1..NET Remoting Concepts
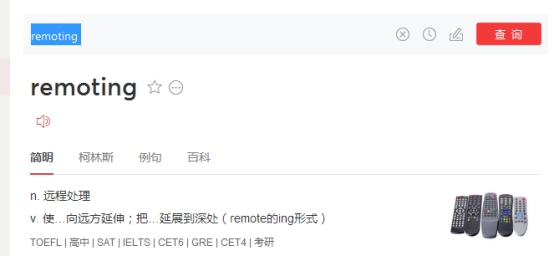
1. A distributed processing method.Literally, he is a remote object development technology based on.net platform, which treats data in remote computers as distributed objects for development.
2. A network communication technology.Since this technology can operate data from a remote computer, of course it is network communication technology.
2. Technologies used by.NET Remoting
1,Xml
2,SOAP
SOAP=Simple Object Transfer Protocol.
3. Serialization
-Binary
-xml
3..NET Remoting Principle
Communication between networks through channels.
1. First access the channel through Remoting to get the server access object
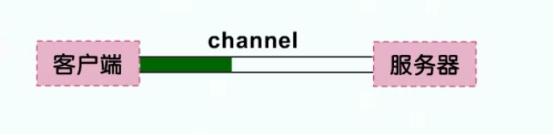
2. Resolve as client object through proxy
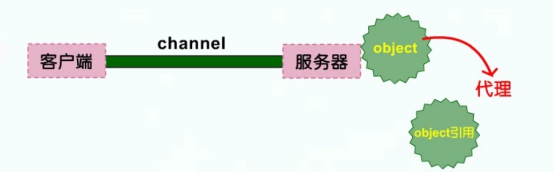
3. You can then manipulate this server object on the client side.
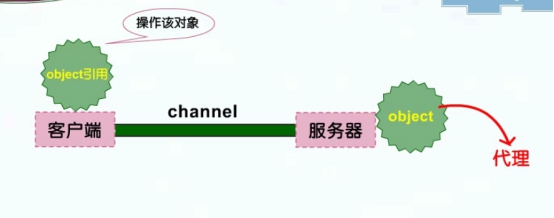
To get server-side objects, the client only needs to know the type of channel (TcpChannel and HttpChannel) and the port number on the server side, not the format of the packet.
It is important to note that when a client acquires a service-side object, it does not acquire the actual object on the service-side, but acquires its reference, which not only guarantees the loose coupling of related objects on the client-side and the server-side, but also optimizes the performance of communication.
4. Key elements in.NET Remoting
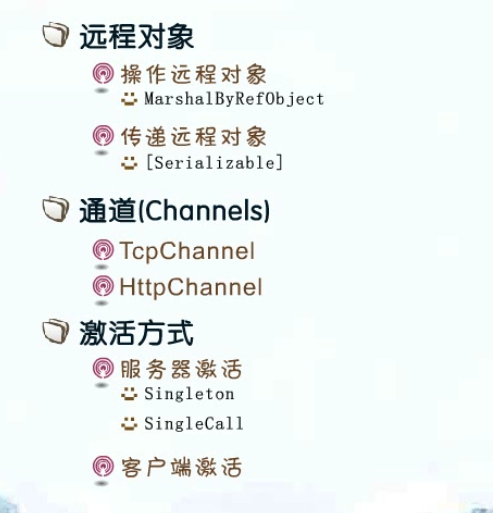
4.1 Remote Objects
Remote objects are the core element of.net remoting and are divided into two types
1. Operating remote objects
2. Passing Remote Objects
Operating on remote objects means that objects are running remotely and clients send operation messages by reference.This remote object must be a derived class of the MarshlByRefObject class in order to pass its object reference to the client.
Delivering a remote object means copying the remote object locally, the client operates on it, and then sending a copy back to the server. Such an operation object must be marked [Serializable]
4.2 Channels
It is divided into TcpChannel and HttpChannel.
TcpChannel uses binary format to serialize message objects, so it has better transmission performance.
