Introduction to multitasking
1. Multitasking execution
- Concurrent
- parallel
Concurrency: alternating tasks over a period of time.
Parallel: for multi-core cpu processing multi tasks, the operating system will arrange an executing software for each core of the cpu, and multiple cores really execute software together. It should be noted here that multi-core CPUs execute multiple tasks in parallel, and multiple software are always executed together.
If the number of tasks is greater than the number of cores of the cpu, it means that multiple tasks are executed concurrently
If the number of tasks is less than or equal to the number of cpu cores, it means that multiple tasks are executed in parallel
2. Summary
- Using multitasking can make full use of CPU resources, improve the execution efficiency of the program, and make your program have the ability to handle multiple tasks.
- There are two ways to execute multitasking: concurrency and parallelism. Here, parallelism is the real meaning of executing multiple tasks together.
process
1. Introduction to process
In Python programs, you can use processes to achieve multitasking. Processes are a way to achieve multitasking.
2. Concept of process
An ongoing program or software is a process. It is the basic unit for the operating system to allocate resources. That is, every time a process is started, the operating system will allocate certain running resources (memory resources) to ensure the operation of the process.
For example, in real life, the company can be understood as a process. The company provides office resources (computers, office desks and chairs, etc.), and the employees really work. Employees can be understood as threads.
be careful:
A program has at least one process running. A process has one thread by default. Multiple threads can be created in the process. Threads depend on the child process. If there is no process, there will be no thread.
3. Role of process
Single process rendering:
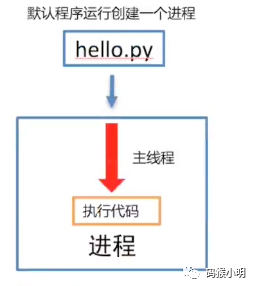
Multi process rendering:
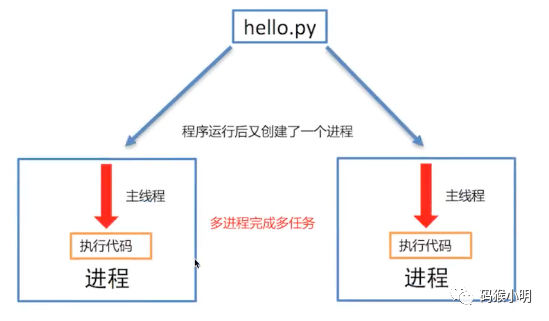
4. Summary
- Process is the basic unit of resource allocation in the operating system
- Process is a way to implement multitasking in Python programs
Use of multiple processes
1. Import process package
#Import process package import multiprocessing
2. Description of the Process class
Proces([group[,targt[,name[,args[,kwargs]]]]])
- Group: Specifies the process group. Currently, only None can be used
- Target: the name of the target task to be executed
- Name: process name
- args: pass parameters to the execution task in tuple mode
- kwargs: pass parameters to the execution task in dictionary mode
Common methods for instance objects created by Process:
- start(): start the child process instance (create child process)
- join(): wait for the execution of the child process to end
- terminate(): terminate the child process immediately regardless of whether the task is completed or not
Common properties of instance objects created by Process:
name: when the alias of a process, the default is Process- N, and N is an integer incremented from 1
Get process number
1. Purpose of obtaining process number
The purpose of obtaining the process number is to verify the relationship between the main process and the sub process, and you can know which main process created the sub process.
There are two operations to get the process number:
- Get current process number
- Gets the current parent process number
2. Get current process number
os.getpid() means to get the current process number
Code demonstration:
import multiprocessing import time import os # Dance task def dance(): # Gets the number of the current process print("dance", os.getpid()) # Get current process print("dance", multiprocessing.current_process()) for i in range(5): print("Dancing···") time.sleep(0.2) # Extension: kill the specified process according to the process number os.kill(os.getpid(), 9) # Singing task def sing(): # Gets the number of the current process print("sing", os.getpid()) # Get current process print("sing", multiprocessing.current_process()) for i in range(5): print("Singing···") time.sleep(0.2) if __name__ == '__main__': # Get current process number print("main", os.getpid()) # Get current process print("main", multiprocessing.current_process()) # Create a child process for dancing # Group: indicates the process group. At present, only None can be used # Target: indicates the name of the target task to be executed (function name, method name) # Name: process name. The default is process - N. n is incremented from 1 dance_process = multiprocessing.Process(target=dance, name="myprocess1") sing_process = multiprocessing.Process(target=sing) # Start the child process to execute the corresponding task dance_process.start() sing_process.start()
3. Gets the current parent process number
os.getppid() means to get the current parent process number
Code demonstration:
