The last article described how to use mybatis plus to automatically generate code. The generated code has the ability to operate the database with a single table, saving development time. Then it describes how to integrate mybatis plus in Spring Boot. This article describes how to use the enhancements of mybatis plus: auto fill and query paging.
Mybatis plus auto fill function
In the matrix web project, all tables in the database have four common fields, create_by,create_time,update_by,update_time, that is, the creator, creation time, update time and updater of the stored table data. When inserting a piece of data into a table, four fields need to be filled automatically. For table data update, the last two fields need to be updated. However, if you manually fill in these four fields in each insert and update business logic, the workload will be increased. Fortunately, mybatis plus has an automatic update plug-in.
It is very simple to implement the auto fill function. You only need to implement the MetaObjectHandler interface and fill logic, and inject it into the spring IOC container. The following code is userutils getCurrentUserWithDefault(); That is, the user who obtains the current request has been mentioned in the article on permission, which will not be repeated here; Then add the annotation @ Component and inject it into the spring IOC container.
@Component @Slf4j public class MyMetaObjectHandler implements MetaObjectHandler { @Override public void insertFill(MetaObject metaObject) { log.info("start insert fill ...."); Object createBy = getFieldValByName("createBy", metaObject); Object updateBy = getFieldValByName("updateBy", metaObject); Object createTime = getFieldValByName("createTime", metaObject); Object updateTime = getFieldValByName("updateTime", metaObject); if (createBy == null) { this.setFieldValByName("createBy", UserUtils.getCurrentUserWithDefault(), metaObject);//Version 3.0.6 and earlier } if (updateBy == null) { this.setFieldValByName("updateBy", UserUtils.getCurrentUserWithDefault(), metaObject); } if (createTime == null) { this.setFieldValByName("createTime", new Date(), metaObject); } if (updateTime == null) { this.setFieldValByName("updateTime", new Date(), metaObject); } } @Override public void updateFill(MetaObject metaObject) { log.info("start update fill ...."); Object updateBy = getFieldValByName("updateBy", metaObject); Object updateTime = getFieldValByName("updateTime", metaObject); if (updateBy == null) { this.setFieldValByName("updateBy", UserUtils.getCurrentUserWithDefault(), metaObject); } if (updateTime == null) { this.setFieldValByName("updateTime", new Date(), metaObject); } } }
- The field must declare a TableField annotation, and the attribute fill selects the corresponding policy. This declaration tells mybatis plus that SQL fields need to be reserved and injected
- The population processor MyMetaObjectHandler needs to declare @ Component injection in Spring Boot
- The setFieldValByName() or setInsertFieldValByName/setUpdateFieldValByName method of the parent class must be used, otherwise it will not be used according to the annotation fieldfill XXX to distinguish.
public enum FieldFill { /** * Do not process by default */ DEFAULT, /** * Insert fill field */ INSERT, /** * Update populated fields */ UPDATE, /** * Insert and update populated fields */ INSERT_UPDATE }
Then add the corresponding annotations to the entity classes mapped in the table. For example, in matrix web, all database mapped entity classes inherit BaseEntity.
@Data public class BaseEntity { @JsonSerialize(using= ToStringSerializer.class) @TableId(value = "id", type = IdType.ID_WORKER) protected Long id; /** * Creation time */ @TableField(value = "create_time", fill = FieldFill.INSERT) protected Date createTime; /** * Creator id */ @TableField(value = "create_by", fill = FieldFill.INSERT) protected String createBy; /** * Update time */ @TableField(value = "update_time", fill = FieldFill.INSERT_UPDATE) protected Date updateTime; /** * Updater */ @TableField(value = "update_by", fill = FieldFill.INSERT_UPDATE) protected String updateBy; }
For example, the SysUser entity class inherits the BaseEntity above.
@Data @EqualsAndHashCode(callSuper = true) @Accessors(chain = true) public class SysUser extends BaseEntity { ... }
When SysUserMapper is called to insert SysUser data, create will be automatically inserted into the database_ by,create_time,update_by,update_time these four data.
