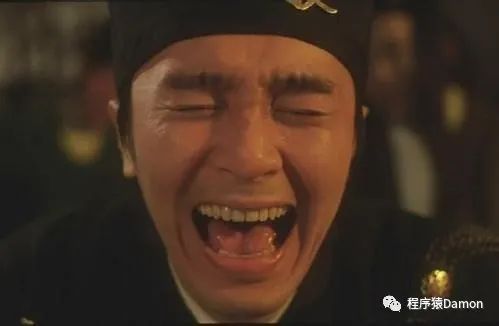
difference
- Ribbon
Ribbon is a load balancer released by Netflix, which helps to control the behavior of HTTP and TCP clients. Ribbon belongs to client load balancing. As we all know, when we first used the Springcloud microservice architecture, we used the honorary product of Netflix: https://docs.spring.io/spring-cloud-netflix/docs/2.2.9.RELEASE/reference/html/ . Unfortunately, Eureka has long been officially abandoned and no longer updated. This may be for a better unified architecture.
- Spring-cloud-loadbalancer
Spring cloud loadbalancer is a new load balancer officially launched. As early as 2017, spring began to try to develop spring cloud loadbalancer instead of ribbon. The project was hosted in the spring cloud incubator. After a period of time, the project was suddenly marked as archive and migrated to spring cloud commons, indicating that the official's determination to unify the public infrastructure is moving forward step by step.
As early as Spring Cloud Hoxton.M2, the first spring cloud loadbalancer was integrated to replace the old ribbon:
Spring Cloud Hoxton.M2 is the first release containing both blocking and non-blocking load balancer client implementations as an alternative to Netflix Ribbon which has entered maintenance mode. To use the new `BlockingLoadBalancerClient` with a `RestTemplate` you will need to include `org.springframework.cloud:spring-cloud-loadbalancer` on your application's classpath. The same dependency can be used in a reactive application when using `@LoadBalanced WebClient.Builder` - the only difference is that Spring Cloud will auto-configure a `ReactorLoadBalancerExchangeFilterFunction` instance. See the [documentation](https://cloud.spring.io/spring-cloud-static/spring-cloud-commons/2.2.0.M2/reference/html/#_spring_resttemplate_as_a_load_balancer_client) for additional information. The new `ReactorLoadBalancerExchangeFilterFunction` can also be autowired and passed directly to `WebClient.Builder` (see the [documentation](https://cloud.spring.io/spring-cloud-commons/reference/html/#webflux-with-reactive-loadbalancer)). For all these features, [Project Reactor](https://projectreactor.io/)-based `RoundRobinLoadBalancer` is used underneath.
It can be seen from the original text that currently only BlockingLoadBalancerClient is supported, which is also based on RestTemplate. We know that ribbon is also based on RestTemplate:
@LoadBalanced @Bean public RestTemplate restTemplate() { SimpleClientHttpRequestFactory requestFactory = new SimpleClientHttpRequestFactory(); requestFactory.setReadTimeout(env.getProperty("client.http.request.readTimeout", Integer.class, 15000)); requestFactory.setConnectTimeout(env.getProperty("client.http.request.connectTimeout", Integer.class, 3000)); RestTemplate rt = new RestTemplate(requestFactory); return rt; }
However, ribbon is obviously more sophisticated in configuration:
backend: ribbon: client: enabled: true ServerListRefreshInterval: 5000 ribbon: ConnectTimeout: 3000 ReadTimeout: 1000 eager-load: enabled: true clients: cas-server,customer-server MaxAutoRetries: 2 MaxAutoRetriesNextServer: 3 OkToRetryOnAllOperations: true NFLoadBalancerRuleClassName: com.netflix.loadbalancer.RoundRobinRule
It can be configured in multiple dimensions: timeout, refresh service list, retry mechanism, etc.
But the spring cloud loadbalancer can not be so good. After all, it is a newly raised cub. However, the spring cloud loadbalancer, which supports both blocking and non blocking load balancers, is introduced in the Spring Cloud Hoxton version for the first time as the Netflix Ribbon that has entered the maintenance state. Next, let's see how to use it.
Actual spring cloud loadbalancer
When using, we learned from the original text that we only need to introduce org.springframework.cloud: spring cloud loadbalancer dependency to use the new BlockingLoadBalancerClient with RestTemplate. At the same time, the introduction of this dependency will also support Reactive applications. Like other uses, you only need to use @ LoadBalanced to modify WebClient.Builder.
Let's first introduce dependencies. Here we use Nacos based service registration and discovery. Let's first inject dependencies:
<dependency> <groupId>com.alibaba.cloud</groupId> <artifactId>spring-cloud-starter-alibaba-nacos-discovery</artifactId> <exclusions> <exclusion> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-netflix-ribbon</artifactId> </exclusion> </exclusions> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-loadbalancer</artifactId> </dependency>
Here, we use a new load balancer. We need to exclude the ribbon dependency, otherwise the loadbalancer is invalid. At the same time, we need to disable the load balancing capability of ribbon:
spring: cloud: loadbalancer: ribbon: enabled: false
After disabling, we use it in combination with RestTemplate and use @ LoadBalanced to decorate WebClient.Builder.
@LoadBalanced//You can't request other services in the form of ip @Bean public RestTemplate restTemplate() { SimpleClientHttpRequestFactory requestFactory = new SimpleClientHttpRequestFactory(); requestFactory.setReadTimeout(env.getProperty("client.http.request.readTimeout", Integer.class, 15000)); requestFactory.setConnectTimeout(env.getProperty("client.http.request.connectTimeout", Integer.class, 3000)); RestTemplate rt = new RestTemplate(requestFactory); return rt; }
The careful student can begin to see that this configuration is the same as that of Ribbon. At this point, we can start the service provider and consumer to test. I won't show it here.
summary
According to the official incubation, the new load balancer will replace the old ribbon. After all, it introduces a new function: Reactive and adds strong support for it. This improves performance.
Meanwhile, spring cloud loadbalancer still has some limitations, such as:
- ribbon provides several default load balancing policies
- Currently, spring cloud loadbalancer only supports the configuration of retry operation
- ribbon supports timeout, lazy load processing, retry and its integration with hystrix advanced properties
In the spring cloud system, Ribbon is still used in most areas, but based on spring cloud-k8s, spring cloud starter kubernetes loadbalancer may be used. As I have practiced before, LB based on Ribbon cannot realize mutual access between services across namespaces.