session information.
User login to set session information
Use self.request.session.set_expiry to set the length of session saved.
# User session information expiration time, per second, set here to 5 days USER_SESSION_EXPIRES = 5 * 24 * 60 * 60
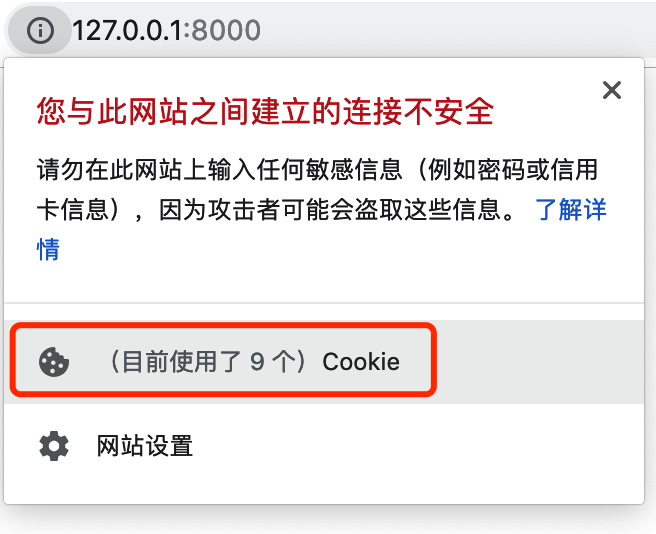
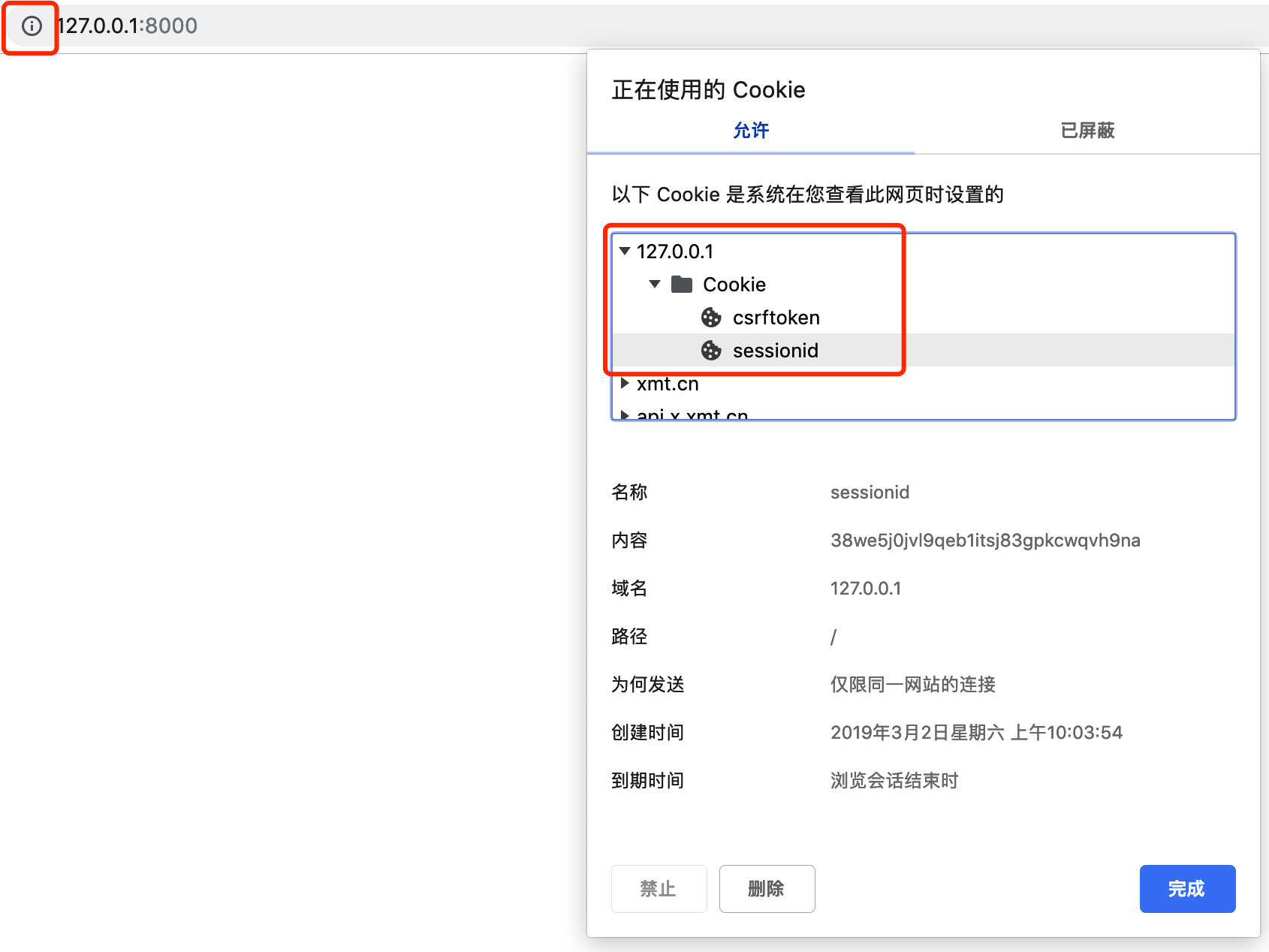
The picture shows that the session is held until the browser closes.
