1 - framework concept
The framework in program development is often the encapsulation of common functions. The program framework is understood as the basis or mechanical standard parts (such as screw and nut standard mechanical parts).
If you want to build a carriage, without a frame, you need to cut wood by yourself, make wood into boards and sticks, and then form wheels, doors, and other parts, and then assemble them. But if you use a frame, you have ready-made wheels, doors and other parts. You only need to assemble them. A framework is a set of reusable design components.
Framework: it is the reusable design of the whole or part of the system and the encapsulation of Java EE underlying technology. A framework is an application skeleton that can be customized by developers. Framework is a semi-finished product, and software is a finished product.
2-three storey structure
2.1 layering method
A medium and large-scale software development needs a clear hierarchy.
Layered package | Function description | effect |
---|---|---|
cn.guardwhy.view | Presentation layer View | Customer oriented, used to process customer input and output, front-end code. |
cn.guardwhy.service | Business layer Service | Process business logic codes, such as login, transfer and registration |
cn.guardwhy.dao | Data access layer DAO Data Access Object | Also called persistence layer. For the database, the operation of adding, deleting, modifying and querying the database is realized |
2.2 advantages and disadvantages of layering
advantage:
- It reduces the coupling degree of code and the relationship between classes. Conducive to team development.
- The project has better scalability and maintainability.
- The reusability is better. The same method can be called by multiple classes.
Disadvantages:
- Lower execution efficiency and greater development workload
- It will lead to cascade modification. If a function is modified, all three layers will be modified.
Introduction to 3-mybatis
3.1 what is Mybatis
- MyBatis is an excellent persistence layer framework.
- MyBatis avoids almost all JDBC code and the process of manually setting parameters and obtaining result sets.
- MyBatis can use simple XML or annotations to configure and map native information, and map the interface and Java entity class [Plain Old Java Objects] into records in the database.
- MyBatis was originally an open source project ibatis of apache. In 2010, this project was migrated from apache to google code and renamed MyBatis. Moved to Github in November 2013.
3.2 persistence
Persistence is a mechanism to convert program data between persistent state and transient state.
That is, save data (such as objects in memory) to a storage device that can be permanently saved (such as disk). The main application of persistence is to store objects in memory in a database, or in disk files, XML data files, etc. JDBC is a persistence mechanism. File IO is also a persistence mechanism.
Why do you need persistence services?
- There are some objects that can't disappear.
- Memory is expensive.
Persistent layer
- Code block to complete persistence. --- > Dao layer (DAO (Data Access Object)
- In most cases, data persistence often means saving the data in memory to disk for solidification, and the implementation process of persistence is mostly completed through various relational databases.
- Compared with other parts of the system, this level should have a clear and strict logical boundary. To put it bluntly, it is used to operate the database.
3.3 advantages and disadvantages of mybatis
advantage
- Easy to learn: mybatis itself is small and simple. There is no third-party dependency. The simplest installation is as long as two jar files + several SQL mapping files are configured.
- Flexible use: Mybatis does not impose any impact on the existing design of the application or database. SQL statements are written in XML for unified management and optimization.
- Decouple SQL and program code: separate business logic and data access logic by providing DAO layer, so as to make the system design clearer, easier to maintain and easier to carry out unit test.
shortcoming
- There is a lot of work when writing SQL statements, especially when there are many fields and associated tables.
- SQL statements depend on the database, resulting in poor database portability, and the database cannot be replaced.
- The framework is still rudimentary, the function is still missing, and the secondary cache mechanism is poor.
3.4 concept of ORM
Object Relational Mapping
- Programming in Java: using object-oriented development.
- SQL statements written in MySQL: a relational database is used to map the data in the table into an object, object relational mapping.
Two mapping methods of mybatis
- Configuration file via XML.
