The main process of nodejs is single threaded, but it has a multi-threaded processing scheme (more ready, multi process scheme), that is, the main process starts different sub processes, the main process receives all requests, and then distributes them to other different nodejs sub processes for processing.
It generally has two implementations:
- The main process listens to a port, and the sub process does not listen to the port, and distributes requests to the sub process through the main process;
- The main process and sub process listen to different ports respectively, and distribute requests to sub processes through the main process.
cluster mode
The cluster mode of Nodejs uses the first implementation. It uses a main thread master and multiple sub thread worker s to form a cluster and distribute requests to sub threads through the main thread. Cluster implements the control of child_ The encapsulation of process realizes the multi process model by creating sub processes through fork method.
Use of cluster
http, cluster and process are built-in modules of nodejs, and no additional installation is required
- Create an http service
// http is a nodejs built-in module const http = require('http') const server = http.createServer((req, res) => { res.write('hello http!') res.end() }) server.listen(3030, () => { console.log('server is listening on http://localhost:3030') }) // Process is the process module of node, from which you can obtain the process information and control the process console.log(`worker ${process.pid} start`)

- Create a cluster In the following program, it will first judge whether there is a main process. If not, it will create a process. It will default to the first process as the main process
- In the source code, you call cluster When using the fork () method, the setupPrimary method will be executed to create the main process. It will use the initialized flag to determine whether it is true and whether it is created for the first time. If so, the main process will be created, otherwise it will be skipped
- Use createWorkerProcess to create a child process. This method actually uses child_process to create a child process
const cluster = require('cluster') // Number of child processes open const workerNum = 3; // If it is the main process if(cluster.isMaster) { // Create child process for(let i = 0; i < workerNum; i++) { // Through cluster Fork create child process cluster.fork() } // If there are child processes, start the related services. Here, three processes will be used to perform the http service demonstration }else { require('./http-server') }

The implementation process is like this: the cluster module applies child_process to create a child process. The child process overwrites the cluster_ Getserver method, so that in server Listen to ensure that only the main process listens to the port, and the main sub process communicates through IPC. Secondly, the main process applies two different modules (round_robin_handle.js and shared_handle.js) to distribute requests to the sub process for processing according to different platforms or protocols.
PM2
PM2 is a background process manager. It is a mature application of multi process scheme, which can help manage and keep applications online.
Basic use
Global installation: npm install pm2@latest -g It is also very simple to use:
- Start (http-server.js is the program to start): PM2 start http-server js
- Restart (program): restart app_name
- Reload (configuration and program): reload app_name
- Stop: pm2 stop app_name
- Delete: pm2 delete app_name
- Monitoring mode: PM2 start XX js --watch
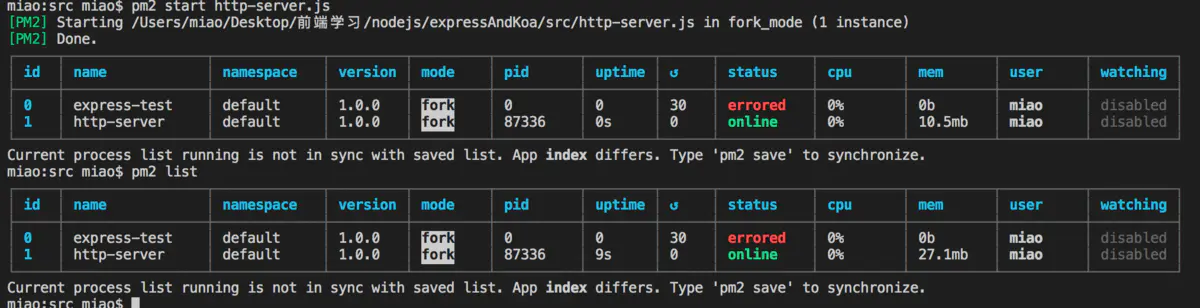
Load balancing:
PM2 for nodejs application can automatically realize load balancing according to the system: PM2 start HTTP server js -i max