This is a bit of a way out with our plan. Our configuration should be able to save for 5 days.
Check the code through breakpoints
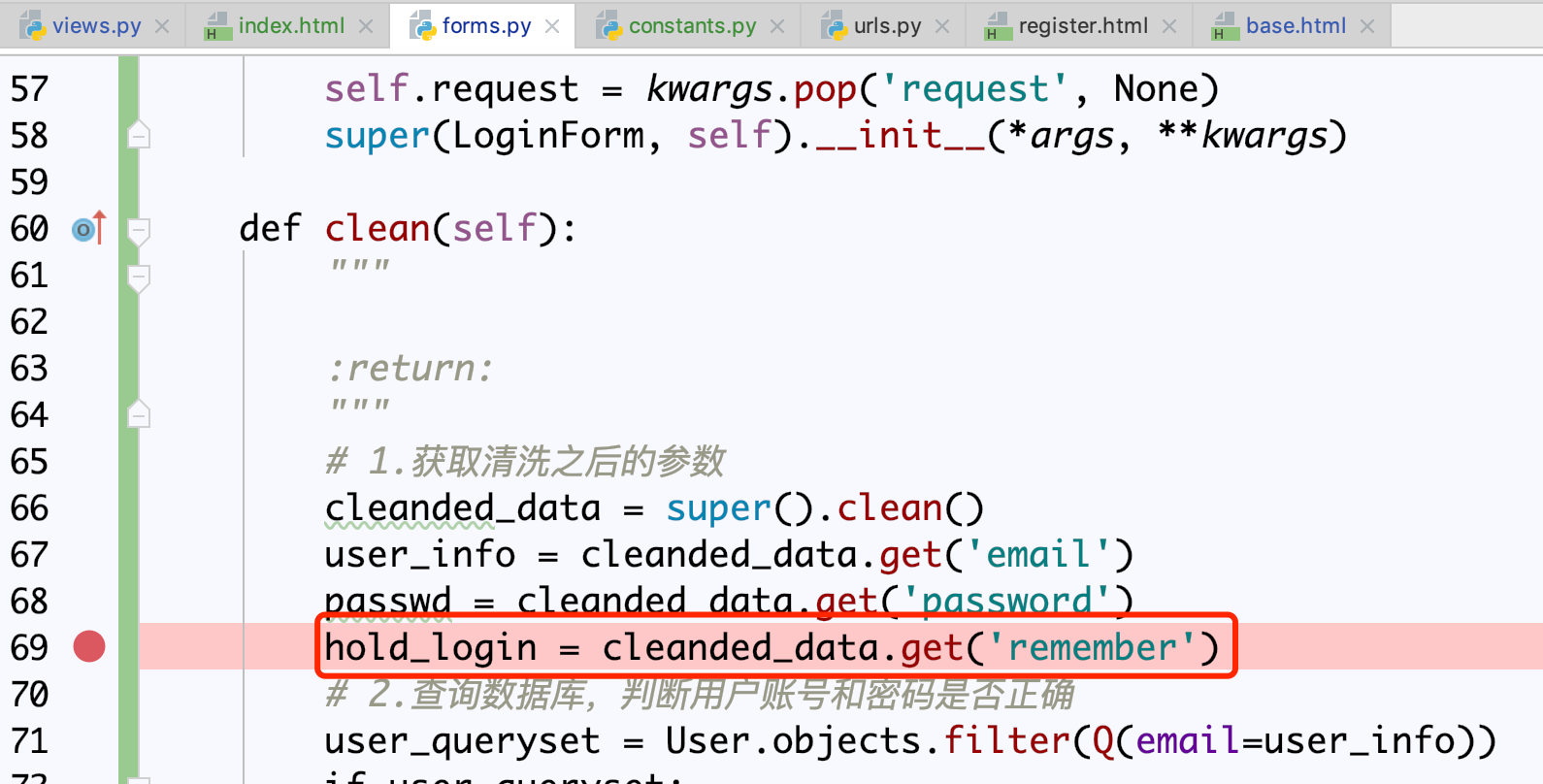
It was found that hold_login took None, and flipped up to find that the original name was inconsistent with the name in forms.