- By annotation
3.5 download and installation
Download from the official website
Mybatis official address: http://www.mybatis.org/mybatis-3/
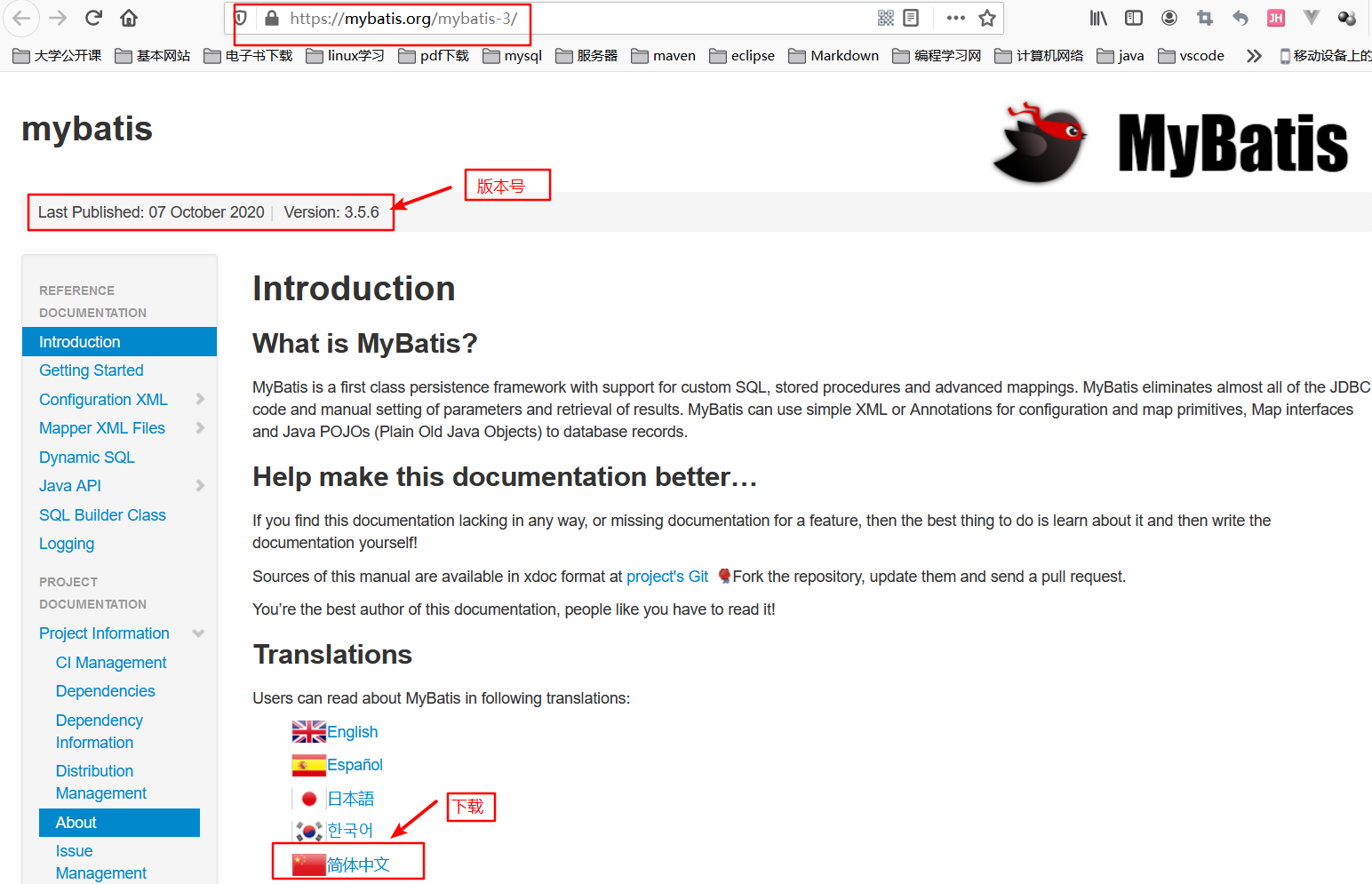
github Download
github address: https://github.com/mybatis/mybatis-3/releases
4 - Introduction to mybatis
4.1 project preparation
4.1. 1 project directory
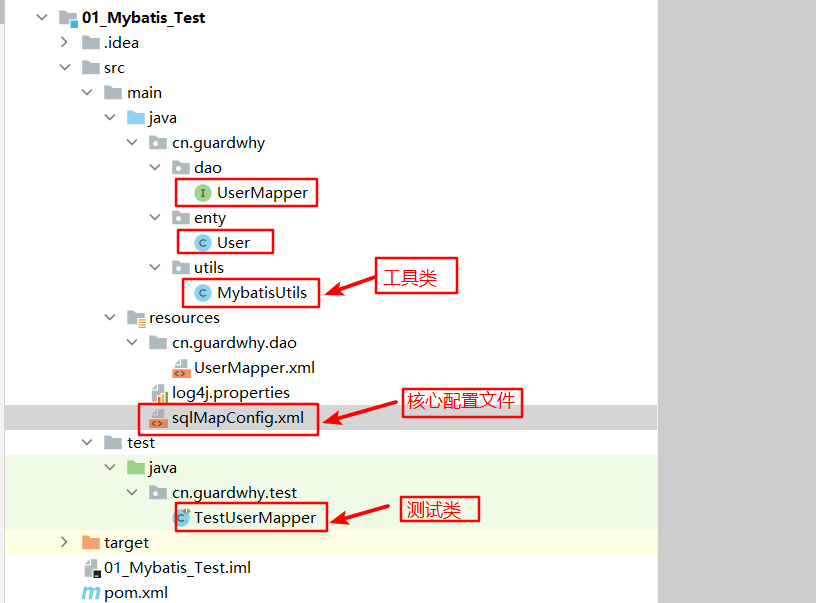
Import dependent
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>cn.guardwhy</groupId> <artifactId>Mybatis</artifactId> <version>1.0-SNAPSHOT</version> <packaging>jar</packaging> <!--Pour in the dependencies required by the project--> <dependencies> <!-- mybatis Correlation dependency--> <dependency> <groupId>org.mybatis</groupId> <artifactId>mybatis</artifactId> <version>3.5.2</version> </dependency> <!-- mysql Database dependency--> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>5.1.47</version> </dependency> <!-- Log dependent dependencies--> <dependency> <groupId>log4j</groupId> <artifactId>log4j</artifactId> <version>1.2.17</version> </dependency> <!-- Test dependent--> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.12</version> </dependency> <!--lombok plug-in unit--> <dependency> <groupId>org.projectlombok</groupId> <artifactId>lombok</artifactId> <version>1.18.16</version> </dependency> </dependencies> </project>
Sql basic structure
-- Create database create database db_mybatis; -- Create data table create table user ( id int primary key auto_increment, username varchar(20) not null, birthday date, sex char(1) default 'male', address varchar(50) ); -- insert data insert into user values (null, 'Hou Dali','1980-10-24','male','Jiangzhou'); insert into user values (null, 'Tian Tian','1992-11-12','female','Yangzhou'); insert into user values (null, 'Yong Qiang Wang','1983-05-20','male','Yangzhou'); insert into user values (null, 'Yang Hong','1995-03-22','female','Qin Yang'); select * from user;
log4j.properties
### set up Logger Output level and output destination ### log4j.rootLogger=debug, stdout ### Output log information to the console ### log4j.appender.stdout=org.apache.log4j.ConsoleAppender log4j.appender.stdout.Target=System.out log4j.appender.stdout.layout=org.apache.log4j.SimpleLayout
db.properties
Write the database connection properties resource file (db.properties) and put it under the resources resource file.
