The following is reproduced from iOS Engineer Genosage The article "Map SDK Series Tutorials - Showing Designated Areas on Maps"
Authors: Genosage
Links: https://juejin.im/post/5d721a29f265da03970bdc8d
Source: Nuggets
Copyright belongs to the author. For commercial reprints, please contact the author for authorization. For non-commercial reprints, please indicate the source.
Map SDK Series Tutorials - Display designated areas on a map
In the process of using Tencent iOS Map SDK, we often encounter scenarios that require maps to show designated areas. I believe you will encounter similar situations. Map SDK provides many interfaces related to it. This article will integrate these interfaces and provide sample codes to realize the display and assignment in multiple scenarios. Regional needs.
It should be noted that this article is suitable for scenarios where no rotation or elevation occurs on the map.
Download Tencent iOS Map SDK, please go to: iOS Map SDK
The specified area contains a single coordinate point
Displaying a fixed coordinate point on a map is one of the most basic functions of SDK.
For example, we get the coordinates of Tiantan Park (39.881190, 116.410490) according to the search function of SDK. Next, we can make the map display this coordinate by setting the center coordinate of the map. At the same time, we can also set zoom Level to specify the zoom level:
// Setting the Center Point self.mapView.centerCoordinate = CLLocationCoordinate2DMake(39.881190,116.410490); // Setting Scale Level self.mapView.zoomLevel = 15;
The results are as follows:
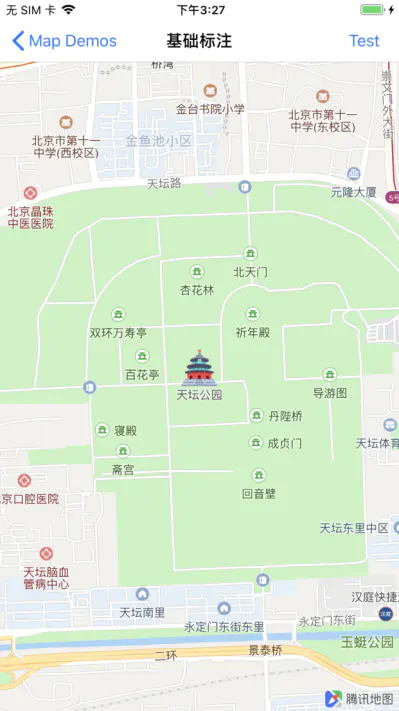
If you want to show the Mercator coordinate point QMapPoint, you need to convert the Mercator coordinate to latitude and longitude through the method QCoordinateForMapPoint (QMapPoint map Point).
The specified area contains multiple coordinate points
Now, if we want to display the search results of Tiantan Park on the map, how should we achieve it?
Firstly, we search Tiantan Park by searching for the first nine coordinate points of search results. Next, we should make our map view contain these nine coordinate points. Map SDK provides the method of QBounding Coordination Regions WithCoordinates (CLLocation Coordination 2D*coordinates, NSUInteger count) to calculate more. After the minimum outer rectangle of the longitude and latitude coordinate points is obtained, we can directly set the region of the map to show the area we want. The complete code is as follows:
