Process oriented & object oriented
- Process oriented thought
- The steps are clear and simple. What to do in the first step and what to do in the second step
- Facing the process, it is suitable to deal with some relatively simple problems
- Object oriented thought
- Birds of a feather flock together in a classified thinking mode. When thinking about problems, we will first solve what classifications are needed, and then think about these classifications separately. Finally, the process oriented thinking is carried out on the details under a certain classification
- Object oriented is suitable for dealing with complex problems and problems requiring multi person cooperation
- For describing complex transactions, in order to grasp the macro and analyze them reasonably as a whole, we need to use object-oriented thinking to analyze the whole system. However, when it comes to micro operation, it still needs process oriented thinking to deal with it
What is object oriented
- Object oriented programming (OOP)
- The essence of object-oriented programming is to organize code in the form of class and organize (encapsulate) data in the form of object
- abstract
- Three characteristics:
- encapsulation
- inherit
- polymorphic
- From the perspective of epistemology, there are objects before classes. Objects are concrete things. Class is abstract, which is the abstraction of objects
- From the perspective of code operation, there are classes before objects. A class is a template for an object
Method review
- Definition of method
- Modifier
- Return type
- break: jump out of switch and end the difference between loop and return
- Method name: pay attention to the specification. See the meaning of the name
- Parameter list: (parameter type, parameter name)
- Exception throw
package com.oop.demo01;
import java.io.IOException;
//Demo01 class
public class Demo01 {
//main method
public static void main(String[] args) {
}
/*
Method definition:
Modifier return value type method name (...) {
//Method body
return Return value;
}
*/
//return ends the method and returns a result
//Specific size
public String sayHello(){
return "hello,word";
}
public int max(int a,int b){
return a>b?a:b;//Ternary operator
}
//Array subscript out of bounds: Arrayindexoutofbounds
//Thrown when reading file throws
public void readFile(String file) throws IOException{
}
}
- Method call: recursion
- Static method
- Non static method
- Formal and argument
- Value passing and reference passing
- this keyword
package com.oop.demo01;
public class Demo02 {
public static void main(String[] args) {
// Student.say();
//Non statically instantiate this class new
//Object type object name = object value;
Student student = new Student();
student.say();
}
//Static a and class are loaded together. When the class exists, it already exists
public static void a(){
// b();
}
//Class b does not exist until it is instantiated
public void b(){
}
}
package com.oop.demo01;
//Class students
public class Student {
//Non static method
public void say(){
System.out.println("Students speak");
}
}
package com.oop.demo01;
public class Demo03 {
public static void main(String[] args) {
//The actual parameter and the type of the actual parameter should correspond
// int add = Demo03.add(1, 2);
int add = new Demo03().add(2, 3);
System.out.println(add);
}
public int add(int a,int b){
return a+b;
}
}
package com.oop.demo01;
//pass by value
public class Demo04 {
public static void main(String[] args) {
int a=1;//definition
System.out.println(a);//1
Demo04.change(a);//call
System.out.println(a);//1
}
//void return value is null
public static void change(int a){
a=10;
}
}
package com.oop.demo01;
//Reference passing: object, essence or value passing
//Object, memory
public class Demo05 {
public static void main(String[] args) {
Person person = new Person();
System.out.println(person.name);//null
Demo05.change(person);
System.out.println(person.name);//Write and read
}
//change method
public static void change(Person person){
//Person is an object: the person pointed to is person = new person(); Is a specific person who can change attributes
person.name="Write and read";
}
}
//Define a Person class with an attribute: name
class Person{
String name;//null
}
Relationship between class and object
- Class is an abstract data type. It is an overall description / definition of something, but it can not represent a specific thing
- Animals, plants, mobile phones, computers