jdbc.driver=com.mysql.jdbc.Driver jdbc.url=jdbc:mysql://localhost:3306/db_mybatis jdbc.username=root jdbc.password=root
Load dB Properties property file
<!--Configure attributes internally: read the internal attributes first,Then read the external properties,The outside will cover the inside,Finally, external attributes work--> <properties resource="db.properties"> <property name="jdbc.username" value="root"/> <property name="jdbc.password" value="root"/> </properties>
4.1.2 User entity class
package cn.guardwhy.domain; /** * Entity class */ import java.sql.Date; @Data @NoArgsConstructor @AllArgsConstructor public class User { private Integer id; private String username; private Date birthday; private String sex; private String address; } }
4.1.3 UserMapper interface
This interface is actually the data access layer: DAO layer
package cn.guardwhy.dao; import cn.guardwhy.enty.User; import java.util.List; /** * Data access layer method */ public interface UserMapper { // Find all users List<User> findAllUsers(); }
4.1.4 UserMapper.xml
- Interface mapping file: usermapper XML to write SQL statements.
- Create the cn/guardwhy/dao folder in resources and usermapper.com in the directory XML Mapping file.
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <!-- Mapping file of entity class: namespace Specifies the full class name of the interface --> <mapper namespace="cn.guardwhy.dao.UserMapper"> <!-- Query statement id: The name of the method in the interface, resultType: The full name of the type class of the returned entity class, parameterType: Type of parameter --> <select id="findAllUsers" resultType="cn.guardwhy.enty.User"> select * from user </select> </mapper>
4.1.5 sqlMapConfig.xml
sqlMapConfig.xml is the core configuration file of mybatis framework, sqlmapconfig XML configuration connection database parameters.
properties | External replaceable properties that can be read from the Java property configuration file. |
---|---|
settings | mybatis global configuration parameters |
typeAliases | Define aliases for Java types |
typeHandlers | The type processor converts the values in the result set into Java types in an appropriate way |
objectFactory | You can specify an object factory for creating result objects |
plugins | Allows you to use plug-ins to intercept calls to some methods in mybatis |
environments | Configure multiple environments to apply SQL mapping to multiple databases. Transaction manager, two transaction manager types. dataSource specifies the type of data source |
mappers | A configuration file that defines SQL mapping statements |
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd"> <configuration> <!--Configure attributes internally: read the internal attributes first,Then read the external properties,The outside will cover the inside,Finally, external attributes work--> <properties resource="db.properties"> <property name="jdbc.username" value="root"/> <property name="jdbc.password" value="root"/> </properties> <!-- A core configuration file can configure multiple operating environments, default Which operating environment is used by default --> <environments default="default"> <!-- One of the running environments, through id To identify--> <environment id="default"> <!--Transaction manager --> <transactionManager type="JDBC"/> <!--data source --> <dataSource type="POOLED"> <property name="driver" value="${jdbc.driver}"/> <property name="url" value="${jdbc.url}"/> <property name="username" value="${jdbc.username}"/> <property name="password" value="${jdbc.password}"/> </dataSource> </environment> </environments> <!-- Load other mapping files --> <mappers> <mapper resource="cn/guardwhy/dao/UserMapper.xml"/> </mappers> </configuration>
4.2 test class implementation
4.2. 1 three objects of mybatis
In mybatis, a session is equivalent to a process of accessing the database, and a session object is similar to a Connection object.
- SqlSessionFactoryBuilder: create a session factory through this factory building class.
- SqlSessionFactory: get a session object from a factory class and create a session object through the session factory object.
- SqlSession: each time you access the database, you need to create a session object, which cannot be shared. After the access is completed, the session needs to be closed.
