Overall project structure
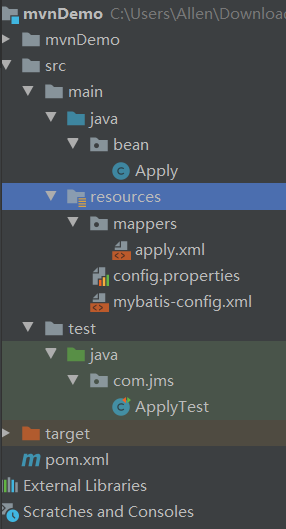
bean code
package bean;
public class Apply {
private long id;
private String sname;
private String qq;
private long enterTime;
private String type;
private String school;
private long number;
private String repLink;
private String goal;
@Override
public String toString() {
return "Apply{" +
"id=" + id +
", sname='" + sname + '\'' +
", qq='" + qq + '\'' +
", enterTime=" + enterTime +
", type='" + type + '\'' +
", school='" + school + '\'' +
", number=" + number +
", repLink='" + repLink + '\'' +
", goal='" + goal + '\'' +
'}';
}
public Apply() {
}
public Apply(int id, String sname, String qq, long enterTime, String type, String school, long number, String repLink, String goal) {
this.id = id;
this.sname = sname;
this.qq = qq;
this.enterTime = enterTime;
this.type = type;
this.school = school;
this.number = number;
this.repLink = repLink;
this.goal = goal;
}
public Apply(String sname, String qq, long enterTime, String type, String school, long number, String repLink, String goal) {
this.sname = sname;
this.qq = qq;
this.enterTime = enterTime;
this.type = type;
this.school = school;
this.number = number;
this.repLink = repLink;
this.goal = goal;
}
public long getId() {
return id;
}
public void setId(long id) {
this.id = id;
}
public String getSname() {
return sname;
}
public void setSname(String sname) {
this.sname = sname;
}
public String getQq() {
return qq;
}
public void setQq(String qq) {
this.qq = qq;
}
public long getEnterTime() {
return enterTime;
}
public void setEnterTime(long enterTime) {
this.enterTime = enterTime;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public String getSchool() {
return school;
}
public void setSchool(String school) {
this.school = school;
}
public long getNumber() {
return number;
}
public void setNumber(long number) {
this.number = number;
}
public String getRepLink() {
return repLink;
}
public void setRepLink(String repLink) {
this.repLink = repLink;
}
public String getGoal() {
return goal;
}
public void setGoal(String goal) {
this.goal = goal;
}
}
bean mapping file apply.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="test">
<!-- There are many configurations in the mapping file sql Sentence -->
<!-- take sql Statement encapsulation to mappedStatement Object, so id Be called statement Of id -->
<!-- parameterType: Specifies the type of the input parameter, which is specified here int type #{} represents a placeholder -- >
<!-- #{id}: the id indicates the parameter to receive the input. The parameter name is id. if you enter -- >
<!-- Parameters are simple types,#Parameter names in {} can be any, value or other names -- >
<!-- resultType: Appoint sql The mapped java Object type, select Appoint resultType Represents the mapping of a single record to java Object. -->
<!-- Table names should be correct, but case insensitive, resultType To write a class name, it is also case insensitive -->
<select id="getApply" parameterType="int" resultType="apply">
SELECT * FROM applytable WHERE id = #{value}
</select>
<insert id="addApply" parameterType="bean.Apply">
insert into applytable(id,sname, qq, entertime, `type`, school,`number`,replink,goal)
values (
null,
#{sname},
#{qq},
#{enterTime},
#{type},
#{school},
#{number},
#{repLink},
#{goal}
)
</insert>
<update id="updateApply" parameterType="bean.Apply">
update applytable set sname = #{sname}, qq = #{qq},
entertime = #{enterTime}, `type` = #{type}, school = #{school},`number` = #{number}, replink = #{repLink}, goal = #{goal}
where id = #{id}
</update>
<select id="getAllApply" resultType="bean.Apply">
select * from applytable order by id desc
</select>
<select id="getTotalApply" resultType="int">
select count(*) from applytable
</select>
<delete id="deleteApply" parameterType="int">
delete from applytable where id = #{id}
</delete>
</mapper>
Database properties file config.properties
jdbc.driver = com.mysql.jdbc.Driver
jdbc.url = jdbc:mysql://localhost:3306/xiuzhenyuan?characterEncoding=UTF-8
jdbc.username = root
jdbc.password = admin
MyBatis configuration file mybatis-config.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<! -- load property file -- >
<properties resource="config.properties">
In <! -- properties, you can also configure some property names and property values -- >
<!-- <property name="jdbc.driver" value=""/> -->
</properties>
<! -- global configuration parameter, set when necessary -- >
<!-- <settings> </settings> -->
<typeAliases>
<! -- alias definition -- >
<! -- define the path of type: type for a single alias alias alias: alias, class name cannot be written wrong
Aliases can be started at will, but it's best to standardize -- >