CLLocationCoordinate2D coordinates[9]; // Search Result Coordinates of Tiantan Park coordinates[0] = CLLocationCoordinate2DMake(39.881190,116.410490); coordinates[1] = CLLocationCoordinate2DMake(39.883247,116.400063); coordinates[2] = CLLocationCoordinate2DMake(39.883710,116.412900); coordinates[3] = CLLocationCoordinate2DMake(39.883654,116.412863); coordinates[4] = CLLocationCoordinate2DMake(39.883320,116.400040); coordinates[5] = CLLocationCoordinate2DMake(39.876980,116.413190); coordinates[6] = CLLocationCoordinate2DMake(39.878160,116.413140); coordinates[7] = CLLocationCoordinate2DMake(39.878980,116.407080); coordinates[8] = CLLocationCoordinate2DMake(39.878560,116.413160); // Computational area circumscribed rectangle QCoordinateRegion region = QBoundingCoordinateRegionWithCoordinates(coordinates, 9); // Setting area self.mapView.region = region;
The results are as follows:
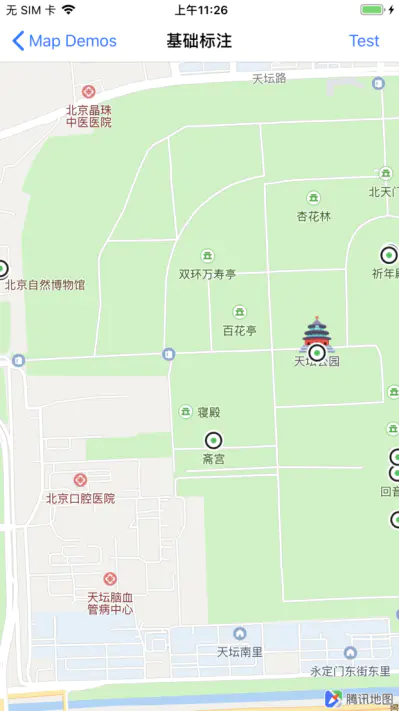
Designated central point
If we want to show the nine coordinate points and designate a coordinate point as the map center, we can use the method of QBounding Coordinate Region WithCoordinates AndCenter (CLLocation Coordination 2D*coordinates, NSUInteger count, CLLocation Coordination 2D Center Coordination) to calculate the smallest outer rectangle to the Temple of Heaven. For example, the central point of the park is as follows:
// Centerpoint coordinates CLLocationCoordinate2D centerCoordinate = CLLocationCoordinate2DMake(39.881190,116.410490); // Computing the Outer Rectangle of the Region Centered on the Center Point QCoordinateRegion region = QBoundingCoordinateRegionWithCoordinatesAndCenter(coordinates, 9, centerCoordinate); // Setting area self.mapView.region = region;
The results are as follows:
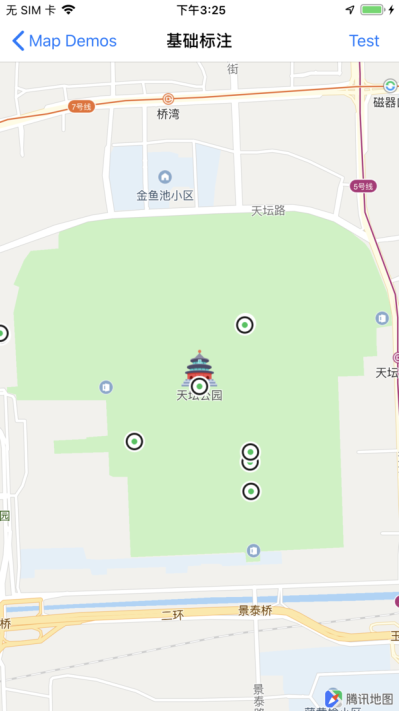
Embedded periphery boundary
If you need to embed some boundaries around the map field of view, you can use the method - (void) setRegion:(QCoordinateRegion) region edge Padding:(UIEdgeInsets) insets animated:(BOOL) animated to set the map area, the code is as follows:
// Computational area circumscribed rectangle QCoordinateRegion region = QBoundingCoordinateRegionWithCoordinates(coordinates, 9); // Setting up areas and boundaries [self.mapView setRegion:region edgePadding:UIEdgeInsetsMake(20, 20, 20, 20) animated:NO];
The results are as follows:
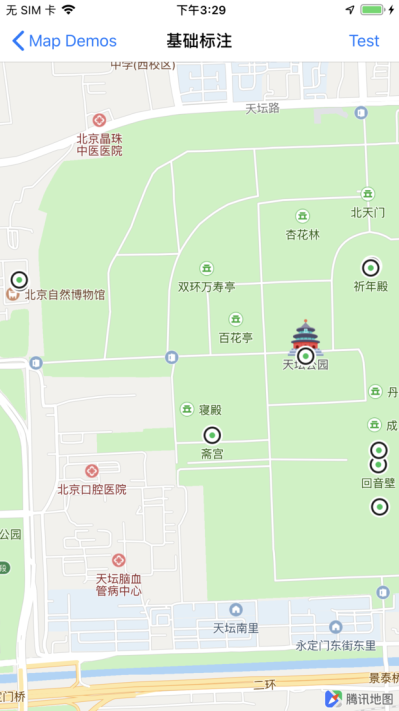
Mercator coordinate points
If you need to show multiple Mercator coordinate points, you can use QBoundingMapRectWithPoints(QMapPoint *points, NSUInteger count) and - (void) setVisibleMapRect:(QMapRect) mapRect Padding:(UIEdgeInsets) insets animated:(BOOL) animated, which are provided by the Map SDK.
Broken Lines and Covers
When we want to show the route or cover on the map, that is, QPolyline QPolygon and QCircle in the map SDK, we can get their properties boundingMapRect directly and then set them up.
