The video of the open class below has been recorded and the students who need it can leave a message
MyBatis, an excellent persistence layer ORM framework with a wide range of applications, has become an almost standard part of the Java Web world, supporting customization of SQL, stored procedures, and advanced mappings.MyBatis avoids almost all JDBC code and setting parameters manually and getting result sets.MyBatis can use simple XML or annotations to configure and map native types, interfaces, and Java POJO s (Plain Old Java Objects, plain old Java objects) as records in the database.
1. Download the source code
Download the latest stable version or the MyBatis source code used in your current project. This lesson takes MyBatis 3.5.1 as an example (the latest version is 3.5.2-SNAPSHOT).
Download address: https://github.com/mybatis/mybatis-3
Note the version selection by reading tag.
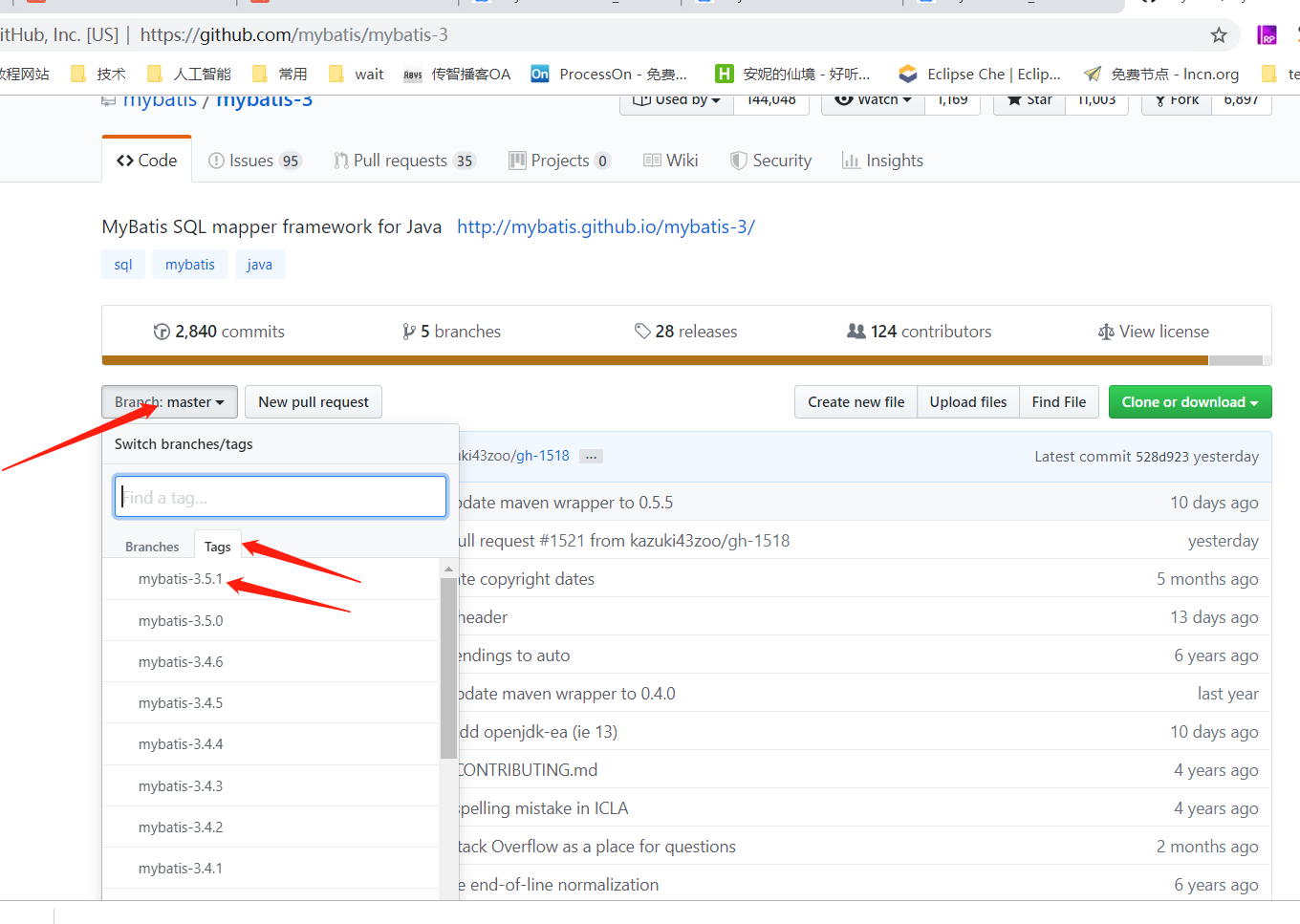
2. Create Source Project Project
After downloading the source code, importing the pom project in the idea requires following steps to modify and adjust the project in order to compile successfully.
- The ognl and javassist in the pom file were modified to <optional>false</optional>
<dependency> <groupId>ognl</groupId> <artifactId>ognl</artifactId> <version>3.2.10</version> <scope>compile</scope> <optional>false</optional> </dependency> <dependency> <groupId>org.javassist</groupId> <artifactId>javassist</artifactId> <version>3.24.1-GA</version> <scope>compile</scope> <optional>false</optional> </dependency>
Remove license.txt from project directory
If not deleted, an error will occur when executing the maven install.Manually download jar packages locally
This depends if the following error occurs when executing maven install locally
[INFO] --- maven-surefire-plugin:2.7.1:test (default-test) @ wms --- [WARNING] Error injecting: org.apache.maven.plugin.surefire.SurefirePlugin java.lang.NoClassDefFoundError: org/apache/maven/surefire/util/NestedCheckedException at java.lang.ClassLoader.defineClass1(Native Method)
Consider manually downloading the corresponding jar package into your local maven repository.
