1 Map interface
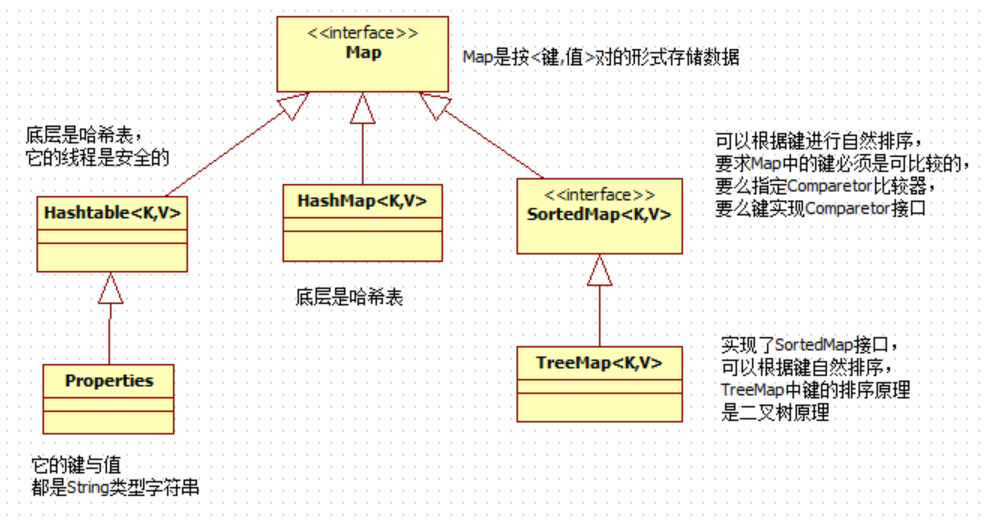
Map and Collection have no inheritance relationship
The Map collection stores data in the form of key and value: key value pairs
Both key and value are reference data types.
Both key and value are memory addresses of storage objects.
Key plays a leading role, and value is an accessory of key.
Common methods in Map interface:
V put(K key,V value); Adds a key value pair to the Map collection
V get(Object key); Get value through Key
void clear(); Empty Map collection
boolean containsKey(Object key); Judge whether the Map contains a key
boolean containsValue(Object value); Judge whether a value is included in the Map
boolean isEmpty(); Judge whether the number of elements in the Map collection is 0
Set keySet(); Get all keys of the Map set (all keys are a set set set)
V remove(Object key); Delete key value pairs through key
int size(); Gets the number of key value pairs in the Map collection
Collection values(); Get all values in the Map collection and return a collection
Set<Map.Entry<K,V>> entrySet(); Convert map set to set set set
import java.util.Collection; import java.util.HashMap; import java.util.Map; public class MapTest01 { public static void main(String[] args) { //Create a Map collection object Map<Integer, String> map = new HashMap<>(); //Adds a key value pair to the Map collection map.put(1, "jack"); map.put(2, "rose"); map.put(3, "Tom"); map.put(4, "Lee"); //Get value through key System.out.println(map.get(2));//rose //Gets the number of key value pairs System.out.println(map.size());//4 //Delete key value through key map.remove(2); System.out.println(map.size());//3 //Determine whether a key is included //The equals method is called at the bottom of the contains method System.out.println(map.containsKey(3));//true //Determine whether a value is included System.out.println(map.containsValue("Lee"));//true //Empty Map collection // map.clear(); // System.out.println(map.size());//0 // //Determine whether the collection is empty // System.out.println(map.isEmpty());//true //Get all value s in the map set Collection<String> c = map.values(); for (String s : c) { System.out.println(s); } } }
Traversal of Map collection:
The first method: get all the keys and traverse the value by traversing the key.
import java.util.HashMap; import java.util.Iterator; import java.util.Map; import java.util.Set; public class MapTest02 { public static void main(String[] args) { Map<Integer, String> map = new HashMap<>(); //Adds a key value pair to the Map collection map.put(1, "jack"); map.put(2, "rose"); map.put(3, "Tom"); map.put(4, "Lee"); //Get all keys. All keys are a Set set Set<Integer> keys = map.keySet(); Iterator<Integer> it = keys.iterator(); while (it.hasNext()) { //Take out one of the key s Integer key = it.next(); //Get value through key String value = map.get(key); System.out.println(key + "=" + value); } System.out.println("----------------"); //foreach to traverse the collection for (Integer key : keys) { System.out.println(key + "=" + map.get(key)); } } }
The second method: set < map, entry < K, V > > entryset ()
This method is to directly convert all Map sets into Set sets. The type of elements in Set sets is Map.Entry
import java.util.HashMap; import java.util.Iterator; import java.util.Map; import java.util.Set; public class MapTest03 { public static void main(String[] args) { Map<Integer, String> map = new HashMap<>(); //Adds a key value pair to the Map collection map.put(1, "jack"); map.put(2, "rose"); map.put(3, "Tom"); map.put(4, "Lee"); Set<Map.Entry<Integer, String>> set = map.entrySet(); //Traverse the set set, one Node at a time //iterator Iterator<Map.Entry<Integer, String>> it = set.iterator(); while (it.hasNext()) { Map.Entry<Integer, String> node = it.next(); Integer key = node.getKey(); String value = (String) node.getValue(); System.out.println(key + "=" + value); } System.out.println("------------------"); //foreach traversal for (Map.Entry<Integer, String> node : set) { System.out.println(node.getKey() + "=" + node.getValue()); } } }
1.1 HashMap set
At the bottom of the HashMap set is the data structure of the hash table / hash table.