The specified area contains multiple annotations
In many scenarios, we need to add annotations to the map and customize their images, as follows:
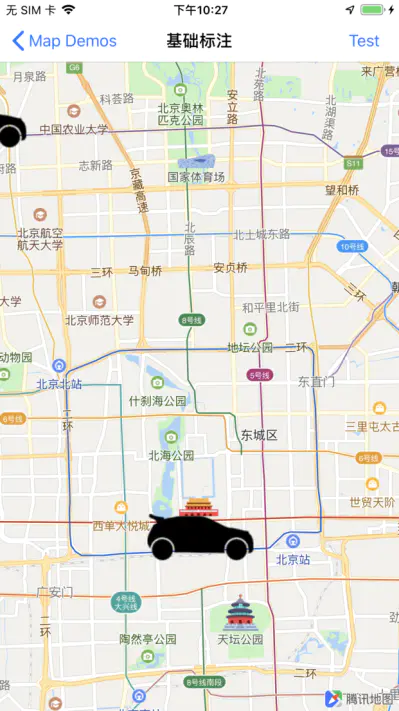
We can use the following code to make these annotations appear in the map field of view:
// Computational inclusion Annotation and MapRect Circumscribed rectangle QMapRect rect = [self.mapView mapRectThatFits:QMapRectNull containsCalloutView:NO annotations:self.annotations edgePadding:UIEdgeInsetsZero]; // Setting area self.mapView.visibleMapRect = rect;
The results are as follows:
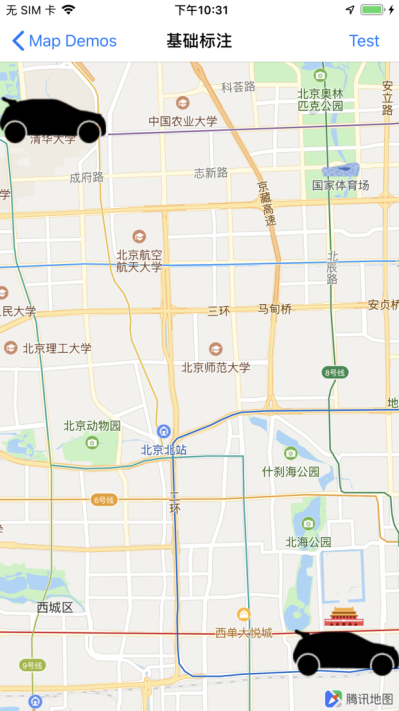
When the annotation shows Callout View, we can include Callout View in the map view by passing in the parameter bContainsCallout View as YES. The display effect is as follows:
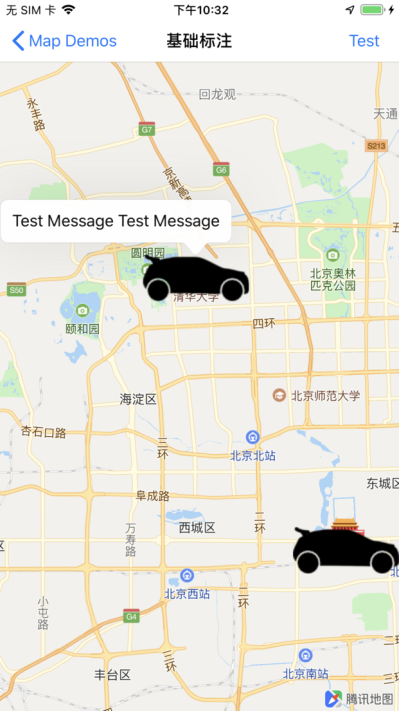
Sometimes we need to specify a region that contains both the current screen view and all the annotations. We can achieve the desired effect by passing in the first parameter, mapRect, as self.mapView.visibleMapRect.
Restrict the display of specified areas
When we need to restrict the view of the map so that it can only show the area we specified, for example, the Forbidden City can be set up by the following code:
CLLocationCoordinate2D coordinates[4]; // Longitudinal and latitudinal coordinates of four vertices of the Forbidden City area rectangle coordinates[0] = CLLocationCoordinate2DMake(39.922878,116.391547); coordinates[1] = CLLocationCoordinate2DMake(39.912917,116.392100); coordinates[2] = CLLocationCoordinate2DMake(39.913312,116.402507); coordinates[3] = CLLocationCoordinate2DMake(39.923277,116.402024); // Computational area circumscribed rectangle QCoordinateRegion region = QBoundingCoordinateRegionWithCoordinates(coordinates, 4); // Computational Planar Projection Rectangle QMapRect rect = QMapRectForCoordinateRegion(region); // Restricted display area [self.mapView setLimitMapRect:rect mode:QMapLimitRectFitWidth];
The results are as follows:
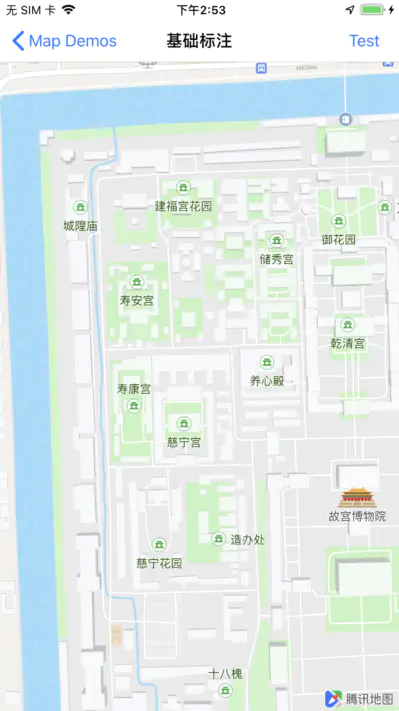