HttpChannel uses SOAP format to serialize message objects, so it has better interoperability.
4.3 Activation Mode
What is the activation method?When working with remote objects, we need to consider a question: when and by whom the remote object is created, which depends on how it is activated.
There are two ways to activate: server activation and client activation.
It is mainly to learn how to activate the server.
Singleton
SingleCall
5..NET Remoting Development Steps
using System.Runtime.Remoting; using System.Runtime.Remoting.Channels; using System.Runtime.Remoting.Channels.Tcp; using System.Runtime.Remoting.Channels.Http;
5.1 Step 1: Create a remote object class
The remote object class must be derived from System.MarshlByRefObject.
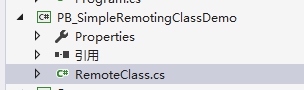
/* * Remote object class must derive from MarshalByRefObject */ public class RemoteClass : MarshalByRefObject { int num = 0; public RemoteClass() { Console.WriteLine("Activated RemoteClass Remote Object"); } public string Method(string name) { Console.WriteLine("No.{0}Second call, parameter is{1}", num++, name); return "hello " + name; } }
Step 2 of 5.2: Create a service-side host program to receive client requests
1. Registration Pipeline
2. Registration Server Activation Object Method
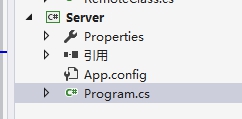
/* * System.Runtime.Remoting needs to be referenced */ class Program { static void Main(string[] args) { //1,Registration Pipeline TcpChannel tcpChannel = new TcpChannel(10000);//Port Specification HttpChannel httpChannel = new HttpChannel(10001); ChannelServices.RegisterChannel(tcpChannel, true); ChannelServices.RegisterChannel(httpChannel, false); //2,Registration Server Activation Mode //WellKnownObjectMode.Singleton Indicates that the generated instance is a singleton pattern //WellKnownObjectMode.SingleCall Indicates that each incoming message is a new object instance RemotingConfiguration.RegisterWellKnownServiceType(typeof(RemoteClass), "HelloTest", WellKnownObjectMode.SingleCall); Console.WriteLine("Here is the server-side host program"); Console.Read(); } }