Modify this line: remember = forms.BooleanField(required=False)
Test again
Check the new expiration time:
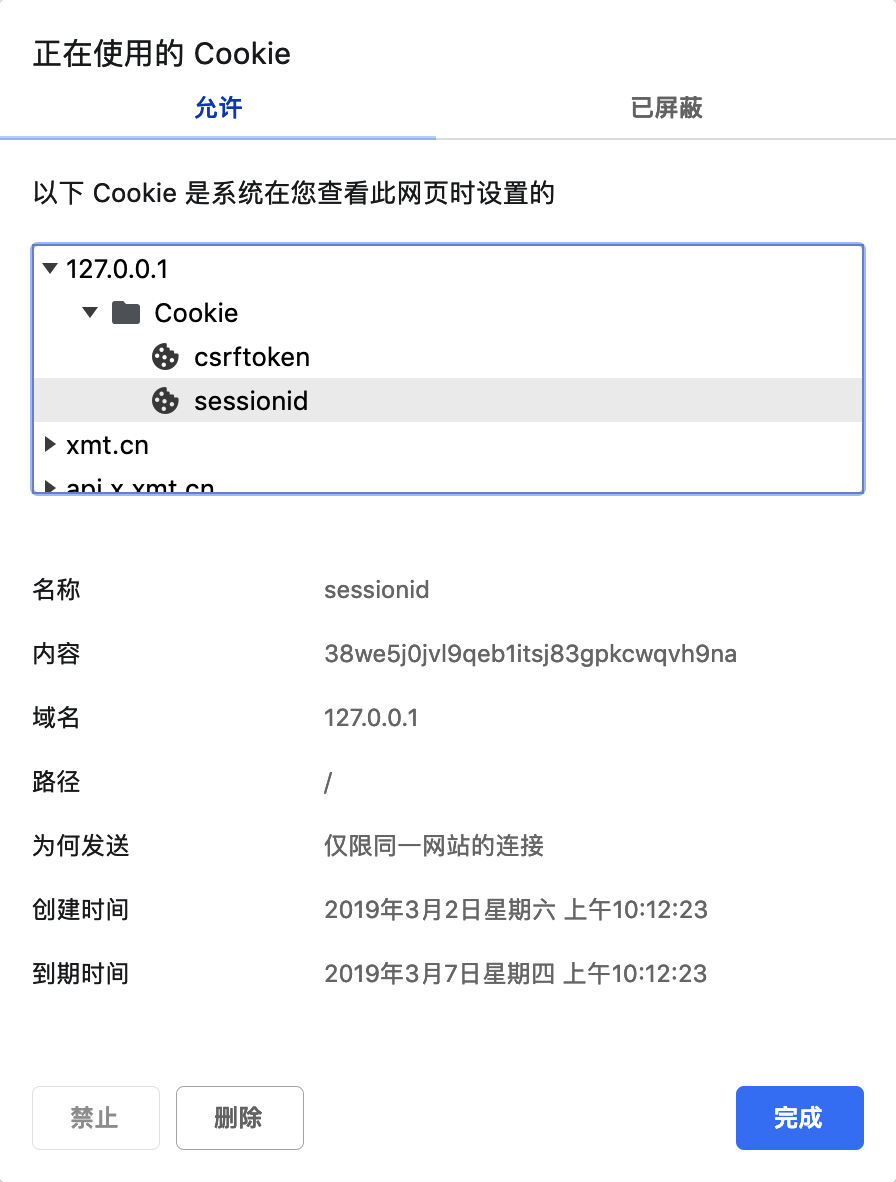
Backend code
The following is the code for the post related section.