<typeAlias type="bean.Apply" alias="apply" />
<! -- batch alias definition specifies the package name, mybatis automatically scans the po class in the package, automatically defines the alias, and alias is the class name (both uppercase and lowercase are OK) - >
<package name="bean.Apply" />
</typeAliases>
<! -- environment configuration will be abolished after integration with spring -- >
<environments default="development">
<environment id="development">
<! -- using jdbc transaction management, transaction control is controlled by mybatis -- >
<transactionManager type="JDBC" />
<! -- database connection pool, managed by mybatis -- >
<dataSource type="POOLED">
<property name="driver" value="${jdbc.driver}" />
<property name="url" value="${jdbc.url}" />
<property name="username" value="${jdbc.username}" />
<property name="password" value="${jdbc.password}" />
</dataSource>
</environment>
</environments>
<! -- load mapping file -- >
<mappers>
<! -- load one mapping file at a time through the resource method -- >
<! -- note the path and xml file here -- >
<mapper resource="mappers/apply.xml" />
<! -- batch load mapper specifies the package name of the mapper interface. mybatis automatically scans all mapper interfaces under the package to load -- >
<! -- follow some specifications: you need to keep the mapper interface class name consistent with the mapper.xml mapping file name, and in a directory -- >
The premise of the upper specification in <! -- is that the mapper proxy method is used
<package name="...." />-->
</mappers>
</configuration>
Unit test code ApplyTest.java
package com.jms;
import bean.Apply;
import org.apache.ibatis.io.Resources;
import org.apache.ibatis.session.SqlSession;
import org.apache.ibatis.session.SqlSessionFactory;
import org.apache.ibatis.session.SqlSessionFactoryBuilder;
import org.junit.Test;
import java.io.IOException;
import java.io.InputStream;
import java.util.List;
public class ApplyTest {
public SqlSessionFactory getSqlSessionFactory() throws IOException {
// mybatis configuration file. The root address of this location is: resources. The path should be correct.
String resource = "mybatis-config.xml";
// Get profile stream
InputStream inputStream = Resources.getResourceAsStream(resource);
// Create a session factory and pass in the profile information of mybatis
SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(inputStream);
return sqlSessionFactory;
}
// Query user information according to id to get a record result
@Test
public void getApply() throws IOException {
// Get SqlSession through factory
SqlSession sqlSession = this.getSqlSessionFactory().openSession();
// Operating the database through SqlSession
// First parameter: id of statement in mapping file, equal to id of = namespace + ". + statement
// Second parameter: Specifies the parameter of type parameterType matching in the mapping file
// sqlSession.selectOne result is an object of type resultType matching in the mapping file
// selectOne finds a record (this is very troublesome)!!! Look back)
//Here, the parameter test.findUserById, test is the namespace, which corresponds to the one in user.xml,
//In the same way, findUserById should also exist in user.xml. Otherwise, an error will be reported
Apply apply = sqlSession.selectOne("test.getApply", 2);
// Release resources
sqlSession.close();
}
@Test
public void addApply() throws IOException {
SqlSession sqlSession = this.getSqlSessionFactory().openSession();
sqlSession.insert("test.addApply", new Apply("Alice", "43523", 3452341, "Html", "SCHOOL", 4, "hgw3204", "DAY DAY UP"));
sqlSession.commit();
sqlSession.close();
}
@Test
public void updateApply() throws IOException {
SqlSession sqlSession = this.getSqlSessionFactory().openSession();
sqlSession.update("test.updateApply", new Apply(2, "Alice", "43523", 3452341, "Html", "SCHOOL", 4, "hgw3204", "DAY DAY UP"));
sqlSession.commit();
sqlSession.close();
}
@Test
public void getAll() throws IOException {
SqlSession sqlSession = this.getSqlSessionFactory().openSession();
final List<Apply> selectList = sqlSession.selectList("test.getAllApply");
for (Apply apply :
selectList) {
System.out.println(apply);
}
sqlSession.close();
}
@Test
public void getTotal() throws IOException {
SqlSession sqlSession = this.getSqlSessionFactory().openSession();
final Integer total = (Integer) sqlSession.selectOne("test.getTotalApply");
System.out.println(total);
}
@Test
public void delete() throws IOException {
SqlSession sqlSession = this.getSqlSessionFactory().openSession();
sqlSession.delete("test.deleteApply", 20);
sqlSession.commit();
sqlSession.close();
}
}
Unit test results
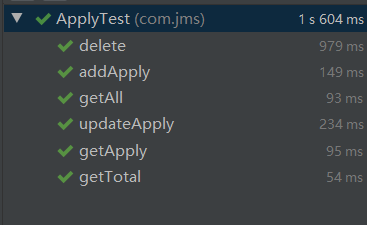