5.3 Step 3: Create a client and invoke a remote object
1. Registration Channel
2. Obtain object proxy from URL
3. Call remote objects using proxy
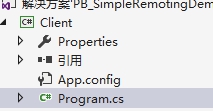
class Program { static void Main(string[] args) { #region TCP mode /* //1,Registration Channel TcpChannel tcpChannel = new TcpChannel();//Client does not need to specify port number ChannelServices.RegisterChannel(tcpChannel, true); //2,Create proxy RemoteClass rc = (RemoteClass)Activator.GetObject(typeof(RemoteClass), "tcp://localhost:10000/HelloTest");//1000 The port number is specified on the server side if (rc == null) { Console.WriteLine("Could not locate TCP Server"); } Console.WriteLine("TCP Method {0}, rc.Method ("Zhang Fei"); //*/ #endregion #region Http mode HttpChannel httpChannel = new HttpChannel(); ChannelServices.RegisterChannel(httpChannel, false); RemoteClass object2 = (RemoteClass)Activator.GetObject(typeof(RemoteClass), "http://localhost:10001/HelloTest"); if (object2 == null) { Console.WriteLine("Could not locate HTTP Server"); } Console.WriteLine("HTTP mode{0}", object2.Method("Guan Yu")); #endregion Console.Read(); } }
5.4 Debugging
1. Set Server as Startup Project
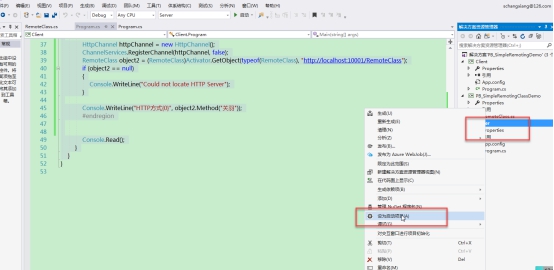
2. f5 Start Server
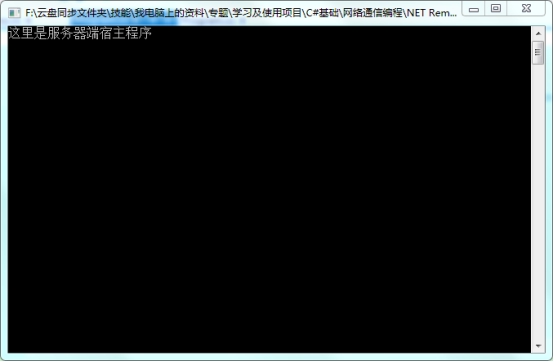
4. Start client
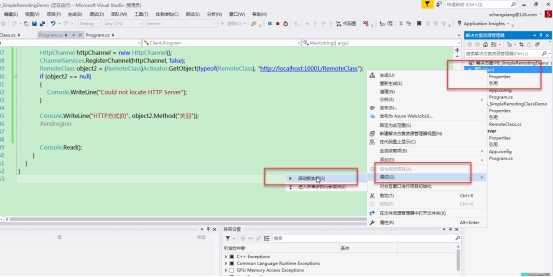
Effect:
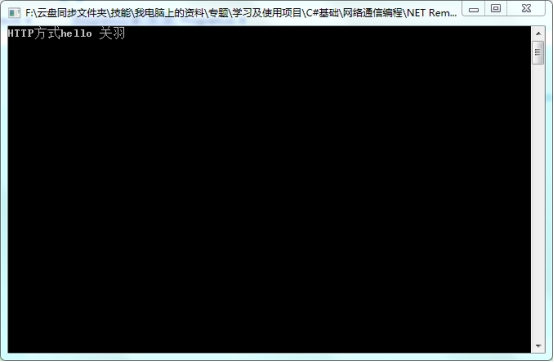
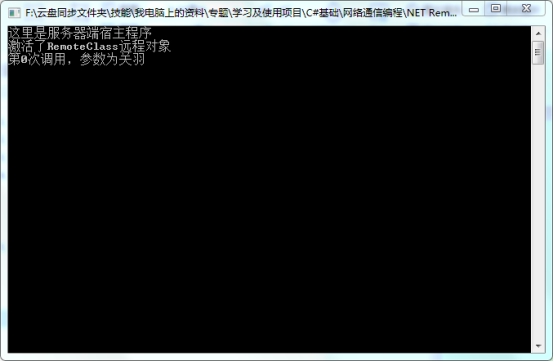
6..NET Remoting Profile
Register without code, use profile
Server:
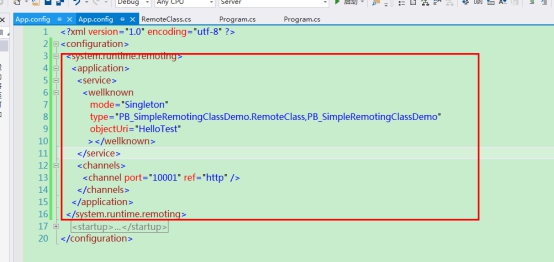
RemotingConfiguration.Configure(AppDomain.CurrentDomain.SetupInformation.ConfigurationFile, true);
Client:
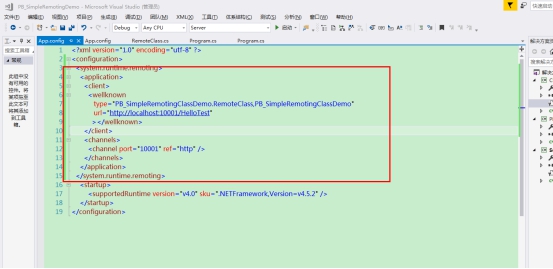
RemotingConfiguration.Configure(AppDomain.CurrentDomain.SetupInformation.ConfigurationFile, true);
RemoteClass obj = new RemoteClass();
Console.WriteLine("HTTP mode {0}", obj.Method("mascot"));