1 Preface
In daily development work, We often need to build the persistent object (PO) corresponding to various data tables and the interface for operating the database (DAO) and the corresponding XML bound to Dao. These operations are repetitive and do not require much technical content. At this time, we can't help but wonder whether there is a tool that can help us automatically generate these files? The answer is: Yes!
The following content of this article is mainly applicable to the development of persistence layer framework using MyBatis. If you don't use MyBatis, this article may not be suitable for your development scenario.
Introduction to 2MyBatis Generator
As an independent tool based on MyBatis, MyBatis Generator can meet the above requirements. It can help us generate PO, DAO, XML and other files corresponding to the data table through simple configuration, minus the time we manually generate these files, so as to effectively improve the development efficiency. MyBatis Generator runs in various ways, mainly through the following ways:
- command line
- Ant
- Maven
- Java
- Eclipse
I usually configure and use it in maven, so this article mainly explains it based on Maven environment.
3 preparation
Introducing plug-ins
Since you want to use MyBatis Generator, you must have configured the database and related dependencies of MyBatis in our project. If not, you can use POM XML file, mainly taking MySQL database as an example.
<dependencies> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> </dependency> <dependency> <groupId>org.mybatis.spring.boot</groupId> <artifactId>mybatis-spring-boot-starter</artifactId> </dependency> </dependencies>
Next, we continue to introduce the related configuration of MyBatis Generator.
<build> <plugins> <plugin> <groupId>org.mybatis.generator</groupId> <artifactId>mybatis-generator-maven-plugin</artifactId> <version>1.4.0</version> </plugin> </plugins> </build>
Plug in configuration
After completing the above steps, we just completed the introduction of MyBatis Generator. To make it work normally, we also need to configure it, and MyBatis Generator is in POM The main configurations in XML are as follows.
- The path to the configuration file for the code generator
Here, we mainly configure the path where the MyBatis Generator configuration file is located. Generally, we put it in the resources path, and the name of the configuration file can be customized. Here, I use MyBatis Generator config XML as an example, you need to add the following configuration to POM XML file.
<configuration> <configurationFile>src/main/resources/mybatis-generator-config.xml</configurationFile> </configuration>

- Overwrite generated files after each new build
Due to the project requirements, it is assumed that new fields need to be added in our database table, and we have used MyBatis Generator to generate relevant files before. At this time, if we want to add the newly added fields to the original generated file, the first way is to manually delete the old file and then regenerate it. The second is to configure in MyBatis Generator so that each newly generated file directly overwrites the old file. The specific configuration is as follows: true means to overwrite, and false means not to overwrite.
<configuration> <overwrite>true</overwrite> </configuration>
However, it should be noted that even if we set overwrite old files, MyBatis Generator will only overwrite the original PO and DAO files. At this time, Mapper will not be overwritten, but will be appended to ensure that the sql statements we add will not be overwritten.
- Database driven dependency
Although in the POM of the project We have configured the database dependencies in the XML file, but we still need to configure them again in the MyBatis Generator configuration. At this point, there are two ways for us to choose.
The first is to introduce database dependency again. The specific configuration method is as follows:
<build> <plugins> <plugin> <groupId>org.mybatis.generator</groupId> <artifactId>mybatis-generator-maven-plugin</artifactId> <version>1.4.0</version> <dependencies> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>8.0.17</version> </dependency> </dependencies> </plugin> </plugins> </build>
The second is to use Maven's includeCompileDependencies attribute. Generally speaking, we must have introduced database dependencies in our project, so we can configure includeCompileDependencies at this time. The specific configuration method is as follows:
<configuration> <includeCompileDependencies>true</includeCompileDependencies> </configuration>
MyBatis Generator configuration
We have introduced MyBatis Generator in the above steps, and it is also in the project configuration file POM The path where the MyBatis Generator configuration file is located, whether to overwrite the file and database dependency configuration are configured in XML. Next, let's see how to configure MyBatis Generator and configure various details in our generated code.
- External profile
Generally, we need to import external files, which are mainly used to configure the project database to facilitate our subsequent settings. The method of importing external configuration files is also very simple. The specific configuration is as follows:
<generatorConfiguration> <!-- Import profile --> <properties resource="generator.properties"/> </generatorConfiguration>
- context configuration
In addition to external configuration, context is undoubtedly the most important configuration in MyBatis Generator. A specific example of context configuration is as follows:
<context id="myContext" targetRuntime="MyBatis3" defaultModelType="flat"> </context>
The meanings of each attribute are as follows:
- id: unique identification. It cannot be repeated. It can be customized according to our own preferences.