The initial capacity of HashMap set is 16, and the loading factor is 0.75 (the capacity will be expanded when the capacity reaches 75%).
HashMap is easy to initialize. It must be a multiple of 2. It is officially recommended.
What kind of data structure is a hash table?
Hash table is a combination of array and one-way linked list.
Array: high efficiency in query, low efficiency in random addition and deletion.
Single Necklace table: it has high efficiency in random addition and deletion and low efficiency in query.
Hash table combines the above two data structures to give full play to their respective advantages.
Hash table / hash table: a one-dimensional array in which each element is a one-way linked list( Array and linked list)
HashMap's kye partial features: disordered and unrepeatable.
Disorder: because it may not be linked to a one-way linked list.
Non repeatable: the equals method is used to ensure that the key of the HashMap set is non repeatable. If the key is repeated, the value will be overwritten.
import java.util.HashMap; import java.util.Iterator; import java.util.Map; import java.util.Set; public class HashMapTest01 { public static void main(String[] args) { Map<Integer, String> map = new HashMap<>(); map.put(1, "jack"); map.put(2, "rose"); map.put(2, "Tom"); map.put(3, "Lee"); map.put(4, "hello"); System.out.println(map.size());//4 Set<Map.Entry<Integer, String>> set = map.entrySet(); Iterator<Map.Entry<Integer, String>> it = set.iterator(); while (it.hasNext()) { Map.Entry<Integer, String> node = it.next(); Integer key = node.getKey(); String value = node.getValue(); System.out.println(key + "=" + value); } } }
Operation results:
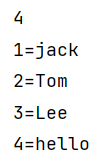
The HashMap set key and value can be empty:
import java.util.HashMap; import java.util.Map; public class HashMapTest03 { public static void main(String[] args) { Map map = new HashMap(); //The HashMap collection allows the key to be empty map.put(null, null); System.out.println(map.size());//1 //key repeat value override map.put(null, 100); System.out.println(map.size());//1 //Get value through jey System.out.println(map.get(null));//100 } }
1.1.1 HashMap collection operation
import java.util.HashMap; import java.util.Map; import java.util.Set; public class HashMapTest { public static void main(String[] args) { //Create a HashMap collection object Map<Integer, String> map = new HashMap<>(); //Add element map.put(11, "hello"); map.put(10, "world"); map.put(18, "java"); map.put(0, "okok"); //key same value override map.put(10, "rose"); //Take element System.out.println(map.get(0));//okok //Traversal set //Get the key first, and then get the valu e through the key Set<Integer> keys = map.keySet(); for (Integer i : keys) { System.out.println(i + "=" + map.get(i)); } //All map sets are transformed into Set sets, and each element in the Set set is a Node Set<Map.Entry<Integer, String>> set = map.entrySet(); for (Map.Entry<Integer, String> ss : set) { System.out.println(ss.getKey() + "=" + ss.getValue()); } } }
1.2 Properties collection
At present, you only need to master the relevant methods of the Properties property class object.
Peoperties is a Map collection that inherits Hashtable. The key and value of Properties are of String type.
Properties are called property class objects.
Properties are thread safe.
import java.util.Properties; public class PropertiesTest01 { public static void main(String[] args) { //Create a Properties object Properties pro = new Properties(); //There are two methods of Properties, one for saving and one for fetching pro.setProperty("a", "jack"); pro.setProperty("b", "rose"); pro.setProperty("hello", "world"); //Get value through key System.out.println(pro.getProperty("a"));//jack System.out.println(pro.getProperty("b"));//rose System.out.println(pro.getProperty("hello"));//world } }
1.3 TreeMap set
treeMap can sort the keys in the map. If the keys in the map use self-defined classes, you need to implement the Comaprable or Comparator interface to complete the sorting.
import java.util.Comparator; import java.util.Map; import java.util.Set; import java.util.TreeMap; /** * Rewrite comparator, descending */ public class TreeMapTest { public static void main(String[] args) { Map<Integer, String> map = new TreeMap<Integer, String>(new Comparator<Integer>() { @Override public int compare(Integer o1, Integer o2) { return o2 - o1; } }); map.put(1001, "jack"); map.put(1003, "zhansan"); map.put(1002, "aangwu"); map.put(100, "abcd"); map.put(1007, "hello"); //Get the key and traverse the key to get the value Set<Integer> keys = map.keySet(); for (Integer i : keys) { System.out.println(i + "=" + map.get(i)); } //Convert all map sets into Set sets Set<Map.Entry<Integer, String>> set = map.entrySet(); for (Map.Entry<Integer, String> ss : set) { System.out.println(ss.getKey() + "=" + ss.getValue()); } } }