def post(self, request): try: json_data = request.POST if not json_data: return to_json_data(errno=Code.PARAMERR, errmsg="The parameter is empty. Please re-enter it.") use_key = [ "email","password", "remember"] dict_data = {} for i in use_key: dict_data[i] = request.POST.get(i) except Exception as e: logging.info('error message:\n{}'.format(e)) return to_json_data(errno=Code.UNKOWNERR, errmsg=error_map[Code.UNKOWNERR]) form = LoginForm(data=dict_data, request=request) if form.is_valid(): return render(request,'index/index.html') else: err_msg_list = [] for item in form.errors.get_json_data().values(): err_msg_list.append(item[0].get('message')) err_msg_str = '/'.join(err_msg_list) return to_json_data(errno=Code.PARAMERR, errmsg=err_msg_str)
Welcome to the source code:( https://github.com/zx490336534/Zxapitest)
PS: Welcome to my public number ~
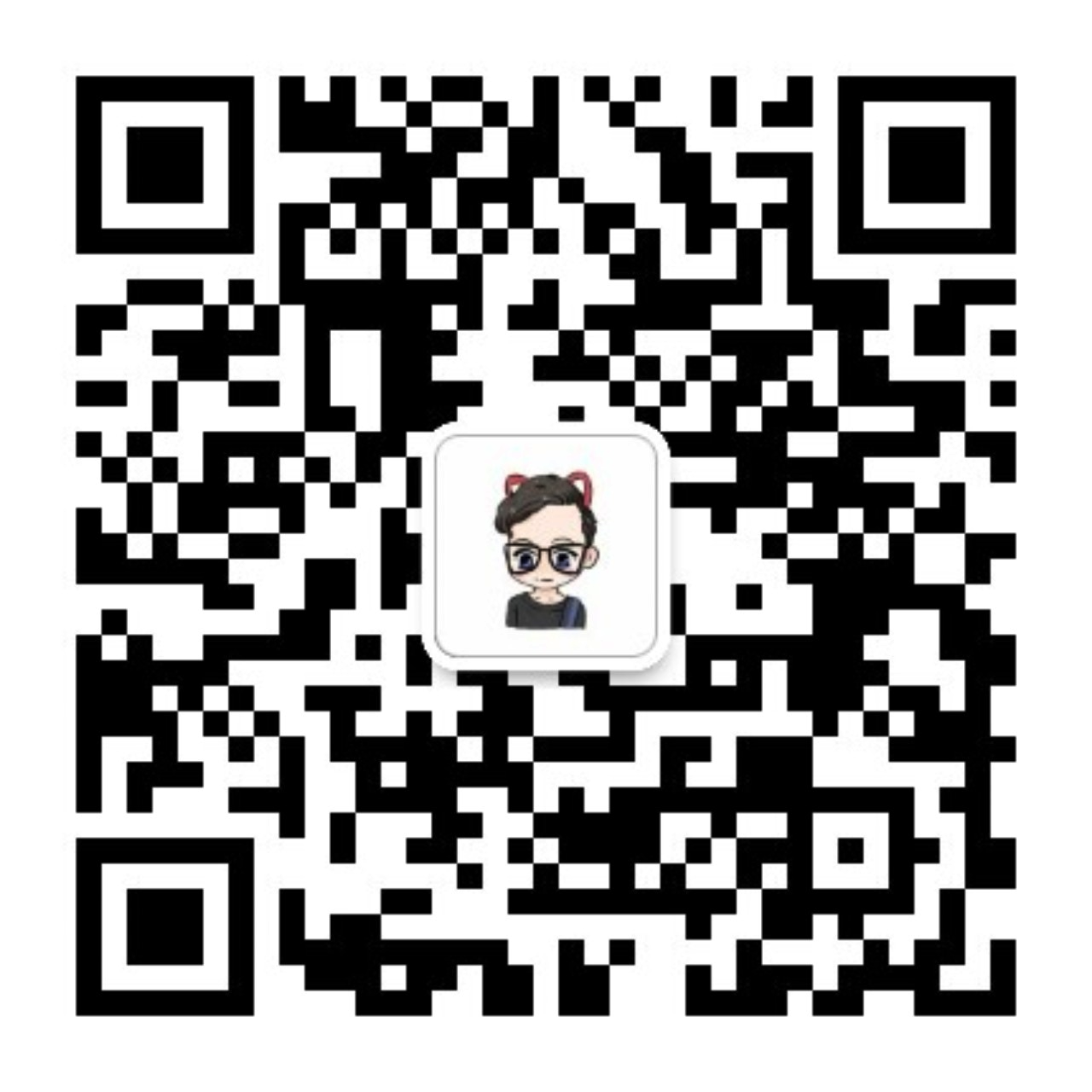