- defaultModelType: not required. There are two optional values. One is conditional, which is also the default value, and the other is flat, which is a common configuration, indicating that a table in the database generates a PO.
- targetRuntime: not required. There are also two optional values here, one is MyBatis3 and the other is MyBatis3Simple. The main difference between the two is that the DAO and Mapper generated under different configurations will be different. The DAO and Mapper generated by the latter will be much less and contain only the most commonly used ones.
In addition to the above configuration, there are many child elements to be configured, and the number and order of these child elements are specified. If they are not configured according to the given rules, errors will be caused. The common child elements and number are configured as follows (sorted from top to bottom according to the specified order):
Child element | Minimum number | Maximum number |
---|---|---|
property | 0 | N |
plugin | 0 | N |
commentGenerator | 0 | 1 |
jdbcConnection | ||
javaTypeResolver | 0 | 1 |
javaModelGenerator | 1 | N |
sqlMapGenerator | 0 | 1 |
javaClientGenerator | 0 | 1 |
table | 1 | N |
Next, we will explain the configuration of each sub element in turn.
context sub element configuration
- property
If we want to set the encoding type of our generated file, you can configure it here. The specific configuration is as follows:
<property name="javaFileEncoding" value="UTF-8"/>
- plugin
By default, the generated PO only contains each attribute declaration and the setter/getter corresponding to each attribute. If we want to generate the equals and hashCode methods of the corresponding PO, we can configure the following plug-ins.
<plugin type="org.mybatis.generator.plugins.EqualsHashCodePlugin"/>
To generate the toString method, you can use the following plug-in:
<plugin type="org.mybatis.generator.plugins.ToStringPlugin"/>
To generate serialization methods for the model, use the following plug-ins:
<plugin type="org.mybatis.generator.plugins.SerializablePlugin"/>
- commentGenerator
This configuration is mainly used to configure generated comments. By default, comments will be generated with time stamps. If we do not need these configurations, we can clear them through the following configuration:
<commentGenerator> <!-- Remove automatically generated comments true: Yes: false:no --> <property name="suppressAllComments" value="true"/> <!-- Remove automatically generated timestamp true: Yes: false:no --> <property name="suppressDate" value="true"/> <!-- Add comments for fields in database tables true: Yes: false:No, only if suppressAllComments by false Effective only when --> <property name="addRemarkComments" value="true"/> </commentGenerator>
- jdbcConnection
Since we want to automatically generate the corresponding file, we must link the database, so we need to configure the database. We talked about importing the external configuration file above. We can define the database configuration in the external file in this way, and then import the file for configuration. The specific steps are as follows:
<jdbcConnection driverClass="${jdbc.driver-class-name}" connectionURL="${jdbc.url}" userId="${jdbc.username}" password="${jdbc.password}"> <!--Higher version mysql-connector-java Need to set nullCatalogMeansCurrent=true--> <property name="nullCatalogMeansCurrent" value="true"/> </jdbcConnection>
- javaTypeResolver
It is mainly used to configure type conversion rules in JDBC and Java. If we do not configure them, the default set of conversion rules will be adopted. If we need to customize them, we can only configure bigDecimal, NUMERIC and time types, and cannot configure other types, otherwise errors will occur. The specific configuration rules are as follows:
<javaTypeResolver> <property name="forceBigDecimals" value="true"/> <property name="useJSR310Types" value="false"/> </javaTypeResolver>
- forceBigDecimals
This attribute defaults to false. At this time, it will resolve JDBC DECIMAL and NUMERIC types to Integer. If this attribute is true, it will resolve JDBC DECIMAL and NUMERIC types to Java math. BigDecimal.
- useJSR310Types
This attribute defaults to false, which resolves all JDBC time types to Java util. Date. If the attribute is true, it will be resolved according to the following rules:
Before conversion | After conversion |
---|---|
DATE | java.time.LocalDate |
TIME | java.time.LocalTime |
TIMESTAMP | java.time.LocalDateTime |
TIME_WITH_TIMEZONE | java.time.OffsetTime |
TIMESTAMP_WITH_TIMEZONE | java.time.OffsetDateTime |
- javaModelGenerator
This is mainly used to configure the package path and project path of the automatically generated PO. It needs to be configured according to your own needs. Here, take my own configuration as an example. For example, the package of my PO is com cunyu1943. mybatisgeneratordemo. Entity, and the project path is src/main/java.