Download address: http://repo1.maven.org/maven2/org/apache/maven/surefire/maven-surefire-common/3.0.0-M3/maven-surefire-common-3.0.0-M3.jar
-
Turn off maven-pdf-plugin
<!-- <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-pdf-plugin</artifactId> </plugin> -->
-
Close test
Ignore test when executing maven install.

-
Execute maven install
Install the MyBatis compilation into the local maven repository.
3. Build a simple demo
Start with the simplest demo, don't integrate Spring first, just look at the simplest and most original MyBatis, familiarize yourself with the MyBatis source code, and then see how to integrate with Spring.
Build demo with official documentation: http://www.mybatis.org/mybatis-3/zh/getting-started.html
Basic usage process
-
Introducing dependencies
<dependency> <groupId>org.mybatis</groupId> <artifactId>mybatis</artifactId> <version>3.5.1</version> </dependency> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>5.1.46</version> </dependency> <dependency> <groupId>log4j</groupId> <artifactId>log4j</artifactId> <version>1.2.17</version> </dependency>
-
Create xml configuration file
mybatis-config.xml
<?xml version="1.0" encoding="utf-8" ?> <!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Cofig 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd"> <configuration> <environments default="development"> <environment id="development"> <transactionManager type="JDBC"></transactionManager> <dataSource type="POOLED"> <property name="driver" value="com.mysql.jdbc.Driver"/> <property name="url" value="jdbc:mysql://localhost:3306/test"/> <property name="username" value="root"/> <property name="password" value="123456"/> </dataSource> </environment> </environments> <mappers> <mapper resource="EmployeeMapper.xml"></mapper> </mappers> </configuration>
EmployeeMapper.xml
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <mapper namespace="com.boxuegu.javaee.mybatissourcelearn.dao.EmployeeMapper"> <select id="getEmployeeById" resultType="com.boxuegu.javaee.mybatissourcelearn.dto.Employee"> select id,last_name lastname,gender, email from bxg_employee where id=#{id} </select> </mapper>
-
Create a dto object
Employee.java
package com.boxuegu.javaee.mybatissourcelearn.dto; /** * @author Jam Fang https://www.jianshu.com/u/0977ede560d4 * @version Creation time: 05.24 15:27 */ public class Employee { private Integer id; private String lastName; private String email; private String gender; @Override public String toString() { return "Employee{" + "id=" + id + ", lastName='" + lastName + '\'' + ", email='" + email + '\'' + ", gender='" + gender + '\'' + '}'; } public Integer getId() { return id; } public void setId(Integer id) { this.id = id; } public String getLastName() { return lastName; } public void setLastName(String lastName) { this.lastName = lastName; } public String getEmail() { return email; } public void setEmail(String email) { this.email = email; } public String getGender() { return gender; } public void setGender(String gender) { this.gender = gender; } }
-
Create a dao
EmployeeMapper.java
package com.boxuegu.javaee.mybatissourcelearn.dao; import com.boxuegu.javaee.mybatissourcelearn.dto.Employee; /** * @author Jam Fang https://www.jianshu.com/u/0977ede560d4 * @version Creation time: 05.24 17:32 */ public interface EmployeeMapper { public Employee getEmployeeById(Integer id); }
-
Create database test tables
-- ---------------------------- -- Table structure for bxg_employee -- ---------------------------- DROP TABLE IF EXISTS `bxg_employee`; CREATE TABLE `bxg_employee` ( `id` int(11) NOT NULL AUTO_INCREMENT, `last_name` varchar(255) DEFAULT NULL, `gender` char(1) DEFAULT NULL, `email` varchar(255) DEFAULT NULL, PRIMARY KEY (`id`) ) ENGINE=InnoDB AUTO_INCREMENT=2 DEFAULT CHARSET=utf8; -- ---------------------------- -- Records of bxg_employee -- ---------------------------- INSERT INTO `bxg_employee` VALUES ('1', 'zhangsan', '1', 'zhangsan@itcast.cn');
-
Create test code
package com.boxuegu.javaee.mybatissourcelearn; import com.boxuegu.javaee.mybatissourcelearn.dao.EmployeeMapper; import com.boxuegu.javaee.mybatissourcelearn.dto.Employee; import org.apache.ibatis.io.Resources; import org.apache.ibatis.session.SqlSession; import org.apache.ibatis.session.SqlSessionFactory; import org.apache.ibatis.session.SqlSessionFactoryBuilder; import java.io.IOException; import java.io.InputStream; /** * @author Jam Fang https://www.jianshu.com/u/0977ede560d4 * @version Creation time: 2019/6/17 13:16 */ public class Test { public static void main(String[] args) { //1. Load Profile String resource = "mybatis-config.xml"; InputStream inputStream = null; try { inputStream = Resources.getResourceAsStream(resource); } catch (IOException e) { e.printStackTrace(); } //2. Get sqlSessionFactory SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(inputStream); //3. Get sqlSession SqlSession sqlSession = sqlSessionFactory.openSession(); try { //4. Get mapper interface implementation EmployeeMapper mapper = sqlSession.getMapper(EmployeeMapper.class); System.out.println("mapper::::" + mapper.getClass()); //5. Execute sql statements Employee employee = mapper.getEmployeeById(1); System.out.println(employee); } finally { sqlSession.close(); } } }