Paging plug-in
Paging plug-ins are often used in Web development. Mybatis plus provides this capability. PaginationInterceptor needs to be injected into the spring ioc container. The code is as follows:
@Configuration public class MybatisPlusConfig { /** * Paging plug-in */ @Bean public PaginationInterceptor paginationInterceptor() { return new PaginationInterceptor(); } }
Now let's take a specific case to illustrate how to paginate. For example, query the page of SysUser. At the Controller layer:
@GetMapping("/pagelist") public RespDTO searchUsers(@RequestParam int page, @RequestParam int pageSize, @RequestParam(required = false) String userId, @RequestParam(required = false) String realname) { PageUtils.check(page, pageSize); PageResultsDTO sysUsers = sysUserService.searchUsers(page, pageSize, userId, realname); return RespDTO.onSuc(sysUsers); }
In the above code, you need to pass two parameters, page and pageSize, that is, the number of pages and the number of pages per page. PageUtils.check(page, pageSize) is to check whether page and pageSize are reasonable. RespDTO is the DTO class returned to the front end. The specific codes are as follows:
public class RespDTO<T> implements Serializable { public int code = 0; public String message = ""; public T data; public String requestId; }
PageResultsDTO is used to store paging data and paging metadata. The code is as follows:
public class PageResultsDTO<T> { public List<T> list = new ArrayList<>(); public int page; public int pageSize; public long totalCount; public int offset; public int totalPage; }
The service layer code needs to wrap the data queried by sysUserMapper into PageResultsDTO. The code is as follows:
public PageResultsDTO searchUsers(int page, int pageSize, String userId, String realname) { Page<SysUser> sysLogPage = new Page<>(page, pageSize); IPage<SysUser> sysUserIPage = sysUserMapper.searchUsers(sysLogPage, userId, realname); PageResultsDTO result = new PageResultsDTO(page, pageSize); result.setTotalCount(sysUserIPage.getTotal()); result.setTotalPage((int) sysUserIPage.getTotal(), pageSize); List<SysUser> records = sysUserIPage.getRecords(); result.setList(transformSysUserDTO(records)); return result; }
The searchUsers method of SysUserMapper will query the database to obtain data:
@Mapper public interface SysUserMapper extends BaseMapper<SysUser> { IPage<SysUser> searchUsers(Page page, @Param("userId") String userId, @Param("realname") String realname); }
The sql statement of SysUserMapper to query the database needs to be written by yourself in SysUserMapper In XML,
<select id="searchUsers" resultMap="BaseResultMap"> SELECT a.id as id ,a.create_time as create_time, a.create_by as create_by,a.update_time as update_time,a.update_by as update_by ,a.user_id as user_id,a.password as password,a.realname as realname,a.type as type,a.mobile as mobile ,a.email as email,a.remarks as remarks ,a.status as status,a.avatar as avatar,a.sex as sex ,c.name as role_name,c.role_id as role_role_id ,e.org_id as org_id,e.simple_name as simple_name,g.code as code,g.url as url from sys_user a LEFT JOIN sys_user_role b on a.user_id=b.user_id LEFT JOIN sys_role c on b.role_id=c.role_id LEFT JOIN sys_user_org d on a.user_id=d.user_id left JOIN sys_org e on d.org_id =e.org_id left join sys_role_menu f on f.role_id=c.role_id left join sys_menu g on g.code=f.menu_code <where> <if test="userId != null and userId!='' "> a.user_id = #{userId} </if> <if test="realname != null and realname!='' "> AND a.realname = #{realname} </if> </where> </select>
This is a complete case of paging using the mybatis plus plug-in. The front-end code is not explained here. The paging effect displayed in the front-end interface is as follows:
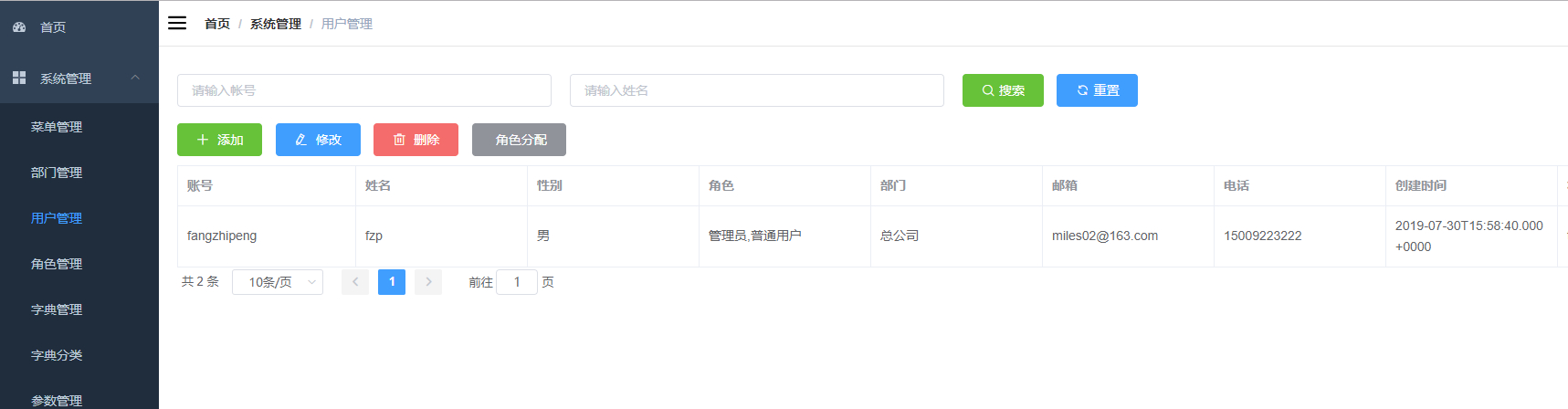
summary
This article describes how to use the enhancements of mybatis plus to automatically fill and page. The next article will describe how to use Durid connection pool and sharding JDBC to separate database read and write.
Participation information:
https://mp.baomidou.com/guide/#%E7%89%B9%E6%80%A7
Source download
https://github.com/forezp/matrix-web