PM2 configuration
We certainly don't want to manually input a bunch of instructions every time we start, so we can manage these configurations in a unified configuration file. Note that the JS file name must be XXX config. JS, I use ecosystem config. js: In the apps array, you can place multiple objects and perform different configurations corresponding to multiple files
// ecosystem.config.js module.exports = { apps : [{ name: "http-server", // Start process name script: "./src/http-server.js", // Startup file instances: 4, // Number of processes started exec_mode: 'cluster', // Multi process and multi instance // Setting environment configuration for different environments // Development environment, corresponding to the parameters after -- env env_development: { NODE_ENV: "dev", watch: true, // The development environment uses true, and other settings are false }, // testing environment env_testing: { NODE_ENV: "test", watch: false, // The development environment uses true, and other settings are false }, // production environment env_production: { NODE_ENV: "prod", watch: false, // The development environment uses true, and others must be set to false }, // Log date format log_date_format: 'YYYY-MM-DD HH:mm Z', // The error log file must be set in a directory outside the project for testing error_file: '~/Desktop/logs/err.log', // Flow log, including console Log, which must be set in a directory outside the project for testing out_file: '~/Desktop/logs/info.log', // The maximum restart data. When the application is determined to be unstable for n consecutive times, restart it again max_restarts: 10, },{ name: "express-test", // Start process name script: "./src/express-test.js", // Startup file instances: 4, // Number of processes started exec_mode: 'cluster', // Multi process and multi instance }] }
Execution configuration: PM2 start ecosystem config. js --env dev
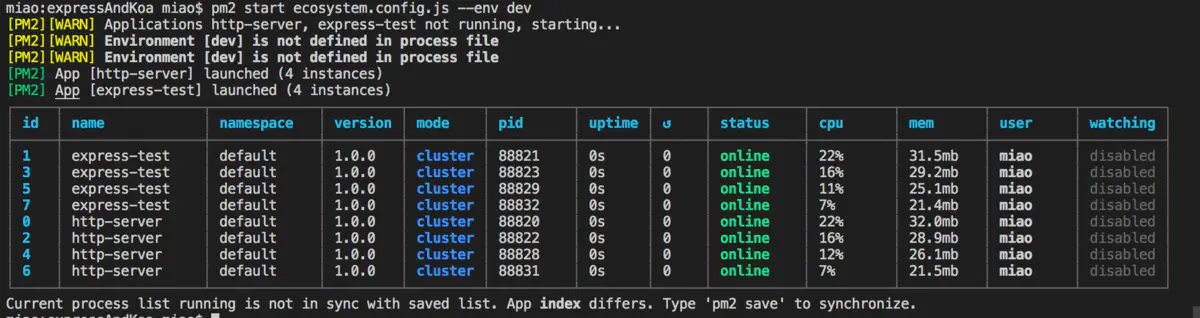
You can see that after startup, pipelining and error logs are generated on the desktop:
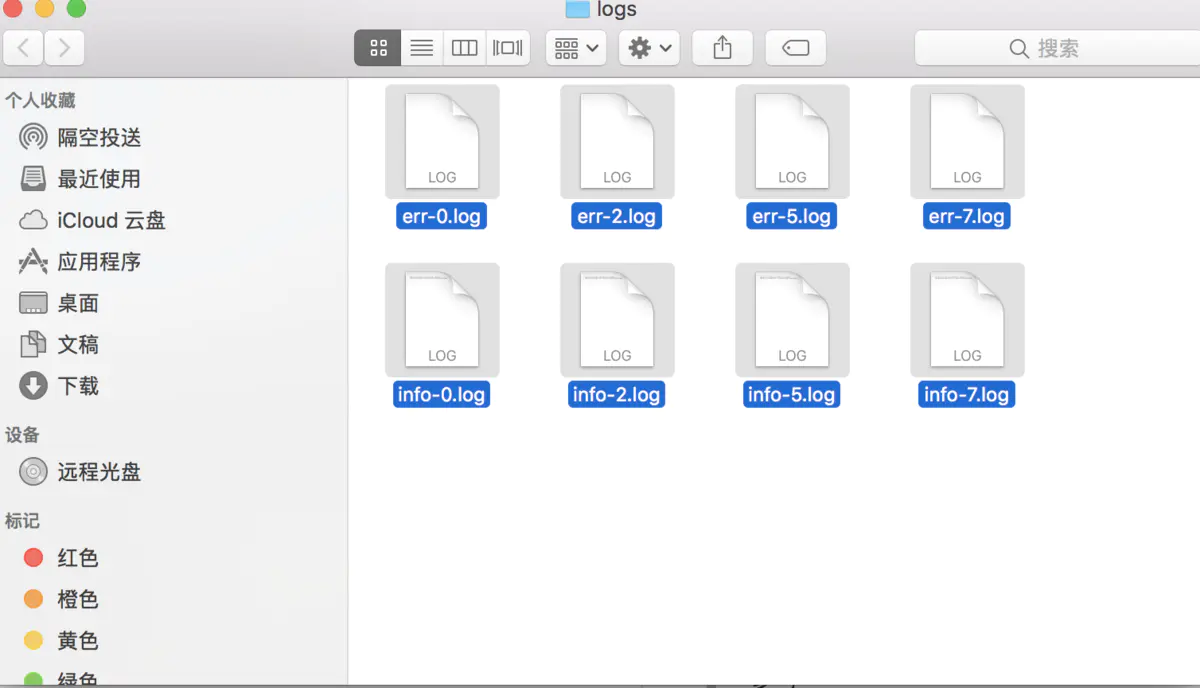
journal
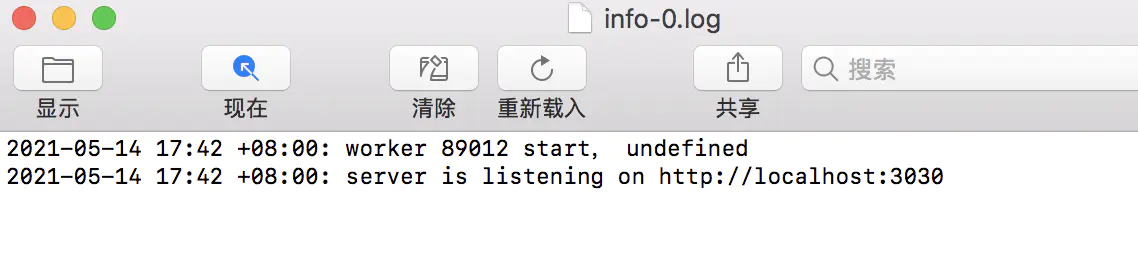
Flow log
reference resources: Node Process module API: http://nodejs.cn/api/process.html pm2 official website: https://pm2.keymetrics.io/docs/usage/pm2-doc-single-page/