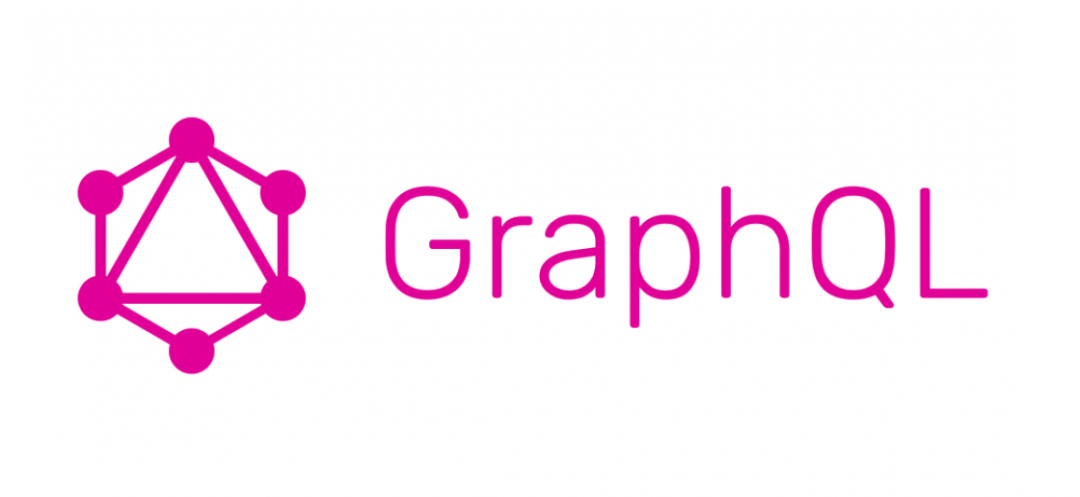
Hot Chocolate is NET platform. You can use it to create GraphQL services, which eliminates the complexity of building mature GraphQL services. Hot Chocolate can connect any service or data source, and create a cohesive service to provide a unified API for your consumers.
In this article, I will NET application, let's start!
01 create GraphQL Server
Here we create an empty NET Web project, and uses NET 6 mini api
dotnet new web -n HotChocolateDemo
Then install the Hot Chocolate package through the command or Nuget
dotnet add ./HotChocolateDemo package HotChocolate.AspNetCore
Next, you need to modify the program CS, inject the GraphQL service
var builder = WebApplication.CreateBuilder(args); var services = builder.Services; services.AddGraphQLServer(); var app = builder.Build(); app.MapGet("/", () => "Hello World!"); app.UseRouting() .UseEndpoints(endpoints => { endpoints.MapGraphQL(); }); app.Run();
Run the project and access the / graphql endpoint

The project will display the above page. This is the GraphQL IDE and banana cake pop provided by the chilicream platform. You can use it to browse and request GraphQL services, which is a bit like a combination of swagger and Postman.
Now it is empty because we have not created any GraphQL API. Next, I will create the simplest query service, and then use Banana Cake Pop to query our GraphQL service.
02 create GraphQL Schema
First, add the following entity class to the project
/// <summary> ///Books /// </summary> public class Book { /// <summary> ///Number /// </summary> public int Id { get; set; } /// <summary> ///Title /// </summary> public string? Title { get; set; } } /// <summary> ///User /// </summary> public class Person { /// <summary> ///Age /// </summary> public int Age { get; set; } /// <summary> ///Name /// </summary> public string? Name { get; set; } }
Next, create a query service for the entity
public class Query { /// <summary> ///Gets the specified book /// </summary> ///< param name = "Id" > Book No. < / param > /// <returns></returns> public Book GetBook(int id) { return new Book { Id = id, Title = "C# in depth." }; } /// <summary> ///Gets the specified user /// </summary> ///< param name = "name" > user name < / param > /// <returns></returns> public Person GetPerson(string name) { return new Person { Age = 20, Name = name }; } }
Then modify the program CS, as follows
services.AddGraphQLServer().AddQueryType<Query>();
03 executing GraphQL query
After the preparation is completed, run the project again, access the / graphql endpoint, Ctrl + Alt + T create a new tab, and then select Schema Reference to view the Query API. As you can see, you can also see the description information of each api, provided that NET project.
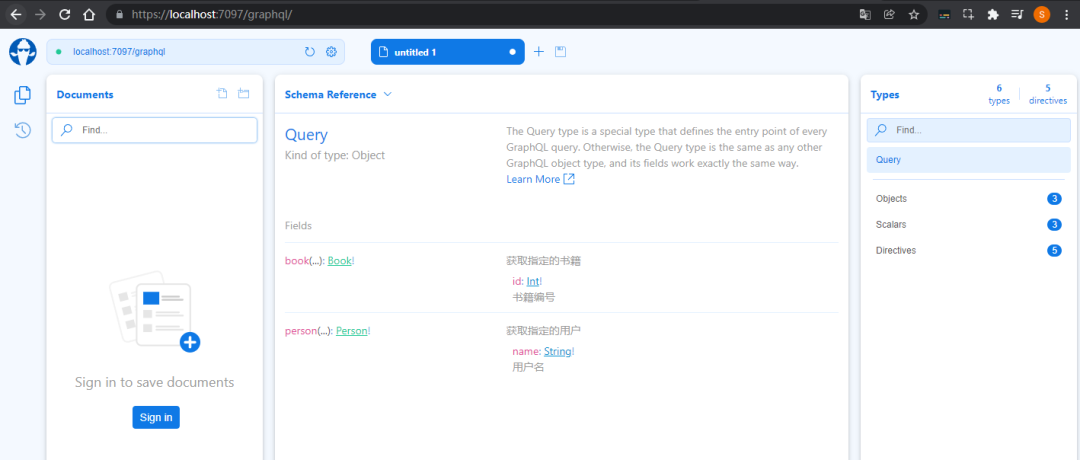
Then you can enter the following query statement to query
query{ book(id:123456){ id title } }
The back-end service will return the following content, which is the simplest query

Using Hot Chocolate to build a GraphQL service is so simple! Of course, I will continue to introduce updates and operations used in combination with EF Core.