Nowadays, the application scenarios of message queuing are more and more large. RabbmitMQ and KafKa are commonly used.
We use BlockingCollection to implement a simple message queue.
Implement message queuing
Create a console application with Vs2017. Create DemoQueueBlock class to encapsulate some common judgments.
HasEle, judge whether there is element
Add add element to queue
Take takes elements from the queue
To avoid exposing BlockingCollection directly to users, we encapsulate a DemoQueueBlock class
/// <summary> ///BlockingCollection demo message queue /// </summary> /// <typeparam name="T"></typeparam> public class DemoQueueBlock<T> where T : class { private static BlockingCollection<T> Colls; public DemoQueueBlock() { } public static bool IsComleted() { if (Colls != null && Colls.IsCompleted) { return true; } return false; } public static bool HasEle() { if (Colls != null && Colls.Count>0) { return true; } return false; } public static bool Add(T msg) { if (Colls == null) { Colls = new BlockingCollection<T>(); } Colls.Add(msg); return true; } public static T Take() { if (Colls == null) { Colls = new BlockingCollection<T>(); } return Colls.Take(); } } /// <summary> //Message body /// </summary> public class DemoMessage { public string BusinessType { get; set; } public string BusinessId { get; set; } public string Body { get; set; } }
Add element to queue
Add elements through the console
//Additive elements while (true) { Console.WriteLine("Please enter the queue"); var read = Console.ReadLine(); if (read == "exit") { return; } DemoQueueBlock<DemoMessage>.Add(new DemoMessage() { BusinessId = read }); }
Consumption queue
Determine whether to get the queue by judging iscompleted
Task.Factory.StartNew(() => { //Take elements from the queue. while (!DemoQueueBlock<DemoMessage>.IsComleted()) { try { var m = DemoQueueBlock<DemoMessage>.Take(); Console.WriteLine("Consumed:" + m.BusinessId); } catch (Exception ex) { Console.WriteLine(ex.Message); } } });
View run results
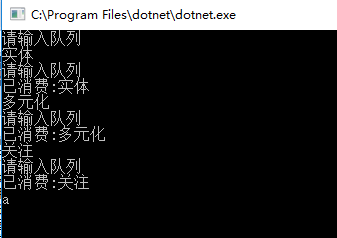
Operation result
In this way, we have implemented a simple message queue.