- Person class, Pet class, Car class, etc. these classes are used to describe / define the characteristics and behavior of a specific thing
- Objects are concrete instances of abstract concepts
- Zhang San is a specific example of people, and the prosperous wealth of Zhang San's family is a specific example of dogs
- It is a concrete instance rather than an abstract concept that can reflect the characteristics and functions
Creating and initializing objects
- Create an object using the new keyword
- When using the new keyword, in addition to allocating memory space, the created object will be initialized by default and the constructor in the class will be called
- Constructors in classes, also known as construction methods, must be called when creating objects
- Characteristics of constructor:
- Must be the same as the name of the class
- There must be no return type and void cannot be written
package com.oop.demo02;
//Student class
public class Student {
//Properties: Fields
String name;//null
int age;//null
//method
public void study(){
//this: represents the current class
System.out.println(this.name+"I'm learning");
}
}
package com.oop.demo02;
//There should be only one main method in a project
public class Application {
public static void main(String[] args) {
//Class: abstract, instantiated
//Class instantiation will return an object of its own
//A student object is a concrete instance of a student class
Student xiaoming = new Student();
Student xiaohong = new Student();
xiaoming.name="Xiao Ming";
xiaoming.age=10;
System.out.println(xiaoming.name);
System.out.println(xiaoming.age);
xiaohong.name="Xiao Hong";
xiaohong.age=10;
System.out.println(xiaohong.name);
System.out.println(xiaohong.age);
}
}
constructor
package com.oop.demo02;
//There should be only one main method in a project
public class Application {
public static void main(String[] args) {
//new instantiates an object
Person person = new Person("Write",12);
System.out.println(person.name);
System.out.println(person.age);
}
}
package com.oop.demo02;
//java--->class
public class Person {
//Even if a class doesn't write anything, it will have a method
//Display definition constructor
String name;
int age;
//The constructor must have the same name as the class
//Nonparametric structure
//Instantiation initial value
/*
Constructor function:
1.When using the new keyword, there must be a constructor, which is essentially calling the constructor
2.Used to initialize values
*/
public Person(){
this.name="Write and read";
}
//Once a parameterized structure is defined, the definition must be displayed if there is no parameter
public Person(String name,int age){
this.name=name;
this.age=age;
}
//alt+insert: one key generation constructor
}
/*
public static void main(String[] args) {
//new Instantiated an object
Person person = new Person("Write down ", 12);
System.out.println(person.name);
System.out.println(person.age);
}
Constructor features:
1.Same as class name
2.no return value
effect:
1.new The essence is to call the constructor
2.Initializes the value of the object
be careful:
1.After defining a parameterized construct, to use a parameterless construct, you must define a parameterless construct
Generate constructor shortcut key: alt+insert
this.Represents the current class =: the value passed in later
*/
package com.oop;
import com.oop.demo03.Pet;
public class Application {
public static void main(String[] args) {
Pet dog = new Pet();
dog.name="chinese rhubarb";
dog.age=2;
dog.shout();
System.out.println(dog.name);
System.out.println(dog.age);
Pet cat = new Pet();
}
}
/*
1.Classes and objects
A class is a template: abstract, and an object is a concrete instance
2.method
Define and call
3.Object reference
Reference type: the basic type (8) is a reference type except the basic type
Objects are operated by reference: stack -- > heap (address)
4.Property: the field file member variable is initialized by default
Number: 0.0
char:u0000
boolean:false
Reference: null
Modifier attribute type attribute name = attribute value
5.Object creation and use
(1)You must use the new keyword to create an object, and you also need the constructor Person tao=new Person();
(2)Object's properties name
(3)Object sleep()
6.class
Static properties
Dynamic behavior method
*/
package com.oop.demo03;
//Pet: pets
//Shoot: call
public class Pet {
public String name;
public int age;
//Nonparametric structure
public void shout(){
System.out.println("Let out a cry");
}
}
Create object memory analysis
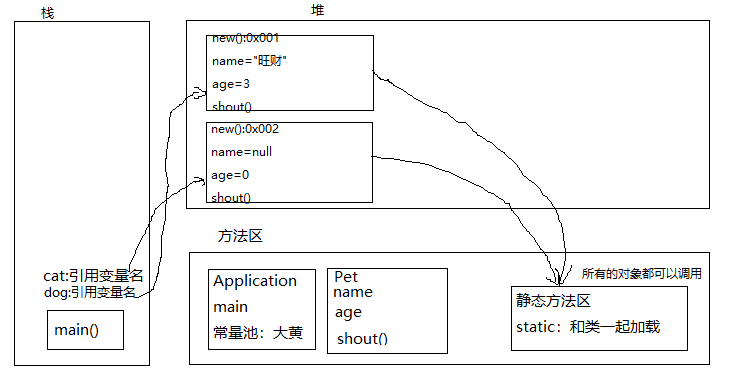