4.2. 2 implementation steps
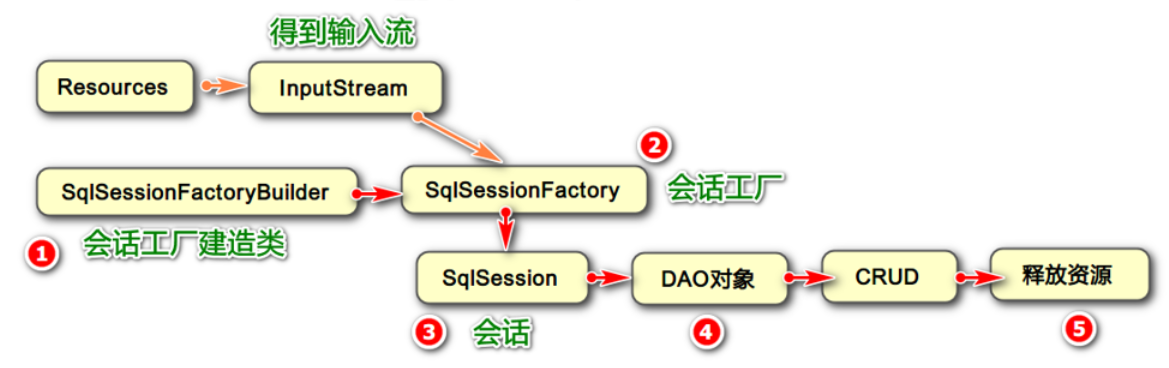
- Through the Resources class provided by the framework, load sqlmapconfig XML to get the file input stream InputStream object
- Instantiate the session factory to create the class SqlSessionFactoryBuilder.
- Through the SqlSessionFactoryBuilder object above, read the input stream of the core configuration file to get the session factory SqlSessionFactory class
- Use the SqlSessionFactory object to create a SqlSession object
It is equivalent to JDBC Medium Connection Object that provides a way to operate the database CRUD method. It provides a getMapper()Method to obtain the implementation object of the interface.
-
Get the UserMapper object of the interface and get the proxy object of the interface
-
Execute the query operation of the database and output user information
-
Close the session and free up resources.
4.2. 3 code example
Tool class code
package cn.guardwhy.utils; import org.apache.ibatis.io.Resources; import org.apache.ibatis.session.SqlSession; import org.apache.ibatis.session.SqlSessionFactory; import org.apache.ibatis.session.SqlSessionFactoryBuilder; import java.io.IOException; import java.io.InputStream; public class MybatisUtils { // 1. Declare a factory object private static SqlSessionFactory factory; // 2. Create a session factory in a static code block static { SqlSessionFactoryBuilder builder = new SqlSessionFactoryBuilder(); // Get input stream try(InputStream inputStream = Resources.getResourceAsStream("sqlMapConfig.xml");){ factory = builder.build(inputStream); }catch(IOException e){ e.printStackTrace(); } } // 3. Get the session factory by static method public static SqlSessionFactory getSessionFactory(){ return factory; } // 4. Get the session object public static SqlSession getSession(){ return factory.openSession(); } }
Test class code
package cn.guardwhy.test; import cn.guardwhy.dao.UserMapper; import cn.guardwhy.enty.User; import cn.guardwhy.utils.MybatisUtils; import org.apache.ibatis.io.Resources; import org.apache.ibatis.session.SqlSession; import org.apache.ibatis.session.SqlSessionFactory; import org.apache.ibatis.session.SqlSessionFactoryBuilder; import org.junit.Test; import java.io.IOException; import java.io.InputStream; import java.util.List; /** * Use db_mybatis database */ public class TestUserMapper { @Test /* * Query all users */ public void selectUser(){ // 1. Get the session object through the tool class SqlSession session = MybatisUtils.getSession(); // 2. Get the UserMapper interface proxy object from the session object UserMapper userMapper = session.getMapper(UserMapper.class); // 3. The proxy object is generated System.out.println(userMapper); // 4. Execute query List<User> users = userMapper.findAllUsers(); // 5. Traversal users.forEach(System.out::println); // 6. Close the session session.close(); } }