# -*- codeing = utf-8 -*- # @Time: 10:51 pm on December 4, 2021 # @Author: Li Minghui # @File : ithui_ Multi process py # @Software : PyCharm import multiprocessing import time import os # Dance task def dance(): # Gets the number of the current process print("dance", os.getpid()) # Get current process print("dance", multiprocessing.current_process()) # Get the current process object and see which process executes the current code. Multiprocessing current_ process() print('main_process_id', os.getpid(), multiprocessing.current_process()) # Gets the number of the parent process print("dance", os.getppid()) for i in range(5): print("Dancing···") time.sleep(0.2) # Extension: kill the specified process according to the process number os.kill(os.getpid(), 9) # Singing task def sing(): # Gets the number of the current process print("sing", os.getpid()) # Get current process print("sing", multiprocessing.current_process()) print('main_process_id', os.getpid(), multiprocessing.current_process()) # Gets the number of the parent process print("sing", os.getppid()) for i in range(5): print("Singing···") time.sleep(0.2) if __name__ == '__main__': # Get current process number print("main", os.getpid()) # Get current process print("main", multiprocessing.current_process()) # Create a child process for dancing # Group: indicates the process group. At present, only None can be used # Target: indicates the name of the target task to be executed (function name, method name) # Name: process name. The default is process - N. n is incremented from 1 dance_process = multiprocessing.Process(target=dance, name="myprocess1") sing_process = multiprocessing.Process(target=sing) # Start the child process to execute the corresponding task dance_process.start() sing_process.start()
Introduction to the process executing tasks with parameters
1. Introduction to the process executing tasks with parameters
There are two ways for the Process class to execute tasks and transfer parameters:
- args indicates that parameters are passed to the execution task as tuples
- kwargs means to pass parameters to the execution task in dictionary mode
2. Use of args parameter
Code demonstration:
# -*- codeing = utf-8 -*- # @Time: 11:27 pm on December 4, 2021 # @Author: Li Minghui # @File : ithui_ Process tasks with parameters py # @Software : PyCharm import multiprocessing import time # Task to display information def show_info(name, age): print(name, age) # Create child process # 1. Pass parameters as tuples sub_process = multiprocessing.Process(target=show_info, args=("Li Si", 20)) # 2. Pass parameters in dictionary # sub_process = multiprocessing.Process(target=show_info, kwargs={"name": "Li Si", "age":20}) # 3. Pass parameters with tuples and dictionaries # sub_process = multiprocessing.Process(target=show_info, args = ("Li Si",), kwargs={"age":20}) # Start process if __name__ == '__main__': sub_process.start()
3. Use of kwargs parameter
Code demonstration:
# -*- codeing = utf-8 -*- # @Time: 11:27 pm on December 4, 2021 # @Author: Li Minghui # @File : ithui_ Process tasks with parameters py # @Software : PyCharm import multiprocessing import time # Task to display information def show_info(name, age): print(name, age) # Create child process # 1. Pass parameters as tuples # sub_process = multiprocessing.Process(target=show_info, args = ("Li Si", 20)) # 2. Pass reference by dictionary sub_process = multiprocessing.Process(target=show_info, kwargs={"name":"Li Si", "age":20}) # 3. Pass parameters with tuples and dictionaries # sub_process = multiprocessing.Process(target=show_info, args = ("Li Si",), kwargs={"age":20}) # Start process if __name__ == '__main__': sub_process.start() 4,args and kwargs Use together Code demonstration: # -*- codeing = utf-8 -*- # @Time: 11:27 pm on December 4, 2021 # @Author: Li Minghui # @File : ithui_ Process tasks with parameters py # @Software : PyCharm import multiprocessing import time # Task to display information def show_info(name, age): print(name, age) # Create child process # 1. Pass parameters as tuples # sub_process = multiprocessing.Process(target=show_info, args = ("Li Si", 20)) # 2. Pass parameters in dictionary # sub_process = multiprocessing.Process(target=show_info, kwargs={"name": "Li Si", "age":20}) # 3. Pass parameters with tuples and dictionaries sub_process = multiprocessing.Process(target=show_info, args=("Li Si",), kwargs={"age":20}) # Start process if __name__ == '__main__': sub_process.start()
Process considerations
1. Introduction to process considerations
- Global variables are not shared between processes
- The main process will wait until the execution of all child processes is completed
2. Global variables are not shared between processes
Code demonstration:
# -*- codeing = utf-8 -*- # @Time: 10:09 PM, December 6, 2021 # @Author: Li Minghui # @File : ithui_ Global variables are not shared between processes py # @Software : PyCharm import multiprocessing import time # Define global variables g_list = list() # Task of adding data def add_data(): for i in range(3): g_list.append(i) print("add:", i) time.sleep(0.2) print("Add complete", g_list) # Task of reading data def read_data(): print("read:", g_list) # Child process for adding data add_process = multiprocessing.Process(target=add_data) # Child process reading data read_process = multiprocessing.Process(target=read_data) if __name__ == '__main__': # Start the process and execute the corresponding task add_process.start() # The current process (main process) waits for the execution of the process adding data to complete, and then the code continues to execute add_process.join() read_process.start()
Execution results:
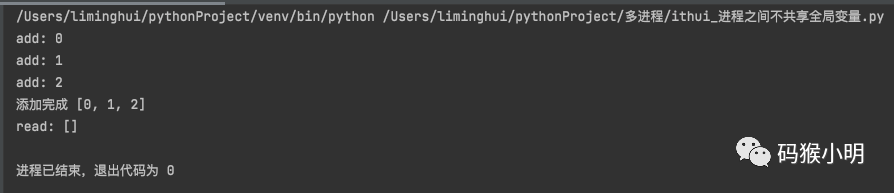
Creating a child process is just a copy of the main process resources. A child process is actually a copy of the main process.
3. The main process will wait until the execution of all child processes is completed
Code demonstration:
# -*- codeing = utf-8 -*- # @Time: 2021 / 12 / 6 10:57 PM # @Author: Li Minghui # @File : ithui_ The main process will wait for the execution of all child processes to end py # @Software : PyCharm import multiprocessing import time def task(): for i in range(10): print("Task execution...") time.sleep(0.2) # Determine whether it is a direct execution module, program entry module if __name__ == '__main__': # Create child process sub_process = multiprocessing.Process(target=task) # Set the child process as the guardian main process, and then the main process exits and the child process is destroyed directly sub_process.daemon = True sub_process.start() # The main process is delayed by 0.5 seconds time.sleep(0.5) print("over")
END