Mybatis framework learning delay loading
Posted by theDog on Sun, 14 Jun 2020 11:24:43 +0200
Delay loading
- It is to load data when it is needed, and not to load data when it is not needed. Lazy loading is also called lazy loading
- Advantages: query from single table first, and then from associated table when necessary, which greatly improves database performance, because query from single table is faster than query from multiple tables.
- Disadvantages: only when the data is needed, the database query will be carried out. Thus, in mass data query, because the query work also consumes time, it may cause users to wait longer, resulting in a decline in user experience.
Implement delayed loading
- association and collection have the function of delayed loading.
Delay loading using allocation
- DAO interface of account persistence layer
- Persistent layer mapping file of account
- Select: fill in the id of the select map we want to call
- column: fill in the parameters we want to pass to the select Map
<resultMap id="AccountU" type="com.lwb.domain.Account">
<!--Establish correspondence-->
<id property="id" column="aid"></id>
<result column="uid" property="uid"/>
<result column="money" property="money"/>
<!--It is used to specify the reference entity property from the table side-->
<association property="user" javaType="com.lwb.domain.User" select="com.lwb.dao.IUserDao.findById" column="uid">
</association>
</resultMap>
- User's persistence layer interface and mapping file

- Enable the delayed loading policy of Mybatis
SqlMapConifg.xml
- test
- Use lazy loading, when data is not used
@Test
public void test11()throws Exception {
List<Account> accounts = accountDao.findAll();
}

@Test
public void test11()throws Exception {
List<Account> accounts = accountDao.findAll();
for (Account account:accounts) {
System.out.println(account.getUser());
}
}
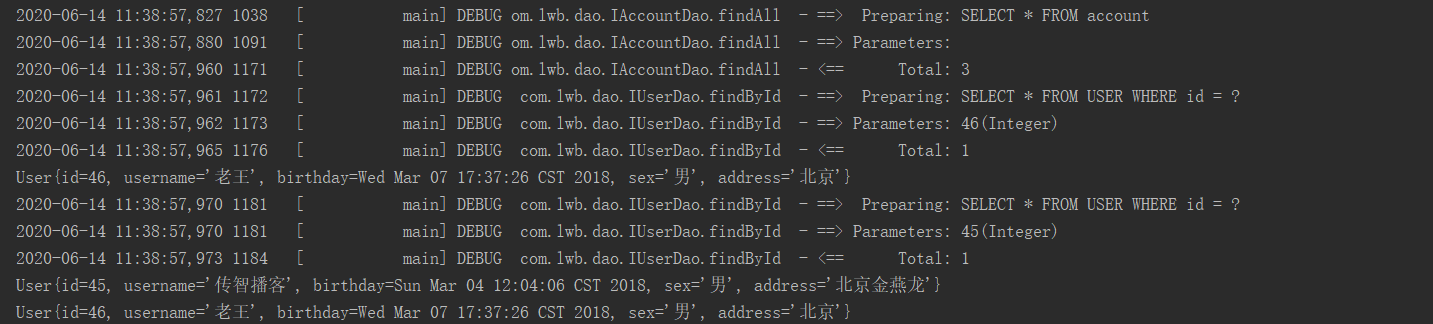
Using Collection to delay loading
- We can also configure the delayed loading policy in the < Collection > node of one to many relationship configuration.
The < Collection > node also has the select attribute and column attribute.
- Add the list < account > attribute to the User entity class
- Method of writing interface between user and account persistence layer
List<User> findAll();
List<Account> findByUid(Integer uid);
- Write user persistence layer mapping configuration
- Collection is used to establish the correspondence between collection properties in one to many
- ofType specifies the data type of the collection element
- select is the unique ID used to specify the query account (dao fully qualified class name of account plus method name)
- column is used to specify which field's value is used as the condition query
<resultMap id="UserA" type="com.lwb.domain.User">
<id property="id" column="id"></id>
<result column="username" property="username"/>
<result column="sex" property="sex"/>
<result column="birthday" property="birthday"/>
<result column="address" property="address"/>
<collection property="accounts" ofType="com.lwb.domain.Account" select="com.lwb.dao.IAccountDao.findByUid" column="id">
</collection>
</resultMap>
<select id="findAll" resultMap="UserA">
select * from USER
</select>
- Write account persistence layer mapping configuration
- test
@Test
public void test12()throws Exception {
List<User> users = dao.findAll();
}

@Test
public void test12()throws Exception {
List<User> users = dao.findAll();
for (User user:users){
System.out.println(user+" "+user.getAccounts());
}
}
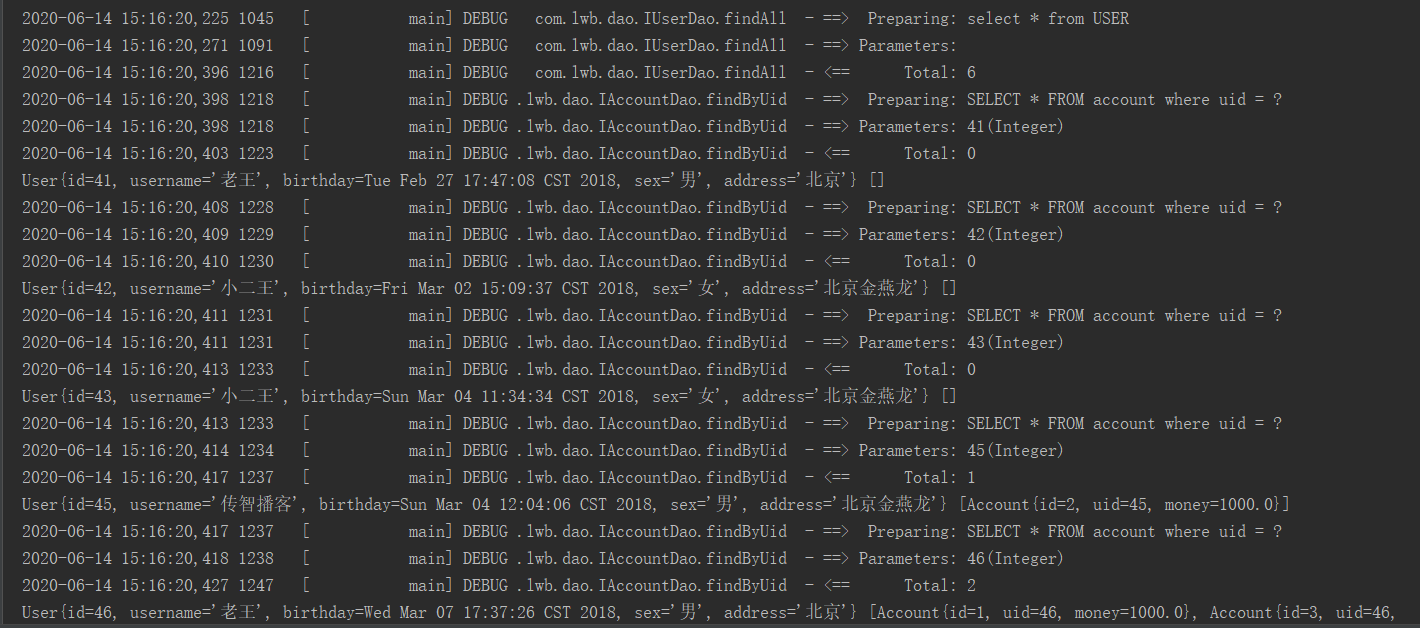
Topics:
Attribute
Database
Mybatis
xml