<javaModelGenerator targetPackage="com.cunyu1943.mybatisgeneratordemo.entity" targetProject="src/main/java"> <!-- Whether to let schema As the suffix of the package, the default is false --> <property name="enableSubPackages" value="false"/> <!-- For String Fields of type set Method, default false --> <property name="trimStrings" value="true"/> </javaModelGenerator>
- sqlMapGenerator
Configure the generated mapper The path where XML is stored. For example, if we want to put it in the src/main/resources/mapper path, the configuration is as follows:
<sqlMapGenerator targetPackage="mapper" targetProject="src/main/resources"> </sqlMapGenerator>
- javaClientGenerator
The path for configuring mapper interface is generally stored in the mapper package of the project. For example, my configuration is:
<javaClientGenerator targetPackage="com.cunyu1943.mybatisgeneratordemo.mapper" targetProject="src/main/java" type="XMLMAPPER"> </javaClientGenerator>
- table
Configure the database table to automatically generate code. Here, one table corresponds to one table. If you want to generate multiple tables, you need to configure multiple tables. The following is a specific example:
<table schema="" tableName="user" domainObjectName="User" enableCountByExample="false" enableDeleteByExample="false" enableSelectByExample="false" enableUpdateByExample="false" selectByExampleQueryId="false"> <!--Use actual column name,Default to false--> <property name="useActualColumnNames" value="false" /> </table>
Among them, schema is the database name. Some databases need to be configured, and some databases do not need to be configured. You need to fill it in here according to your own database, but it is recommended to fill it in, so that different databases can also be applied. tableName corresponds to the database table name; domainObjectName corresponds to the generated entity class name. By default, it can not be configured. If it is not configured, it will convert the indication to class name according to Pascal naming method; Enablexxbyexample is true by default, and an Example help class will be generated by default. However, the configuration can only take effect when targetRuntime="MyBatis3". When targetRuntime="MyBatis3Simple", enablexxbyexample configuration will not work anyway.
4 execute generation
After the above configuration, we get the overall MyBatis Generator configuration. The complete configuration is as follows. You can modify the configuration according to your own needs.
<?xml version="1.0" encoding="UTF-8" ?> <!--mybatis Code generator related configuration for--> <!DOCTYPE generatorConfiguration PUBLIC "-//mybatis.org//DTD MyBatis Generator Configuration 1.0//EN" "http://mybatis.org/dtd/mybatis-generator-config_1_0.dtd"> <generatorConfiguration> <!-- Import profile --> <properties resource="generator.properties"/> <!-- One database one context,context The child elements of must be in the order it is given property*,plugin*,commentGenerator?,jdbcConnection,javaTypeResolver?, javaModelGenerator,sqlMapGenerator?,javaClientGenerator?,table+ --> <context id="myContext" targetRuntime="MyBatis3Simple" defaultModelType="flat"> <!-- Set the encoding of the generated file--> <property name="javaFileEncoding" value="UTF-8"/> <!-- This plug-in is generated for Java Model object added equals and hashCode method --> <plugin type="org.mybatis.generator.plugins.EqualsHashCodePlugin"/> <!-- increase toString method--> <plugin type="org.mybatis.generator.plugins.ToStringPlugin"/> <!-- Generate serialization method--> <plugin type="org.mybatis.generator.plugins.SerializablePlugin"/> <!-- notes --> <commentGenerator> <!-- Generate comments true: No: false: yes --> <property name="suppressAllComments" value="true"/> <!-- Remove timestamp true: Yes: false:no --> <property name="suppressDate" value="true"/> <!