- Membership Card Management System mysql Database Creation Statement
- Member card management system oracle database creation statement
- Membership card management system SQL Server database creation statement
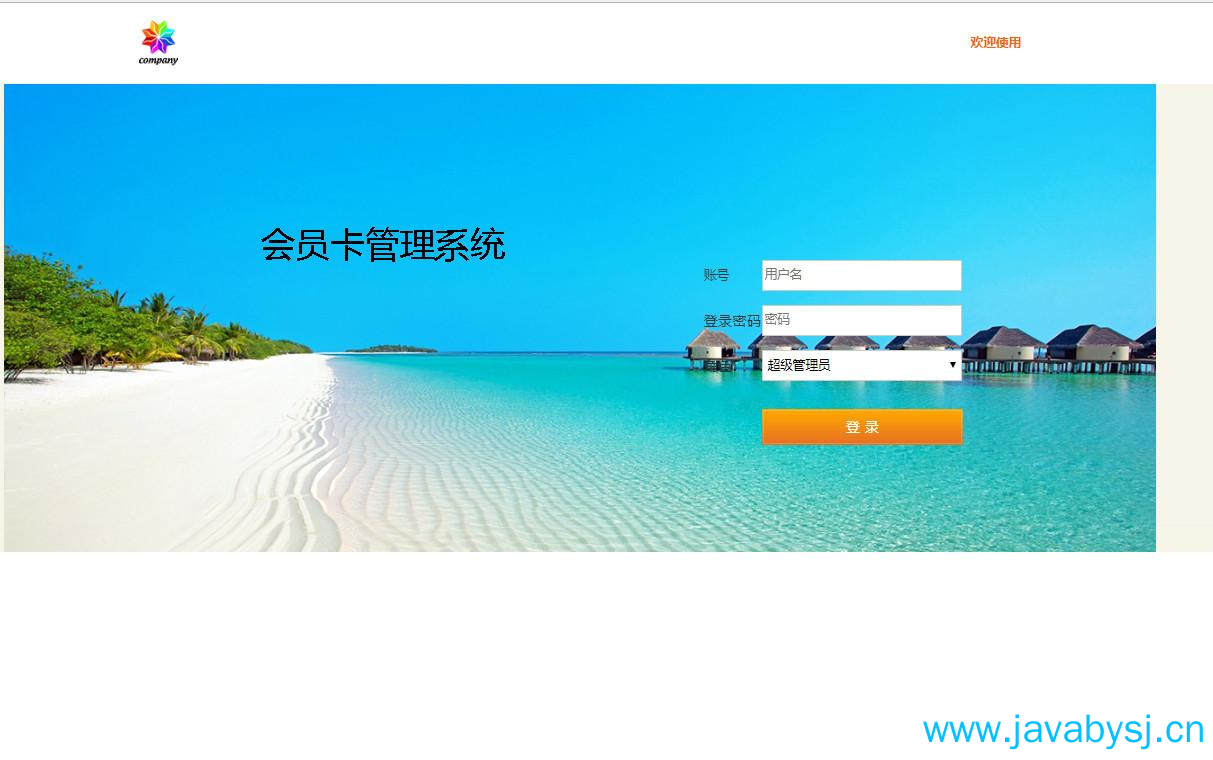
Membership card management system mysql database version source code:
Super Administrator Table Creation Statement is as follows:
create table t_admin( id int primary key auto_increment comment 'Primary key', username varchar(100) comment 'Super Administrator Account', password varchar(100) comment 'Super Administrator Password' ) comment 'Super Administrator'; insert into t_admin(username,password) values('admin','123456');
The rotation chart creation statement is as follows:
create table t_lbt( id int primary key auto_increment comment 'Primary key', types varchar(100) comment 'classification' ) comment 'Rotary Planting Map';
The message table creation statement is as follows:
create table t_xiaoxi( id int primary key auto_increment comment 'Primary key', scName varchar(100) comment 'Name', pic varchar(100) comment 'picture', content1 varchar(100) comment 'content', content2 varchar(100) comment 'Food Recommendation' ) comment 'news';
The collection table creation statement is as follows:
create table t_shoucang( id int primary key auto_increment comment 'Primary key', wdxxId int comment 'Comment information', customerId int comment 'Commentator', content varchar(100) comment 'Comments' ) comment 'Collection';
The bulletin table creation statement is as follows:
create table t_gg( id int primary key auto_increment comment 'Primary key', insertDate datetime comment 'Comment Date', pic varchar(100) comment 'picture', productId int comment 'product', customerId int comment 'user' ) comment 'Notice';
The comment table creation statement is as follows:
create table t_pinglun( id int primary key auto_increment comment 'Primary key', insertDate datetime comment 'date', customerId int comment 'user', title varchar(100) comment 'Title', content varchar(100) comment 'content' ) comment 'comment';
The table creation statement is as follows:
create table t_sc( id int primary key auto_increment comment 'Primary key', insertDate datetime comment 'date', v1 varchar(100) comment 'Title', pic varchar(100) comment 'picture', showDate datetime comment 'date', v3 varchar(100) comment 'content' ) comment 'Ingredients';
Membership card management system oracle database version source code:
Super Administrator Table Creation Statement is as follows:
create table t_admin( id integer, username varchar(100), password varchar(100) ); insert into t_admin(id,username,password) values(1,'admin','123456'); --Super Administrator Field Annotated comment on column t_admin.id is 'Primary key'; comment on column t_admin.username is 'Super Administrator Account'; comment on column t_admin.password is 'Super Administrator Password'; --Super Administrator Table Annotated comment on table t_admin is 'Super Administrator';
The rotation chart creation statement is as follows:
create table t_lbt( id integer, types varchar(100) ); --Annotation of Roadcasting Map Fields comment on column t_lbt.id is 'Primary key'; comment on column t_lbt.types is 'classification'; --Annotation of Rotary Planting Charts comment on table t_lbt is 'Rotary Planting Map';
The message table creation statement is as follows:
create table t_xiaoxi( id integer, scName varchar(100), pic varchar(100), content1 varchar(100), content2 varchar(100) ); --Message field annotated comment on column t_xiaoxi.id is 'Primary key'; comment on column t_xiaoxi.scName is 'Name'; comment on column t_xiaoxi.pic is 'picture'; comment on column t_xiaoxi.content1 is 'content'; comment on column t_xiaoxi.content2 is 'Food Recommendation'; --Message table annotated comment on table t_xiaoxi is 'news';
The collection table creation statement is as follows:
create table t_shoucang( id integer, wdxxId int, customerId int, content varchar(100) ); --Collection field annotated comment on column t_shoucang.id is 'Primary key'; comment on column t_shoucang.wdxxId is 'Comment information'; comment on column t_shoucang.customerId is 'Commentator'; comment on column t_shoucang.content is 'Comments'; --Notes on Collection Table comment on table t_shoucang is 'Collection';
The bulletin table creation statement is as follows:
create table t_gg( id integer, insertDate datetime, pic varchar(100), productId int, customerId int ); --Annotation of bulletin field comment on column t_gg.id is 'Primary key'; comment on column t_gg.insertDate is 'Comment Date'; comment on column t_gg.pic is 'picture'; comment on column t_gg.productId is 'product'; comment on column t_gg.customerId is 'user'; --Annotation of bulletin sheet comment on table t_gg is 'Notice';
The comment table creation statement is as follows:
create table t_pinglun( id integer, insertDate datetime, customerId int, title varchar(100), content varchar(100) ); --Comment fields with comments comment on column t_pinglun.id is 'Primary key'; comment on column t_pinglun.insertDate is 'date'; comment on column t_pinglun.customerId is 'user'; comment on column t_pinglun.title is 'Title'; comment on column t_pinglun.content is 'content'; --Comments comment on table t_pinglun is 'comment';
The table creation statement is as follows:
create table t_sc( id integer, insertDate datetime, v1 varchar(100), pic varchar(100), showDate datetime, v3 varchar(100) ); --Annotation of Food Material Field comment on column t_sc.id is 'Primary key'; comment on column t_sc.insertDate is 'date'; comment on column t_sc.v1 is 'Title'; comment on column t_sc.pic is 'picture'; comment on column t_sc.showDate is 'date'; comment on column t_sc.v3 is 'content'; --Notes on the table of ingredients comment on table t_sc is 'Ingredients';
oracle is unique, and the corresponding sequence is as follows:
create sequence s_t_lbt; create sequence s_t_xiaoxi; create sequence s_t_shoucang; create sequence s_t_gg; create sequence s_t_pinglun; create sequence s_t_sc;
Membership card management system sqlserver database version source code:
Super Administrator Table Creation Statement is as follows:
--Super Administrator create table t_admin( id int identity(1,1) primary key not null,--Primary key username varchar(100),--Super Administrator Account password varchar(100)--Super Administrator Password ); insert into t_admin(username,password) values('admin','123456');
The rotation chart creation statement is as follows:
--Annotation of Rotary Planting Charts create table t_lbt( id int identity(1,1) primary key not null,--Primary key types varchar(100)--classification );
The message table creation statement is as follows:
--Message table annotations create table t_xiaoxi( id int identity(1,1) primary key not null,--Primary key scName varchar(100),--Name pic varchar(100),--picture content1 varchar(100),--content content2 varchar(100)--Food Recommendation );
The collection table creation statement is as follows:
--Notes to Collection Table create table t_shoucang( id int identity(1,1) primary key not null,--Primary key wdxxId int,--Comment information customerId int,--Commentator content varchar(100)--Comments );
The bulletin table creation statement is as follows:
--Annotations to bulletin tables create table t_gg( id int identity(1,1) primary key not null,--Primary key insertDate datetime,--Comment Date pic varchar(100),--picture productId int,--product customerId int--user );
The comment table creation statement is as follows:
--Comments create table t_pinglun( id int identity(1,1) primary key not null,--Primary key insertDate datetime,--date customerId int,--user title varchar(100),--Title content varchar(100)--content );
The table creation statement is as follows:
--Notes on Food Material List create table t_sc( id int identity(1,1) primary key not null,--Primary key insertDate datetime,--date v1 varchar(100),--Title pic varchar(100),--picture showDate datetime,--date v3 varchar(100)--content );