-- Add comments for fields in database tables true: Yes: false:No, only if suppressAllComments by false Effective only when --> <property name="addRemarkComments" value="true"/> </commentGenerator> <!-- jdbc connect --> <jdbcConnection driverClass="${jdbc.driver-class-name}" connectionURL="${jdbc.url}" userId="${jdbc.username}" password="${jdbc.password}"> <!--Higher version mysql-connector-java Need to set nullCatalogMeansCurrent=true--> <property name="nullCatalogMeansCurrent" value="true"/> </jdbcConnection> <!-- Type conversion --> <javaTypeResolver> <!--Whether to use bigDecimal,default false. false,hold JDBC DECIMAL and NUMERIC Type resolves to Integer true,hold JDBC DECIMAL and NUMERIC Type resolves to java.math.BigDecimal--> <property name="forceBigDecimals" value="true"/> <!--default false false,Will all JDBC The time type of resolves to java.util.Date true,take JDBC The time type of is resolved according to the following rules DATE -> java.time.LocalDate TIME -> java.time.LocalTime TIMESTAMP -> java.time.LocalDateTime TIME_WITH_TIMEZONE -> java.time.OffsetTime TIMESTAMP_WITH_TIMEZONE -> java.time.OffsetDateTime --> <property name="useJSR310Types" value="false"/> </javaTypeResolver> <!-- Generate entity class address --> <javaModelGenerator targetPackage="com.cunyu1943.mybatisgeneratordemo.entity" targetProject="src/main/java"> <!-- Whether to let schema As the suffix of the package, the default is false --> <property name="enableSubPackages" value="false"/> <!-- For string Fields of type set Method, default false --> <property name="trimStrings" value="true"/> </javaModelGenerator> <!-- generate Mapper.xml file --> <sqlMapGenerator targetPackage="mapper" targetProject="src/main/resources"> </sqlMapGenerator> <!-- generate XxxMapper.java Interface--> <javaClientGenerator targetPackage="com.cunyu1943.mybatisgeneratordemo.mapper" targetProject="src/main/java" type="XMLMAPPER"> </javaClientGenerator> <!-- schema Is the database name, oracle Configuration required, mysql No configuration is required. tableName Is the corresponding database table name domainObjectName Is the name of the entity class to be generated(You can not specify it. The table name is converted to class name by Pascal naming method by default) enableXXXByExample Default to true, by true A corresponding is generated Example Help class to help you query conditions. If you don't want it, it can be set as false --> <table schema="" tableName="user" domainObjectName="User" enableCountByExample="false" enableDeleteByExample="false" enableSelectByExample="false" enableUpdateByExample="false" selectByExampleQueryId="false"> <!--Use actual column name,Default to false--> <property name="useActualColumnNames" value="false"/> </table> </context> </generatorConfiguration>
Where, about the external file generator The configuration of properties is as follows. It mainly configures the relevant properties of the database.
jdbc.username=root jdbc.password=123456 jdbc.url=jdbc:mysql://localhost:3306/community?characterEncoding=UTF-8&serverTimezone=Asia/Shanghai jdbc.driver-class-name=com.mysql.cj.jdbc.Driver
Finally, when all the configuration is complete, you can use the Maven tool for code generation. The specific operation method is as follows: Click MyBatis Generator in Maven configuration of the project to generate.
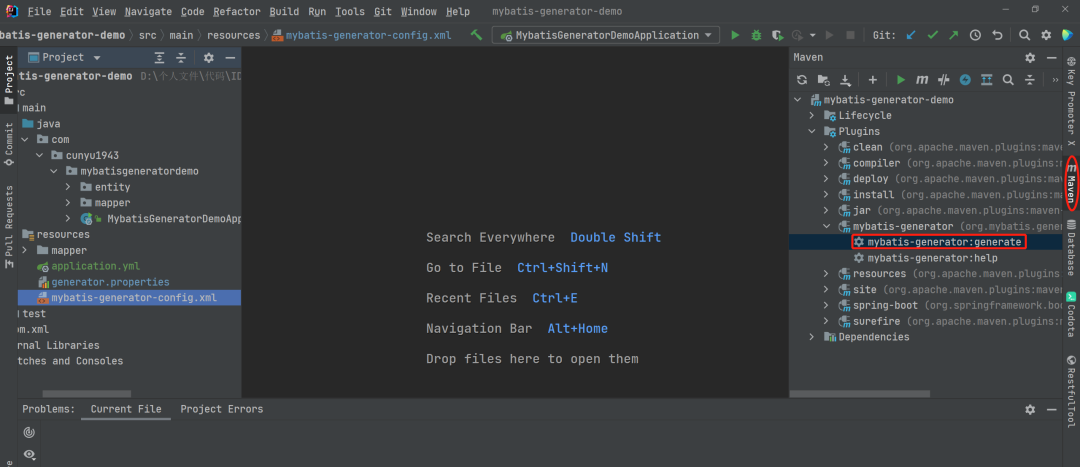
5 Summary
The above is to use Maven and MyBatis Generator to configure and generate project PO, Mapper and xxxmapper The specific construction process of XML. If you also happen to have this demand, try it quickly. If you encounter any problems during the construction process, please leave a message in the comment area. I will reply as soon as I see it.
Finally, I have passed the relevant code of this example to Github. If you need a brother, you can get it yourself.
🎉🎉🎉 Portal - > mybatis generator demo [1]
reference material
[1]mybatis-generator-demo: https://github.com/cunyu1943/java-learning-demos/tree/main/mybatis-generator-demo